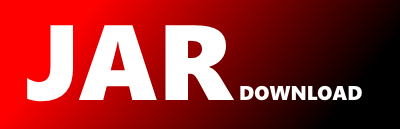
com.landawn.abacus.util.CommonUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-util-se Show documentation
Show all versions of abacus-util-se Show documentation
A general programming library in Java/Android. It's easy to learn and simple to use with concise and powerful APIs.
/*
* Copyright (c) 2015, Haiyang Li.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.landawn.abacus.util;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.io.Serializable;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.lang.reflect.Proxy;
import java.nio.charset.Charset;
import java.security.SecureRandom;
import java.util.AbstractMap;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.Deque;
import java.util.EnumMap;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.HashSet;
import java.util.IdentityHashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.NavigableMap;
import java.util.NavigableSet;
import java.util.PriorityQueue;
import java.util.Queue;
import java.util.Random;
import java.util.RandomAccess;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TreeMap;
import java.util.TreeSet;
import java.util.UUID;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedDeque;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.DelayQueue;
import java.util.concurrent.Delayed;
import java.util.concurrent.LinkedBlockingDeque;
import java.util.concurrent.LinkedBlockingQueue;
import com.landawn.abacus.DataSet;
import com.landawn.abacus.DirtyMarker;
import com.landawn.abacus.annotation.NullSafe;
import com.landawn.abacus.core.DirtyMarkerUtil;
import com.landawn.abacus.core.MapEntity;
import com.landawn.abacus.core.RowDataSet;
import com.landawn.abacus.parser.ParserUtil;
import com.landawn.abacus.parser.ParserUtil.EntityInfo;
import com.landawn.abacus.parser.ParserUtil.PropInfo;
import com.landawn.abacus.type.Type;
import com.landawn.abacus.type.TypeFactory;
import com.landawn.abacus.util.Iterables.Slice;
import com.landawn.abacus.util.u.Nullable;
import com.landawn.abacus.util.u.Optional;
import com.landawn.abacus.util.u.OptionalInt;
import com.landawn.abacus.util.function.Function;
import com.landawn.abacus.util.function.IntFunction;
import com.landawn.abacus.util.function.Supplier;
import com.landawn.abacus.util.function.ToDoubleFunction;
import com.landawn.abacus.util.function.ToIntFunction;
import com.landawn.abacus.util.function.ToLongFunction;
// TODO: Auto-generated Javadoc
/**
*
* Note: This class includes codes copied from Apache Commons Lang, Google Guava and other open source projects under the Apache License 2.0.
* The methods copied from other libraries/frameworks/projects may be modified in this class.
*
* Class N
is a general java utility class. It provides the most daily used operations for Object/primitive types/String/Array/Collection/Map/Entity...:
*
* When to throw exception? It's designed to avoid throwing any unnecessary
* exception if the contract defined by method is not broken. for example, if
* user tries to reverse a null or empty String. the input String will be
* returned. But exception will be thrown if trying to repeat/swap a null or
* empty string or operate Array/Collection by adding/removing...
*
* @author Haiyang Li
*
* @version $Revision: 0.8 $ 07/03/10
*
* @see com.landawn.abacus.util.IOUtil
* @see com.landawn.abacus.util.StringUtil
* @see com.landawn.abacus.util.Iterables
* @see com.landawn.abacus.util.Iterators
* @see com.landawn.abacus.util.Maps
* @see com.landawn.abacus.util.Primitives
* @see com.landawn.abacus.util.Array
* @see com.landawn.abacus.util.Seq
*/
class CommonUtil {
// ... it has to be big enough to make it's safety to add element to
/** The Constant POOL_SIZE. */
// ArrayBlockingQueue.
static final int POOL_SIZE = Internals.POOL_SIZE;
/**
* An empty immutable {@code Boolean} array.
*/
static final Boolean[] EMPTY_BOOLEAN_OBJECT_ARRAY = new Boolean[0];
/**
* An empty immutable {@code Character} array.
*/
static final Character[] EMPTY_CHARACTER_OBJECT_ARRAY = new Character[0];
/**
* An empty immutable {@code Byte} array.
*/
static final Byte[] EMPTY_BYTE_OBJECT_ARRAY = new Byte[0];
/**
* An empty immutable {@code Short} array.
*/
static final Short[] EMPTY_SHORT_OBJECT_ARRAY = new Short[0];
/**
* An empty immutable {@code Integer} array.
*/
static final Integer[] EMPTY_INTEGER_OBJECT_ARRAY = new Integer[0];
/**
* An empty immutable {@code Long} array.
*/
static final Long[] EMPTY_LONG_OBJECT_ARRAY = new Long[0];
/**
* An empty immutable {@code Float} array.
*/
static final Float[] EMPTY_FLOAT_OBJECT_ARRAY = new Float[0];
/**
* An empty immutable {@code Double} array.
*/
static final Double[] EMPTY_DOUBLE_OBJECT_ARRAY = new Double[0];
/**
* An empty immutable {@code Class} array.
*/
static final Class>[] EMPTY_CLASS_ARRAY = new Class[0];
/** The Constant NULL_STRING. */
static final String NULL_STRING = "null".intern();
/** The Constant NULL_CHAR_ARRAY. */
static final char[] NULL_CHAR_ARRAY = NULL_STRING.toCharArray();
/** The Constant TRUE. */
static final String TRUE = Boolean.TRUE.toString().intern();
/** The Constant TRUE_CHAR_ARRAY. */
static final char[] TRUE_CHAR_ARRAY = TRUE.toCharArray();
/** The Constant FALSE. */
static final String FALSE = Boolean.FALSE.toString().intern();
/** The Constant FALSE_CHAR_ARRAY. */
static final char[] FALSE_CHAR_ARRAY = FALSE.toCharArray();
/** The Constant CHAR_0. */
// ...
static final char CHAR_0 = WD.CHAR_0;
/**
* The Constant CHAR_LF.
*
* @see JLF:
* Escape Sequences for Character and String Literals
* @since 2.2
*/
static final char CHAR_LF = WD.CHAR_LF;
/**
* The Constant CHAR_CR.
*
* @see JLF:
* Escape Sequences for Character and String Literals
* @since 2.2
*/
static final char CHAR_CR = WD.CHAR_CR;
/** The element separator. */
public static final String ELEMENT_SEPARATOR = ", ".intern();
/** The element separator char array. */
public static final char[] ELEMENT_SEPARATOR_CHAR_ARRAY = ELEMENT_SEPARATOR.toCharArray();
// ...
/**
* The index value when an element is not found in a list or array:
* {@code -1}. This value is returned by methods in this class and can also
* be used in comparisons with values returned by various method from
* {@link java.util.List} .
*/
public static final int INDEX_NOT_FOUND = -1;
/** The Constant EMPTY_STRING. */
// ...
public static final String EMPTY_STRING = "".intern();
/**
* An empty immutable {@code boolean} array.
*/
public static final boolean[] EMPTY_BOOLEAN_ARRAY = new boolean[0];
/**
* An empty immutable {@code char} array.
*/
public static final char[] EMPTY_CHAR_ARRAY = new char[0];
/**
* An empty immutable {@code byte} array.
*/
public static final byte[] EMPTY_BYTE_ARRAY = new byte[0];
/**
* An empty immutable {@code short} array.
*/
public static final short[] EMPTY_SHORT_ARRAY = new short[0];
/**
* An empty immutable {@code int} array.
*/
public static final int[] EMPTY_INT_ARRAY = new int[0];
/**
* An empty immutable {@code long} array.
*/
public static final long[] EMPTY_LONG_ARRAY = new long[0];
/**
* An empty immutable {@code float} array.
*/
public static final float[] EMPTY_FLOAT_ARRAY = new float[0];
/**
* An empty immutable {@code double} array.
*/
public static final double[] EMPTY_DOUBLE_ARRAY = new double[0];
/**
* An empty immutable {@code Boolean} array.
*/
public static final Boolean[] EMPTY_BOOLEAN_OBJ_ARRAY = new Boolean[0];
/**
* An empty immutable {@code Character} array.
*/
public static final Character[] EMPTY_CHAR_OBJ_ARRAY = new Character[0];
/**
* An empty immutable {@code Byte} array.
*/
public static final Byte[] EMPTY_BYTE_OBJ_ARRAY = new Byte[0];
/**
* An empty immutable {@code Short} array.
*/
public static final Short[] EMPTY_SHORT_OBJ_ARRAY = new Short[0];
/**
* An empty immutable {@code Integer} array.
*/
public static final Integer[] EMPTY_INT_OBJ_ARRAY = new Integer[0];
/**
* An empty immutable {@code Long} array.
*/
public static final Long[] EMPTY_LONG_OBJ_ARRAY = new Long[0];
/**
* An empty immutable {@code Float} array.
*/
public static final Float[] EMPTY_FLOAT_OBJ_ARRAY = new Float[0];
/**
* An empty immutable {@code Double} array.
*/
public static final Double[] EMPTY_DOUBLE_OBJ_ARRAY = new Double[0];
/**
* An empty immutable {@code String} array.
*/
public static final String[] EMPTY_STRING_ARRAY = new String[0];
/**
* An empty immutable {@code Object} array.
*/
public static final Object[] EMPTY_OBJECT_ARRAY = new Object[0];
/** The Constant EMPTY_LIST. */
@SuppressWarnings("rawtypes")
static final List EMPTY_LIST = Collections.emptyList();
/** The Constant EMPTY_SET. */
@SuppressWarnings("rawtypes")
static final Set EMPTY_SET = Collections.emptySet();
/** The Constant EMPTY_MAP. */
@SuppressWarnings("rawtypes")
static final Map EMPTY_MAP = Collections.emptyMap();
/** The Constant EMPTY_ITERATOR. */
@SuppressWarnings("rawtypes")
static final Iterator EMPTY_ITERATOR = Collections.emptyIterator();
/** The Constant EMPTY_LIST_ITERATOR. */
@SuppressWarnings("rawtypes")
static final ListIterator EMPTY_LIST_ITERATOR = Collections.emptyListIterator();
/** The Constant NULL_MASK. */
// ...
static final Object NULL_MASK = new NullMask();
/** The Constant BACKSLASH_ASTERISK. */
static final String BACKSLASH_ASTERISK = "*";
/** The Constant REVERSE_THRESHOLD. */
// ...
static final int REVERSE_THRESHOLD = 18;
/** The Constant FILL_THRESHOLD. */
static final int FILL_THRESHOLD = 25;
/** The Constant REPLACEALL_THRESHOLD. */
static final int REPLACEALL_THRESHOLD = 11;
/** The Constant RAND. */
// ...
static final Random RAND = new SecureRandom();
/** The Constant NULL_MIN_COMPARATOR. */
@SuppressWarnings("rawtypes")
static final Comparator NULL_MIN_COMPARATOR = Comparators.nullsFirst();
/** The Constant NULL_MAX_COMPARATOR. */
@SuppressWarnings("rawtypes")
static final Comparator NULL_MAX_COMPARATOR = Comparators.nullsLast();
/** The Constant NATURAL_ORDER. */
@SuppressWarnings("rawtypes")
static final Comparator NATURAL_ORDER = Comparators.naturalOrder();
/** The Constant CLASS_EMPTY_ARRAY. */
// ...
static final Map, Object> CLASS_EMPTY_ARRAY = new ConcurrentHashMap<>();
static {
CLASS_EMPTY_ARRAY.put(boolean.class, CommonUtil.EMPTY_BOOLEAN_ARRAY);
CLASS_EMPTY_ARRAY.put(Boolean.class, CommonUtil.EMPTY_BOOLEAN_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(char.class, CommonUtil.EMPTY_CHAR_ARRAY);
CLASS_EMPTY_ARRAY.put(Character.class, CommonUtil.EMPTY_CHARACTER_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(byte.class, CommonUtil.EMPTY_BYTE_ARRAY);
CLASS_EMPTY_ARRAY.put(Byte.class, CommonUtil.EMPTY_BYTE_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(short.class, CommonUtil.EMPTY_SHORT_ARRAY);
CLASS_EMPTY_ARRAY.put(Short.class, CommonUtil.EMPTY_SHORT_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(int.class, CommonUtil.EMPTY_INT_ARRAY);
CLASS_EMPTY_ARRAY.put(Integer.class, CommonUtil.EMPTY_INTEGER_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(long.class, CommonUtil.EMPTY_LONG_ARRAY);
CLASS_EMPTY_ARRAY.put(Long.class, CommonUtil.EMPTY_LONG_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(float.class, CommonUtil.EMPTY_FLOAT_ARRAY);
CLASS_EMPTY_ARRAY.put(Float.class, CommonUtil.EMPTY_FLOAT_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(double.class, CommonUtil.EMPTY_DOUBLE_ARRAY);
CLASS_EMPTY_ARRAY.put(Double.class, CommonUtil.EMPTY_DOUBLE_OBJECT_ARRAY);
CLASS_EMPTY_ARRAY.put(String.class, CommonUtil.EMPTY_STRING_ARRAY);
CLASS_EMPTY_ARRAY.put(Object.class, CommonUtil.EMPTY_OBJECT_ARRAY);
}
/** The Constant CLASS_TYPE_ENUM. */
// ...
static final Map, Integer> CLASS_TYPE_ENUM = new HashMap<>();
static {
CLASS_TYPE_ENUM.put(boolean.class, 1);
CLASS_TYPE_ENUM.put(char.class, 2);
CLASS_TYPE_ENUM.put(byte.class, 3);
CLASS_TYPE_ENUM.put(short.class, 4);
CLASS_TYPE_ENUM.put(int.class, 5);
CLASS_TYPE_ENUM.put(long.class, 6);
CLASS_TYPE_ENUM.put(float.class, 7);
CLASS_TYPE_ENUM.put(double.class, 8);
CLASS_TYPE_ENUM.put(String.class, 9);
CLASS_TYPE_ENUM.put(boolean[].class, 11);
CLASS_TYPE_ENUM.put(char[].class, 12);
CLASS_TYPE_ENUM.put(byte[].class, 13);
CLASS_TYPE_ENUM.put(short[].class, 14);
CLASS_TYPE_ENUM.put(int[].class, 15);
CLASS_TYPE_ENUM.put(long[].class, 16);
CLASS_TYPE_ENUM.put(float[].class, 17);
CLASS_TYPE_ENUM.put(double[].class, 18);
CLASS_TYPE_ENUM.put(String[].class, 19);
CLASS_TYPE_ENUM.put(Boolean.class, 21);
CLASS_TYPE_ENUM.put(Character.class, 22);
CLASS_TYPE_ENUM.put(Byte.class, 23);
CLASS_TYPE_ENUM.put(Short.class, 24);
CLASS_TYPE_ENUM.put(Integer.class, 25);
CLASS_TYPE_ENUM.put(Long.class, 26);
CLASS_TYPE_ENUM.put(Float.class, 27);
CLASS_TYPE_ENUM.put(Double.class, 28);
}
/** The Constant enumListPool. */
// ...
private static final Map>, ImmutableList extends Enum>>> enumListPool = new ObjectPool<>(POOL_SIZE);
/** The Constant enumSetPool. */
private static final Map>, ImmutableSet extends Enum>>> enumSetPool = new ObjectPool<>(POOL_SIZE);
/** The Constant enumMapPool. */
private static final Map>, ImmutableBiMap extends Enum>, String>> enumMapPool = new ObjectPool<>(POOL_SIZE);
/** The Constant nameTypePool. */
private static final Map> nameTypePool = new ObjectPool<>(POOL_SIZE);
/** The Constant clsTypePool. */
private static final Map, Type>> clsTypePool = new ObjectPool<>(POOL_SIZE);
/** The Constant listElementDataField. */
// ...
static final Field listElementDataField;
/** The Constant listSizeField. */
static final Field listSizeField;
/** The is list element data field gettable. */
static volatile boolean isListElementDataFieldGettable = true;
/** The is list element data field settable. */
static volatile boolean isListElementDataFieldSettable = true;
static {
Field tmp = null;
try {
tmp = String.class.getDeclaredField("offset");
} catch (Throwable e) {
// ignore.
}
if (tmp == null) {
try {
tmp = String.class.getDeclaredField("count");
} catch (Throwable e) {
// ignore.
}
}
if (tmp == null) {
try {
tmp = String.class.getDeclaredField("value");
} catch (Throwable e) {
// ignore.
}
}
tmp = null;
try {
tmp = ArrayList.class.getDeclaredField("elementData");
} catch (Throwable e) {
// ignore.
}
listElementDataField = tmp != null && tmp.getType().equals(Object[].class) ? tmp : null;
if (listElementDataField != null) {
ClassUtil.setAccessibleQuietly(listElementDataField, true);
}
tmp = null;
try {
tmp = ArrayList.class.getDeclaredField("size");
} catch (Throwable e) {
// ignore.
}
listSizeField = tmp != null && tmp.getType().equals(int.class) ? tmp : null;
if (listSizeField != null) {
ClassUtil.setAccessibleQuietly(listSizeField, true);
}
}
/** The Constant charStringCache. */
static final String[] charStringCache = new String[128];
/** The Constant intStringCacheLow. */
static final int intStringCacheLow = -1001;
/** The Constant intStringCacheHigh. */
static final int intStringCacheHigh = 10001;
/** The Constant intStringCache. */
static final String[] intStringCache = new String[intStringCacheHigh - intStringCacheLow];
/** The Constant stringIntCache. */
static final Map stringIntCache = new HashMap<>((int) (intStringCache.length * 1.5));
static {
for (int i = 0, j = intStringCacheLow, len = intStringCache.length; i < len; i++, j++) {
intStringCache[i] = Integer.valueOf(j).toString();
stringIntCache.put(intStringCache[i], j);
}
for (int i = 0; i < charStringCache.length; i++) {
charStringCache[i] = String.valueOf((char) i);
}
}
/** The Constant MIN_SIZE_FOR_COPY_ALL. */
private static final int MIN_SIZE_FOR_COPY_ALL = 9;
/**
* Constructor for.
*/
CommonUtil() {
// no instance();
}
/**
*
* @param
* @param typeName
* @return
*/
@SuppressWarnings("unchecked")
public static Type typeOf(final String typeName) {
N.checkArgNotNull(typeName, "typeName");
Type> type = nameTypePool.get(typeName);
if (type == null) {
type = TypeFactory.getType(typeName);
nameTypePool.put(typeName, type);
}
return (Type) type;
}
/**
*
* @param
* @param cls
* @return
*/
@SuppressWarnings("unchecked")
public static Type typeOf(final Class> cls) {
N.checkArgNotNull(cls, "cls");
Type> type = clsTypePool.get(cls);
if (type == null) {
type = TypeFactory.getType(cls);
clsTypePool.put(cls, type);
}
return (Type) type;
}
/**
*
* @param
* @param targetClass
* @param str
* @return
*/
@SuppressWarnings("unchecked")
public static T valueOf(final Class extends T> targetClass, final String str) {
return (str == null) ? defaultValueOf(targetClass) : (T) CommonUtil.typeOf(targetClass).valueOf(str);
}
/**
* Default value of.
*
* @param
* @param cls
* @return
*/
@SuppressWarnings("unchecked")
public static T defaultValueOf(final Class cls) {
return (T) CommonUtil.typeOf(cls).defaultValue();
}
/**
* Default if null.
*
* @param b
* @return true, if successful
*/
public static boolean defaultIfNull(Boolean b) {
if (b == null) {
return false;
}
return b.booleanValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return true, if successful
*/
public static boolean defaultIfNull(Boolean b, boolean defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.booleanValue();
}
/**
* Default if null.
*
* @param c
* @return
*/
public static char defaultIfNull(Character c) {
if (c == null) {
return CommonUtil.CHAR_0;
}
return c.charValue();
}
/**
* Default if null.
