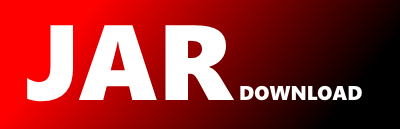
com.landawn.abacus.util.Synchronized Maven / Gradle / Ivy
/*
* Copyright (C) 2016 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.util;
/**
* This class provides a way to perform synchronized operations on a given object.
* It provides static methods to perform operations like run, call, test, accept, and apply on a given object in a synchronized manner.
* It also provides instance methods to perform these operations on the object provided at the time of creation of the Synchronized object.
*
* @param The type of the object on which the synchronized operations are to be performed.
* @param
*/
@SuppressWarnings("java:S2445")
public final class Synchronized {
private final T mutex;
Synchronized(final T mutex) {
N.checkArgNotNull(mutex);
this.mutex = mutex;
}
/**
* Creates a new Synchronized object on the provided {@code mutex}.
*
* This method is useful when you want to perform synchronized operations on a given object.
* The returned Synchronized object provides methods to perform operations like run, call, test, accept, and apply on the {@code mutex} in a synchronized manner.
*
* @param The type of the mutex.
* @param mutex The object on which the synchronized operations are to be performed.
* @return A new Synchronized object on the provided {@code mutex}.
* @throws IllegalArgumentException if the provided {@code mutex} is {@code null}.
*/
public static Synchronized on(final T mutex) throws IllegalArgumentException {
N.checkArgNotNull(mutex);
return new Synchronized<>(mutex);
}
/**
* Executes the provided {@code cmd} in a synchronized manner on the provided {@code mutex}.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a runnable task that can throw an exception.
*
* @param The type of the mutex.
* @param The type of the exception that the {@code cmd} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param cmd The runnable task that might throw an exception.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code cmd} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code cmd}.
*/
public static void run(final T mutex, final Throwables.Runnable cmd) throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(cmd);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
cmd.run();
}
}
/**
* Executes the provided {@code cmd} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* This method is useful when you want to run a piece of code that might throw an exception, you need the result of that code, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a callable task that can throw an exception and returns a result.
*
* @param The type of the mutex.
* @param The type of the result.
* @param The type of the exception that the {@code cmd} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param cmd The callable task that might throw an exception and returns a result.
* @return The result of the {@code cmd}.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code cmd} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code cmd}.
*/
public static R call(final T mutex, final Throwables.Callable cmd) throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(cmd);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
return cmd.call();
}
}
/**
* Tests the provided {@code predicate} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* This method is useful when you want to test a condition that might throw an exception, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a predicate that can throw an exception and returns a boolean result.
*
* @param The type of the mutex.
* @param The type of the exception that the {@code predicate} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param predicate The predicate that might throw an exception and returns a boolean result.
* @return The result of the {@code predicate}.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code predicate} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code predicate}.
*/
public static boolean test(final T mutex, final Throwables.Predicate super T, E> predicate) throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(predicate);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
return predicate.test(mutex);
}
}
/**
* Tests the provided {@code predicate} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* This method is useful when you want to test a condition that might throw an exception, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a bi-predicate that can throw an exception and returns a boolean result.
*
* @param The type of the mutex.
* @param The type of the second argument to the predicate.
* @param The type of the exception that the {@code predicate} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param u The second argument to the predicate. This argument should be of type U.
* @param predicate The bi-predicate that might throw an exception and returns a boolean result.
* @return The result of the {@code predicate}.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code predicate} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code predicate}.
*/
public static boolean test(final T mutex, final U u, final Throwables.BiPredicate super T, ? super U, E> predicate)
throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(predicate);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
return predicate.test(mutex, u);
}
}
/**
* Executes the provided {@code consumer} in a synchronized manner on the provided {@code mutex}.
*
* This method is useful when you want to perform an operation that might throw an exception, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a consumer that can throw an exception.
*
* @param The type of the mutex.
* @param The type of the exception that the {@code consumer} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param consumer The consumer that might throw an exception.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code consumer} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code consumer}.
*/
public static void accept(final T mutex, final Throwables.Consumer super T, E> consumer) throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(consumer);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
consumer.accept(mutex);
}
}
/**
* Executes the provided {@code consumer} in a synchronized manner on the provided {@code mutex} and {@code u}.
*
* This method is useful when you want to perform an operation that might throw an exception, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a bi-consumer that can throw an exception.
*
* @param The type of the mutex.
* @param The type of the second argument to the consumer.
* @param The type of the exception that the {@code consumer} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param u The second argument to the consumer. This argument should be of type U.
* @param consumer The bi-consumer that might throw an exception.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code consumer} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code consumer}.
*/
public static void accept(final T mutex, final U u, final Throwables.BiConsumer super T, ? super U, E> consumer)
throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(consumer);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
consumer.accept(mutex, u);
}
}
/**
* Executes the provided {@code function} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* This method is useful when you want to run a piece of code that might throw an exception, you need the result of that code, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a function that can throw an exception and returns a result.
*
* @param The type of the mutex.
* @param The type of the result.
* @param The type of the exception that the {@code function} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param function The function that might throw an exception and returns a result.
* @return The result of the {@code function}.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code function} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code function}.
*/
public static R apply(final T mutex, final Throwables.Function super T, ? extends R, E> function)
throws IllegalArgumentException, E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(function);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
return function.apply(mutex);
}
}
/**
* Executes the provided {@code function} in a synchronized manner on the provided {@code mutex} and {@code u}, and returns the result.
*
* This method is useful when you want to run a piece of code that might throw an exception, you need the result of that code, and you need to ensure that the execution is synchronized.
* It allows you to handle exceptions in a specific way by providing a bi-function that can throw an exception and returns a result.
*
* @param The type of the mutex.
* @param The type of the second argument to the function.
* @param The type of the result.
* @param The type of the exception that the {@code function} might throw.
* @param mutex The object on which the synchronized operations are to be performed.
* @param u The second argument to the function. This argument should be of type U.
* @param function The bi-function that might throw an exception and returns a result.
* @return The result of the {@code function}.
* @throws IllegalArgumentException if the provided {@code mutex} or {@code function} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code function}.
*/
public static R apply(final T mutex, final U u, final Throwables.BiFunction super T, ? super U, ? extends R, E> function)
throws E {
N.checkArgNotNull(mutex);
N.checkArgNotNull(function);
//noinspection SynchronizationOnLocalVariableOrMethodParameter
synchronized (mutex) {
return function.apply(mutex, u);
}
}
/**
* Executes the provided {@code cmd} in a synchronized manner on the provided {@code mutex}.
*
* @param The type of the exception that the {@code cmd} might throw.
* @param cmd The runnable task that might throw an exception.
* @throws IllegalArgumentException if the provided {@code cmd} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code cmd}.
*/
public void run(final Throwables.Runnable cmd) throws IllegalArgumentException, E {
N.checkArgNotNull(cmd);
synchronized (mutex) {
cmd.run();
}
}
/**
* Executes the provided {@code cmd} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* @param The type of the result.
* @param The type of the exception that the {@code cmd} might throw.
* @param cmd The callable task that might throw an exception and returns a result.
* @return The result of the {@code cmd}.
* @throws IllegalArgumentException if the provided {@code cmd} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code cmd}.
*/
public R call(final Throwables.Callable cmd) throws IllegalArgumentException, E {
N.checkArgNotNull(cmd);
synchronized (mutex) {
return cmd.call();
}
}
/**
* Tests the provided {@code predicate} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* @param The type of the exception that the {@code predicate} might throw.
* @param predicate The predicate that might throw an exception and returns a boolean result.
* @return The result of the {@code predicate}.
* @throws IllegalArgumentException if the provided {@code predicate} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code predicate}.
*/
public boolean test(final Throwables.Predicate super T, E> predicate) throws IllegalArgumentException, E {
N.checkArgNotNull(predicate);
synchronized (mutex) {
return predicate.test(mutex);
}
}
/**
* Executes the provided {@code consumer} in a synchronized manner on the provided {@code mutex}.
*
* @param The type of the exception that the {@code consumer} might throw.
* @param consumer The consumer that might throw an exception.
* @throws IllegalArgumentException if the provided {@code consumer} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code consumer}.
*/
public void accept(final Throwables.Consumer super T, E> consumer) throws IllegalArgumentException, E {
N.checkArgNotNull(consumer);
synchronized (mutex) {
consumer.accept(mutex);
}
}
/**
* Executes the provided {@code function} in a synchronized manner on the provided {@code mutex} and returns the result.
*
* @param The type of the result.
* @param The type of the exception that the {@code function} might throw.
* @param function The function that might throw an exception and returns a result.
* @return The result of the {@code function}.
* @throws IllegalArgumentException if the provided {@code function} is {@code null}.
* @throws E if an exception occurs during the execution of the {@code function}.
*/
public R apply(final Throwables.Function super T, ? extends R, E> function) throws IllegalArgumentException, E {
N.checkArgNotNull(function);
synchronized (mutex) {
return function.apply(mutex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy