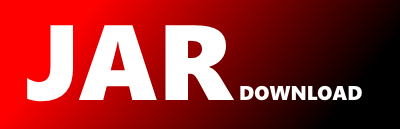
com.laamella.parameter_source.ObjectParameterSource Maven / Gradle / Ivy
package com.laamella.parameter_source;
import java.util.Optional;
/**
* A parameter source that stores objects.
* If a retrieve value is not of the requested type,
* conversion will be attempted.
*/
public interface ObjectParameterSource extends ParameterSource {
default Optional getOptionalString(String key) {
return getOptionalObject(key).map(Object::toString);
}
default Optional getOptionalInteger(String key) {
Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy