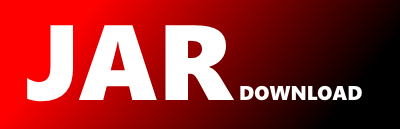
com.lark.oapi.service.bitable.v1.model.AppTableFieldProperty Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.bitable.v1.model;
import com.lark.oapi.core.response.EmptyData;
import com.lark.oapi.service.bitable.v1.enums.*;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.SerializedName;
import com.lark.oapi.core.annotation.Body;
import com.lark.oapi.core.annotation.Path;
import com.lark.oapi.core.annotation.Query;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lark.oapi.core.utils.Strings;
import com.lark.oapi.core.response.BaseResponse;
public class AppTableFieldProperty {
/**
* 单选、多选字段的选项信息
* 示例值:
*/
@SerializedName("options")
private AppTableFieldPropertyOption[] options;
/**
* 数字、公式字段的显示格式
*
示例值:0
*/
@SerializedName("formatter")
private String formatter;
/**
* 日期、创建时间、最后更新时间字段的显示格式
*
示例值:日期格式
*/
@SerializedName("date_formatter")
private String dateFormatter;
/**
* 日期字段中新纪录自动填写创建时间
*
示例值:false
*/
@SerializedName("auto_fill")
private Boolean autoFill;
/**
* 人员字段中允许添加多个成员,单向关联、双向关联中允许添加多个记录
*
示例值:false
*/
@SerializedName("multiple")
private Boolean multiple;
/**
* 单向关联、双向关联字段中关联的数据表的id
*
示例值:tblsRc9GRRXKqhvW
*/
@SerializedName("table_id")
private String tableId;
/**
* 单向关联、双向关联字段中关联的数据表的名字
*
示例值:"table2"
*/
@SerializedName("table_name")
private String tableName;
/**
* 双向关联字段中关联的数据表中对应的双向关联字段的名字
*
示例值:"table1-双向关联"
*/
@SerializedName("back_field_name")
private String backFieldName;
/**
* 自动编号类型
*
示例值:
*/
@SerializedName("auto_serial")
private AppFieldPropertyAutoSerial autoSerial;
/**
* 地理位置输入方式
*
示例值:
*/
@SerializedName("location")
private AppFieldPropertyLocation location;
/**
* 公式字段的表达式
*
示例值:bitable::$table[tblNj92WQBAasdEf].$field[fldMV60rYs]*2
*/
@SerializedName("formula_expression")
private String formulaExpression;
/**
* 字段支持的编辑模式
*
示例值:
*/
@SerializedName("allowed_edit_modes")
private AllowedEditModes allowedEditModes;
/**
* 进度、评分等字段的数据范围最小值
*
示例值:0
*/
@SerializedName("min")
private Double min;
/**
* 进度、评分等字段的数据范围最大值
*
示例值:10
*/
@SerializedName("max")
private Double max;
/**
* 进度等字段是否支持自定义范围
*
示例值:true
*/
@SerializedName("range_customize")
private Boolean rangeCustomize;
/**
* 货币币种
*
示例值:CNY
*/
@SerializedName("currency_code")
private String currencyCode;
/**
* 评分字段的相关设置
*
示例值:
*/
@SerializedName("rating")
private Rating rating;
// builder 开始
public AppTableFieldProperty() {
}
public AppTableFieldProperty(Builder builder) {
/**
* 单选、多选字段的选项信息
*
示例值:
*/
this.options = builder.options;
/**
* 数字、公式字段的显示格式
*
示例值:0
*/
this.formatter = builder.formatter;
/**
* 日期、创建时间、最后更新时间字段的显示格式
*
示例值:日期格式
*/
this.dateFormatter = builder.dateFormatter;
/**
* 日期字段中新纪录自动填写创建时间
*
示例值:false
*/
this.autoFill = builder.autoFill;
/**
* 人员字段中允许添加多个成员,单向关联、双向关联中允许添加多个记录
*
示例值:false
*/
this.multiple = builder.multiple;
/**
* 单向关联、双向关联字段中关联的数据表的id
*
示例值:tblsRc9GRRXKqhvW
*/
this.tableId = builder.tableId;
/**
* 单向关联、双向关联字段中关联的数据表的名字
*
示例值:"table2"
*/
this.tableName = builder.tableName;
/**
* 双向关联字段中关联的数据表中对应的双向关联字段的名字
*
示例值:"table1-双向关联"
*/
this.backFieldName = builder.backFieldName;
/**
* 自动编号类型
*
示例值:
*/
this.autoSerial = builder.autoSerial;
/**
* 地理位置输入方式
*
示例值:
*/
this.location = builder.location;
/**
* 公式字段的表达式
*
示例值:bitable::$table[tblNj92WQBAasdEf].$field[fldMV60rYs]*2
*/
this.formulaExpression = builder.formulaExpression;
/**
* 字段支持的编辑模式
*
示例值:
*/
this.allowedEditModes = builder.allowedEditModes;
/**
* 进度、评分等字段的数据范围最小值
*
示例值:0
*/
this.min = builder.min;
/**
* 进度、评分等字段的数据范围最大值
*
示例值:10
*/
this.max = builder.max;
/**
* 进度等字段是否支持自定义范围
*
示例值:true
*/
this.rangeCustomize = builder.rangeCustomize;
/**
* 货币币种
*
示例值:CNY
*/
this.currencyCode = builder.currencyCode;
/**
* 评分字段的相关设置
*
示例值:
*/
this.rating = builder.rating;
}
public static Builder newBuilder() {
return new Builder();
}
public AppTableFieldPropertyOption[] getOptions() {
return this.options;
}
public void setOptions(AppTableFieldPropertyOption[] options) {
this.options = options;
}
public String getFormatter() {
return this.formatter;
}
public void setFormatter(String formatter) {
this.formatter = formatter;
}
public String getDateFormatter() {
return this.dateFormatter;
}
public void setDateFormatter(String dateFormatter) {
this.dateFormatter = dateFormatter;
}
public Boolean getAutoFill() {
return this.autoFill;
}
public void setAutoFill(Boolean autoFill) {
this.autoFill = autoFill;
}
public Boolean getMultiple() {
return this.multiple;
}
public void setMultiple(Boolean multiple) {
this.multiple = multiple;
}
public String getTableId() {
return this.tableId;
}
public void setTableId(String tableId) {
this.tableId = tableId;
}
public String getTableName() {
return this.tableName;
}
public void setTableName(String tableName) {
this.tableName = tableName;
}
public String getBackFieldName() {
return this.backFieldName;
}
public void setBackFieldName(String backFieldName) {
this.backFieldName = backFieldName;
}
public AppFieldPropertyAutoSerial getAutoSerial() {
return this.autoSerial;
}
public void setAutoSerial(AppFieldPropertyAutoSerial autoSerial) {
this.autoSerial = autoSerial;
}
public AppFieldPropertyLocation getLocation() {
return this.location;
}
public void setLocation(AppFieldPropertyLocation location) {
this.location = location;
}
public String getFormulaExpression() {
return this.formulaExpression;
}
public void setFormulaExpression(String formulaExpression) {
this.formulaExpression = formulaExpression;
}
public AllowedEditModes getAllowedEditModes() {
return this.allowedEditModes;
}
public void setAllowedEditModes(AllowedEditModes allowedEditModes) {
this.allowedEditModes = allowedEditModes;
}
public Double getMin() {
return this.min;
}
public void setMin(Double min) {
this.min = min;
}
public Double getMax() {
return this.max;
}
public void setMax(Double max) {
this.max = max;
}
public Boolean getRangeCustomize() {
return this.rangeCustomize;
}
public void setRangeCustomize(Boolean rangeCustomize) {
this.rangeCustomize = rangeCustomize;
}
public String getCurrencyCode() {
return this.currencyCode;
}
public void setCurrencyCode(String currencyCode) {
this.currencyCode = currencyCode;
}
public Rating getRating() {
return this.rating;
}
public void setRating(Rating rating) {
this.rating = rating;
}
public static class Builder {
/**
* 单选、多选字段的选项信息
*
示例值:
*/
private AppTableFieldPropertyOption[] options;
/**
* 数字、公式字段的显示格式
*
示例值:0
*/
private String formatter;
/**
* 日期、创建时间、最后更新时间字段的显示格式
*
示例值:日期格式
*/
private String dateFormatter;
/**
* 日期字段中新纪录自动填写创建时间
*
示例值:false
*/
private Boolean autoFill;
/**
* 人员字段中允许添加多个成员,单向关联、双向关联中允许添加多个记录
*
示例值:false
*/
private Boolean multiple;
/**
* 单向关联、双向关联字段中关联的数据表的id
*
示例值:tblsRc9GRRXKqhvW
*/
private String tableId;
/**
* 单向关联、双向关联字段中关联的数据表的名字
*
示例值:"table2"
*/
private String tableName;
/**
* 双向关联字段中关联的数据表中对应的双向关联字段的名字
*
示例值:"table1-双向关联"
*/
private String backFieldName;
/**
* 自动编号类型
*
示例值:
*/
