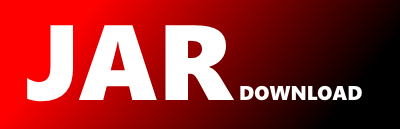
com.landawn.abacus.util.Try Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-util-all Show documentation
Show all versions of abacus-util-all Show documentation
A general programming library in Java
/*
* Copyright (C) 2016 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.util;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.Reader;
import java.io.Writer;
import com.landawn.abacus.util.stream.Stream;
/**
* Catch checked exception and convert it to RuntimeException
.
*
* @since 0.8
*
* @author Haiyang Li
*/
public final class Try {
private final T t;
Try(final T t) {
this.t = t;
}
public static Try of(final T t) {
return new Try<>(t);
}
public static Try of(final Supplier supplier) {
try {
return new Try<>(supplier.get());
} catch (Exception e) {
throw N.toRuntimeException(e);
}
}
public static Try reader(final File file) {
try {
return of((Reader) new FileReader(file));
} catch (FileNotFoundException e) {
throw N.toRuntimeException(e);
}
}
public static Try writer(final File file) {
try {
return of((Writer) new FileWriter(file));
} catch (IOException e) {
throw N.toRuntimeException(e);
}
}
public static Try> stream(final File file) {
final Reader reader = IOUtil.newBufferedReader(file);
return new Try<>(Stream.of(reader).onClose(new java.lang.Runnable() {
@Override
public void run() {
IOUtil.close(reader);
}
}));
}
// public static java.lang.Runnable of(final Try.Runnable run) {
// return new java.lang.Runnable() {
// @Override
// public void run() {
// try {
// run.run();
// } catch (Exception e) {
// throw N.toRuntimeException(e);
// }
// }
// };
// }
//
// public static Try.Callable of(final java.util.concurrent.Callable call) {
// return new Try.Callable() {
// @Override
// public R call() {
// try {
// return call.call();
// } catch (Exception e) {
// throw N.toRuntimeException(e);
// }
// }
// };
// }
/**
*
* @param cmd
* @throws RuntimeException if some error happens
*/
public static void run(final Try.Runnable extends Exception> cmd) {
try {
cmd.run();
} catch (Exception e) {
throw N.toRuntimeException(e);
}
}
public static void run(final Try.Runnable extends Exception> cmd, final com.landawn.abacus.util.function.Consumer super Exception> actionOnError) {
N.requireNonNull(actionOnError);
try {
cmd.run();
} catch (Exception e) {
actionOnError.accept(e);
}
}
/**
*
* @param cmd
* @return
* @throws RuntimeException if some error happens
*/
public static R call(final java.util.concurrent.Callable cmd) {
try {
return cmd.call();
} catch (Exception e) {
throw N.toRuntimeException(e);
}
}
public static R call(final java.util.concurrent.Callable cmd, final com.landawn.abacus.util.function.Function super Exception, R> actionOnError) {
N.requireNonNull(actionOnError);
try {
return cmd.call();
} catch (Exception e) {
return actionOnError.apply(e);
}
}
public static R call(final java.util.concurrent.Callable cmd, final com.landawn.abacus.util.function.Supplier supplier) {
N.requireNonNull(supplier);
try {
return cmd.call();
} catch (Exception e) {
return supplier.get();
}
}
public static R call(final java.util.concurrent.Callable cmd, final R defaultValue) {
try {
return cmd.call();
} catch (Exception e) {
return defaultValue;
}
}
/**
*
* @param cmd
* @param predicate
* @param supplier
* @return the value returned Supplier.get()
if some error happens and predicate
return true.
* @throws RuntimeException if some error happens and predicate
return false.
*/
public static R call(final java.util.concurrent.Callable cmd, final com.landawn.abacus.util.function.Predicate super Exception> predicate,
final com.landawn.abacus.util.function.Supplier supplier) {
N.requireNonNull(predicate);
N.requireNonNull(supplier);
try {
return cmd.call();
} catch (Exception e) {
if (predicate.test(e)) {
return supplier.get();
} else {
throw N.toRuntimeException(e);
}
}
}
/**
*
* @param cmd
* @param predicate
* @param defaultValue
* @return the defaultValue()
if some error happens and predicate
return true.