*
* @param c
* @param defaultForNull
* @return
*/
public static char defaultIfNull(Character c, char defaultForNull) {
if (c == null) {
return defaultForNull;
}
return c.charValue();
}
/**
* Default if null.
*
* @param b
* @return
*/
public static byte defaultIfNull(Byte b) {
if (b == null) {
return (byte) 0;
}
return b.byteValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return
*/
public static byte defaultIfNull(Byte b, byte defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.byteValue();
}
/**
* Default if null.
*
* @param b
* @return
*/
public static short defaultIfNull(Short b) {
if (b == null) {
return (short) 0;
}
return b.shortValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return
*/
public static short defaultIfNull(Short b, short defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.shortValue();
}
/**
* Default if null.
*
* @param b
* @return
*/
public static int defaultIfNull(Integer b) {
if (b == null) {
return 0;
}
return b.intValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return
*/
public static int defaultIfNull(Integer b, int defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.intValue();
}
/**
* Default if null.
*
* @param b
* @return
*/
public static long defaultIfNull(Long b) {
if (b == null) {
return 0;
}
return b.longValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return
*/
public static long defaultIfNull(Long b, long defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.longValue();
}
/**
* Default if null.
*
* @param b
* @return
*/
public static float defaultIfNull(Float b) {
if (b == null) {
return 0;
}
return b.floatValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return
*/
public static float defaultIfNull(Float b, float defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.floatValue();
}
/**
* Default if null.
*
* @param b
* @return
*/
public static double defaultIfNull(Double b) {
if (b == null) {
return 0;
}
return b.doubleValue();
}
/**
* Default if null.
*
* @param b
* @param defaultForNull
* @return
*/
public static double defaultIfNull(Double b, double defaultForNull) {
if (b == null) {
return defaultForNull;
}
return b.doubleValue();
}
/**
* Default if null.
*
* @param
* @param obj
* @param defaultForNull
* @return
*/
public static T defaultIfNull(final T obj, final T defaultForNull) {
return obj == null ? defaultForNull : obj;
}
/**
*
* @param val
* @return
*/
public static String stringOf(final boolean val) {
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final char val) {
if (val < 128) {
return charStringCache[val];
}
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final byte val) {
if (val > intStringCacheLow && val < intStringCacheHigh) {
return intStringCache[val - intStringCacheLow];
}
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final short val) {
if (val > intStringCacheLow && val < intStringCacheHigh) {
return intStringCache[val - intStringCacheLow];
}
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final int val) {
if (val > intStringCacheLow && val < intStringCacheHigh) {
return intStringCache[val - intStringCacheLow];
}
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final long val) {
if (val > intStringCacheLow && val < intStringCacheHigh) {
return intStringCache[(int) (val - intStringCacheLow)];
}
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final float val) {
return String.valueOf(val);
}
/**
*
* @param val
* @return
*/
public static String stringOf(final double val) {
return String.valueOf(val);
}
/**
*
* @param obj
* @return null
if the specified object is null.
*/
public static String stringOf(final Object obj) {
return (obj == null) ? null : CommonUtil.typeOf(obj.getClass()).stringOf(obj);
}
/**
* Enum list of.
*
* @param
* @param enumClass
* @return
*/
public static > ImmutableList enumListOf(final Class enumClass) {
ImmutableList enumList = (ImmutableList) enumListPool.get(enumClass);
if (enumList == null) {
enumList = ImmutableList.of(CommonUtil.asList(enumClass.getEnumConstants()));
enumListPool.put(enumClass, enumList);
}
return enumList;
}
/**
* Enum set of.
*
* @param
* @param enumClass
* @return
*/
public static > ImmutableSet enumSetOf(final Class enumClass) {
ImmutableSet enumSet = (ImmutableSet) enumSetPool.get(enumClass);
if (enumSet == null) {
enumSet = ImmutableSet.of(EnumSet.allOf(enumClass));
enumSetPool.put(enumClass, enumSet);
}
return enumSet;
}
/**
* Enum map of.
*
* @param
* @param enumClass
* @return
*/
public static > ImmutableBiMap enumMapOf(final Class enumClass) {
ImmutableBiMap enumMap = (ImmutableBiMap) enumMapPool.get(enumClass);
if (enumMap == null) {
final EnumMap keyMap = new EnumMap<>(enumClass);
final Map valueMap = new HashMap<>();
for (final E e : enumClass.getEnumConstants()) {
keyMap.put(e, e.name());
valueMap.put(e.name(), e);
}
enumMap = ImmutableBiMap.of(new BiMap<>(keyMap, valueMap));
enumMapPool.put(enumClass, enumMap);
}
return enumMap;
}
/**
*
* @param
* @param cls
* @return T
*/
public static T newInstance(final Class cls) {
if (Modifier.isAbstract(cls.getModifiers())) {
if (cls.equals(Map.class)) {
return (T) new HashMap<>();
} else if (cls.equals(List.class)) {
return (T) new ArrayList<>();
} else if (cls.equals(Set.class)) {
return (T) CommonUtil.newHashSet();
} else if (cls.equals(Queue.class)) {
return (T) new LinkedList<>();
} else if (cls.equals(Deque.class)) {
return (T) new LinkedList<>();
} else if (cls.equals(SortedSet.class) || cls.equals(NavigableSet.class)) {
return (T) new TreeSet<>();
} else if (cls.equals(SortedMap.class) || cls.equals(NavigableMap.class)) {
return (T) new TreeMap<>();
}
}
try {
if (Modifier.isStatic(cls.getModifiers()) == false && (cls.isAnonymousClass() || cls.isMemberClass())) {
// http://stackoverflow.com/questions/2097982/is-it-possible-to-create-an-instance-of-nested-class-using-java-reflection
final List> toInstantiate = new ArrayList<>();
Class> parent = cls.getEnclosingClass();
do {
toInstantiate.add(parent);
parent = parent.getEnclosingClass();
} while (parent != null && Modifier.isStatic(parent.getModifiers()) == false && (parent.isAnonymousClass() || parent.isMemberClass()));
if (parent != null) {
toInstantiate.add(parent);
}
CommonUtil.reverse(toInstantiate);
Object instance = null;
for (Class> current : toInstantiate) {
instance = instance == null ? invoke(ClassUtil.getDeclaredConstructor(current))
: invoke(ClassUtil.getDeclaredConstructor(current, instance.getClass()), instance);
}
return invoke(ClassUtil.getDeclaredConstructor(cls, instance.getClass()), instance);
} else {
final Constructor constructor = ClassUtil.getDeclaredConstructor(cls);
if (constructor == null) {
throw new IllegalArgumentException("No default constructor found in class: " + ClassUtil.getCanonicalClassName(cls));
}
return invoke(constructor);
}
} catch (InstantiationException | IllegalAccessException | IllegalArgumentException | InvocationTargetException e) {
throw N.toRuntimeException(e);
}
}
/**
*
* @param
* @param c
* @param args
* @return
* @throws InstantiationException the instantiation exception
* @throws IllegalAccessException the illegal access exception
* @throws IllegalArgumentException the illegal argument exception
* @throws InvocationTargetException the invocation target exception
*/
@SuppressWarnings("unchecked")
private static T invoke(final Constructor c, final Object... args)
throws InstantiationException, IllegalAccessException, IllegalArgumentException, InvocationTargetException {
if (c.isAccessible() == false) {
ClassUtil.setAccessible(c, true);
}
return c.newInstance(args);
}
/**
* New proxy instance.
*
* @param
* @param interfaceClass
* @param h
* @return
*/
public static T newProxyInstance(final Class interfaceClass, final InvocationHandler h) {
return newProxyInstance(CommonUtil.asArray(interfaceClass), h);
}
/**
* Refer to {@code java.lang.reflect}
*
* @param
* @param interfaceClasses
* @param h
* @return
*/
public static T newProxyInstance(final Class>[] interfaceClasses, final InvocationHandler h) {
return (T) Proxy.newProxyInstance(CommonUtil.class.getClassLoader(), interfaceClasses, h);
}
/**
*
* @param
* @param componentType
* @param length
* @return T[]
*/
@SuppressWarnings("unchecked")
public static T newArray(final Class> componentType, final int length) {
return (T) Array.newInstance(componentType, length);
}
/**
*
* @param
* @param cls
* @return
*/
@SuppressWarnings("unchecked")
public static T newEntity(final Class cls) {
return newEntity(cls, null);
}
/**
*
* @param
* @param cls
* @param entityName
* @return
*/
public static T newEntity(final Class cls, final String entityName) {
if (MapEntity.class.isAssignableFrom(cls)) {
return (T) new MapEntity(entityName);
}
final Class> enclosingClass = ClassUtil.getEnclosingClass(cls);
if (enclosingClass == null || Modifier.isStatic(cls.getModifiers())) {
return newInstance(cls);
} else {
return ClassUtil.invokeConstructor(ClassUtil.getDeclaredConstructor(cls, enclosingClass), newInstance(enclosingClass));
}
}
/**
* Returns a set backed by the specified map.
*
* @param
* @param map the backing map
* @return
* @see Collections#newSetFromMap(Map)
*/
public static Set newSetFromMap(final Map map) {
return Collections.newSetFromMap(map);
}
/**
* Inits the hash capacity.
*
* @param size
* @return
*/
public static int initHashCapacity(final int size) {
CommonUtil.checkArgNotNegative(size, "size");
if (size == 0) {
return 0;
}
int res = size < MAX_HASH_LENGTH ? (int) (size * 1.25) + 1 : MAX_ARRAY_SIZE;
switch (res / 64) {
case 0:
case 1:
return res;
case 2:
case 3:
case 4:
return 128;
default:
return 256;
}
}
/**
* New array list.
*
* @param
* @return
*/
public static ArrayList newArrayList() {
return new ArrayList<>();
}
/**
* New array list.
*
* @param
* @param initialCapacity
* @return
*/
public static ArrayList newArrayList(int initialCapacity) {
return new ArrayList<>(initialCapacity);
}
/**
* New array list.
*
* @param
* @param c
* @return
*/
public static ArrayList newArrayList(Collection extends T> c) {
return CommonUtil.isNullOrEmpty(c) ? new ArrayList() : new ArrayList<>(c);
}
/**
* New linked list.
*
* @param
* @return
*/
public static LinkedList newLinkedList() {
return new LinkedList<>();
}
/**
* New linked list.
*
* @param
* @param c
* @return
*/
public static LinkedList newLinkedList(Collection extends T> c) {
return CommonUtil.isNullOrEmpty(c) ? new LinkedList() : new LinkedList<>(c);
}
/**
* New hash set.
*
* @param
* @return
*/
public static Set newHashSet() {
return new HashSet<>();
}
/**
* New hash set.
*
* @param
* @param initialCapacity
* @return
*/
public static Set newHashSet(int initialCapacity) {
return new HashSet<>(initialCapacity);
}
/**
* New hash set.
*
* @param
* @param c
* @return
*/
public static Set newHashSet(Collection extends T> c) {
return CommonUtil.isNullOrEmpty(c) ? new HashSet() : new HashSet<>(c);
}
/**
* New linked hash set.
*
* @param
* @return
*/
public static Set newLinkedHashSet() {
return new LinkedHashSet<>();
}
/**
* New linked hash set.
*
* @param
* @param initialCapacity
* @return
*/
public static Set newLinkedHashSet(int initialCapacity) {
return new LinkedHashSet<>(initialCapacity);
}
/**
* New linked hash set.
*
* @param
* @param c
* @return
*/
public static Set newLinkedHashSet(Collection extends T> c) {
return CommonUtil.isNullOrEmpty(c) ? new LinkedHashSet() : new LinkedHashSet<>(c);
}
/**
* New tree set.
*
* @param
* @return
*/
public static > TreeSet newTreeSet() {
return new TreeSet<>();
}
/**
* New tree set.
*
* @param
* @param comparator
* @return
*/
public static TreeSet newTreeSet(Comparator super T> comparator) {
return new TreeSet<>(comparator);
}
/**
* New tree set.
*
* @param
* @param c
* @return
*/
public static > TreeSet newTreeSet(Collection extends T> c) {
return CommonUtil.isNullOrEmpty(c) ? new TreeSet() : new TreeSet<>(c);
}
/**
* New tree set.
*
* @param
* @param c
* @return
*/
public static TreeSet newTreeSet(SortedSet c) {
return CommonUtil.isNullOrEmpty(c) ? new TreeSet() : new TreeSet<>(c);
}
/**
*
* @param
* @return
*/
public static Multiset newMultiset() {
return new Multiset<>();
}
/**
*
* @param
* @param initialCapacity
* @return
*/
public static Multiset newMultiset(final int initialCapacity) {
return new Multiset<>(initialCapacity);
}
/**
*
* @param
* @param valueMapType
* @return
*/
@SuppressWarnings("rawtypes")
public static Multiset newMultiset(final Class extends Map> valueMapType) {
return new Multiset<>(valueMapType);
}
/**
*
* @param
* @param mapSupplier
* @return
*/
public static Multiset newMultiset(final Supplier extends Map> mapSupplier) {
return new Multiset<>(mapSupplier);
}
/**
*
* @param
* @param c
* @return
*/
public static Multiset newMultiset(final Collection extends T> c) {
return new Multiset<>(c);
}
/**
* New array deque.
*
* @param
* @return
*/
public static ArrayDeque newArrayDeque() {
return new ArrayDeque<>();
}
/**
* Constructs an empty array deque with an initial capacity sufficient to hold the specified number of elements.
*
* @param
* @param numElements lower bound on initial capacity of the deque.
* @return
*/
public static ArrayDeque newArrayDeque(int numElements) {
return new ArrayDeque<>(numElements);
}
/**
* Constructs a deque containing the elements of the specified collection, in the order they are returned by the collection's iterator.
*
* @param
* @param c
* @return
*/
public static ArrayDeque newArrayDeque(Collection extends E> c) {
return new ArrayDeque<>(c);
}
/**
*
* @param the key type
* @param the value type
* @param key
* @param value
* @return
*/
public static Map.Entry newEntry(K key, V value) {
return new AbstractMap.SimpleEntry<>(key, value);
}
/**
* New immutable entry.
*
* @param the key type
* @param the value type
* @param key
* @param value
* @return
*/
public static ImmutableEntry newImmutableEntry(K key, V value) {
return new ImmutableEntry<>(key, value);
}
/**
* New hash map.
*
* @param the key type
* @param the value type
* @return
*/
public static Map newHashMap() {
return new HashMap<>();
}
/**
* New hash map.
*
* @param the key type
* @param the value type
* @param initialCapacity
* @return
*/
public static Map newHashMap(int initialCapacity) {
return new HashMap<>(initialCapacity);
}
/**
* New hash map.
*
* @param the key type
* @param the value type
* @param m
* @return
*/
public static Map newHashMap(Map extends K, ? extends V> m) {
return CommonUtil.isNullOrEmpty(m) ? new HashMap() : new HashMap<>(m);
}
/**
* New hash map.
*
* @param the key type
* @param the value type
* @param
* @param c
* @param keyMapper
* @return
* @throws E the e
*/
public static Map newHashMap(final Collection extends V> c, final Throwables.Function super V, ? extends K, E> keyMapper)
throws E {
CommonUtil.checkArgNotNull(keyMapper);
if (isNullOrEmpty(c)) {
return new HashMap<>();
}
final HashMap result = new HashMap<>(CommonUtil.initHashCapacity(c.size()));
for (V v : c) {
result.put(keyMapper.apply(v), v);
}
return result;
}
/**
* New linked hash map.
*
* @param the key type
* @param the value type
* @return
*/
public static Map newLinkedHashMap() {
return new LinkedHashMap<>();
}
/**
* New linked hash map.
*
* @param the key type
* @param the value type
* @param initialCapacity
* @return
*/
public static Map newLinkedHashMap(int initialCapacity) {
return new LinkedHashMap<>(initialCapacity);
}
/**
* New linked hash map.
*
* @param the key type
* @param the value type
* @param m
* @return
*/
public static Map newLinkedHashMap(Map extends K, ? extends V> m) {
return CommonUtil.isNullOrEmpty(m) ? new LinkedHashMap() : new LinkedHashMap<>(m);
}
/**
* New linked hash map.
*
* @param the key type
* @param the value type
* @param
* @param c
* @param keyMapper
* @return
* @throws E the e
*/
public static Map newLinkedHashMap(final Collection extends V> c,
final Throwables.Function super V, ? extends K, E> keyMapper) throws E {
CommonUtil.checkArgNotNull(keyMapper);
if (isNullOrEmpty(c)) {
return new LinkedHashMap<>();
}
final Map result = new LinkedHashMap<>(CommonUtil.initHashCapacity(c.size()));
for (V v : c) {
result.put(keyMapper.apply(v), v);
}
return result;
}
/**
* New tree map.
*
* @param the key type
* @param the value type
* @return
*/
public static , V> TreeMap newTreeMap() {
return new TreeMap<>();
}
/**
* New tree map.
*
* @param
* @param the key type
* @param the value type
* @param comparator
* @return
*/
public static TreeMap newTreeMap(Comparator comparator) {
return new TreeMap<>(comparator);
}
/**
* New tree map.
*
* @param the key type
* @param the value type
* @param m
* @return
*/
public static , V> TreeMap newTreeMap(Map extends K, ? extends V> m) {
return CommonUtil.isNullOrEmpty(m) ? new TreeMap() : new TreeMap<>(m);
}
/**
* New tree map.
*
* @param the key type
* @param the value type
* @param m
* @return
*/
public static TreeMap newTreeMap(SortedMap m) {
return CommonUtil.isNullOrEmpty(m) ? new TreeMap() : new TreeMap<>(m);
}
/**
* New identity hash map.
*
* @param the key type
* @param the value type
* @return
*/
public static IdentityHashMap newIdentityHashMap() {
return new IdentityHashMap<>();
}
/**
* New identity hash map.
*
* @param the key type
* @param the value type
* @param initialCapacity
* @return
*/
public static IdentityHashMap newIdentityHashMap(int initialCapacity) {
return new IdentityHashMap<>(initialCapacity);
}
/**
* New identity hash map.
*
* @param the key type
* @param the value type
* @param m
* @return
*/
public static IdentityHashMap newIdentityHashMap(Map extends K, ? extends V> m) {
return CommonUtil.isNullOrEmpty(m) ? new IdentityHashMap() : new IdentityHashMap<>(m);
}
/**
* New concurrent hash map.
*
* @param the key type
* @param the value type
* @return
*/
public static ConcurrentHashMap newConcurrentHashMap() {
return new ConcurrentHashMap<>();
}
/**
* New concurrent hash map.
*
* @param the key type
* @param the value type
* @param initialCapacity
* @return
*/
public static ConcurrentHashMap newConcurrentHashMap(int initialCapacity) {
return new ConcurrentHashMap<>(initialCapacity);
}
/**
* New concurrent hash map.
*
* @param the key type
* @param the value type
* @param m
* @return
*/
public static ConcurrentHashMap newConcurrentHashMap(Map extends K, ? extends V> m) {
return CommonUtil.isNullOrEmpty(m) ? new ConcurrentHashMap() : new ConcurrentHashMap<>(m);
}
/**
* New bi map.
*
* @param the key type
* @param the value type
* @return
*/
public static BiMap newBiMap() {
return new BiMap<>();
}
/**
* New bi map.
*
* @param the key type
* @param the value type
* @param initialCapacity
* @return
*/
public static BiMap newBiMap(int initialCapacity) {
return new BiMap<>(initialCapacity);
}
/**
* New bi map.
*
* @param the key type
* @param the value type
* @param initialCapacity
* @param loadFactor
* @return
*/
public static BiMap newBiMap(int initialCapacity, float loadFactor) {
return new BiMap<>(initialCapacity, loadFactor);
}
/**
* New bi map.
*
* @param