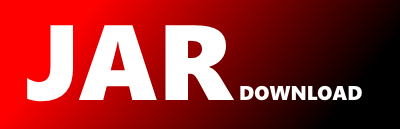
com.landawn.abacus.util.stream.AbstractLongStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-android Show documentation
Show all versions of abacus-android Show documentation
A general programming library in Java
/*
* Copyright (C) 2016 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.util.stream;
import java.util.Collection;
import java.util.HashSet;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Random;
import java.util.Set;
import java.util.concurrent.atomic.AtomicLong;
import com.landawn.abacus.util.Fn;
import com.landawn.abacus.util.IndexedLong;
import com.landawn.abacus.util.Joiner;
import com.landawn.abacus.util.LongIterator;
import com.landawn.abacus.util.LongList;
import com.landawn.abacus.util.LongMatrix;
import com.landawn.abacus.util.LongSummaryStatistics;
import com.landawn.abacus.util.Multiset;
import com.landawn.abacus.util.MutableLong;
import com.landawn.abacus.util.N;
import com.landawn.abacus.util.Nth;
import com.landawn.abacus.util.Optional;
import com.landawn.abacus.util.OptionalLong;
import com.landawn.abacus.util.Pair;
import com.landawn.abacus.util.Percentage;
import com.landawn.abacus.util.Try;
import com.landawn.abacus.util.function.BiConsumer;
import com.landawn.abacus.util.function.BiPredicate;
import com.landawn.abacus.util.function.BinaryOperator;
import com.landawn.abacus.util.function.Consumer;
import com.landawn.abacus.util.function.Function;
import com.landawn.abacus.util.function.LongBiFunction;
import com.landawn.abacus.util.function.LongBiPredicate;
import com.landawn.abacus.util.function.LongConsumer;
import com.landawn.abacus.util.function.LongFunction;
import com.landawn.abacus.util.function.LongPredicate;
import com.landawn.abacus.util.function.LongTriFunction;
import com.landawn.abacus.util.function.ObjLongConsumer;
import com.landawn.abacus.util.function.Predicate;
import com.landawn.abacus.util.function.Supplier;
import com.landawn.abacus.util.function.ToLongFunction;
/**
* This class is a sequential, stateful and immutable stream implementation.
*
* @since 0.8
*
* @author Haiyang Li
*/
abstract class AbstractLongStream extends LongStream {
AbstractLongStream(final boolean sorted, final Collection closeHandlers) {
super(sorted, closeHandlers);
}
@Override
public LongStream flattMap(final LongFunction mapper) {
return flatMap(new LongFunction() {
@Override
public LongStream apply(long t) {
return LongStream.of(mapper.apply(t));
}
});
}
@Override
public Stream flattMapToObj(final LongFunction extends Collection> mapper) {
return flatMapToObj(new LongFunction>() {
@Override
public Stream apply(long t) {
return Stream.of(mapper.apply(t));
}
});
}
@Override
public LongStream skip(final long n, final LongConsumer action) {
N.checkArgNotNegative(n, "n");
if (n == 0) {
return this;
}
final LongPredicate filter = isParallel() ? new LongPredicate() {
final AtomicLong cnt = new AtomicLong(n);
@Override
public boolean test(long value) {
return cnt.getAndDecrement() > 0;
}
} : new LongPredicate() {
final MutableLong cnt = MutableLong.of(n);
@Override
public boolean test(long value) {
return cnt.getAndDecrement() > 0;
}
};
return dropWhile(filter, action);
}
@Override
public LongStream removeIf(final LongPredicate predicate) {
N.checkArgNotNull(predicate);
return filter(new LongPredicate() {
@Override
public boolean test(long value) {
return predicate.test(value) == false;
}
});
}
@Override
public LongStream removeIf(final LongPredicate predicate, final LongConsumer action) {
N.checkArgNotNull(predicate);
N.checkArgNotNull(predicate);
return filter(new LongPredicate() {
@Override
public boolean test(long value) {
if (predicate.test(value)) {
action.accept(value);
return false;
}
return true;
}
});
}
@Override
public LongStream dropWhile(final LongPredicate predicate, final LongConsumer action) {
N.checkArgNotNull(predicate);
N.checkArgNotNull(action);
return dropWhile(new LongPredicate() {
@Override
public boolean test(long value) {
if (predicate.test(value)) {
action.accept(value);
return true;
}
return false;
}
});
}
@Override
public LongStream step(final long step) {
N.checkArgPositive(step, "step");
if (step == 1) {
return this;
}
final long skip = step - 1;
final LongIteratorEx iter = this.iteratorEx();
final LongIterator longIterator = new LongIteratorEx() {
@Override
public boolean hasNext() {
return iter.hasNext();
}
@Override
public long nextLong() {
final long next = iter.nextLong();
iter.skip(skip);
return next;
}
};
return newStream(longIterator, sorted);
}
@Override
public Stream split(final int size) {
return splitToList(size).map(new Function() {
@Override
public LongStream apply(LongList t) {
return new ArrayLongStream(t.array(), 0, t.size(), sorted, null);
}
});
}
@Override
public Stream split(final LongPredicate predicate) {
return splitToList(predicate).map(new Function() {
@Override
public LongStream apply(LongList t) {
return new ArrayLongStream(t.array(), 0, t.size(), sorted, null);
}
});
}
@Override
public Stream splitToList(final LongPredicate predicate) {
final BiPredicate predicate2 = new BiPredicate() {
@Override
public boolean test(Long t, Object u) {
return predicate.test(t);
}
};
return splitToList(null, predicate2, null);
}
@Override
public Stream split(final U seed, final BiPredicate super Long, ? super U> predicate, final Consumer super U> seedUpdate) {
return splitToList(seed, predicate, seedUpdate).map(new Function() {
@Override
public LongStream apply(LongList t) {
return new ArrayLongStream(t.array(), 0, t.size(), sorted, null);
}
});
}
@Override
public Stream sliding(final int windowSize, final int increment) {
return slidingToList(windowSize, increment).map(new Function() {
@Override
public LongStream apply(LongList t) {
return new ArrayLongStream(t.array(), 0, t.size(), sorted, null);
}
});
}
@Override
public LongStream collapse(final LongBiPredicate collapsible, final LongBiFunction mergeFunction) {
final LongIteratorEx iter = iteratorEx();
return newStream(new LongIteratorEx() {
private boolean hasNext = false;
private long next = 0;
@Override
public boolean hasNext() {
return hasNext || iter.hasNext();
}
@Override
public long nextLong() {
long res = hasNext ? next : (next = iter.nextLong());
while ((hasNext = iter.hasNext())) {
if (collapsible.test(next, (next = iter.nextLong()))) {
res = mergeFunction.apply(res, next);
} else {
break;
}
}
return res;
}
}, false);
}
@Override
public LongStream scan(final LongBiFunction accumulator) {
final LongIteratorEx iter = iteratorEx();
return newStream(new LongIteratorEx() {
private long res = 0;
private boolean isFirst = true;
@Override
public boolean hasNext() {
return iter.hasNext();
}
@Override
public long nextLong() {
if (isFirst) {
isFirst = false;
return (res = iter.nextLong());
} else {
return (res = accumulator.apply(res, iter.nextLong()));
}
}
}, false);
}
@Override
public LongStream scan(final long seed, final LongBiFunction accumulator) {
final LongIteratorEx iter = iteratorEx();
return newStream(new LongIteratorEx() {
private long res = seed;
@Override
public boolean hasNext() {
return iter.hasNext();
}
@Override
public long nextLong() {
return (res = accumulator.apply(res, iter.nextLong()));
}
}, false);
}
@Override
public Map toMap(LongFunction extends K> keyExtractor, LongFunction extends V> valueMapper) {
final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy