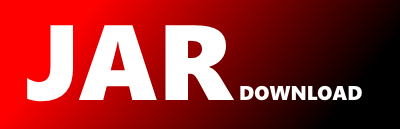
com.landawn.abacus.util.ObjListIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-common Show documentation
Show all versions of abacus-common Show documentation
A general programming library in Java/Android. It's easy to learn and simple to use with concise and powerful APIs.
The newest version!
/*
* Copyright (c) 2024, Haiyang Li.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.landawn.abacus.util;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.ListIterator;
import java.util.NoSuchElementException;
import com.landawn.abacus.util.u.Nullable;
import com.landawn.abacus.util.stream.Stream;
/**
*
* @see ObjIterator
* @see BiIterator
* @see TriIterator
* @see com.landawn.abacus.util.Iterators
* @see com.landawn.abacus.util.Enumerations
*
*/
@SuppressWarnings({ "java:S6548" })
public abstract class ObjListIterator extends ImmutableIterator implements ListIterator {
@SuppressWarnings("rawtypes")
private static final ObjListIterator EMPTY = new ObjListIterator() {
@Override
public boolean hasNext() {
return false;
}
@Override
public Object next() {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
@Override
public boolean hasPrevious() {
return false;
}
@Override
public Object previous() {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
@Override
public int nextIndex() {
return 0;
}
@Override
public int previousIndex() {
return -1;
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void set(final Object e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void add(final Object e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
};
/**
*
* @param
* @return
*/
public static ObjListIterator empty() {
return EMPTY;
}
/**
*
* @param
* @param val
* @return
*/
public static ObjListIterator just(final T val) {
return of(List.of(val));
}
/**
*
* @param
* @param a
* @return
*/
@SafeVarargs
public static ObjListIterator of(final T... a) {
if (N.isEmpty(a)) {
return empty();
}
return of(List.of(a));
}
/**
*
* @param
* @param a
* @param fromIndex
* @param toIndex
* @return
* @throws IndexOutOfBoundsException
*/
public static ObjListIterator of(final T[] a, final int fromIndex, final int toIndex) throws IndexOutOfBoundsException {
N.checkFromToIndex(fromIndex, toIndex, a == null ? 0 : a.length);
if (N.isEmpty(a) || fromIndex == toIndex) {
return empty();
} else if (fromIndex == 0 && toIndex == a.length) {
return of(Arrays.asList(a));
} else {
return of(Arrays.asList(a).subList(fromIndex, toIndex));
}
}
/**
*
* @param
* @param list
* @return
*/
public static ObjListIterator of(final List extends T> list) {
return list == null ? ObjListIterator.empty() : of(list.listIterator());
}
/**
*
* @param
* @param iter
* @return
*/
public static ObjListIterator of(final ListIterator extends T> iter) {
if (iter == null) {
return empty();
} else if (iter instanceof ObjListIterator) {
return (ObjListIterator) iter;
}
return new ObjListIterator<>() {
@Override
public boolean hasNext() {
return iter.hasNext();
}
@Override
public T next() {
return iter.next();
}
@Override
public boolean hasPrevious() {
return iter.hasPrevious();
}
@Override
public T previous() {
return iter.previous();
}
@Override
public int nextIndex() {
return iter.nextIndex();
}
@Override
public int previousIndex() {
return iter.previousIndex();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void set(final T e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void add(final T e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
};
}
/**
*
* @param n
* @return
* @throws IllegalArgumentException
*/
public ObjListIterator skip(final long n) throws IllegalArgumentException {
N.checkArgNotNegative(n, cs.n);
if (n <= 0) {
return this;
}
final ObjListIterator iter = this;
return new ObjListIterator<>() {
private boolean skipped = false;
@Override
public boolean hasNext() {
if (!skipped) {
skip();
}
return iter.hasNext();
}
@Override
public T next() {
if (!hasNext()) {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
return iter.next();
}
@Override
public boolean hasPrevious() {
return iter.hasPrevious();
}
@Override
public T previous() {
return iter.previous();
}
@Override
public int nextIndex() {
return iter.nextIndex();
}
@Override
public int previousIndex() {
return iter.previousIndex();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void set(final T e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void add(final T e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
private void skip() {
long idx = 0;
while (idx++ < n && iter.hasNext()) {
iter.next();
}
skipped = true;
}
};
}
/**
*
* @param count
* @return
* @throws IllegalArgumentException
*/
public ObjListIterator limit(final long count) throws IllegalArgumentException {
N.checkArgNotNegative(count, cs.count);
if (count == 0) {
return ObjListIterator.empty();
}
final ObjListIterator iter = this;
return new ObjListIterator<>() {
private long cnt = count;
@Override
public boolean hasNext() {
return cnt > 0 && iter.hasNext();
}
@Override
public T next() {
if (!hasNext()) {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
cnt--;
return iter.next();
}
@Override
public boolean hasPrevious() {
return iter.hasPrevious();
}
@Override
public T previous() {
return iter.previous();
}
@Override
public int nextIndex() {
return iter.nextIndex();
}
@Override
public int previousIndex() {
return iter.previousIndex();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void set(final T e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
/**
*
* @throws UnsupportedOperationException
* @deprecated - UnsupportedOperationException
*/
@Deprecated
@Override
public void add(final T e) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
};
}
public Nullable first() {
if (hasNext()) {
return Nullable.of(next());
} else {
return Nullable.empty();
}
}
public u.Optional firstNonNull() {
T next = null;
while (hasNext()) {
next = next();
if (next != null) {
return u.Optional.of(next);
}
}
return u.Optional.empty();
}
public Nullable last() {
if (hasNext()) {
T next = next();
while (hasNext()) {
next = next();
}
return Nullable.of(next);
} else {
return Nullable.empty();
}
}
public Object[] toArray() {
return toArray(N.EMPTY_OBJECT_ARRAY);
}
/**
*
* @param
* @param a
* @return
*/
public A[] toArray(final A[] a) {
return toList().toArray(a);
}
public List toList() {
final List list = new ArrayList<>();
while (hasNext()) {
list.add(next());
}
return list;
}
public Stream stream() {
return Stream.of(this);
}
/**
*
* @param
* @param action
* @throws IllegalArgumentException
* @throws E the e
*/
public void foreachRemaining(final Throwables.Consumer super T, E> action) throws IllegalArgumentException, E {
N.checkArgNotNull(action);
while (hasNext()) {
action.accept(next());
}
}
/**
*
* @param
* @param action
* @throws IllegalArgumentException
* @throws E the e
*/
public void foreachIndexed(final Throwables.IntObjConsumer super T, E> action) throws IllegalArgumentException, E {
N.checkArgNotNull(action);
int idx = 0;
while (hasNext()) {
action.accept(idx++, next());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy