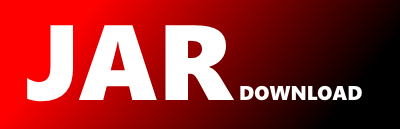
com.landawn.abacus.condition.NotBetween Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-query Show documentation
Show all versions of abacus-query Show documentation
Abacus Data Access and Analysis
/*
* Copyright (C) 2021 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.condition;
import java.util.ArrayList;
import java.util.List;
import com.landawn.abacus.util.N;
import com.landawn.abacus.util.NamingPolicy;
import com.landawn.abacus.util.Strings;
import com.landawn.abacus.util.WD;
/**
*
* @author Haiyang Li
* @since 0.8
*/
public class NotBetween extends AbstractCondition {
// For Kryo
final String propName;
private Object minValue;
private Object maxValue;
// For Kryo
NotBetween() {
propName = null;
}
/**
*
*
* @param propName
* @param minValue
* @param maxValue
*/
public NotBetween(final String propName, final Object minValue, final Object maxValue) {
super(Operator.NOT_BETWEEN);
if (Strings.isEmpty(propName)) {
throw new IllegalArgumentException("property name can't be null or empty.");
}
this.propName = propName;
this.minValue = minValue;
this.maxValue = maxValue;
}
/**
* Gets the prop name.
*
* @return
*/
public String getPropName() {
return propName;
}
/**
* Gets the min value.
*
* @param
* @return
*/
@SuppressWarnings("unchecked")
public T getMinValue() {
return (T) minValue;
}
/**
* Sets the min value.
*
* @param minValue the new min value
* @deprecated Condition should be immutable except using {@code clearParameter()} to release resources.
*/
@Deprecated
public void setMinValue(final Object minValue) {
this.minValue = minValue;
}
/**
* Gets the max value.
*
* @param
* @return
*/
@SuppressWarnings("unchecked")
public T getMaxValue() {
return (T) maxValue;
}
/**
* Sets the max value.
*
* @param maxValue the new max value
* @deprecated Condition should be immutable except using {@code clearParameter()} to release resources.
*/
@Deprecated
public void setMaxValue(final Object maxValue) {
this.maxValue = maxValue;
}
/**
* Gets the parameters.
*
* @return
*/
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy