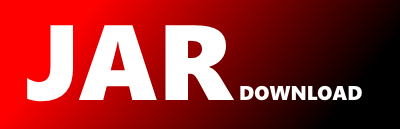
com.landawn.abacus.parser.DeserializationConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of abacus-util-se Show documentation
Show all versions of abacus-util-se Show documentation
A general programming library in Java/Android. It's easy to learn and simple to use with concise and powerful APIs.
/*
* Copyright (C) 2015 HaiYang Li
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package com.landawn.abacus.parser;
import java.util.HashMap;
import java.util.Map;
import com.landawn.abacus.type.Type;
import com.landawn.abacus.util.N;
/**
* The Class DeserializationConfig.
*
* @author Haiyang Li
* @param
* @since 0.8
*/
public abstract class DeserializationConfig> extends ParserConfig {
/** The ignore unknown property. */
boolean ignoreUnknownProperty = true;
/** The element type. */
Type> elementType;
/** The key type. */
Type> keyType;
/** The value type. */
Type> valueType;
/** The prop types. */
Map> propTypes;
/**
* Checks if is ignore unknown property.
*
* @return true, if is ignore unknown property
*/
public boolean isIgnoreUnknownProperty() {
return ignoreUnknownProperty;
}
/**
* Sets the ignore unknown property.
*
* @param ignoreUnknownProperty
* @return
*/
public C setIgnoreUnknownProperty(boolean ignoreUnknownProperty) {
this.ignoreUnknownProperty = ignoreUnknownProperty;
return (C) this;
}
/**
* Gets the element type.
*
* @param
* @return
*/
public Type getElementType() {
return (Type) elementType;
}
/**
* Sets the element type.
*
* @param cls
* @return
*/
public C setElementType(Class> cls) {
return setElementType(N.typeOf(cls));
}
/**
* Sets the element type.
*
* @param type
* @return
*/
public C setElementType(Type> type) {
this.elementType = type;
return (C) this;
}
/**
* Sets the element type.
*
* @param type
* @return
*/
public C setElementType(String type) {
return setElementType(N.typeOf(type));
}
/**
* Gets the map key type.
*
* @param
* @return
*/
public Type getMapKeyType() {
return (Type) keyType;
}
/**
* Sets the map key type.
*
* @param cls
* @return
*/
public C setMapKeyType(Class> cls) {
return this.setMapKeyType(N.typeOf(cls));
}
/**
* Sets the map key type.
*
* @param keyType
* @return
*/
public C setMapKeyType(Type> keyType) {
this.keyType = keyType;
return (C) this;
}
/**
* Sets the map key type.
*
* @param keyType
* @return
*/
public C setMapKeyType(String keyType) {
return this.setMapKeyType(N.typeOf(keyType));
}
/**
* Gets the map value type.
*
* @param
* @return
*/
public Type getMapValueType() {
return (Type) valueType;
}
/**
* Sets the map value type.
*
* @param cls
* @return
*/
public C setMapValueType(Class> cls) {
return this.setMapValueType(N.typeOf(cls));
}
/**
* Sets the map value type.
*
* @param valueType
* @return
*/
public C setMapValueType(Type> valueType) {
this.valueType = valueType;
return (C) this;
}
/**
* Sets the map value type.
*
* @param valueType
* @return
*/
public C setMapValueType(String valueType) {
return this.setMapValueType(N.typeOf(valueType));
}
/**
* Gets the prop types.
*
* @return
*/
public Map> getPropTypes() {
return propTypes;
}
/**
* Sets the prop types.
*
* @param propTypes
* @return
*/
public C setPropTypes(Map> propTypes) {
this.propTypes = propTypes;
return (C) this;
}
/**
* Gets the prop type.
*
* @param
* @param propName
* @return
*/
public Type getPropType(String propName) {
return propTypes == null ? null : (Type) propTypes.get(propName);
}
/**
* Sets the prop type.
*
* @param propName
* @param cls
* @return
*/
public C setPropType(String propName, Class> cls) {
return setPropType(propName, N.typeOf(cls));
}
/**
* Sets the prop type.
*
* @param propName
* @param type
* @return
*/
public C setPropType(String propName, Type> type) {
if (propTypes == null) {
propTypes = new HashMap<>();
}
this.propTypes.put(propName, type);
return (C) this;
}
/**
* Sets the prop type.
*
* @param propName
* @param type
* @return
*/
public C setPropType(String propName, String type) {
return setPropType(propName, N.typeOf(type));
}
/**
* Checks for prop type.
*
* @param propName
* @return true, if successful
*/
public boolean hasPropType(String propName) {
return propTypes != null && propTypes.containsKey(propName);
}
/**
*
* @return
*/
@Override
public int hashCode() {
int h = 17;
h = 31 * h + N.hashCode(getIgnoredPropNames());
h = 31 * h + N.hashCode(ignoreUnknownProperty);
h = 31 * h + N.hashCode(elementType);
h = 31 * h + N.hashCode(keyType);
h = 31 * h + N.hashCode(valueType);
h = 31 * h + N.hashCode(propTypes);
return h;
}
/**
*
* @param obj
* @return true, if successful
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj instanceof DeserializationConfig) {
final DeserializationConfig other = (DeserializationConfig) obj;
if (N.equals(getIgnoredPropNames(), other.getIgnoredPropNames()) && N.equals(ignoreUnknownProperty, other.ignoreUnknownProperty)
&& N.equals(elementType, other.elementType) && N.equals(keyType, other.keyType) && N.equals(valueType, other.valueType)
&& N.equals(propTypes, other.propTypes)) {
return true;
}
}
return false;
}
/**
*
* @return
*/
@Override
public String toString() {
return "{ignoredPropNames=" + N.toString(getIgnoredPropNames()) + ", ignoreUnknownProperty=" + N.toString(ignoreUnknownProperty) + ", elementType="
+ N.toString(elementType) + ", keyType=" + N.toString(keyType) + ", valueType=" + N.toString(valueType) + ", propTypes=" + N.toString(propTypes)
+ "}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy