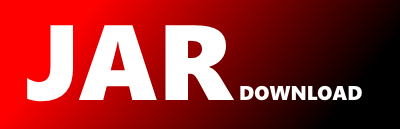
com.landawn.abacus.util.ImmutableArray Maven / Gradle / Ivy
/*
* Copyright (C) 2020 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.util;
import java.util.function.Consumer;
import com.landawn.abacus.annotation.Beta;
import com.landawn.abacus.util.stream.Stream;
@com.landawn.abacus.annotation.Immutable
public final class ImmutableArray implements Iterable, Immutable {
private final T[] elements;
private final int length;
ImmutableArray(final T[] elements) {
this.elements = elements == null ? (T[]) N.EMPTY_OBJECT_ARRAY : elements;
length = N.len(this.elements);
}
/**
*
* @param
* @param e1
* @return
*/
public static ImmutableArray of(final T e1) {
return new ImmutableArray<>(N.asArray(e1));
}
/**
*
* @param
* @param e1
* @param e2
* @return
*/
public static ImmutableArray of(final T e1, final T e2) {
return new ImmutableArray<>(N.asArray(e1, e2));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3) {
return new ImmutableArray<>(N.asArray(e1, e2, e3));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4, final T e5) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4, e5));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4, final T e5, final T e6) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4, e5, e6));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4, final T e5, final T e6, final T e7) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4, e5, e6, e7));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @param e8
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4, final T e5, final T e6, final T e7, final T e8) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4, e5, e6, e7, e8));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @param e8
* @param e9
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4, final T e5, final T e6, final T e7, final T e8, final T e9) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4, e5, e6, e7, e8, e9));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @param e8
* @param e9
* @param e10
* @return
*/
public static ImmutableArray of(final T e1, final T e2, final T e3, final T e4, final T e5, final T e6, final T e7, final T e8, final T e9,
final T e10) {
return new ImmutableArray<>(N.asArray(e1, e2, e3, e4, e5, e6, e7, e8, e9, e10));
}
/**
*
* @param
* @param elements
* @return
*/
public static ImmutableArray copyOf(final T[] elements) {
return new ImmutableArray<>(elements == null ? null : elements.clone());
}
/**
* Wraps the provided array into an ImmutableArray. Changes to the specified array will be reflected in the ImmutableArray
*
* @param
* @param elements
* @return an {@code ImmutableArray} backed by the specified {@code elements}
* @deprecated the ImmutableArray may be modified through the specified {@code elements}
*/
@Deprecated
@Beta
public static ImmutableArray wrap(final T[] elements) {
return new ImmutableArray<>(elements);
}
public int length() {
return length;
}
public boolean isEmpty() {
return length == 0;
}
/**
*
* @param index
* @return
*/
public T get(final int index) {
return elements[index];
}
/**
*
* @param valueToFind
* @return
*/
public int indexOf(final T valueToFind) {
return N.indexOf(elements, valueToFind);
}
/**
*
* @param valueToFind
* @return
*/
public int lastIndexOf(final T valueToFind) {
return N.lastIndexOf(elements, valueToFind);
}
/**
*
* @param valueToFind
* @return
*/
public boolean contains(final T valueToFind) {
return N.contains(elements, valueToFind);
}
/**
*
* @param fromIndex
* @param toIndex
* @return
* @throws IndexOutOfBoundsException
*/
public ImmutableArray copy(final int fromIndex, final int toIndex) throws IndexOutOfBoundsException {
N.checkFromToIndex(fromIndex, toIndex, length);
return new ImmutableArray<>(N.copyOfRange(elements, fromIndex, toIndex));
}
public ImmutableList asList() {
return ImmutableList.wrap(N.asList(elements));
}
@Override
public ObjIterator iterator() {
return ObjIterator.of(elements);
}
public Stream stream() {
return Stream.of(elements);
}
/**
*
* @param consumer
* @throws IllegalArgumentException
*/
@Override
public void forEach(final Consumer super T> consumer) throws IllegalArgumentException {
N.checkArgNotNull(consumer, "consumer"); // NOSONAR
for (int i = 0; i < length; i++) {
consumer.accept(elements[i]);
}
}
/**
*
* @param
* @param consumer
* @throws IllegalArgumentException
* @throws E
*/
@Beta
public void foreach(final Throwables.Consumer super T, E> consumer) throws IllegalArgumentException, E { // NOSONAR
N.checkArgNotNull(consumer, "consumer"); // NOSONAR
for (int i = 0; i < length; i++) {
consumer.accept(elements[i]);
}
}
/**
*
* @param
* @param consumer
* @throws IllegalArgumentException
* @throws E
*/
@Beta
public void foreachIndexed(final Throwables.IntObjConsumer super T, E> consumer) throws IllegalArgumentException, E { // NOSONAR
N.checkArgNotNull(consumer, "consumer"); // NOSONAR
for (int i = 0; i < length; i++) {
consumer.accept(i, elements[i]);
}
}
@Override
public int hashCode() {
return N.hashCode(elements) * 31;
}
/**
*
* @param obj
* @return
*/
@Override
public boolean equals(final Object obj) {
return obj instanceof ImmutableArray && N.equals(elements, ((ImmutableArray) obj).elements);
}
@Override
public String toString() {
return N.toString(elements);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy