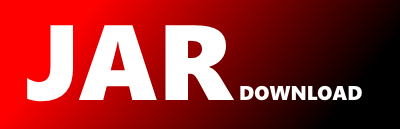
com.landawn.abacus.util.Throwables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-common Show documentation
Show all versions of abacus-common Show documentation
A general programming library in Java/Android. It's easy to learn and simple to use with concise and powerful APIs.
The newest version!
/*
* Copyright (C) 2019 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.util;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.Reader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.NoSuchElementException;
import com.landawn.abacus.annotation.Beta;
import com.landawn.abacus.annotation.Internal;
import com.landawn.abacus.util.u.Nullable;
/**
* The Throwables class is a utility class that provides methods for handling exceptions.
* It includes methods for running commands that may throw exceptions, calling methods that may throw exceptions, and more.
* It also provides a variety of functional interfaces that can throw exceptions.
*
*/
@SuppressWarnings({ "java:S6539" })
public final class Throwables {
private Throwables() {
// Singleton for utility class.
}
/**
* Executes the provided {@code cmd} that may throw an exception.
*
* This method is useful when you want to run a piece of code that might throw an exception.
* If an exception occurs during the execution of the {@code cmd}, it is rethrown as a RuntimeException.
*
* @param cmd The runnable task that might throw an exception. Must not be {@code null}.
* @throws RuntimeException if an exception occurs during the execution of the {@code cmd}.
* @see Try#run(Throwables.Runnable)
*/
@Beta
public static void run(final Throwables.Runnable extends Throwable> cmd) {
try {
cmd.run();
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
}
/**
* Executes the provided {@code cmd} and if an exception occurs, applies the {@code actionOnError} consumer on the exception.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you want to handle that exception in a specific way.
*
* @param cmd The runnable task that might throw an exception, must not be {@code null}.
* @param actionOnError The consumer to handle any exceptions thrown by the {@code cmd}, must not be {@code null}.
* @see Try#run(Throwables.Runnable, java.util.function.Consumer)
*/
@Beta
public static void run(final Throwables.Runnable extends Throwable> cmd, final java.util.function.Consumer super Throwable> actionOnError) {
try {
cmd.run();
} catch (final Throwable e) {
actionOnError.accept(e);
}
}
/**
* Executes the provided {@code cmd} that may throw an exception and returns the result.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you need the result of that code.
* If an exception occurs during the execution of the {@code cmd}, it is rethrown as a RuntimeException.
*
* @param The type of the result.
* @param cmd The callable task that might throw an exception and returns a result. Must not be {@code null}.
* @return The result of the {@code cmd}.
* @throws RuntimeException if an exception occurs during the execution of the {@code cmd}.
* @see Try#call(java.util.concurrent.Callable)
*/
@Beta
public static R call(final Throwables.Callable cmd) {
try {
return cmd.call();
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
}
/**
* Executes the provided {@code cmd} that may throw an exception and returns the result.
* If an exception occurs during the execution of the {@code cmd}, the {@code actionOnError} function is applied to the exception to provide a return value.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you need the result of that code.
* It allows you to handle exceptions in a specific way by providing a function that can transform an exception into a return value.
*
* @param The type of the result.
* @param cmd The callable task that might throw an exception and returns a result. Must not be {@code null}.
* @param actionOnError The function to apply to the exception if one is thrown by the {@code cmd}. Must not be {@code null}.
* @return The result of the {@code cmd} or the result of applying the {@code actionOnError} function to the exception if one is thrown.
* @see Try#call(java.util.concurrent.Callable, java.util.function.Function)
*/
@Beta
public static R call(final Throwables.Callable cmd,
final java.util.function.Function super Throwable, ? extends R> actionOnError) {
try {
return cmd.call();
} catch (final Throwable e) {
return actionOnError.apply(e);
}
}
/**
* Executes the provided {@code cmd} that may throw an exception and returns the result.
* If an exception occurs during the execution of the {@code cmd}, the {@code supplier} is used to provide a return value.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you need the result of that code.
* It allows you to handle exceptions in a specific way by providing a supplier that can provide a return value when an exception occurs.
*
* @param The type of the result.
* @param cmd The callable task that might throw an exception and returns a result. Must not be {@code null}.
* @param supplier The supplier to provide a return value when an exception occurs. Must not be {@code null}.
* @return The result of the {@code cmd} or the result of the {@code supplier} if an exception occurs.
* @see Try#call(java.util.concurrent.Callable, java.util.function.Supplier)
*/
@Beta
public static R call(final Throwables.Callable cmd, final java.util.function.Supplier supplier) {
N.checkArgNotNull(supplier);
try {
return cmd.call();
} catch (final Throwable e) {
return supplier.get();
}
}
/**
* Executes the provided {@code cmd} that may throw an exception and returns the result.
* If an exception occurs during the execution of the {@code cmd}, the provided default value is returned.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you need the result of that code.
* It allows you to handle exceptions in a specific way by providing a default value that will be returned when an exception occurs.
*
* @param The type of the result.
* @param cmd The callable task that might throw an exception and returns a result. Must not be {@code null}.
* @param defaultValue The default value to return if an exception occurs during the execution of the {@code cmd}.
* @return The result of the {@code cmd} or the default value if an exception occurs.
* @see Try#call(java.util.concurrent.Callable, Object)
*/
@Beta
public static R call(final Throwables.Callable cmd, final R defaultValue) {
try {
return cmd.call();
} catch (final Throwable e) {
return defaultValue;
}
}
/**
* Executes the provided {@code cmd} and if an exception occurs, applies the {@code supplier} to provide a return value.
* The {@code predicate} is used to test the exception. If the {@code predicate} returns {@code true}, the {@code supplier} is used to provide a return value.
* If the {@code predicate} returns {@code false}, the exception is rethrown as a RuntimeException.
*
* @param The type of the result.
* @param cmd The callable task that might throw an exception, must not be {@code null}.
* @param predicate The predicate to test the exception, must not be {@code null}.
* @param supplier The supplier to provide a return value when an exception occurs and the {@code predicate} returns {@code true}, must not be {@code null}.
* @return The result of the {@code cmd} or the result of the {@code supplier} if an exception occurs and the {@code predicate} returns {@code true}.
* @throws RuntimeException if an exception occurs and the {@code predicate} returns {@code false}.
* @see Try#call(java.util.concurrent.Callable, java.util.function.Predicate, java.util.function.Supplier)
*/
@Beta
public static R call(final Throwables.Callable cmd, final java.util.function.Predicate super Throwable> predicate,
final java.util.function.Supplier supplier) {
N.checkArgNotNull(supplier);
try {
return cmd.call();
} catch (final Throwable e) {
if (predicate.test(e)) {
return supplier.get();
} else {
throw ExceptionUtil.toRuntimeException(e, true);
}
}
}
/**
* Executes the provided {@code cmd} that may throw an exception and returns the result.
* If an exception occurs during the execution of the {@code cmd}, the provided default value is returned if the {@code predicate} returns {@code true}.
* If the {@code predicate} returns {@code false}, the exception is rethrown as a RuntimeException.
*
* This method is useful when you want to run a piece of code that might throw an exception, and you need the result of that code.
* It allows you to handle exceptions in a specific way by providing a default value that will be returned when an exception occurs and the {@code predicate} returns {@code true}.
*
* @param The type of the result.
* @param cmd The callable task that might throw an exception and returns a result. Must not be {@code null}.
* @param predicate The predicate to test the exception. If it returns {@code true}, the default value is returned. If it returns {@code false}, the exception is rethrown. Must not be {@code null}.
* @param defaultValue The default value to return if an exception occurs during the execution of the {@code cmd} and the {@code predicate} returns {@code true}.
* @return The result of the {@code cmd} or the default value if an exception occurs and the {@code predicate} returns {@code true}.
* @throws RuntimeException if an exception occurs and the {@code predicate} returns {@code false}.
* @see Try#call(java.util.concurrent.Callable, java.util.function.Predicate, Object)
*/
@Beta
public static R call(final Throwables.Callable cmd, final java.util.function.Predicate super Throwable> predicate,
final R defaultValue) {
try {
return cmd.call();
} catch (final Throwable e) {
if (predicate.test(e)) {
return defaultValue;
} else {
throw ExceptionUtil.toRuntimeException(e, true);
}
}
}
@SuppressWarnings("rawtypes")
private static final Throwables.Iterator EMPTY = new Throwables.Iterator() {
@Override
public boolean hasNext() {
return false;
}
@Override
public Object next() {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
};
/**
*
* @param
* @param
* @see ObjIterator
*/
@SuppressWarnings({ "java:S6548" })
public abstract static class Iterator implements AutoCloseable, Immutable {
/**
*
* @param
* @param
* @return
*/
public static Throwables.Iterator empty() {
return EMPTY;
}
/**
*
* @param
* @param
* @param val
* @return
*/
public static Throwables.Iterator just(final T val) {
return new Throwables.Iterator<>() {
private boolean done = false;
@Override
public boolean hasNext() {
return !done;
}
@Override
public T next() {
if (done) {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
done = true;
return val;
}
};
}
/**
*
* @param
* @param
* @param a
* @return
*/
@SafeVarargs
public static Throwables.Iterator of(final T... a) {
return N.isEmpty(a) ? EMPTY : of(a, 0, a.length);
}
/**
*
* @param
* @param
* @param a
* @param fromIndex
* @param toIndex
* @return
* @throws IndexOutOfBoundsException
*/
public static Throwables.Iterator of(final T[] a, final int fromIndex, final int toIndex)
throws IndexOutOfBoundsException {
N.checkFromToIndex(fromIndex, toIndex, a == null ? 0 : a.length);
if (N.isEmpty(a) || fromIndex == toIndex) {
return EMPTY;
}
return new Throwables.Iterator<>() {
private int cursor = fromIndex;
@Override
public boolean hasNext() {
return cursor < toIndex;
}
@Override
public T next() {
if (cursor >= toIndex) {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
return a[cursor++];
}
@Override
public void advance(final long n) throws E {
if (n > toIndex - cursor) {
cursor = toIndex;
} else {
cursor += (int) n;
}
}
@Override
public long count() {
return toIndex - cursor; //NOSONAR
}
};
}
/**
*
* @param
* @param
* @param iterable
* @return
*/
public static Iterator of(final Iterable extends T> iterable) {
if (iterable == null) {
return empty();
}
final java.util.Iterator extends T> iter = iterable.iterator();
return new Throwables.Iterator<>() {
@Override
public boolean hasNext() {
return iter.hasNext();
}
@Override
public T next() throws E {
return iter.next();
}
};
}
/**
*
* @param
* @param
* @param iter
* @return
*/
public static Throwables.Iterator of(final java.util.Iterator extends T> iter) {
if (iter == null) {
return EMPTY;
}
return new Throwables.Iterator<>() {
@Override
public boolean hasNext() throws E {
return iter.hasNext();
}
@Override
public T next() throws E {
return iter.next();
}
};
}
/**
* Returns a Throwables.Iterator instance that is created lazily using the provided Supplier.
* The Supplier is responsible for producing the Iterator instance when the Iterator's methods are first called.
*
* @param The type of the elements in the Iterator.
* @param The type of the exception that may be thrown.
* @param iteratorSupplier A Supplier that provides the Throwables.Iterator when needed.
* @return A Throwables.Iterator that is initialized on the first call to hasNext() or next().
* @throws IllegalArgumentException if iteratorSupplier is {@code null}.
*/
public static Throwables.Iterator defer(final java.util.function.Supplier> iteratorSupplier) {
N.checkArgNotNull(iteratorSupplier, cs.iteratorSupplier);
return new Throwables.Iterator<>() {
private Throwables.Iterator iter = null;
private boolean isInitialized = false;
@Override
public boolean hasNext() throws E {
if (!isInitialized) {
init();
}
return iter.hasNext();
}
@Override
public T next() throws E {
if (!isInitialized) {
init();
}
return iter.next();
}
@Override
public void advance(final long n) throws IllegalArgumentException, E {
N.checkArgNotNegative(n, cs.n);
if (!isInitialized) {
init();
}
iter.advance(n);
}
@Override
public long count() throws E {
if (!isInitialized) {
init();
}
return iter.count();
}
@Override
protected void closeResource() {
if (!isInitialized) {
init();
}
if (iter != null) {
iter.close();
}
}
private void init() {
if (!isInitialized) {
isInitialized = true;
iter = iteratorSupplier.get();
}
}
};
}
/**
*
* @param
* @param
* @param a
* @return
*/
@SafeVarargs
public static Throwables.Iterator concat(final Throwables.Iterator extends T, ? extends E>... a) {
return concat(N.asList(a));
}
/**
*
* @param
* @param
* @param c
* @return
*/
public static Throwables.Iterator concat(final Collection extends Throwables.Iterator extends T, ? extends E>> c) {
if (N.isEmpty(c)) {
return Iterator.empty();
}
return new Throwables.Iterator<>() {
private final java.util.Iterator extends Throwables.Iterator extends T, ? extends E>> iter = c.iterator();
private Throwables.Iterator extends T, ? extends E> cur;
@Override
public boolean hasNext() throws E {
while ((cur == null || !cur.hasNext()) && iter.hasNext()) {
cur = iter.next();
}
return cur != null && cur.hasNext();
}
@Override
public T next() throws E {
if ((cur == null || !cur.hasNext()) && !hasNext()) {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
return cur.next();
}
};
}
/**
* It's caller's responsibility to close the specified {@code reader}.
