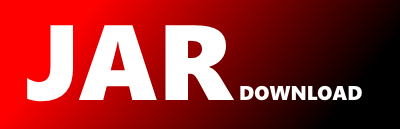
com.landawn.abacus.util.Optional Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-android Show documentation
Show all versions of abacus-android Show documentation
A general programming library in Java
/*
* Copyright (c) 2017, Haiyang Li.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.landawn.abacus.util;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.Set;
import com.landawn.abacus.util.function.Supplier;
import com.landawn.abacus.util.stream.Stream;
public final class Optional {
private static final Optional> EMPTY = new Optional<>();
private final T value;
private Optional() {
this.value = null;
}
private Optional(T value) {
this.value = Objects.requireNonNull(value);
}
public static Optional empty() {
return (Optional) EMPTY;
}
public static Optional of(T value) {
return new Optional<>(value);
}
public static Optional ofNullable(T value) {
if (value == null) {
return empty();
}
return new Optional<>(value);
}
public static Optional from(java.util.Optional optional) {
return optional.isPresent() ? of(optional.get()) : Optional. empty();
}
public T get() throws NoSuchElementException {
return orElseThrow();
}
public boolean isPresent() {
return value != null;
}
public boolean isEmpty() {
return value == null;
}
public void ifPresent(Try.Consumer super T, E> action) throws E {
Objects.requireNonNull(action);
if (isPresent()) {
action.accept(value);
}
}
public void ifPresentOrElse(Try.Consumer super T, E> action, Try.Runnable emptyAction) throws E, E2 {
Objects.requireNonNull(action);
Objects.requireNonNull(emptyAction);
if (isPresent()) {
action.accept(value);
} else {
emptyAction.run();
}
}
public Optional filter(Try.Predicate super T, E> predicate) throws E {
Objects.requireNonNull(predicate);
if (isPresent() && predicate.test(value)) {
return this;
} else {
return empty();
}
}
public Nullable map(final Try.Function super T, ? extends U, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return Nullable. of(mapper.apply(value));
} else {
return Nullable. empty();
}
}
public OptionalBoolean mapToBoolean(final Try.ToBooleanFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalBoolean.of(mapper.applyAsBoolean(value));
} else {
return OptionalBoolean.empty();
}
}
public OptionalChar mapToChar(final Try.ToCharFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalChar.of(mapper.applyAsChar(value));
} else {
return OptionalChar.empty();
}
}
public OptionalByte mapToByte(final Try.ToByteFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalByte.of(mapper.applyAsByte(value));
} else {
return OptionalByte.empty();
}
}
public OptionalShort mapToShort(final Try.ToShortFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalShort.of(mapper.applyAsShort(value));
} else {
return OptionalShort.empty();
}
}
public OptionalInt mapToInt(final Try.ToIntFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalInt.of(mapper.applyAsInt(value));
} else {
return OptionalInt.empty();
}
}
public OptionalLong mapToLong(final Try.ToLongFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalLong.of(mapper.applyAsLong(value));
} else {
return OptionalLong.empty();
}
}
public OptionalFloat mapToFloat(final Try.ToFloatFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalFloat.of(mapper.applyAsFloat(value));
} else {
return OptionalFloat.empty();
}
}
public OptionalDouble mapToDouble(final Try.ToDoubleFunction super T, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return OptionalDouble.of(mapper.applyAsDouble(value));
} else {
return OptionalDouble.empty();
}
}
public Optional flatMap(Try.Function super T, Optional, E> mapper) throws E {
Objects.requireNonNull(mapper);
if (isPresent()) {
return Objects.requireNonNull(mapper.apply(value));
} else {
return empty();
}
}
public Optional or(Try.Supplier, E> supplier) throws E {
Objects.requireNonNull(supplier);
if (isPresent()) {
return this;
} else {
return Objects.requireNonNull((Optional) supplier.get());
}
}
public T orNull() {
return isPresent() ? value : null;
}
public T orElse(T other) {
return isPresent() ? value : other;
}
public T orElseGet(Try.Supplier extends T, E> other) throws E {
if (isPresent()) {
return value;
} else {
return other.get();
}
}
// public T orElseNull() {
// return isPresent() ? value : null;
// }
public T orElseThrow() throws NoSuchElementException {
if (isPresent()) {
return value;
} else {
throw new NoSuchElementException("No value is present");
}
}
public T orElseThrow(Supplier extends X> exceptionSupplier) throws X {
if (isPresent()) {
return value;
} else {
throw exceptionSupplier.get();
}
}
public Stream stream() {
if (isPresent()) {
return Stream.of(value);
} else {
return Stream. empty();
}
}
public java.util.Optional __() {
return isPresent() ? java.util.Optional.of(value) : java.util.Optional. empty();
}
public List toList() {
if (isPresent()) {
return N.asList(value);
} else {
return new ArrayList<>();
}
}
public Set toSet() {
if (isPresent()) {
return N.asSet(value);
} else {
return new HashSet<>();
}
}
public ImmutableList toImmutableList() {
if (isPresent()) {
return ImmutableList.of(value);
} else {
return ImmutableList. empty();
}
}
public ImmutableSet toImmutableSet() {
if (isPresent()) {
return ImmutableSet.of(value);
} else {
return ImmutableSet. empty();
}
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj instanceof Optional) {
final Optional> other = (Optional>) obj;
return N.equals(value, other.value);
}
return false;
}
@Override
public int hashCode() {
return N.hashCode(isPresent()) * 31 + N.hashCode(value);
}
@Override
public String toString() {
if (isPresent()) {
return String.format("Optional[%s]", value);
}
return "Optional.empty";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy