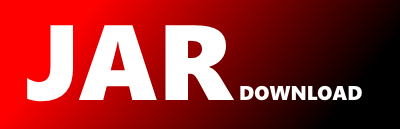
com.landawn.abacus.util.JSONUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-android Show documentation
Show all versions of abacus-android Show documentation
A general programming library in Java
/*
* Copyright (c) 2015, Haiyang Li.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.landawn.abacus.util;
import java.lang.reflect.Array;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.landawn.abacus.parser.ParserUtil;
import com.landawn.abacus.parser.ParserUtil.EntityInfo;
import com.landawn.abacus.parser.ParserUtil.PropInfo;
import com.landawn.abacus.type.Type;
/**
*
* @since 0.8
*
* @author Haiyang Li
*/
public final class JSONUtil {
private JSONUtil() {
// singleton.
}
public static JSONObject wrap(final Map map) {
return new JSONObject(map);
}
/**
* wrap(entity) -> wrap(Maps.deepEntity2Map(entity, true))
*
* @param entity
* @return
* @see Maps#deepEntity2Map(Object)
*/
@SuppressWarnings("unchecked")
public static JSONObject wrap(final Object entity) {
return new JSONObject(entity instanceof Map ? (Map) entity : Maps.deepEntity2Map(entity, true));
}
public static JSONArray wrap(final boolean[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final char[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final byte[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final short[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final int[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final long[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final float[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final double[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final Object[] array) throws JSONException {
return new JSONArray(array);
}
public static JSONArray wrap(final Collection> coll) {
return new JSONArray(coll);
}
public static Map unwrap(final JSONObject jsonObject) throws JSONException {
return unwrap(Map.class, jsonObject);
}
public static T unwrap(final Class extends T> cls, final JSONObject jsonObject) throws JSONException {
return unwrap(N. typeOf(cls), jsonObject);
}
@SuppressWarnings("unchecked")
public static T unwrap(Type extends T> type, final JSONObject jsonObject) throws JSONException {
type = type.clazz().equals(Object.class) ? N. typeOf("Map") : type;
final Class> cls = type.clazz();
if (type.clazz().isAssignableFrom(JSONObject.class)) {
return (T) jsonObject;
} else if (type.isMap()) {
final Map map = (Map) N.newInstance(cls);
final Iterator iter = jsonObject.keys();
final Type> valueType = type.getParameterTypes()[1];
String key = null;
Object value = null;
while (iter.hasNext()) {
key = iter.next();
value = jsonObject.get(key);
if (value == JSONObject.NULL) {
value = null;
} else if (value != null) {
if (value instanceof JSONObject) {
value = unwrap(valueType, (JSONObject) value);
} else if (value instanceof JSONArray) {
value = unwrap(valueType, (JSONArray) value);
}
}
map.put(key, value);
}
return (T) map;
} else {
final Object entity = N.newEntity(cls, null);
final EntityInfo entityInfo = ParserUtil.getEntityInfo(cls);
final Iterator iter = jsonObject.keys();
String key = null;
Object value = null;
PropInfo propInfo = null;
while (iter.hasNext()) {
key = iter.next();
value = jsonObject.get(key);
propInfo = entityInfo.getPropInfo(key);
value = jsonObject.get(key);
if (value == JSONObject.NULL) {
value = null;
} else if (value != null) {
if (value instanceof JSONObject) {
value = unwrap(propInfo.type, (JSONObject) value);
} else if (value instanceof JSONArray) {
value = unwrap(propInfo.type, (JSONArray) value);
}
}
propInfo.setPropValue(entity, value);
}
return (T) entity;
}
}
public static List unwrap(final JSONArray jsonArray) throws JSONException {
return unwrap(List.class, jsonArray);
}
/**
*
* @param cls
* array or collection class
* @param jsonArray
* @return
*/
public static T unwrap(final Class extends T> cls, final JSONArray jsonArray) throws JSONException {
return unwrap(N. typeOf(cls), jsonArray);
}
@SuppressWarnings("unchecked")
public static T unwrap(Type extends T> type, final JSONArray jsonArray) throws JSONException {
type = type.clazz().equals(Object.class) ? N. typeOf("List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy