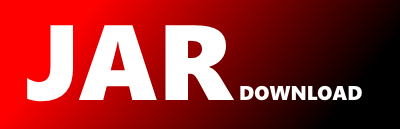
com.lark.oapi.service.im.v1.resource.ChatMembers Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.im.v1.resource;
import com.lark.oapi.core.token.AccessTokenType;
import com.lark.oapi.core.Transport;
import com.lark.oapi.core.response.RawResponse;
import com.lark.oapi.core.utils.UnmarshalRespUtil;
import com.lark.oapi.core.utils.Jsons;
import com.lark.oapi.core.utils.Sets;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.nio.charset.StandardCharsets;
import com.lark.oapi.core.Config;
import com.lark.oapi.core.request.RequestOptions;
import java.io.ByteArrayOutputStream;
import com.lark.oapi.service.im.v1.model.*;
import java.io.*;
import java.util.Map;
import java.util.HashMap;
import java.util.Arrays;
import java.util.List;
import java.util.ArrayList;
public class ChatMembers {
private static final Logger log = LoggerFactory.getLogger(ChatMembers.class);
private final Config config;
public ChatMembers(Config config) {
this.config = config;
}
/**
* 将用户或机器人拉入群聊,将用户或机器人拉入群聊。
* 注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability); - 如需拉用户进群,需要机器人对用户有[可用性](https://open.feishu.cn/document/home/introduction-to-scope-and-authorization/availability); - 机器人或授权用户必须在群组中;- 外部租户不能被加入到内部群中;- 操作内部群时,操作者须与群组在同一租户下; - 在开启 ==仅群主和群管理员可添加群成员== 的设置时,仅有群主/管理员 或 创建群组且具备 ==更新应用所创建群的群信息== 权限的机器人,可以拉用户或者机器人进群; - 在未开启 ==仅群主和群管理员可添加群成员== 的设置时,所有群成员都可以拉用户或机器人进群 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/create ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/CreateChatMembersSample.java ;
*/
public CreateChatMembersResp create(CreateChatMembersReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/chats/:chat_id/members"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
CreateChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, CreateChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 将用户或机器人拉入群聊,将用户或机器人拉入群聊。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability); - 如需拉用户进群,需要机器人对用户有[可用性](https://open.feishu.cn/document/home/introduction-to-scope-and-authorization/availability); - 机器人或授权用户必须在群组中;- 外部租户不能被加入到内部群中;- 操作内部群时,操作者须与群组在同一租户下; - 在开启 ==仅群主和群管理员可添加群成员== 的设置时,仅有群主/管理员 或 创建群组且具备 ==更新应用所创建群的群信息== 权限的机器人,可以拉用户或者机器人进群; - 在未开启 ==仅群主和群管理员可添加群成员== 的设置时,所有群成员都可以拉用户或机器人进群 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/create ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/CreateChatMembersSample.java ;
*/
public CreateChatMembersResp create(CreateChatMembersReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/chats/:chat_id/members"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
CreateChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, CreateChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 将用户或机器人移出群聊,将用户或机器人移出群聊。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 用户或机器人在任何条件下均可移除自己出群(即主动退群);- 仅有群主/管理员 或 创建群组并且具备 ==更新应用所创建群的群信息== 权限的机器人,可以移除其他用户或者机器人;- 每次请求,最多移除50个用户或者5个机器人;- 操作内部群时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/delete ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/DeleteChatMembersSample.java ;
*/
public DeleteChatMembersResp delete(DeleteChatMembersReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "DELETE"
, "/open-apis/im/v1/chats/:chat_id/members"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
DeleteChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, DeleteChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 将用户或机器人移出群聊,将用户或机器人移出群聊。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 用户或机器人在任何条件下均可移除自己出群(即主动退群);- 仅有群主/管理员 或 创建群组并且具备 ==更新应用所创建群的群信息== 权限的机器人,可以移除其他用户或者机器人;- 每次请求,最多移除50个用户或者5个机器人;- 操作内部群时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/delete ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/DeleteChatMembersSample.java ;
*/
public DeleteChatMembersResp delete(DeleteChatMembersReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "DELETE"
, "/open-apis/im/v1/chats/:chat_id/members"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
DeleteChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, DeleteChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取群成员列表,获取用户/机器人所在群的群成员列表。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability); - 机器人或授权用户必须在群组中; - 该接口不会返回群内的机器人成员; - 由于返回的群成员列表会过滤掉机器人成员,因此返回的群成员个数可能会小于指定的page_size; - 如果有同一时间加入群的群成员,会一次性返回,这会导致返回的群成员个数可能会大于指定的page_size;- 获取内部群信息时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/GetChatMembersSample.java ;
*/
public GetChatMembersResp get(GetChatMembersReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/chats/:chat_id/members"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
GetChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取群成员列表,获取用户/机器人所在群的群成员列表。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability); - 机器人或授权用户必须在群组中; - 该接口不会返回群内的机器人成员; - 由于返回的群成员列表会过滤掉机器人成员,因此返回的群成员个数可能会小于指定的page_size; - 如果有同一时间加入群的群成员,会一次性返回,这会导致返回的群成员个数可能会大于指定的page_size;- 获取内部群信息时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/GetChatMembersSample.java ;
*/
public GetChatMembersResp get(GetChatMembersReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/chats/:chat_id/members"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
GetChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 判断用户或机器人是否在群里,根据使用的access_token判断对应的用户或者机器人是否在群里。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 获取内部群信息时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/is_in_chat ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/IsInChatChatMembersSample.java ;
*/
public IsInChatChatMembersResp isInChat(IsInChatChatMembersReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/chats/:chat_id/members/is_in_chat"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
IsInChatChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, IsInChatChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members/is_in_chat"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 判断用户或机器人是否在群里,根据使用的access_token判断对应的用户或者机器人是否在群里。
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 获取内部群信息时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/is_in_chat ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/IsInChatChatMembersSample.java ;
*/
public IsInChatChatMembersResp isInChat(IsInChatChatMembersReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/chats/:chat_id/members/is_in_chat"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
IsInChatChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, IsInChatChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members/is_in_chat"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 用户或机器人主动加入群聊,用户或机器人主动加入群聊。
*
注意事项:;- 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 目前仅支持加入公开群;- 操作内部群时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/me_join ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/MeJoinChatMembersSample.java ;
*/
public MeJoinChatMembersResp meJoin(MeJoinChatMembersReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/chats/:chat_id/members/me_join"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
MeJoinChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, MeJoinChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members/me_join"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 用户或机器人主动加入群聊,用户或机器人主动加入群聊。
*
注意事项:;- 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 目前仅支持加入公开群;- 操作内部群时,操作者须与群组在同一租户下 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-members/me_join ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/MeJoinChatMembersSample.java ;
*/
public MeJoinChatMembersResp meJoin(MeJoinChatMembersReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/chats/:chat_id/members/me_join"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
MeJoinChatMembersResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, MeJoinChatMembersResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/members/me_join"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
}