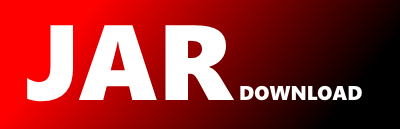
com.landawn.abacus.util.ImmutableNavigableSet Maven / Gradle / Ivy
/*
* Copyright (C) 2017 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.util;
import java.util.*;
import com.landawn.abacus.annotation.Beta;
import com.landawn.abacus.annotation.SuppressFBWarnings;
/**
*
* @param
*/
@SuppressFBWarnings("EQ_DOESNT_OVERRIDE_EQUALS")
public final class ImmutableNavigableSet extends ImmutableSortedSet implements NavigableSet { //NOSONAR
@SuppressWarnings("rawtypes")
private static final ImmutableNavigableSet EMPTY = new ImmutableNavigableSet(N.emptyNavigableSet());
private final NavigableSet navigableSet;
ImmutableNavigableSet(final NavigableSet extends E> navigableSet) {
super(navigableSet);
this.navigableSet = (NavigableSet) navigableSet;
}
/**
*
* @param
* @return
*/
public static ImmutableNavigableSet empty() {
return EMPTY;
}
/**
*
* @param
* @param e
* @return
*/
public static > ImmutableNavigableSet just(final E e) {
return new ImmutableNavigableSet<>(new TreeSet<>(Collections.singletonList(e)));
}
/**
*
* @param
* @param e1
* @return
*/
public static > ImmutableNavigableSet of(final E e1) {
return new ImmutableNavigableSet<>(new TreeSet<>(Collections.singletonList(e1)));
}
/**
*
* @param
* @param e1
* @param e2
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4, final E e5) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4, e5)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4, final E e5, final E e6) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4, e5, e6)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4, final E e5, final E e6,
final E e7) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4, e5, e6, e7)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @param e8
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4, final E e5, final E e6,
final E e7, final E e8) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4, e5, e6, e7, e8)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @param e8
* @param e9
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4, final E e5, final E e6,
final E e7, final E e8, final E e9) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4, e5, e6, e7, e8, e9)));
}
/**
*
* @param
* @param e1
* @param e2
* @param e3
* @param e4
* @param e5
* @param e6
* @param e7
* @param e8
* @param e9
* @param e10
* @return
*/
public static > ImmutableNavigableSet of(final E e1, final E e2, final E e3, final E e4, final E e5, final E e6,
final E e7, final E e8, final E e9, final E e10) {
return new ImmutableNavigableSet<>(new TreeSet<>(Arrays.asList(e1, e2, e3, e4, e5, e6, e7, e8, e9, e10)));
}
/**
* Returns an ImmutableNavigableSet containing the elements of the specified collection.
* If the provided collection is already an instance of ImmutableNavigableSet, it is directly returned.
* If the provided collection is {@code null} or empty, an empty ImmutableNavigableSet is returned.
* Otherwise, a new ImmutableNavigableSet is created with the elements of the provided collection.
*
* @param the type of elements in the collection
* @param c the collection whose elements are to be placed into this set
* @return an ImmutableNavigableSet containing the elements of the specified collection
*/
public static ImmutableNavigableSet copyOf(final Collection extends E> c) {
if (c instanceof ImmutableNavigableSet) {
return (ImmutableNavigableSet) c;
} else if (N.isEmpty(c)) {
return empty();
} else {
return new ImmutableNavigableSet<>(new TreeSet<>(c));
}
}
/**
* Wraps the provided NavigableSet into an ImmutableNavigableSet. Changes to the specified NavigableSet will be reflected in the ImmutableNavigableSet.
* If the provided NavigableSet is already an instance of ImmutableNavigableSet, it is directly returned.
* If the provided NavigableSet is {@code null}, an empty ImmutableNavigableSet is returned.
* Otherwise, returns a new ImmutableNavigableSet backed by provided NavigableSet.
*
* @param the type of elements in the NavigableSet
* @param navigableSet the NavigableSet to be wrapped into an ImmutableNavigableSet
* @return an ImmutableNavigableSet backed by the provided NavigableSet
*/
@Beta
public static ImmutableNavigableSet wrap(final NavigableSet extends E> navigableSet) {
if (navigableSet instanceof ImmutableNavigableSet) {
return (ImmutableNavigableSet) navigableSet;
} else if (navigableSet == null) {
return empty();
} else {
return new ImmutableNavigableSet<>(navigableSet);
}
}
/**
* This method is deprecated and will throw an UnsupportedOperationException if used.
*
* @param
* @param sortedSet
* @return
* @throws UnsupportedOperationException
* @deprecated throws {@code UnsupportedOperationException}
*/
@Deprecated
public static ImmutableSortedSet wrap(final SortedSet extends E> sortedSet) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
/**
*
* @param e
* @return
*/
@Override
public E lower(final E e) {
return navigableSet.lower(e);
}
/**
*
* @param e
* @return
*/
@Override
public E floor(final E e) {
return navigableSet.floor(e);
}
/**
*
* @param e
* @return
*/
@Override
public E ceiling(final E e) {
return navigableSet.ceiling(e);
}
/**
*
* @param e
* @return
*/
@Override
public E higher(final E e) {
return navigableSet.higher(e);
}
/**
*
* @return
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public E pollFirst() throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
/**
*
* @return
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public E pollLast() throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@Override
public ImmutableNavigableSet descendingSet() {
return wrap(navigableSet.descendingSet());
}
@Override
public ObjIterator descendingIterator() {
return ObjIterator.of(navigableSet.descendingIterator());
}
/**
*
* @param fromElement
* @param fromInclusive
* @param toElement
* @param toInclusive
* @return
*/
@Override
public ImmutableNavigableSet subSet(final E fromElement, final boolean fromInclusive, final E toElement, final boolean toInclusive) {
return wrap(navigableSet.subSet(fromElement, fromInclusive, toElement, toInclusive));
}
/**
*
* @param toElement
* @param inclusive
* @return
*/
@Override
public ImmutableNavigableSet headSet(final E toElement, final boolean inclusive) {
return wrap(navigableSet.headSet(toElement, inclusive));
}
/**
*
* @param fromElement
* @param inclusive
* @return
*/
@Override
public ImmutableNavigableSet tailSet(final E fromElement, final boolean inclusive) {
return wrap(navigableSet.tailSet(fromElement, inclusive));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy