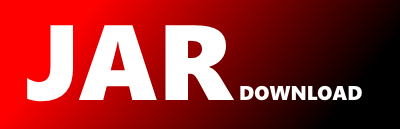
com.lark.oapi.service.bitable.v1.model.AppTableFormPatchedField Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.bitable.v1.model;
import com.lark.oapi.core.response.EmptyData;
import com.lark.oapi.service.bitable.v1.enums.*;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.SerializedName;
import com.lark.oapi.core.annotation.Body;
import com.lark.oapi.core.annotation.Path;
import com.lark.oapi.core.annotation.Query;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lark.oapi.core.utils.Strings;
import com.lark.oapi.core.response.BaseResponse;
public class AppTableFormPatchedField {
/**
* 上一个表单问题 ID,用于支持调整表单问题的顺序,通过前一个表单问题的 field_id 来确定位置;如果 pre_field_id 为空字符串,则说明要排到首个表单问题
* 示例值:fldjX7dUj5
*/
@SerializedName("pre_field_id")
private String preFieldId;
/**
* 表单问题
*
示例值:多行文本
*/
@SerializedName("title")
private String title;
/**
* 问题描述
*
示例值:多行文本描述
*/
@SerializedName("description")
private String description;
/**
* 是否必填
*
示例值:true
*/
@SerializedName("required")
private Boolean required;
/**
* 是否可见,当值为 false 时,不允许更新其他字段。
*
示例值:true
*/
@SerializedName("visible")
private Boolean visible;
// builder 开始
public AppTableFormPatchedField() {
}
public AppTableFormPatchedField(Builder builder) {
/**
* 上一个表单问题 ID,用于支持调整表单问题的顺序,通过前一个表单问题的 field_id 来确定位置;如果 pre_field_id 为空字符串,则说明要排到首个表单问题
*
示例值:fldjX7dUj5
*/
this.preFieldId = builder.preFieldId;
/**
* 表单问题
*
示例值:多行文本
*/
this.title = builder.title;
/**
* 问题描述
*
示例值:多行文本描述
*/
this.description = builder.description;
/**
* 是否必填
*
示例值:true
*/
this.required = builder.required;
/**
* 是否可见,当值为 false 时,不允许更新其他字段。
*
示例值:true
*/
this.visible = builder.visible;
}
public static Builder newBuilder() {
return new Builder();
}
public String getPreFieldId() {
return this.preFieldId;
}
public void setPreFieldId(String preFieldId) {
this.preFieldId = preFieldId;
}
public String getTitle() {
return this.title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDescription() {
return this.description;
}
public void setDescription(String description) {
this.description = description;
}
public Boolean getRequired() {
return this.required;
}
public void setRequired(Boolean required) {
this.required = required;
}
public Boolean getVisible() {
return this.visible;
}
public void setVisible(Boolean visible) {
this.visible = visible;
}
public static class Builder {
/**
* 上一个表单问题 ID,用于支持调整表单问题的顺序,通过前一个表单问题的 field_id 来确定位置;如果 pre_field_id 为空字符串,则说明要排到首个表单问题
*
示例值:fldjX7dUj5
*/
private String preFieldId;
/**
* 表单问题
*
示例值:多行文本
*/
private String title;
/**
* 问题描述
*
示例值:多行文本描述
*/
private String description;
/**
* 是否必填
*
示例值:true
*/
private Boolean required;
/**
* 是否可见,当值为 false 时,不允许更新其他字段。
*
示例值:true
*/
private Boolean visible;
/**
* 上一个表单问题 ID,用于支持调整表单问题的顺序,通过前一个表单问题的 field_id 来确定位置;如果 pre_field_id 为空字符串,则说明要排到首个表单问题
*
示例值:fldjX7dUj5
*
* @param preFieldId
* @return
*/
public Builder preFieldId(String preFieldId) {
this.preFieldId = preFieldId;
return this;
}
/**
* 表单问题
*
示例值:多行文本
*
* @param title
* @return
*/
public Builder title(String title) {
this.title = title;
return this;
}
/**
* 问题描述
*
示例值:多行文本描述
*
* @param description
* @return
*/
public Builder description(String description) {
this.description = description;
return this;
}
/**
* 是否必填
*
示例值:true
*
* @param required
* @return
*/
public Builder required(Boolean required) {
this.required = required;
return this;
}
/**
* 是否可见,当值为 false 时,不允许更新其他字段。
*
示例值:true
*
* @param visible
* @return
*/
public Builder visible(Boolean visible) {
this.visible = visible;
return this;
}
public AppTableFormPatchedField build() {
return new AppTableFormPatchedField(this);
}
}
}