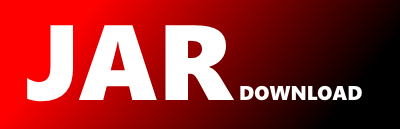
com.landawn.abacus.util.Difference Maven / Gradle / Ivy
Go to download
The Bouncy Castle Crypto package is a Java implementation of cryptographic algorithms. This jar contains JCE provider and lightweight API for the Bouncy Castle Cryptography APIs for JDK 1.8 and up. Note: this package includes the NTRU encryption algorithms.
The newest version!
Please wait ...