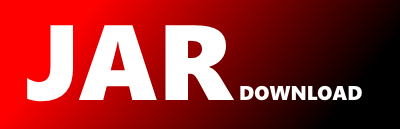
com.lark.oapi.service.attendance.v1.model.QueryUserFlowReq Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.attendance.v1.model;
import com.lark.oapi.core.response.EmptyData;
import com.lark.oapi.service.attendance.v1.enums.*;
import com.google.gson.annotations.SerializedName;
import com.lark.oapi.core.annotation.Body;
import com.lark.oapi.core.annotation.Path;
import com.lark.oapi.core.annotation.Query;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lark.oapi.core.utils.Strings;
import com.lark.oapi.core.response.BaseResponse;
public class QueryUserFlowReq {
/**
* 请求体中的 user_ids 和响应体中的 user_id 的员工工号类型
* 示例值:employee_id
*/
@Query
@SerializedName("employee_type")
private String employeeType;
/**
* 由于新入职用户可以复用已离职用户的employee_no/employee_id。如果true,返回employee_no/employee_id对应的所有在职+离职用户数据;如果false,只返回employee_no/employee_id对应的在职或最近一个离职用户数据
*
示例值:true
*/
@Query
@SerializedName("include_terminated_user")
private Boolean includeTerminatedUser;
@Body
private QueryUserFlowReqBody body;
// builder 开始
public QueryUserFlowReq() {
}
public QueryUserFlowReq(Builder builder) {
/**
* 请求体中的 user_ids 和响应体中的 user_id 的员工工号类型
*
示例值:employee_id
*/
this.employeeType = builder.employeeType;
/**
* 由于新入职用户可以复用已离职用户的employee_no/employee_id。如果true,返回employee_no/employee_id对应的所有在职+离职用户数据;如果false,只返回employee_no/employee_id对应的在职或最近一个离职用户数据
*
示例值:true
*/
this.includeTerminatedUser = builder.includeTerminatedUser;
this.body = builder.body;
}
public static Builder newBuilder() {
return new Builder();
}
public String getEmployeeType() {
return this.employeeType;
}
public void setEmployeeType(String employeeType) {
this.employeeType = employeeType;
}
public Boolean getIncludeTerminatedUser() {
return this.includeTerminatedUser;
}
public void setIncludeTerminatedUser(Boolean includeTerminatedUser) {
this.includeTerminatedUser = includeTerminatedUser;
}
public QueryUserFlowReqBody getQueryUserFlowReqBody() {
return this.body;
}
public void setQueryUserFlowReqBody(QueryUserFlowReqBody body) {
this.body = body;
}
public static class Builder {
private String employeeType; // 请求体中的 user_ids 和响应体中的 user_id 的员工工号类型
private Boolean includeTerminatedUser; // 由于新入职用户可以复用已离职用户的employee_no/employee_id。如果true,返回employee_no/employee_id对应的所有在职+离职用户数据;如果false,只返回employee_no/employee_id对应的在职或最近一个离职用户数据
private QueryUserFlowReqBody body;
/**
* 请求体中的 user_ids 和响应体中的 user_id 的员工工号类型
*
示例值:employee_id
*
* @param employeeType
* @return
*/
public Builder employeeType(String employeeType) {
this.employeeType = employeeType;
return this;
}
/**
* 请求体中的 user_ids 和响应体中的 user_id 的员工工号类型
*
示例值:employee_id
*
* @param employeeType {@link com.lark.oapi.service.attendance.v1.enums.QueryUserFlowEmployeeTypeEnum}
* @return
*/
public Builder employeeType(com.lark.oapi.service.attendance.v1.enums.QueryUserFlowEmployeeTypeEnum employeeType) {
this.employeeType = employeeType.getValue();
return this;
}
/**
* 由于新入职用户可以复用已离职用户的employee_no/employee_id。如果true,返回employee_no/employee_id对应的所有在职+离职用户数据;如果false,只返回employee_no/employee_id对应的在职或最近一个离职用户数据
*
示例值:true
*
* @param includeTerminatedUser
* @return
*/
public Builder includeTerminatedUser(Boolean includeTerminatedUser) {
this.includeTerminatedUser = includeTerminatedUser;
return this;
}
public QueryUserFlowReqBody getQueryUserFlowReqBody() {
return this.body;
}
/**
* body
*
* @param body
* @return
*/
public Builder queryUserFlowReqBody(QueryUserFlowReqBody body) {
this.body = body;
return this;
}
public QueryUserFlowReq build() {
return new QueryUserFlowReq(this);
}
}
}