private AppFieldPropertyAutoSerial autoSerial;
/**
* 地理位置输入方式
*
示例值:
*/
private AppFieldPropertyLocation location;
/**
* 公式字段的表达式
*
示例值:bitable::$table[tblNj92WQBAasdEf].$field[fldMV60rYs]*2
*/
private String formulaExpression;
/**
* 字段支持的编辑模式
*
示例值:
*/
private AllowedEditModes allowedEditModes;
/**
* 进度、评分等字段的数据范围最小值
*
示例值:0
*/
private Double min;
/**
* 进度、评分等字段的数据范围最大值
*
示例值:10
*/
private Double max;
/**
* 进度等字段是否支持自定义范围
*
示例值:true
*/
private Boolean rangeCustomize;
/**
* 货币币种
*
示例值:CNY
*/
private String currencyCode;
/**
* 评分字段的相关设置
*
示例值:
*/
private Rating rating;
/**
* 单选、多选字段的选项信息
*
示例值:
*
* @param options
* @return
*/
public Builder options(AppTableFieldPropertyOption[] options) {
this.options = options;
return this;
}
/**
* 数字、公式字段的显示格式
*
示例值:0
*
* @param formatter
* @return
*/
public Builder formatter(String formatter) {
this.formatter = formatter;
return this;
}
/**
* 日期、创建时间、最后更新时间字段的显示格式
*
示例值:日期格式
*
* @param dateFormatter
* @return
*/
public Builder dateFormatter(String dateFormatter) {
this.dateFormatter = dateFormatter;
return this;
}
/**
* 日期字段中新纪录自动填写创建时间
*
示例值:false
*
* @param autoFill
* @return
*/
public Builder autoFill(Boolean autoFill) {
this.autoFill = autoFill;
return this;
}
/**
* 人员字段中允许添加多个成员,单向关联、双向关联中允许添加多个记录
*
示例值:false
*
* @param multiple
* @return
*/
public Builder multiple(Boolean multiple) {
this.multiple = multiple;
return this;
}
/**
* 单向关联、双向关联字段中关联的数据表的id
*
示例值:tblsRc9GRRXKqhvW
*
* @param tableId
* @return
*/
public Builder tableId(String tableId) {
this.tableId = tableId;
return this;
}
/**
* 单向关联、双向关联字段中关联的数据表的名字
*
示例值:"table2"
*
* @param tableName
* @return
*/
public Builder tableName(String tableName) {
this.tableName = tableName;
return this;
}
/**
* 双向关联字段中关联的数据表中对应的双向关联字段的名字
*
示例值:"table1-双向关联"
*
* @param backFieldName
* @return
*/
public Builder backFieldName(String backFieldName) {
this.backFieldName = backFieldName;
return this;
}
/**
* 自动编号类型
*
示例值:
*
* @param autoSerial
* @return
*/
public Builder autoSerial(AppFieldPropertyAutoSerial autoSerial) {
this.autoSerial = autoSerial;
return this;
}
/**
* 地理位置输入方式
*
示例值:
*
* @param location
* @return
*/
public Builder location(AppFieldPropertyLocation location) {
this.location = location;
return this;
}
/**
* 公式字段的表达式
*
示例值:bitable::$table[tblNj92WQBAasdEf].$field[fldMV60rYs]*2
*
* @param formulaExpression
* @return
*/
public Builder formulaExpression(String formulaExpression) {
this.formulaExpression = formulaExpression;
return this;
}
/**
* 字段支持的编辑模式
*
示例值:
*
* @param allowedEditModes
* @return
*/
public Builder allowedEditModes(AllowedEditModes allowedEditModes) {
this.allowedEditModes = allowedEditModes;
return this;
}
/**
* 进度、评分等字段的数据范围最小值
*
示例值:0
*
* @param min
* @return
*/
public Builder min(Double min) {
this.min = min;
return this;
}
/**
* 进度、评分等字段的数据范围最大值
*
示例值:10
*
* @param max
* @return
*/
public Builder max(Double max) {
this.max = max;
return this;
}
/**
* 进度等字段是否支持自定义范围
*
示例值:true
*
* @param rangeCustomize
* @return
*/
public Builder rangeCustomize(Boolean rangeCustomize) {
this.rangeCustomize = rangeCustomize;
return this;
}
/**
* 货币币种
*
示例值:CNY
*
* @param currencyCode
* @return
*/
public Builder currencyCode(String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/**
* 评分字段的相关设置
*
示例值:
*
* @param rating
* @return
*/
public Builder rating(Rating rating) {
this.rating = rating;
return this;
}
public AppTableFieldProperty build() {
return new AppTableFieldProperty(this);
}
}
}