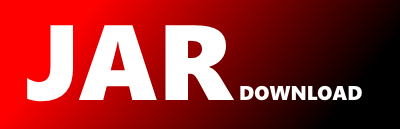
com.landawn.abacus.condition.Criteria Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-util-se Show documentation
Show all versions of abacus-util-se Show documentation
A general programming library in Java/Android. It's easy to learn and simple to use with concise and powerful APIs.
/*
* Copyright (C) 2015 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.condition;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.landawn.abacus.condition.ConditionFactory.CF;
import com.landawn.abacus.util.N;
import com.landawn.abacus.util.NamingPolicy;
import com.landawn.abacus.util.SortDirection;
import com.landawn.abacus.util.WD;
// TODO: Auto-generated Javadoc
/**
* At present, Supports
* {@code Where, OrderBy, GroupBy, Having, Join, Limit, ForUpdate, Union, UnionAll, Intersect, Except} clause. Each
* {@code clause} is independent. A {@code clause} should not be included in another {@code clause}. If there more than
* one {@code clause}, they should be composed in one {@code Criteria} condition.
*
* @author Haiyang Li
* @since 0.8
*/
public class Criteria extends AbstractCondition {
/** The Constant serialVersionUID. */
private static final long serialVersionUID = 7534336752211519239L;
/** The Constant aggregationOperators. */
private static final Set aggregationOperators = N.newHashSet();
static {
aggregationOperators.add(Operator.UNION_ALL);
aggregationOperators.add(Operator.UNION);
aggregationOperators.add(Operator.INTERSECT);
aggregationOperators.add(Operator.EXCEPT);
aggregationOperators.add(Operator.MINUS);
}
/** The distinct. */
private boolean distinct = false;
/** The condition list. */
private List conditionList;
/**
* Instantiates a new criteria.
*/
public Criteria() {
super(Operator.EMPTY);
conditionList = new ArrayList<>();
}
/**
* Checks if is distinct.
*
* @return true, if is distinct
*/
public boolean isDistinct() {
return distinct;
}
/**
* Gets the joins.
*
* @return
*/
public List getJoins() {
List joins = new ArrayList<>();
for (Condition cond : conditionList) {
if (cond instanceof Join) {
joins.add((Join) cond);
}
}
return joins;
}
/**
* Gets the where.
*
* @return
*/
public Cell getWhere() {
return (Cell) find(Operator.WHERE);
}
/**
* Gets the group by.
*
* @return
*/
public Cell getGroupBy() {
return (Cell) find(Operator.GROUP_BY);
}
/**
* Gets the having.
*
* @return
*/
public Cell getHaving() {
return (Cell) find(Operator.HAVING);
}
/**
* Gets the aggregation.
*
* @return
*/
@SuppressWarnings("unchecked")
public List getAggregation() {
List result = null;
for (Condition cond : conditionList) {
if (aggregationOperators.contains(cond.getOperator())) {
if (result == null) {
result = new ArrayList<>();
}
result.add((Cell) cond);
}
}
if (result == null) {
result = N.emptyList();
}
return result;
}
/**
* Gets the order by.
*
* @return
*/
public Cell getOrderBy() {
return (Cell) find(Operator.ORDER_BY);
}
/**
* Gets the limit.
*
* @return
*/
public Limit getLimit() {
return (Limit) find(Operator.LIMIT);
}
/**
* Gets the conditions.
*
* @return
*/
public List getConditions() {
return conditionList;
}
/**
*
* @param operator
* @return
*/
public List get(Operator operator) {
List conditions = new ArrayList<>();
for (Condition cond : conditionList) {
if (cond.getOperator().equals(operator)) {
conditions.add(cond);
}
}
return conditions;
}
/**
*
* @param conditions
*/
void add(Condition... conditions) {
addConditions(conditions);
}
/**
*
* @param conditions
*/
void add(Collection extends Condition> conditions) {
addConditions(conditions);
}
/**
*
* @param operator
*/
void remove(Operator operator) {
List conditions = get(operator);
remove(conditions);
}
/**
*
* @param conditions
*/
void remove(Condition... conditions) {
for (Condition cond : conditions) {
conditionList.remove(cond);
}
}
/**
*
* @param conditions
*/
void remove(Collection extends Condition> conditions) {
conditionList.removeAll(conditions);
}
/**
* Clear.
*/
public void clear() {
conditionList.clear();
}
/**
* Gets the parameters.
*
* @return
*/
@SuppressWarnings("unchecked")
@Override
public List | |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy