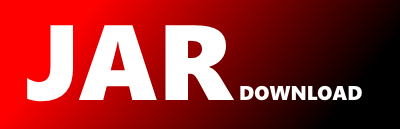
com.lark.oapi.service.im.v1.resource.ChatModeration Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.im.v1.resource;
import com.lark.oapi.core.token.AccessTokenType;
import com.lark.oapi.core.Transport;
import com.lark.oapi.core.response.RawResponse;
import com.lark.oapi.core.utils.UnmarshalRespUtil;
import com.lark.oapi.core.utils.Jsons;
import com.lark.oapi.core.utils.Sets;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.nio.charset.StandardCharsets;
import com.lark.oapi.core.Config;
import com.lark.oapi.core.request.RequestOptions;
import java.io.ByteArrayOutputStream;
import com.lark.oapi.service.im.v1.model.*;
import java.io.*;
import java.util.Map;
import java.util.HashMap;
import java.util.Arrays;
import java.util.List;
import java.util.ArrayList;
public class ChatModeration {
private static final Logger log = LoggerFactory.getLogger(ChatModeration.class);
private final Config config;
public ChatModeration(Config config) {
this.config = config;
}
/**
* 获取群成员发言权限,获取群发言模式、可发言用户名单等
* 注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability); - 机器人 或 授权用户 必须在群里 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-moderation/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/GetChatModerationSample.java ;
*/
public GetChatModerationResp get(GetChatModerationReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/chats/:chat_id/moderation"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
GetChatModerationResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetChatModerationResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/moderation"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取群成员发言权限,获取群发言模式、可发言用户名单等
*
注意事项:; - 应用需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability); - 机器人 或 授权用户 必须在群里 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-moderation/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/GetChatModerationSample.java ;
*/
public GetChatModerationResp get(GetChatModerationReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/chats/:chat_id/moderation"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
GetChatModerationResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetChatModerationResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/moderation"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 更新群发言权限,更新群组的发言权限设置,可设置为全员可发言、仅管理员可发言 或 指定用户可发言。
*
注意事项:; - 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 若以用户授权调用接口,**当授权用户是群主**时,可更新群发言权限;- 若以租户授权调用接口(即以机器人身份调用接口),当**机器人是群主** 或者 **机器人是群组创建者、具备==更新应用所创建群的群信息==权限且仍在群内**时,可更新群发言权限 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-moderation/update ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UpdateChatModerationSample.java ;
*/
public UpdateChatModerationResp update(UpdateChatModerationReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PUT"
, "/open-apis/im/v1/chats/:chat_id/moderation"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
UpdateChatModerationResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UpdateChatModerationResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/moderation"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 更新群发言权限,更新群组的发言权限设置,可设置为全员可发言、仅管理员可发言 或 指定用户可发言。
*
注意事项:; - 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 若以用户授权调用接口,**当授权用户是群主**时,可更新群发言权限;- 若以租户授权调用接口(即以机器人身份调用接口),当**机器人是群主** 或者 **机器人是群组创建者、具备==更新应用所创建群的群信息==权限且仍在群内**时,可更新群发言权限 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/chat-moderation/update ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UpdateChatModerationSample.java ;
*/
public UpdateChatModerationResp update(UpdateChatModerationReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PUT"
, "/open-apis/im/v1/chats/:chat_id/moderation"
, Sets.newHashSet(AccessTokenType.User, AccessTokenType.Tenant)
, req);
// 反序列化
UpdateChatModerationResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UpdateChatModerationResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/chats/:chat_id/moderation"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
}