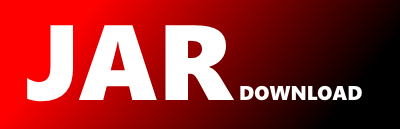
com.larksuite.oapi.core.api.BatchReqCall Maven / Gradle / Ivy
package com.larksuite.oapi.core.api;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.concurrent.*;
import java.util.concurrent.atomic.AtomicInteger;
public class BatchReqCall {
private static final ExecutorService defaultExecutorService = Executors.newCachedThreadPool(new ThreadFactory() {
protected final AtomicInteger mThreadNum = new AtomicInteger(1);
@Override
public Thread newThread(Runnable runnable) {
String mPrefix = "larksuite-oapi-thread-";
String name = mPrefix + mThreadNum.getAndIncrement();
return new Thread(null, runnable, name, 0);
}
});
private final ExecutorService executorService;
private final List> reqCallers;
@SafeVarargs
public BatchReqCall(ReqCaller... reqCallers) {
this(defaultExecutorService, reqCallers);
}
@SafeVarargs
public BatchReqCall(ExecutorService executorService, ReqCaller... reqCallers) {
this.executorService = executorService;
this.reqCallers = new ArrayList<>();
int i = 0;
for (ReqCaller reqCaller : reqCallers) {
reqCaller.setIdx$$(i);
this.reqCallers.add(reqCaller);
i++;
}
}
public List> call() throws InterruptedException, ExecutionException {
List> reqCallResults = new ArrayList<>();
if (this.reqCallers.size() == 0) {
return reqCallResults;
}
List>> futures = executorService.invokeAll(this.reqCallers);
for (Future> future : futures) {
ReqCallResult reqCallResult = new ReqCallResult<>(future.get());
reqCallResults.add(reqCallResult);
}
reqCallResults.sort(Comparator.comparingInt(o -> o.getReqCaller().getIdx$$()));
return reqCallResults;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy