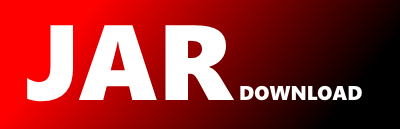
com.lark.oapi.service.hire.v1.model.RoleDetail Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.hire.v1.model;
import com.lark.oapi.core.response.EmptyData;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.SerializedName;
import com.lark.oapi.core.annotation.Body;
import com.lark.oapi.core.annotation.Path;
import com.lark.oapi.core.annotation.Query;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lark.oapi.core.utils.Strings;
import com.lark.oapi.core.response.BaseResponse;
public class RoleDetail {
/**
* 角色ID
* 示例值:6930815272790114324
*/
@SerializedName("id")
private String id;
/**
* 角色名称
*
示例值:
*/
@SerializedName("name")
private I18n name;
/**
* 角色描述
*
示例值:
*/
@SerializedName("description")
private I18n description;
/**
* 更新时间
*
示例值:1716535727510
*/
@SerializedName("modify_time")
private String modifyTime;
/**
* 停启用状态
*
示例值:
*/
@SerializedName("role_status")
private Integer roleStatus;
/**
* 角色类型
*
示例值:
*/
@SerializedName("role_type")
private Integer roleType;
/**
* 适用范围
*
示例值:
*/
@SerializedName("scope_of_application")
private Integer scopeOfApplication;
/**
* 是否在角色上配置业务管理范围
*
示例值:true
*/
@SerializedName("has_business_management_scope")
private Boolean hasBusinessManagementScope;
/**
* 社招权限配置
*
示例值:
*/
@SerializedName("socail_permission_collection")
private PermissionCollection socailPermissionCollection;
/**
* 校招权限配置
*
示例值:
*/
@SerializedName("campus_permission_collection")
private PermissionCollection campusPermissionCollection;
// builder 开始
public RoleDetail() {
}
public RoleDetail(Builder builder) {
/**
* 角色ID
*
示例值:6930815272790114324
*/
this.id = builder.id;
/**
* 角色名称
*
示例值:
*/
this.name = builder.name;
/**
* 角色描述
*
示例值:
*/
this.description = builder.description;
/**
* 更新时间
*
示例值:1716535727510
*/
this.modifyTime = builder.modifyTime;
/**
* 停启用状态
*
示例值:
*/
this.roleStatus = builder.roleStatus;
/**
* 角色类型
*
示例值:
*/
this.roleType = builder.roleType;
/**
* 适用范围
*
示例值:
*/
this.scopeOfApplication = builder.scopeOfApplication;
/**
* 是否在角色上配置业务管理范围
*
示例值:true
*/
this.hasBusinessManagementScope = builder.hasBusinessManagementScope;
/**
* 社招权限配置
*
示例值:
*/
this.socailPermissionCollection = builder.socailPermissionCollection;
/**
* 校招权限配置
*
示例值:
*/
this.campusPermissionCollection = builder.campusPermissionCollection;
}
public static Builder newBuilder() {
return new Builder();
}
public String getId() {
return this.id;
}
public void setId(String id) {
this.id = id;
}
public I18n getName() {
return this.name;
}
public void setName(I18n name) {
this.name = name;
}
public I18n getDescription() {
return this.description;
}
public void setDescription(I18n description) {
this.description = description;
}
public String getModifyTime() {
return this.modifyTime;
}
public void setModifyTime(String modifyTime) {
this.modifyTime = modifyTime;
}
public Integer getRoleStatus() {
return this.roleStatus;
}
public void setRoleStatus(Integer roleStatus) {
this.roleStatus = roleStatus;
}
public Integer getRoleType() {
return this.roleType;
}
public void setRoleType(Integer roleType) {
this.roleType = roleType;
}
public Integer getScopeOfApplication() {
return this.scopeOfApplication;
}
public void setScopeOfApplication(Integer scopeOfApplication) {
this.scopeOfApplication = scopeOfApplication;
}
public Boolean getHasBusinessManagementScope() {
return this.hasBusinessManagementScope;
}
public void setHasBusinessManagementScope(Boolean hasBusinessManagementScope) {
this.hasBusinessManagementScope = hasBusinessManagementScope;
}
public PermissionCollection getSocailPermissionCollection() {
return this.socailPermissionCollection;
}
public void setSocailPermissionCollection(PermissionCollection socailPermissionCollection) {
this.socailPermissionCollection = socailPermissionCollection;
}
public PermissionCollection getCampusPermissionCollection() {
return this.campusPermissionCollection;
}
public void setCampusPermissionCollection(PermissionCollection campusPermissionCollection) {
this.campusPermissionCollection = campusPermissionCollection;
}
public static class Builder {
/**
* 角色ID
*
示例值:6930815272790114324
*/
private String id;
/**
* 角色名称
*
示例值:
*/
private I18n name;
/**
* 角色描述
*
示例值:
*/
private I18n description;
/**
* 更新时间
*
示例值:1716535727510
*/
private String modifyTime;
/**
* 停启用状态
*
示例值:
*/
private Integer roleStatus;
/**
* 角色类型
*
示例值:
*/
private Integer roleType;
/**
* 适用范围
*
示例值:
*/
private Integer scopeOfApplication;
/**
* 是否在角色上配置业务管理范围
*
示例值:true
*/
private Boolean hasBusinessManagementScope;
/**
* 社招权限配置
*
示例值:
*/
private PermissionCollection socailPermissionCollection;
/**
* 校招权限配置
*
示例值:
*/
private PermissionCollection campusPermissionCollection;
/**
* 角色ID
*
示例值:6930815272790114324
*
* @param id
* @return
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* 角色名称
*
示例值:
*
* @param name
* @return
*/
public Builder name(I18n name) {
this.name = name;
return this;
}
/**
* 角色描述
*
示例值:
*
* @param description
* @return
*/
public Builder description(I18n description) {
this.description = description;
return this;
}
/**
* 更新时间
*
示例值:1716535727510
*
* @param modifyTime
* @return
*/
public Builder modifyTime(String modifyTime) {
this.modifyTime = modifyTime;
return this;
}
/**
* 停启用状态
*
示例值:
*
* @param roleStatus
* @return
*/
public Builder roleStatus(Integer roleStatus) {
this.roleStatus = roleStatus;
return this;
}
/**
* 角色类型
*
示例值:
*
* @param roleType
* @return
*/
public Builder roleType(Integer roleType) {
this.roleType = roleType;
return this;
}
/**
* 适用范围
*
示例值:
*
* @param scopeOfApplication
* @return
*/
public Builder scopeOfApplication(Integer scopeOfApplication) {
this.scopeOfApplication = scopeOfApplication;
return this;
}
/**
* 是否在角色上配置业务管理范围
*
示例值:true
*
* @param hasBusinessManagementScope
* @return
*/
public Builder hasBusinessManagementScope(Boolean hasBusinessManagementScope) {
this.hasBusinessManagementScope = hasBusinessManagementScope;
return this;
}
/**
* 社招权限配置
*
示例值:
*
* @param socailPermissionCollection
* @return
*/
public Builder socailPermissionCollection(PermissionCollection socailPermissionCollection) {
this.socailPermissionCollection = socailPermissionCollection;
return this;
}
/**
* 校招权限配置
*
示例值:
*
* @param campusPermissionCollection
* @return
*/
public Builder campusPermissionCollection(PermissionCollection campusPermissionCollection) {
this.campusPermissionCollection = campusPermissionCollection;
return this;
}
public RoleDetail build() {
return new RoleDetail(this);
}
}
}