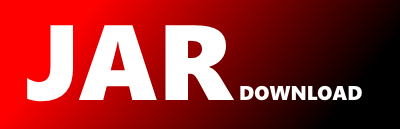
com.lark.oapi.service.contact.v3.resource.Unit Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.contact.v3.resource;
import com.lark.oapi.core.token.AccessTokenType;
import com.lark.oapi.core.Transport;
import com.lark.oapi.core.response.RawResponse;
import com.lark.oapi.core.utils.UnmarshalRespUtil;
import com.lark.oapi.core.utils.Jsons;
import com.lark.oapi.core.utils.Sets;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.nio.charset.StandardCharsets;
import com.lark.oapi.core.Config;
import com.lark.oapi.core.request.RequestOptions;
import java.io.ByteArrayOutputStream;
import com.lark.oapi.service.contact.v3.model.*;
import java.io.*;
import java.util.Map;
import java.util.HashMap;
import java.util.Arrays;
import java.util.List;
import java.util.ArrayList;
public class Unit {
private static final Logger log = LoggerFactory.getLogger(Unit.class);
private final Config config;
public Unit(Config config) {
this.config = config;
}
/**
* 建立部门与单位的绑定关系,通过该接口建立部门与单位的绑定关系。由于单位是旗舰版付费功能,企业需开通相关版本,否则会绑定失败,不同版本请参考[飞书版本对比](https://www.feishu.cn/service)
* 官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/bind_department ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/BindDepartmentUnitSample.java ;
*/
public BindDepartmentUnitResp bindDepartment(BindDepartmentUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/contact/v3/unit/bind_department"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
BindDepartmentUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, BindDepartmentUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/bind_department"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 建立部门与单位的绑定关系,通过该接口建立部门与单位的绑定关系。由于单位是旗舰版付费功能,企业需开通相关版本,否则会绑定失败,不同版本请参考[飞书版本对比](https://www.feishu.cn/service)
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/bind_department ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/BindDepartmentUnitSample.java ;
*/
public BindDepartmentUnitResp bindDepartment(BindDepartmentUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/contact/v3/unit/bind_department"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
BindDepartmentUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, BindDepartmentUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/bind_department"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 创建单位,该接口用于创建单位。注意:单位功能属于旗舰版付费功能,企业需开通对应版本才可以创建单位,不同版本请参考[飞书版本对比](https://www.feishu.cn/service)。
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/create ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/CreateUnitSample.java ;
*/
public CreateUnitResp create(CreateUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/contact/v3/unit"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
CreateUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, CreateUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 创建单位,该接口用于创建单位。注意:单位功能属于旗舰版付费功能,企业需开通对应版本才可以创建单位,不同版本请参考[飞书版本对比](https://www.feishu.cn/service)。
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/create ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/CreateUnitSample.java ;
*/
public CreateUnitResp create(CreateUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/contact/v3/unit"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
CreateUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, CreateUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 删除单位,使用该接口删除单位,需要有更新单位的权限。注意:如果单位的单位类型被其它的业务使用,不允许删除。
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/delete ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/DeleteUnitSample.java ;
*/
public DeleteUnitResp delete(DeleteUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "DELETE"
, "/open-apis/contact/v3/unit/:unit_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
DeleteUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, DeleteUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/:unit_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 删除单位,使用该接口删除单位,需要有更新单位的权限。注意:如果单位的单位类型被其它的业务使用,不允许删除。
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/delete ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/DeleteUnitSample.java ;
*/
public DeleteUnitResp delete(DeleteUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "DELETE"
, "/open-apis/contact/v3/unit/:unit_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
DeleteUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, DeleteUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/:unit_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取单位信息,该接口用于获取单位信息
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/GetUnitSample.java ;
*/
public GetUnitResp get(GetUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/contact/v3/unit/:unit_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
GetUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/:unit_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取单位信息,该接口用于获取单位信息
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/GetUnitSample.java ;
*/
public GetUnitResp get(GetUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/contact/v3/unit/:unit_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
GetUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/:unit_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 批量获取单位列表,通过该接口获取企业的单位列表,需获取单位的权限
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/list ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/ListUnitSample.java ;
*/
public ListUnitResp list(ListUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/contact/v3/unit"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ListUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ListUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 批量获取单位列表,通过该接口获取企业的单位列表,需获取单位的权限
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/list ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/ListUnitSample.java ;
*/
public ListUnitResp list(ListUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/contact/v3/unit"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ListUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ListUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取单位绑定的部门列表,通过该接口获取单位绑定的部门列表,需具有获取单位的权限
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/list_department ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/ListDepartmentUnitSample.java ;
*/
public ListDepartmentUnitResp listDepartment(ListDepartmentUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/contact/v3/unit/list_department"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ListDepartmentUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ListDepartmentUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/list_department"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取单位绑定的部门列表,通过该接口获取单位绑定的部门列表,需具有获取单位的权限
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/list_department ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/ListDepartmentUnitSample.java ;
*/
public ListDepartmentUnitResp listDepartment(ListDepartmentUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/contact/v3/unit/list_department"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ListDepartmentUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ListDepartmentUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/list_department"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 修改单位信息,调用该接口,需要有更新单位的权限。注意:单位功能属于旗舰版付费功能,企业需开通对应版本才可以修改单位
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/patch ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/PatchUnitSample.java ;
*/
public PatchUnitResp patch(PatchUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/contact/v3/unit/:unit_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
PatchUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, PatchUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/:unit_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 修改单位信息,调用该接口,需要有更新单位的权限。注意:单位功能属于旗舰版付费功能,企业需开通对应版本才可以修改单位
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/patch ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/PatchUnitSample.java ;
*/
public PatchUnitResp patch(PatchUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/contact/v3/unit/:unit_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
PatchUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, PatchUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/:unit_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 解除部门与单位的绑定关系,通过该接口解除部门与单位的绑定关系,需更新单位的权限,需对应部门的通讯录权限。由于单位是旗舰版付费功能,企业需开通相关功能,否则会解绑失败
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/unbind_department ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/UnbindDepartmentUnitSample.java ;
*/
public UnbindDepartmentUnitResp unbindDepartment(UnbindDepartmentUnitReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/contact/v3/unit/unbind_department"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UnbindDepartmentUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UnbindDepartmentUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/unbind_department"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 解除部门与单位的绑定关系,通过该接口解除部门与单位的绑定关系,需更新单位的权限,需对应部门的通讯录权限。由于单位是旗舰版付费功能,企业需开通相关功能,否则会解绑失败
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/contact-v3/unit/unbind_department ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/contactv3/UnbindDepartmentUnitSample.java ;
*/
public UnbindDepartmentUnitResp unbindDepartment(UnbindDepartmentUnitReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/contact/v3/unit/unbind_department"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UnbindDepartmentUnitResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UnbindDepartmentUnitResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/contact/v3/unit/unbind_department"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
}