*
* @param reader
* @return
*/
public static Throwables.Iterator ofLines(final Reader reader) {
if (reader == null) {
return empty();
}
return new Throwables.Iterator<>() {
private final BufferedReader br = reader instanceof BufferedReader ? (BufferedReader) reader : new BufferedReader(reader);
private String cachedLine;
/** A flag indicating if the iterator has been fully read. */
private boolean finished = false;
@Override
public boolean hasNext() throws IOException {
if (cachedLine != null) {
return true;
} else if (finished) {
return false;
} else {
cachedLine = br.readLine();
if (cachedLine == null) {
finished = true;
return false;
} else {
return true;
}
}
}
@Override
public String next() throws IOException {
if (!hasNext()) {
throw new NoSuchElementException("No more lines");
}
final String res = cachedLine;
cachedLine = null;
return res;
}
};
}
/**
*
* @return
* @throws E
*/
public abstract boolean hasNext() throws E;
/**
*
* @return
* @throws E
*/
public abstract T next() throws E;
/**
*
* @param n
* @throws IllegalArgumentException
* @throws E the e
*/
public void advance(long n) throws IllegalArgumentException, E {
N.checkArgNotNegative(n, cs.n);
while (n-- > 0 && hasNext()) {
next();
}
}
/**
*
* @return
* @throws E the e
*/
public long count() throws E {
long result = 0;
while (hasNext()) {
next();
result++;
}
return result;
}
private boolean isClosed = false;
@Override
public final void close() {
if (isClosed) {
return;
}
isClosed = true;
closeResource();
}
@Internal
protected void closeResource() {
}
/**
*
* @param predicate
* @return
*/
public Throwables.Iterator filter(final Throwables.Predicate super T, E> predicate) {
final Throwables.Iterator iter = this;
return new Throwables.Iterator<>() {
private final T NONE = (T) N.NULL_MASK; //NOSONAR
private T next = NONE;
private T tmp = null;
@Override
public boolean hasNext() throws E {
if (next == NONE) {
while (iter.hasNext()) {
tmp = iter.next();
if (predicate.test(tmp)) {
next = tmp;
break;
}
}
}
return next != NONE;
}
@Override
public T next() throws E {
if (!hasNext()) {
throw new NoSuchElementException(InternalUtil.ERROR_MSG_FOR_NO_SUCH_EX);
}
tmp = next;
next = NONE;
return tmp;
}
};
}
/**
*
* @param
* @param mapper
* @return
*/
public Throwables.Iterator map(final Throwables.Function super T, U, E> mapper) {
final Throwables.Iterator iter = this;
return new Throwables.Iterator<>() {
@Override
public boolean hasNext() throws E {
return iter.hasNext();
}
@Override
public U next() throws E {
return mapper.apply(iter.next());
}
};
}
/**
*
* @return
* @throws E
*/
public Nullable first() throws E {
if (hasNext()) {
return Nullable.of(next());
} else {
return Nullable.empty();
}
}
/**
*
* @return
* @throws E
*/
public u.Optional firstNonNull() throws E {
T next = null;
while (hasNext()) {
next = next();
if (next != null) {
return u.Optional.of(next);
}
}
return u.Optional.empty();
}
/**
*
* @return
* @throws E
*/
public Nullable last() throws E {
if (hasNext()) {
T next = next();
while (hasNext()) {
next = next();
}
return Nullable.of(next);
} else {
return Nullable.empty();
}
}
/**
*
* @return
* @throws E
*/
public Object[] toArray() throws E {
return toArray(N.EMPTY_OBJECT_ARRAY);
}
/**
*
* @param
* @param a
* @return
* @throws E
*/
public A[] toArray(final A[] a) throws E {
return toList().toArray(a);
}
/**
*
* @return
* @throws E
*/
public List toList() throws E {
final List list = new ArrayList<>();
while (hasNext()) {
list.add(next());
}
return list;
}
/**
*
* @param action
* @throws E
*/
public void forEachRemaining(final java.util.function.Consumer super T> action) throws E { // NOSONAR
while (hasNext()) {
action.accept(next());
}
}
/**
*
* @param
* @param action
* @throws E the e
* @throws E2
*/
public void foreachRemaining(final Throwables.Consumer super T, E2> action) throws E, E2 { // NOSONAR
while (hasNext()) {
action.accept(next());
}
}
/**
*
* @param
* @param action
* @throws E the e
* @throws E2
*/
public void foreachIndexed(final Throwables.IntObjConsumer super T, E2> action) throws E, E2 {
int idx = 0;
while (hasNext()) {
action.accept(idx++, next());
}
}
}
/**
* The Interface Runnable.
*
* @param
*/
public interface Runnable {
/**
*
* @throws E the e
*/
void run() throws E;
@Beta
default com.landawn.abacus.util.function.Runnable unchecked() {
return () -> {
try {
run();
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface Callable.
*
* @param
* @param
*/
public interface Callable {
/**
*
* @return
* @throws E the e
*/
R call() throws E;
@Beta
default com.landawn.abacus.util.function.Callable unchecked() {
return () -> {
try {
return call();
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface Supplier.
*
* @param
* @param
*/
public interface Supplier {
/**
*
* @return
* @throws E the e
*/
T get() throws E;
@Beta
default com.landawn.abacus.util.function.Supplier unchecked() {
return () -> {
try {
return get();
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface BooleanSupplier.
*
* @param
*/
public interface BooleanSupplier {
/**
* Gets the as boolean.
*
* @return
* @throws E the e
*/
boolean getAsBoolean() throws E; // NOSONAR
}
/**
* The Interface CharSupplier.
*
* @param
*/
public interface CharSupplier {
/**
* Gets the as char.
*
* @return
* @throws E the e
*/
char getAsChar() throws E;
}
/**
* The Interface ByteSupplier.
*
* @param
*/
public interface ByteSupplier {
/**
* Gets the as byte.
*
* @return
* @throws E the e
*/
byte getAsByte() throws E;
}
/**
* The Interface ShortSupplier.
*
* @param
*/
public interface ShortSupplier {
/**
* Gets the as short.
*
* @return
* @throws E the e
*/
short getAsShort() throws E;
}
/**
* The Interface IntSupplier.
*
* @param
*/
public interface IntSupplier {
/**
* Gets the as int.
*
* @return
* @throws E the e
*/
int getAsInt() throws E;
}
/**
* The Interface LongSupplier.
*
* @param
*/
public interface LongSupplier {
/**
* Gets the as long.
*
* @return
* @throws E the e
*/
long getAsLong() throws E;
}
/**
* The Interface FloatSupplier.
*
* @param
*/
public interface FloatSupplier {
/**
* Gets the as float.
*
* @return
* @throws E the e
*/
float getAsFloat() throws E;
}
/**
* The Interface DoubleSupplier.
*
* @param
*/
public interface DoubleSupplier {
/**
* Gets the as double.
*
* @return
* @throws E the e
*/
double getAsDouble() throws E;
}
/**
* The Interface Predicate.
*
* @param
* @param
*/
public interface Predicate {
/**
*
* @param t
* @return
* @throws E the e
*/
boolean test(T t) throws E;
default Predicate negate() {
return t -> !test(t);
}
@Beta
default com.landawn.abacus.util.function.Predicate unchecked() {
return t -> {
try {
return test(t);
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface BiPredicate.
*
* @param
* @param
* @param
*/
public interface BiPredicate {
/**
*
* @param t
* @param u
* @return
* @throws E the e
*/
boolean test(T t, U u) throws E;
@Beta
default com.landawn.abacus.util.function.BiPredicate unchecked() {
return (t, u) -> {
try {
return test(t, u);
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface TriPredicate.
*
* @param
* @param
* @param
* @param
*/
public interface TriPredicate {
/**
*
* @param a
* @param b
* @param c
* @return
* @throws E the e
*/
boolean test(A a, B b, C c) throws E;
}
/**
* The Interface QuadPredicate.
*
* @param
* @param
* @param
* @param
* @param
*/
public interface QuadPredicate {
/**
*
* @param a
* @param b
* @param c
* @param d
* @return
* @throws E the e
*/
boolean test(A a, B b, C c, D d) throws E;
}
/**
* The Interface Function.
*
* @param
* @param
* @param
*/
public interface Function {
/**
*
* @param t
* @return
* @throws E the e
*/
R apply(T t) throws E;
@Beta
default com.landawn.abacus.util.function.Function unchecked() {
return t -> {
try {
return apply(t);
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface BiFunction.
*
* @param
* @param
* @param
* @param
*/
public interface BiFunction {
/**
*
* @param t
* @param u
* @return
* @throws E the e
*/
R apply(T t, U u) throws E;
@Beta
default com.landawn.abacus.util.function.BiFunction unchecked() {
return (t, u) -> {
try {
return apply(t, u);
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface TriFunction.
*
* @param
* @param
* @param
* @param
* @param
*/
public interface TriFunction {
/**
*
* @param a
* @param b
* @param c
* @return
* @throws E the e
*/
R apply(A a, B b, C c) throws E;
}
/**
* The Interface QuadFunction.
*
* @param
* @param
* @param
* @param
* @param
* @param
*/
public interface QuadFunction {
/**
*
* @param a
* @param b
* @param c
* @param d
* @return
* @throws E the e
*/
R apply(A a, B b, C c, D d) throws E;
}
/**
* The Interface Consumer.
*
* @param
* @param
*/
public interface Consumer {
/**
*
* @param t
* @throws E the e
*/
void accept(T t) throws E;
@Beta
default com.landawn.abacus.util.function.Consumer unchecked() {
return t -> {
try {
accept(t);
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface BiConsumer.
*
* @param
* @param
* @param
*/
public interface BiConsumer {
/**
*
* @param t
* @param u
* @throws E the e
*/
void accept(T t, U u) throws E;
@Beta
default com.landawn.abacus.util.function.BiConsumer unchecked() {
return (t, u) -> {
try {
accept(t, u);
} catch (final Throwable e) {
throw ExceptionUtil.toRuntimeException(e, true);
}
};
}
}
/**
* The Interface TriConsumer.
*
* @param
* @param
* @param
* @param
*/
public interface TriConsumer {
/**
*
* @param a
* @param b
* @param c
* @throws E the e
*/
void accept(A a, B b, C c) throws E;
}
/**
* The Interface QuadConsumer.
*
* @param
* @param
* @param
* @param
* @param
*/
public interface QuadConsumer {
/**
*
* @param a
* @param b
* @param c
* @param d
* @throws E the e
*/
void accept(A a, B b, C c, D d) throws E;
}
/**
* The Interface BooleanConsumer.
*
* @param
*/
public interface BooleanConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(boolean t) throws E;
}
/**
* The Interface BooleanPredicate.
*
* @param
*/
public interface BooleanPredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(boolean value) throws E;
}
/**
* The Interface BooleanFunction.
*
* @param
* @param
*/
public interface BooleanFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(boolean value) throws E;
}
/**
* The Interface CharConsumer.
*
* @param
*/
public interface CharConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(char t) throws E;
}
/**
* The Interface CharPredicate.
*
* @param
*/
public interface CharPredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(char value) throws E;
}
/**
* The Interface CharFunction.
*
* @param
* @param
*/
public interface CharFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(char value) throws E;
}
/**
* The Interface ByteConsumer.
*
* @param
*/
public interface ByteConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(byte t) throws E;
}
/**
* The Interface BytePredicate.
*
* @param
*/
public interface BytePredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(byte value) throws E;
}
/**
* The Interface ByteFunction.
*
* @param
* @param
*/
public interface ByteFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(byte value) throws E;
}
/**
* The Interface ShortConsumer.
*
* @param
*/
public interface ShortConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(short t) throws E;
}
/**
* The Interface ShortPredicate.
*
* @param
*/
public interface ShortPredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(short value) throws E;
}
/**
* The Interface ShortFunction.
*
* @param
* @param
*/
public interface ShortFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(short value) throws E;
}
/**
* The Interface IntConsumer.
*
* @param
*/
public interface IntConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(int t) throws E;
}
/**
* The Interface IntPredicate.
*
* @param
*/
public interface IntPredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(int value) throws E;
}
/**
* The Interface IntFunction.
*
* @param
* @param
*/
public interface IntFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(int value) throws E;
}
/**
* The Interface IntToLongFunction.
*
* @param
*/
public interface IntToLongFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
long applyAsLong(int value) throws E;
}
/**
* The Interface IntToDoubleFunction.
*
* @param
*/
public interface IntToDoubleFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
double applyAsDouble(int value) throws E;
}
/**
* The Interface LongConsumer.
*
* @param
*/
public interface LongConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(long t) throws E;
}
/**
* The Interface LongPredicate.
*
* @param
*/
public interface LongPredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(long value) throws E;
}
/**
* The Interface LongFunction.
*
* @param
* @param
*/
public interface LongFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(long value) throws E;
}
/**
* The Interface LongToIntFunction.
*
* @param
*/
public interface LongToIntFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
int applyAsInt(long value) throws E;
}
/**
* The Interface LongToDoubleFunction.
*
* @param
*/
public interface LongToDoubleFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
double applyAsDouble(long value) throws E;
}
/**
* The Interface FloatConsumer.
*
* @param
*/
public interface FloatConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(float t) throws E;
}
/**
* The Interface FloatPredicate.
*
* @param
*/
public interface FloatPredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(float value) throws E;
}
/**
* The Interface FloatFunction.
*
* @param
* @param
*/
public interface FloatFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(float value) throws E;
}
/**
* The Interface DoubleConsumer.
*
* @param
*/
public interface DoubleConsumer {
/**
*
* @param t
* @throws E the e
*/
void accept(double t) throws E;
}
/**
* The Interface DoublePredicate.
*
* @param
*/
public interface DoublePredicate {
/**
*
* @param value
* @return
* @throws E the e
*/
boolean test(double value) throws E;
}
/**
* The Interface DoubleFunction.
*
* @param
* @param
*/
public interface DoubleFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
R apply(double value) throws E;
}
/**
* The Interface DoubleToIntFunction.
*
* @param
*/
public interface DoubleToIntFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
int applyAsInt(double value) throws E;
}
/**
* The Interface DoubleToLongFunction.
*
* @param
*/
public interface DoubleToLongFunction {
/**
*
* @param value
* @return
* @throws E the e
*/
long applyAsLong(double value) throws E;
}
/**
* The Interface ToBooleanFunction.
*
* @param
* @param
*/
public interface ToBooleanFunction {
/**
* Apply as boolean.
*
* @param t
* @return
* @throws E the e
*/
boolean applyAsBoolean(T t) throws E;
}
/**
* The Interface ToCharFunction.
*
* @param
* @param
*/
public interface ToCharFunction {
/**
* Apply as char.
*
* @param t
* @return
* @throws E the e
*/
char applyAsChar(T t) throws E;
}
/**
* The Interface ToByteFunction.
*
* @param
* @param
*/
public interface ToByteFunction {
/**
* Apply as byte.
*
* @param t
* @return
* @throws E the e
*/
byte applyAsByte(T t) throws E;
}
/**
* The Interface ToShortFunction.
*
* @param
* @param
*/
public interface ToShortFunction {
/**
* Apply as short.
*
* @param t
* @return
* @throws E the e
*/
short applyAsShort(T t) throws E;
}
/**
* The Interface ToIntFunction.
*
* @param
* @param
*/
public interface ToIntFunction {
/**
* Apply as int.
*
* @param t
* @return
* @throws E the e
*/
int applyAsInt(T t) throws E;
}
/**
* The Interface ToLongFunction.
*
* @param
* @param