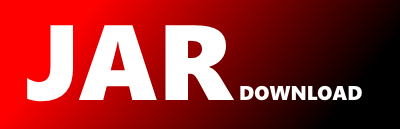
com.landawn.abacus.util.Seq Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-util-all-jdk7 Show documentation
Show all versions of abacus-util-all-jdk7 Show documentation
A general programming library in Java
/*
* Copyright (c) 2017, Haiyang Li.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.landawn.abacus.util;
import java.util.AbstractCollection;
import java.util.AbstractList;
import java.util.AbstractSet;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.ConcurrentModificationException;
import java.util.Deque;
import java.util.HashSet;
import java.util.IdentityHashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Objects;
import java.util.Random;
import java.util.RandomAccess;
import java.util.Set;
import com.landawn.abacus.util.function.BiConsumer;
import com.landawn.abacus.util.function.BiFunction;
import com.landawn.abacus.util.function.BinaryOperator;
import com.landawn.abacus.util.function.Function;
import com.landawn.abacus.util.function.IntFunction;
import com.landawn.abacus.util.function.Supplier;
import com.landawn.abacus.util.function.ToDoubleFunction;
import com.landawn.abacus.util.function.ToIntFunction;
import com.landawn.abacus.util.function.ToLongFunction;
import com.landawn.abacus.util.stream.Collector;
import com.landawn.abacus.util.stream.Collectors;
/**
* It's an read-only wrapper for Collection
to support more daily used/functional methods.
* All the operations are null safety. And an empty String
/Array
/Collection
/Optional
/Nullable
will be returned if possible, instead of null.
*
*
* Seq
should not be passed as a parameter or returned as a result because it's a pure utility class for the operations/calculation based on Collection/Array
*
* @since 0.8
*
* @author Haiyang Li
*/
public final class Seq extends ImmutableCollection {
@SuppressWarnings("rawtypes")
private static final Seq EMPTY = new Seq<>(Collections.EMPTY_LIST);
/**
* The returned Seq
and the specified Collection
are backed by the same data.
* Any changes to one will appear in the other.
*
* @param c
*/
Seq(final Collection c) {
super(c);
}
public static Seq just(T t) {
return of(t);
}
@SafeVarargs
public static Seq of(final T... a) {
if (N.isNullOrEmpty(a)) {
return EMPTY;
}
return of(Arrays.asList(a));
}
/**
* The returned Seq
and the specified Collection
are backed by the same data.
* Any changes to one will appear in the other.
*
* @param c
* @return
*/
public static Seq of(Collection c) {
if (N.isNullOrEmpty(c)) {
return EMPTY;
}
return new Seq<>(c);
}
/**
*
* @param map
* @return
*/
public static Seq> of(Map map) {
if (N.isNullOrEmpty(map)) {
return EMPTY;
}
return of(map.entrySet());
}
// /**
// * Returns the Collection
the Seq
is backed with recursively.
// *
// * @return
// */
// public Collection interior() {
// if (coll == null) {
// return coll;
// }
//
// Collection tmp = coll;
//
// if (tmp instanceof Seq) {
// while (tmp instanceof Seq) {
// tmp = ((Seq) tmp).coll;
// }
// }
//
// if (tmp instanceof SubCollection) {
// while (tmp instanceof SubCollection) {
// tmp = ((SubCollection) tmp).c;
// }
// }
//
// if (tmp instanceof Seq) {
// return ((Seq) tmp).interior();
// } else {
// return tmp;
// }
// }
@Override
public boolean contains(Object e) {
if (N.isNullOrEmpty(coll)) {
return false;
}
return coll.contains(e);
}
@Override
public boolean containsAll(Collection> c) {
if (N.isNullOrEmpty(c)) {
return true;
} else if (N.isNullOrEmpty(coll)) {
return false;
}
return coll.containsAll(c);
}
public boolean containsAll(Object[] a) {
if (N.isNullOrEmpty(a)) {
return true;
} else if (N.isNullOrEmpty(coll)) {
return false;
}
return containsAll(Arrays.asList(a));
}
public boolean containsAny(Collection> c) {
if (N.isNullOrEmpty(coll) || N.isNullOrEmpty(c)) {
return false;
}
return !disjoint(c);
}
public boolean containsAny(Object[] a) {
if (N.isNullOrEmpty(coll) || N.isNullOrEmpty(a)) {
return false;
}
return !disjoint(a);
}
public boolean disjoint(final Collection> c) {
return Seq.disjoint(this.coll, c);
}
public boolean disjoint(final Object[] a) {
if (N.isNullOrEmpty(coll) || N.isNullOrEmpty(a)) {
return true;
}
return disjoint(Arrays.asList(a));
}
/**
*
* @param b
* @return
* @see IntList#intersection(IntList)
*/
public List intersection(Collection> b) {
if (N.isNullOrEmpty(coll) || N.isNullOrEmpty(b)) {
return new ArrayList<>();
}
final Multiset> bOccurrences = Multiset.from(b);
final List result = new ArrayList<>(N.min(9, size(), b.size()));
for (T e : coll) {
if (bOccurrences.getAndRemove(e) > 0) {
result.add(e);
}
}
return result;
}
public List intersection(final Object[] a) {
if (N.isNullOrEmpty(coll) || N.isNullOrEmpty(a)) {
return new ArrayList<>();
}
return intersection(Arrays.asList(a));
}
/**
*
* @param b
* @return
* @see IntList#difference(IntList)
*/
public List difference(Collection> b) {
if (N.isNullOrEmpty(coll)) {
return new ArrayList<>();
} else if (N.isNullOrEmpty(b)) {
return new ArrayList<>(coll);
}
final Multiset> bOccurrences = Multiset.from(b);
final List result = new ArrayList<>(N.min(size(), N.max(9, size() - b.size())));
for (T e : coll) {
if (bOccurrences.getAndRemove(e) < 1) {
result.add(e);
}
}
return result;
}
public List difference(final Object[] a) {
if (N.isNullOrEmpty(coll)) {
return new ArrayList<>();
} else if (N.isNullOrEmpty(a)) {
return new ArrayList<>(coll);
}
return difference(Arrays.asList(a));
}
/**
*
* @param b
* @return this.difference(b).addAll(b.difference(this))
* @see IntList#symmetricDifference(IntList)
*/
public List symmetricDifference(Collection b) {
if (N.isNullOrEmpty(b)) {
return N.isNullOrEmpty(coll) ? new ArrayList() : new ArrayList<>(coll);
} else if (N.isNullOrEmpty(coll)) {
return new ArrayList<>(b);
}
final Multiset> bOccurrences = Multiset.from(b);
final List result = new ArrayList<>(N.max(9, Math.abs(size() - b.size())));
for (T e : coll) {
if (bOccurrences.getAndRemove(e) < 1) {
result.add(e);
}
}
for (T e : b) {
if (bOccurrences.getAndRemove(e) > 0) {
result.add(e);
}
if (bOccurrences.isEmpty()) {
break;
}
}
return result;
}
public List symmetricDifference(final T[] a) {
if (N.isNullOrEmpty(a)) {
return N.isNullOrEmpty(coll) ? new ArrayList() : new ArrayList<>(coll);
} else if (N.isNullOrEmpty(coll)) {
return N.asList(a);
}
return symmetricDifference(Arrays.asList(a));
}
public int occurrencesOf(final Object objectToFind) {
return N.isNullOrEmpty(coll) ? 0 : N.occurrencesOf(coll, objectToFind);
}
@SuppressWarnings("rawtypes")
public Nullable min() {
return size() == 0 ? (Nullable) Nullable.empty() : Nullable.of((T) N.min((Collection) coll));
}
public Nullable min(Comparator super T> cmp) {
return size() == 0 ? (Nullable) Nullable.empty() : Nullable.of(N.min(coll, cmp));
}
@SuppressWarnings("rawtypes")
public Nullable minBy(final Function super T, ? extends Comparable> keyExtractor) {
return min(Fn.comparingBy(keyExtractor));
}
@SuppressWarnings("rawtypes")
public Nullable max() {
return size() == 0 ? (Nullable) Nullable.empty() : Nullable.of((T) N.max((Collection) coll));
}
public Nullable max(Comparator super T> cmp) {
return size() == 0 ? (Nullable) Nullable.empty() : Nullable.of(N.max(coll, cmp));
}
@SuppressWarnings("rawtypes")
public Nullable maxBy(final Function super T, ? extends Comparable> keyExtractor) {
return max(Fn.comparingBy(keyExtractor));
}
@SuppressWarnings("rawtypes")
public Nullable median() {
return size() == 0 ? (Nullable) Nullable.empty() : Nullable.of((T) N.median((Collection) coll));
}
public Nullable median(Comparator super T> cmp) {
return size() == 0 ? (Nullable) Nullable.empty() : Nullable.of(N.median(coll, cmp));
}
@SuppressWarnings("rawtypes")
public Nullable kthLargest(final int k) {
return size() < k ? (Nullable) Nullable.empty() : Nullable.of((T) N.kthLargest((Collection) coll, k));
}
public Nullable kthLargest(final int k, Comparator super T> cmp) {
return size() < k ? (Nullable) Nullable.empty() : Nullable.of(N.kthLargest(coll, k, cmp));
}
public int sumInt() {
if (N.isNullOrEmpty(coll)) {
return 0;
}
return sumInt((ToIntFunction) Fn.numToInt());
}
public int sumInt(final Try.ToIntFunction super T, E> mapper) throws E {
if (N.isNullOrEmpty(coll)) {
return 0;
}
return N.sumInt(coll, mapper);
}
public long sumLong() {
if (N.isNullOrEmpty(coll)) {
return 0L;
}
return sumLong((ToLongFunction) Fn.numToLong());
}
public long sumLong(final Try.ToLongFunction super T, E> mapper) throws E {
if (N.isNullOrEmpty(coll)) {
return 0L;
}
return N.sumLong(coll, mapper);
}
public double sumDouble() {
if (N.isNullOrEmpty(coll)) {
return 0D;
}
return sumDouble((ToDoubleFunction) Fn.numToDouble());
}
public double sumDouble(final Try.ToDoubleFunction super T, E> mapper) throws E {
if (N.isNullOrEmpty(coll)) {
return 0L;
}
return N.sumDouble(coll, mapper);
}
public OptionalDouble averageInt() {
if (N.isNullOrEmpty(coll)) {
return OptionalDouble.empty();
}
return averageInt((ToIntFunction) Fn.numToInt());
}
public OptionalDouble averageInt(final Try.ToIntFunction super T, E> mapper) throws E {
return N.averageInt(coll, mapper);
}
public OptionalDouble averageLong() {
if (N.isNullOrEmpty(coll)) {
return OptionalDouble.empty();
}
return averageLong((ToLongFunction) Fn.numToLong());
}
public OptionalDouble averageLong(final Try.ToLongFunction super T, E> mapper) throws E {
return N.averageLong(coll, mapper);
}
public OptionalDouble averageDouble() {
if (N.isNullOrEmpty(coll)) {
return OptionalDouble.empty();
}
return averageDouble((ToDoubleFunction) Fn.numToDouble());
}
public OptionalDouble averageDouble(final Try.ToDoubleFunction super T, E> mapper) throws E {
return N.averageDouble(coll, mapper);
}
public void foreach(final Try.Consumer super T, E> action) throws E {
N.forEach(coll, action);
}
// public void forEach(int fromIndex, final int toIndex, final Consumer super T> action) throws E {
// N.forEach(coll, fromIndex, toIndex, action);
// }
public void forEach(final Try.IndexedConsumer super T, E> action) throws E {
N.forEach(coll, action);
}
// public void forEach(int fromIndex, final int toIndex, final IndexedConsumer super T> action) throws E {
// N.forEach(coll, fromIndex, toIndex, action);
// }
public R forEach(final R seed, Try.BiFunction accumulator,
final Try.BiPredicate super R, ? super T, E2> conditionToBreak) throws E, E2 {
return N.forEach(coll, seed, accumulator, conditionToBreak);
}
// public R forEach(int fromIndex, final int toIndex, final R seed, final BiFunction accumulator,
// final BiPredicate super R, ? super T> conditionToBreak) throws E {
// return N.forEach(coll, fromIndex, toIndex, seed, accumulator, conditionToBreak);
// }
/**
* Execute accumulator
on each element till true
is returned by conditionToBreak
*
* @param seed The seed element is both the initial value of the reduction and the default result if there are no elements.
* @param accumulator
* @param conditionToBreak break if true
is return.
* @return
*/
public R forEach(final R seed, final Try.IndexedBiFunction accumulator,
final Try.BiPredicate super R, ? super T, E2> conditionToBreak) throws E, E2 {
return N.forEach(coll, seed, accumulator, conditionToBreak);
}
public void forEach(final Try.Function super T, ? extends Collection, E> flatMapper,
final Try.BiConsumer super T, ? super U, E2> action) throws E, E2 {
N.forEach(coll, flatMapper, action);
}
public void forEach(
final Try.Function super T, ? extends Collection, E> flatMapper, final Try.Function super T2, ? extends Collection, E2> flatMapper2,
final Try.TriConsumer super T, ? super T2, ? super T3, E3> action) throws E, E2, E3 {
N.forEach(coll, flatMapper, flatMapper2, action);
}
// public void forEachNonNull(final Consumer super T> action) throws E {
// N.forEachNonNull(coll, action);
// }
public void forEachNonNull(final Try.Function super T, ? extends Collection, E> flatMapper,
final Try.BiConsumer super T, ? super U, E2> action) throws E, E2 {
N.forEachNonNull(coll, flatMapper, action);
}
public void forEachNonNull(
final Try.Function super T, ? extends Collection, E> flatMapper, final Try.Function super T2, ? extends Collection, E2> flatMapper2,
final Try.TriConsumer super T, ? super T2, ? super T3, E3> action) throws E, E2, E3 {
N.forEachNonNull(coll, flatMapper, flatMapper2, action);
}
public void forEachPair(final Try.BiConsumer super T, ? super T, E> action) throws E {
forEachPair(action, 1);
}
public void forEachPair(final Try.BiConsumer super T, ? super T, E> action, final int increment) throws E {
final int windowSize = 2;
N.checkArgument(windowSize > 0 && increment > 0, "'windowSize'=%s and 'increment'=%s must not be less than 1", windowSize, increment);
if (N.isNullOrEmpty(coll)) {
return;
}
final Iterator iter = coll.iterator();
final T NONE = (T) N.NULL_MASK;
T prev = NONE;
while (iter.hasNext()) {
if (increment > windowSize && prev != NONE) {
int skipNum = increment - windowSize;
while (skipNum-- > 0 && iter.hasNext()) {
iter.next();
}
if (iter.hasNext() == false) {
break;
}
prev = NONE;
}
if (increment == 1) {
action.accept(prev == NONE ? iter.next() : prev, (prev = (iter.hasNext() ? iter.next() : null)));
} else {
action.accept(iter.next(), (prev = (iter.hasNext() ? iter.next() : null)));
}
}
}
public void forEachTriple(final Try.TriConsumer super T, ? super T, ? super T, E> action) throws E {
forEachTriple(action, 1);
}
public void forEachTriple(final Try.TriConsumer super T, ? super T, ? super T, E> action, final int increment) throws E {
final int windowSize = 3;
N.checkArgument(windowSize > 0 && increment > 0, "'windowSize'=%s and 'increment'=%s must not be less than 1", windowSize, increment);
if (N.isNullOrEmpty(coll)) {
return;
}
final Iterator iter = coll.iterator();
final T NONE = (T) N.NULL_MASK;
T prev = NONE;
T prev2 = NONE;
while (iter.hasNext()) {
if (increment > windowSize && prev != NONE) {
int skipNum = increment - windowSize;
while (skipNum-- > 0 && iter.hasNext()) {
iter.next();
}
if (iter.hasNext() == false) {
break;
}
prev = NONE;
}
if (increment == 1) {
action.accept(prev2 == NONE ? iter.next() : prev2, (prev2 = (prev == NONE ? (iter.hasNext() ? iter.next() : null) : prev)),
(prev = (iter.hasNext() ? iter.next() : null)));
} else if (increment == 2) {
action.accept(prev == NONE ? iter.next() : prev, (prev2 = (iter.hasNext() ? iter.next() : null)),
(prev = (iter.hasNext() ? iter.next() : null)));
} else {
action.accept(iter.next(), (prev2 = (iter.hasNext() ? iter.next() : null)), (prev = (iter.hasNext() ? iter.next() : null)));
}
}
}
// /**
// * Execute accumulator
on each element till true
is returned by conditionToBreak
// *
// * @param fromIndex
// * @param toIndex
// * @param seed The seed element is both the initial value of the reduction and the default result if there are no elements.
// * @param accumulator
// * @param conditionToBreak break if true
is return.
// * @return
// */
// public R forEach(int fromIndex, final int toIndex, final R seed, final IndexedBiFunction accumulator,
// final BiPredicate super R, ? super T> conditionToBreak) throws E {
// return N.forEach(coll, fromIndex, toIndex, seed, accumulator, conditionToBreak);
// }
public Nullable first() {
if (size() == 0) {
return Nullable.empty();
}
if (coll instanceof List && coll instanceof RandomAccess) {
return Nullable.of(((List) coll).get(0));
} else {
return Nullable.of(coll.iterator().next());
}
}
/**
* Return at most first n
elements.
*
* @param n
* @return
*/
public List first(final int n) {
N.checkArgument(n >= 0, "'n' can't be negative: " + n);
if (N.isNullOrEmpty(coll) || n == 0) {
return new ArrayList<>();
} else if (coll.size() <= n) {
return new ArrayList<>(coll);
} else if (coll instanceof List) {
return new ArrayList<>(((List) coll).subList(0, n));
} else {
return new ArrayList<>(slice(0, n));
}
}
public Nullable last() {
if (size() == 0) {
return Nullable.empty();
}
if (coll instanceof List && coll instanceof RandomAccess) {
return Nullable.of(((List) coll).get(size() - 1));
} else {
final Iterator iter = iterator();
T e = null;
while (iter.hasNext()) {
e = iter.next();
}
return Nullable.of(e);
}
}
/**
* Return at most last n
elements.
*
* @param n
* @return
*/
public List last(final int n) {
N.checkArgument(n >= 0, "'n' can't be negative: " + n);
if (N.isNullOrEmpty(coll) || n == 0) {
return new ArrayList<>();
} else if (coll.size() <= n) {
return new ArrayList<>(coll);
} else if (coll instanceof List) {
return new ArrayList<>(((List) coll).subList(coll.size() - n, coll.size()));
} else {
return new ArrayList<>(slice(coll.size() - n, coll.size()));
}
}
public Nullable findFirst(Try.Predicate super T, E> predicate) throws E {
if (size() == 0) {
return Nullable.empty();
}
for (T e : coll) {
if (predicate.test(e)) {
return Nullable.of(e);
}
}
return Nullable.empty();
}
public Nullable findLast(Try.Predicate super T, E> predicate) throws E {
if (size() == 0) {
return Nullable.empty();
}
if (coll instanceof List) {
final List list = (List) coll;
if (coll instanceof RandomAccess) {
for (int i = size() - 1; i >= 0; i--) {
if (predicate.test(list.get(i))) {
return Nullable.of(list.get(i));
}
}
} else {
final ListIterator iter = list.listIterator(list.size());
T pre = null;
while (iter.hasPrevious()) {
if (predicate.test((pre = iter.previous()))) {
return Nullable.of(pre);
}
}
}
return Nullable.empty();
} else if (coll instanceof Deque) {
final Iterator iter = ((Deque) coll).descendingIterator();
T next = null;
while (iter.hasNext()) {
if (predicate.test((next = iter.next()))) {
return Nullable.of(next);
}
}
return Nullable.empty();
} else {
final T[] a = (T[]) coll.toArray();
for (int i = a.length - 1; i >= 0; i--) {
if (predicate.test(a[i])) {
return Nullable.of(a[i]);
}
}
return Nullable.empty();
}
}
public OptionalInt findFirstIndex(Try.Predicate super T, E> predicate) throws E {
if (size() == 0) {
return OptionalInt.empty();
}
int idx = 0;
for (T e : coll) {
if (predicate.test(e)) {
return OptionalInt.of(idx);
}
idx++;
}
return OptionalInt.empty();
}
public OptionalInt findLastIndex(Try.Predicate super T, E> predicate) throws E {
if (size() == 0) {
return OptionalInt.empty();
}
if (coll instanceof List) {
final List list = (List) coll;
if (coll instanceof RandomAccess) {
for (int i = size() - 1; i >= 0; i--) {
if (predicate.test(list.get(i))) {
return OptionalInt.of(i);
}
}
} else {
final ListIterator iter = list.listIterator(list.size());
for (int i = size() - 1; iter.hasPrevious(); i--) {
if (predicate.test(iter.previous())) {
return OptionalInt.of(i);
}
}
}
return OptionalInt.empty();
} else if (coll instanceof Deque) {
final Iterator iter = ((Deque) coll).descendingIterator();
for (int i = coll.size() - 1; iter.hasNext(); i--) {
if (predicate.test(iter.next())) {
return OptionalInt.of(i);
}
}
return OptionalInt.empty();
} else {
final T[] a = (T[]) coll.toArray();
for (int i = a.length - 1; i >= 0; i--) {
if (predicate.test(a[i])) {
return OptionalInt.of(i);
}
}
return OptionalInt.empty();
}
}
public Nullable findFirstOrLast(final Try.Predicate super T, E> predicateForFirst,
final Try.Predicate super T, E2> predicateForLast) throws E, E2 {
if (N.isNullOrEmpty(coll)) {
return Nullable. empty();
}
final Nullable res = findFirst(predicateForFirst);
return res.isPresent() ? res : findLast(predicateForLast);
}
public OptionalInt findFirstOrLastIndex(final Try.Predicate super T, E> predicateForFirst,
final Try.Predicate super T, E2> predicateForLast) throws E, E2 {
if (N.isNullOrEmpty(coll)) {
return OptionalInt.empty();
}
final OptionalInt res = findFirstIndex(predicateForFirst);
return res.isPresent() ? res : findLastIndex(predicateForLast);
}
public Pair, Nullable> findFirstAndLast(final Try.Predicate super T, E> predicate) throws E {
return findFirstAndLast(predicate, predicate);
}
public Pair, Nullable> findFirstAndLast(final Try.Predicate super T, E> predicateForFirst,
final Try.Predicate super T, E2> predicateForLast) throws E, E2 {
if (N.isNullOrEmpty(coll)) {
return Pair.of(Nullable. empty(), Nullable. empty());
}
return Pair.of(findFirst(predicateForFirst), findLast(predicateForLast));
}
public Pair findFirstAndLastIndex(final Try.Predicate super T, E> predicate) throws E {
return findFirstAndLastIndex(predicate, predicate);
}
public Pair findFirstAndLastIndex(final Try.Predicate super T, E> predicateForFirst,
final Try.Predicate super T, E2> predicateForLast) throws E, E2 {
if (N.isNullOrEmpty(coll)) {
return Pair.of(OptionalInt.empty(), OptionalInt.empty());
}
return Pair.of(findFirstIndex(predicateForFirst), findLastIndex(predicateForLast));
}
public boolean allMatch(Try.Predicate super T, E> filter) throws E {
if (N.isNullOrEmpty(coll)) {
return true;
}
for (T e : coll) {
if (filter.test(e) == false) {
return false;
}
}
return true;
}
public boolean anyMatch(Try.Predicate super T, E> filter) throws E {
if (N.isNullOrEmpty(coll)) {
return false;
}
for (T e : coll) {
if (filter.test(e)) {
return true;
}
}
return false;
}
public boolean noneMatch(Try.Predicate super T, E> filter) throws E {
if (N.isNullOrEmpty(coll)) {
return true;
}
for (T e : coll) {
if (filter.test(e)) {
return false;
}
}
return true;
}
public boolean hasDuplicates() {
return N.hasDuplicates(coll, false);
}
public int count(Try.Predicate super T, E> filter) throws E {
return N.count(coll, filter);
}
public List filter(Try.Predicate super T, E> filter) throws E {
return N.filter(coll, filter);
}
public List filter(Try.Predicate super T, E> filter, final int max) throws E {
return N.filter(coll, filter, max);
}
public List filter(final U seed, final Try.BiPredicate super T, ? super U, E> predicate) throws E {
return filter(new Try.Predicate() {
@Override
public boolean test(T value) throws E {
return predicate.test(value, seed);
}
});
}
public List takeWhile(Try.Predicate super T, E> filter) throws E {
final List result = new ArrayList<>(N.min(9, size()));
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
if (filter.test(e)) {
result.add(e);
} else {
break;
}
}
return result;
}
public List takeWhile(final U seed, final Try.BiPredicate super T, ? super U, E> predicate) throws E {
return takeWhile(new Try.Predicate() {
@Override
public boolean test(T value) throws E {
return predicate.test(value, seed);
}
});
}
public List takeWhileInclusive(Try.Predicate super T, E> filter) throws E {
final List result = new ArrayList<>(N.min(9, size()));
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.add(e);
if (filter.test(e) == false) {
break;
}
}
return result;
}
public List takeWhileInclusive(final U seed, final Try.BiPredicate super T, ? super U, E> predicate) throws E {
return takeWhileInclusive(new Try.Predicate() {
@Override
public boolean test(T value) throws E {
return predicate.test(value, seed);
}
});
}
public List dropWhile(Try.Predicate super T, E> filter) throws E {
final List result = new ArrayList<>(N.min(9, size()));
if (N.isNullOrEmpty(coll)) {
return result;
}
final Iterator iter = iterator();
T e = null;
while (iter.hasNext()) {
e = iter.next();
if (filter.test(e) == false) {
result.add(e);
break;
}
}
while (iter.hasNext()) {
result.add(iter.next());
}
return result;
}
public List dropWhile(final U seed, final Try.BiPredicate super T, ? super U, E> predicate) throws E {
return dropWhile(new Try.Predicate() {
@Override
public boolean test(T value) throws E {
return predicate.test(value, seed);
}
});
}
public List skipUntil(final Try.Predicate super T, E> filter) throws E {
return dropWhile(new Try.Predicate() {
@Override
public boolean test(T value) throws E {
return !filter.test(value);
}
});
}
public List skipUntil(final U seed, final Try.BiPredicate super T, ? super U, E> predicate) throws E {
return dropWhile(new Try.Predicate() {
@Override
public boolean test(T value) throws E {
return !predicate.test(value, seed);
}
});
}
public List map(final Try.Function super T, ? extends R, E> func) throws E {
return N.map(coll, func);
}
public BooleanList mapToBoolean(final Try.ToBooleanFunction super T, E> func) throws E {
return N.mapToBoolean(coll, func);
}
public CharList mapToChar(final Try.ToCharFunction super T, E> func) throws E {
return N.mapToChar(coll, func);
}
public ByteList mapToByte(final Try.ToByteFunction super T, E> func) throws E {
return N.mapToByte(coll, func);
}
public ShortList mapToShort(final Try.ToShortFunction super T, E> func) throws E {
return N.mapToShort(coll, func);
}
public IntList mapToInt(final Try.ToIntFunction super T, E> func) throws E {
return N.mapToInt(coll, func);
}
public LongList mapToLong(final Try.ToLongFunction super T, E> func) throws E {
return N.mapToLong(coll, func);
}
public FloatList mapToFloat(final Try.ToFloatFunction super T, E> func) throws E {
return N.mapToFloat(coll, func);
}
public DoubleList mapToDouble(final Try.ToDoubleFunction super T, E> func) throws E {
return N.mapToDouble(coll, func);
}
public List flatMap(final Try.Function super T, ? extends Collection extends R>, E> func) throws E {
final List result = new ArrayList<>(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public List flattMap(final Try.Function super T, ? extends R[], E> func) throws E {
final List result = new ArrayList<>(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
R[] a = null;
for (T e : coll) {
a = func.apply(e);
if (N.notNullOrEmpty(a)) {
if (a.length < 9) {
for (R r : a) {
result.add(r);
}
} else {
result.addAll(Arrays.asList(a));
}
}
}
return result;
}
public BooleanList flatMapToBoolean(final Try.Function super T, ? extends Collection, E> func) throws E {
final BooleanList result = new BooleanList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (boolean b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public BooleanList flattMapToBoolean(final Try.Function super T, boolean[], E> func) throws E {
final BooleanList result = new BooleanList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public CharList flatMapToChar(final Try.Function super T, ? extends Collection, E> func) throws E {
final CharList result = new CharList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (char b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public CharList flattMapToChar(final Try.Function super T, char[], E> func) throws E {
final CharList result = new CharList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public ByteList flatMapToByte(final Try.Function super T, ? extends Collection, E> func) throws E {
final ByteList result = new ByteList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (byte b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public ByteList flattMapToByte(final Try.Function super T, byte[], E> func) throws E {
final ByteList result = new ByteList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public ShortList flatMapToShort(final Try.Function super T, ? extends Collection, E> func) throws E {
final ShortList result = new ShortList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (short b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public ShortList flattMapToShort(final Try.Function super T, short[], E> func) throws E {
final ShortList result = new ShortList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public IntList flatMapToInt(final Try.Function super T, ? extends Collection, E> func) throws E {
final IntList result = new IntList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (int b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public IntList flattMapToInt(final Try.Function super T, int[], E> func) throws E {
final IntList result = new IntList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public LongList flatMapToLong(final Try.Function super T, ? extends Collection, E> func) throws E {
final LongList result = new LongList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (long b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public LongList flattMapToLong(final Try.Function super T, long[], E> func) throws E {
final LongList result = new LongList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public FloatList flatMapToFloat(final Try.Function super T, ? extends Collection, E> func) throws E {
final FloatList result = new FloatList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (float b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public FloatList flattMapToFloat(final Try.Function super T, float[], E> func) throws E {
final FloatList result = new FloatList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public DoubleList flatMapToDouble(final Try.Function super T, ? extends Collection, E> func) throws E {
final DoubleList result = new DoubleList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
for (double b : func.apply(e)) {
result.add(b);
}
}
return result;
}
public DoubleList flattMapToDouble(final Try.Function super T, double[], E> func) throws E {
final DoubleList result = new DoubleList(size() > N.MAX_ARRAY_SIZE / 2 ? N.MAX_ARRAY_SIZE : size() * 2);
if (N.isNullOrEmpty(coll)) {
return result;
}
for (T e : coll) {
result.addAll(func.apply(e));
}
return result;
}
public List flatMap(final Try.Function super T, ? extends Collection, E> mapper,
final Try.BiFunction super T, ? super U, ? extends R, E2> func) throws E, E2 {
if (N.isNullOrEmpty(coll)) {
return new ArrayList();
}
final List result = new ArrayList(N.max(9, coll.size()));
for (T e : coll) {
final Collection c = mapper.apply(e);
if (N.notNullOrEmpty(c)) {
for (U u : c) {
result.add(func.apply(e, u));
}
}
}
return result;
}
public List flatMap(
final Try.Function super T, ? extends Collection, E> mapper2, final Try.Function super T2, ? extends Collection, E2> mapper3,
final Try.TriFunction super T, ? super T2, ? super T3, R, E3> func) throws E, E2, E3 {
if (N.isNullOrEmpty(coll)) {
return new ArrayList();
}
final List result = new ArrayList(N.max(9, coll.size()));
for (T e : coll) {
final Collection c2 = mapper2.apply(e);
if (N.notNullOrEmpty(c2)) {
for (T2 t2 : c2) {
final Collection c3 = mapper3.apply(t2);
if (N.notNullOrEmpty(c3)) {
for (T3 t3 : c3) {
result.add(func.apply(e, t2, t3));
}
}
}
}
}
return result;
}
/**
* Merge series of adjacent elements which satisfy the given predicate using
* the merger function and return a new stream.
*
*
* This method only run sequentially, even in parallel stream.
*
* @param collapsible
* @param mergeFunction
* @return
*/
public List collapse(final Try.BiPredicate super T, ? super T, E> collapsible,
final Try.BiFunction super T, ? super T, T, E2> mergeFunction) throws E, E2 {
final List result = new ArrayList<>();
if (N.isNullOrEmpty(coll)) {
return result;
}
final Iterator iter = iterator();
boolean hasNext = false;
T next = null;
while (hasNext || iter.hasNext()) {
T res = hasNext ? next : (next = iter.next());
while ((hasNext = iter.hasNext())) {
if (collapsible.test(next, (next = iter.next()))) {
res = mergeFunction.apply(res, next);
} else {
break;
}
}
result.add(res);
}
return result;
}
/**
* Merge series of adjacent elements which satisfy the given predicate using
* the merger function and return a new stream.
*
*
* This method only run sequentially, even in parallel stream.
*
* @param collapsible
* @param collector
* @return
*/
public List collapse(final Try.BiPredicate super T, ? super T, E> collapsible, final Collector super T, A, R> collector)
throws E {
final List result = new ArrayList<>();
if (N.isNullOrEmpty(coll)) {
return result;
}
final Supplier supplier = collector.supplier();
final BiConsumer accumulator = collector.accumulator();
final Function finisher = collector.finisher();
final Iterator iter = iterator();
boolean hasNext = false;
T next = null;
while (hasNext || iter.hasNext()) {
final A c = supplier.get();
accumulator.accept(c, hasNext ? next : (next = iter.next()));
while ((hasNext = iter.hasNext())) {
if (collapsible.test(next, (next = iter.next()))) {
accumulator.accept(c, next);
} else {
break;
}
}
result.add(finisher.apply(c));
}
return result;
}
/**
* Returns a {@code Stream} produced by iterative application of a accumulation function
* to an initial element {@code identity} and next element of the current stream.
* Produces a {@code Stream} consisting of {@code identity}, {@code acc(identity, value1)},
* {@code acc(acc(identity, value1), value2)}, etc.
*
* This is an intermediate operation.
*
*
Example:
*
* accumulator: (a, b) -> a + b
* stream: [1, 2, 3, 4, 5]
* result: [1, 3, 6, 10, 15]
*
*
*
* This method only run sequentially, even in parallel stream.
*
* @param accumulator the accumulation function
* @return the new stream which has the extract same size as this stream.
*/
public List scan(final Try.BiFunction super T, ? super T, T, E> accumulator) throws E {
final List result = new ArrayList<>();
if (N.isNullOrEmpty(coll)) {
return result;
}
final Iterator iter = iterator();
T next = null;
if (iter.hasNext()) {
result.add((next = iter.next()));
}
while (iter.hasNext()) {
result.add((next = accumulator.apply(next, iter.next())));
}
return result;
}
/**
* Returns a {@code Stream} produced by iterative application of a accumulation function
* to an initial element {@code identity} and next element of the current stream.
* Produces a {@code Stream} consisting of {@code identity}, {@code acc(identity, value1)},
* {@code acc(acc(identity, value1), value2)}, etc.
*
* This is an intermediate operation.
*
*
Example:
*
* seed:10
* accumulator: (a, b) -> a + b
* stream: [1, 2, 3, 4, 5]
* result: [11, 13, 16, 20, 25]
*
*
*
* This method only run sequentially, even in parallel stream.
*
* @param seed the initial value. it's only used once by accumulator
to calculate the fist element in the returned stream.
* It will be ignored if this stream is empty and won't be the first element of the returned stream.
*
* @param accumulator the accumulation function
* @return the new stream which has the extract same size as this stream.
*/
public List scan(final R seed, final Try.BiFunction super R, ? super T, R, E> accumulator) throws E {
final List result = new ArrayList<>();
if (N.isNullOrEmpty(coll)) {
return result;
}
final Iterator iter = iterator();
R next = seed;
while (iter.hasNext()) {
result.add((next = accumulator.apply(next, iter.next())));
}
return result;
}
/**
* This is equivalent to:
*
*
* if (isEmpty()) {
* return Nullable.empty();
* }
*
* final Iterator iter = iterator();
* T result = iter.next();
*
* while (iter.hasNext()) {
* result = accumulator.apply(result, iter.next());
* }
*
* return Nullable.of(result);
*
*
*
* @param accumulator
* @return
*/
public Nullable reduce(Try.BinaryOperator accumulator) throws E {
if (isEmpty()) {
return Nullable.empty();
}
final Iterator iter = iterator();
T result = iter.next();
while (iter.hasNext()) {
result = accumulator.apply(result, iter.next());
}
return Nullable.of(result);
}
/**
* This is equivalent to:
*
*
* if (isEmpty()) {
* return identity;
* }
*
* final Iterator iter = iterator();
* U result = identity;
*
* while (iter.hasNext()) {
* result = accumulator.apply(result, iter.next());
* }
*
* return result;
*
*
*
* @param identity
* @param accumulator
* @return
*/
public U reduce(U identity, Try.BiFunction accumulator) throws E {
if (isEmpty()) {
return identity;
}
final Iterator iter = iterator();
U result = identity;
while (iter.hasNext()) {
result = accumulator.apply(result, iter.next());
}
return result;
}
public R collect(final Supplier supplier, final Try.BiConsumer accumulator) throws E {
final R result = supplier.get();
if (N.notNullOrEmpty(coll)) {
for (T e : coll) {
accumulator.accept(result, e);
}
}
return result;
}
public R collect(final Collector super T, A, R> collector) {
final BiConsumer accumulator = collector.accumulator();
final A result = collector.supplier().get();
if (N.notNullOrEmpty(coll)) {
for (T e : coll) {
accumulator.accept(result, e);
}
}
return collector.finisher().apply(result);
}
public