* @throws RuntimeException if some error happens and predicate
return false.
*/
public static R call(final java.util.concurrent.Callable cmd, final com.landawn.abacus.util.function.Predicate super Exception> predicate,
final R defaultValue) {
N.requireNonNull(predicate);
try {
return cmd.call();
} catch (Exception e) {
if (predicate.test(e)) {
return defaultValue;
} else {
throw N.toRuntimeException(e);
}
}
}
public void run(final Try.Consumer super T, ? extends Exception> cmd) {
try {
cmd.accept(t);
} catch (Exception e) {
throw N.toRuntimeException(e);
} finally {
IOUtil.close(t);
}
}
public void run(final Try.Consumer super T, ? extends Exception> cmd, final com.landawn.abacus.util.function.Consumer super Exception> actionOnError) {
N.requireNonNull(actionOnError);
try {
cmd.accept(t);
} catch (Exception e) {
actionOnError.accept(e);
} finally {
IOUtil.close(t);
}
}
public R call(final Try.Function super T, R, ? extends Exception> cmd) {
try {
return cmd.apply(t);
} catch (Exception e) {
throw N.toRuntimeException(e);
} finally {
IOUtil.close(t);
}
}
public R call(final Try.Function super T, R, ? extends Exception> cmd,
final com.landawn.abacus.util.function.Function super Exception, R> actionOnError) {
N.requireNonNull(actionOnError);
try {
return cmd.apply(t);
} catch (Exception e) {
return actionOnError.apply(e);
} finally {
IOUtil.close(t);
}
}
public R call(final Try.Function super T, R, ? extends Exception> cmd, final com.landawn.abacus.util.function.Supplier supplier) {
N.requireNonNull(supplier);
try {
return cmd.apply(t);
} catch (Exception e) {
return supplier.get();
} finally {
IOUtil.close(t);
}
}
public R call(final Try.Function super T, R, ? extends Exception> cmd, final R defaultValue) {
try {
return cmd.apply(t);
} catch (Exception e) {
return defaultValue;
} finally {
IOUtil.close(t);
}
}
public R call(final Try.Function super T, R, ? extends Exception> cmd, final com.landawn.abacus.util.function.Predicate super Exception> predicate,
final com.landawn.abacus.util.function.Supplier supplier) {
N.requireNonNull(predicate);
N.requireNonNull(supplier);
try {
return cmd.apply(t);
} catch (Exception e) {
if (predicate.test(e)) {
return supplier.get();
} else {
throw N.toRuntimeException(e);
}
} finally {
IOUtil.close(t);
}
}
public R call(final Try.Function super T, R, ? extends Exception> cmd, final com.landawn.abacus.util.function.Predicate super Exception> predicate,
final R defaultValue) {
N.requireNonNull(predicate);
try {
return cmd.apply(t);
} catch (Exception e) {
if (predicate.test(e)) {
return defaultValue;
} else {
throw N.toRuntimeException(e);
}
} finally {
IOUtil.close(t);
}
}
public static interface Callable extends java.util.concurrent.Callable {
@Override
R call() throws E;
}
public static interface Runnable {
void run() throws E;
}
public static interface Supplier {
T get() throws E;
}
public static interface Predicate {
boolean test(T t) throws E;
default Predicate negate() throws E {
return (t) -> !test(t);
}
}
public static interface BiPredicate {
boolean test(T t, U u) throws E;
default BiPredicate negate() throws E {
return (T t, U u) -> !test(t, u);
}
}
public static interface TriPredicate {
boolean test(A a, B b, C c) throws E;
}
public static interface Function {
R apply(T t) throws E;
}
public static interface BiFunction {
R apply(T t, U u) throws E;
}
public static interface TriFunction {
R apply(A a, B b, C c) throws E;
}
public static interface Consumer {
void accept(T t) throws E;
}
public static interface BiConsumer {
void accept(T t, U u) throws E;
}
public static interface TriConsumer {
void accept(A a, B b, C c) throws E;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy