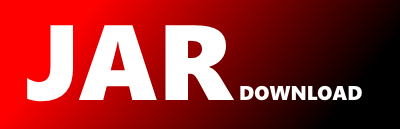
com.lark.oapi.service.im.v1.resource.Message Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.im.v1.resource;
import com.lark.oapi.core.token.AccessTokenType;
import com.lark.oapi.core.Transport;
import com.lark.oapi.core.response.RawResponse;
import com.lark.oapi.core.utils.UnmarshalRespUtil;
import com.lark.oapi.core.utils.Jsons;
import com.lark.oapi.core.utils.Sets;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.nio.charset.StandardCharsets;
import com.lark.oapi.core.Config;
import com.lark.oapi.core.request.RequestOptions;
import java.io.ByteArrayOutputStream;
import com.lark.oapi.service.im.v1.model.*;
import java.io.*;
import java.util.Map;
import java.util.HashMap;
import java.util.Arrays;
import java.util.List;
import java.util.ArrayList;
public class Message {
private static final Logger log = LoggerFactory.getLogger(Message.class);
private final Config config;
public Message(Config config) {
this.config = config;
}
/**
* 发送消息,给指定用户或者会话发送消息,支持文本、富文本、可交互的[消息卡片](https://open.feishu.cn/document/ukTMukTMukTM/uczM3QjL3MzN04yNzcDN)、群名片、个人名片、图片、视频、音频、文件、表情包。
* 注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 给用户发送消息,需要机器人对用户有[可用性](https://open.feishu.cn/document/home/introduction-to-scope-and-authorization/availability);- 给群组发送消息,需要机器人在群组中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/create ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/CreateMessageSample.java ;
*/
public CreateMessageResp create(CreateMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
CreateMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, CreateMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送消息,给指定用户或者会话发送消息,支持文本、富文本、可交互的[消息卡片](https://open.feishu.cn/document/ukTMukTMukTM/uczM3QjL3MzN04yNzcDN)、群名片、个人名片、图片、视频、音频、文件、表情包。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 给用户发送消息,需要机器人对用户有[可用性](https://open.feishu.cn/document/home/introduction-to-scope-and-authorization/availability);- 给群组发送消息,需要机器人在群组中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/create ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/CreateMessageSample.java ;
*/
public CreateMessageResp create(CreateMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
CreateMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, CreateMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 撤回消息,机器人撤回机器人自己发送的消息或群主撤回群内消息。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ,撤回消息时机器人仍需要在会话内;- 机器人可以撤回单聊和群组内,自己发送 且 发送时间不超过1天(24小时)的消息;- 若机器人要撤回群内他人发送的消息,则机器人必须是该群的群主、管理员 或者 创建者,且消息发送时间不超过1年;- 无法撤回通过「[批量发送消息](https://open.feishu.cn/document/ukTMukTMukTM/ucDO1EjL3gTNx4yN4UTM)」接口发送的消息 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/delete ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/DeleteMessageSample.java ;
*/
public DeleteMessageResp delete(DeleteMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "DELETE"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
DeleteMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, DeleteMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 撤回消息,机器人撤回机器人自己发送的消息或群主撤回群内消息。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ,撤回消息时机器人仍需要在会话内;- 机器人可以撤回单聊和群组内,自己发送 且 发送时间不超过1天(24小时)的消息;- 若机器人要撤回群内他人发送的消息,则机器人必须是该群的群主、管理员 或者 创建者,且消息发送时间不超过1年;- 无法撤回通过「[批量发送消息](https://open.feishu.cn/document/ukTMukTMukTM/ucDO1EjL3gTNx4yN4UTM)」接口发送的消息 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/delete ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/DeleteMessageSample.java ;
*/
public DeleteMessageResp delete(DeleteMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "DELETE"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
DeleteMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, DeleteMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,转发一条消息
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=forward&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ForwardMessageSample.java ;
*/
public ForwardMessageResp forward(ForwardMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/:message_id/forward"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ForwardMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ForwardMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/forward"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,转发一条消息
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=forward&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ForwardMessageSample.java ;
*/
public ForwardMessageResp forward(ForwardMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/:message_id/forward"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ForwardMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ForwardMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/forward"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取指定消息的内容,通过 message_id 查询消息内容。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 机器人必须在群组中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/GetMessageSample.java ;
*/
public GetMessageResp get(GetMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
GetMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取指定消息的内容,通过 message_id 查询消息内容。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 机器人必须在群组中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/get ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/GetMessageSample.java ;
*/
public GetMessageResp get(GetMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
GetMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, GetMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取会话历史消息,获取会话(包括单聊、群组)的历史消息(聊天记录)。
*
接口级别权限默认只能获取单聊(p2p)消息,如果需要获取群组(group)消息,应用还必须拥有 **==获取群组中所有消息==** 权限 ;
*
- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 获取消息时,机器人必须在群组中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/list ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ListMessageSample.java ;
*/
public ListMessageResp list(ListMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/messages"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ListMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ListMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 获取会话历史消息,获取会话(包括单聊、群组)的历史消息(聊天记录)。
*
接口级别权限默认只能获取单聊(p2p)消息,如果需要获取群组(group)消息,应用还必须拥有 **==获取群组中所有消息==** 权限 ;
*
- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 获取消息时,机器人必须在群组中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/list ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ListMessageSample.java ;
*/
public ListMessageResp list(ListMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/messages"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ListMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ListMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,合并转发多条消息
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=merge_forward&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/MergeForwardMessageSample.java ;
*/
public MergeForwardMessageResp mergeForward(MergeForwardMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/merge_forward"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
MergeForwardMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, MergeForwardMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/merge_forward"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,合并转发多条消息
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=merge_forward&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/MergeForwardMessageSample.java ;
*/
public MergeForwardMessageResp mergeForward(MergeForwardMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/merge_forward"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
MergeForwardMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, MergeForwardMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/merge_forward"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 更新应用发送的消息,更新应用已发送的消息卡片内容。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 若以user_access_token更新消息,该操作用户必须是卡片消息的发送者;- 仅支持对所有人更新**未撤回**的[「共享卡片」](ukTMukTMukTM/uAjNwUjLwYDM14CM2ATN)消息,需在卡片的config属性中,显式声明 =="update_multi":true==。 ;- **不支持更新批量消息**;- 文本消息请求体最大不能超过150KB;卡片及富文本消息请求体最大不能超过30KB;- 仅支持修改14天内发送的消息;- 单条消息更新频控为**5QPS** ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/patch ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/PatchMessageSample.java ;
*/
public PatchMessageResp patch(PatchMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
PatchMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, PatchMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 更新应用发送的消息,更新应用已发送的消息卡片内容。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability);- 若以user_access_token更新消息,该操作用户必须是卡片消息的发送者;- 仅支持对所有人更新**未撤回**的[「共享卡片」](ukTMukTMukTM/uAjNwUjLwYDM14CM2ATN)消息,需在卡片的config属性中,显式声明 =="update_multi":true==。 ;- **不支持更新批量消息**;- 文本消息请求体最大不能超过150KB;卡片及富文本消息请求体最大不能超过30KB;- 仅支持修改14天内发送的消息;- 单条消息更新频控为**5QPS** ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/patch ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/PatchMessageSample.java ;
*/
public PatchMessageResp patch(PatchMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
PatchMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, PatchMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=push_follow_up&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/PushFollowUpMessageSample.java ;
*/
public PushFollowUpMessageResp pushFollowUp(PushFollowUpMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/:message_id/push_follow_up"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
PushFollowUpMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, PushFollowUpMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/push_follow_up"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=push_follow_up&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/PushFollowUpMessageSample.java ;
*/
public PushFollowUpMessageResp pushFollowUp(PushFollowUpMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/:message_id/push_follow_up"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
PushFollowUpMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, PushFollowUpMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/push_follow_up"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 查询消息已读信息,查询消息的已读信息。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 只能查询机器人自己发送,且发送时间不超过7天的消息;- 查询消息已读信息时机器人仍需要在会话内;- 本接口不支持查询批量消息 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/read_users ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ReadUsersMessageSample.java ;
*/
public ReadUsersMessageResp readUsers(ReadUsersMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/messages/:message_id/read_users"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ReadUsersMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ReadUsersMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/read_users"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 查询消息已读信息,查询消息的已读信息。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 只能查询机器人自己发送,且发送时间不超过7天的消息;- 查询消息已读信息时机器人仍需要在会话内;- 本接口不支持查询批量消息 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/read_users ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ReadUsersMessageSample.java ;
*/
public ReadUsersMessageResp readUsers(ReadUsersMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "GET"
, "/open-apis/im/v1/messages/:message_id/read_users"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
ReadUsersMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ReadUsersMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/read_users"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 回复消息,回复指定消息,支持文本、富文本、卡片、群名片、个人名片、图片、视频、文件等多种消息类型。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 回复私聊消息,需要机器人对用户有[可用性](https://open.feishu.cn/document/home/introduction-to-scope-and-authorization/availability);- 回复群组消息,需要机器人在群中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/reply ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ReplyMessageSample.java ;
*/
public ReplyMessageResp reply(ReplyMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/:message_id/reply"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
ReplyMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ReplyMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/reply"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 回复消息,回复指定消息,支持文本、富文本、卡片、群名片、个人名片、图片、视频、文件等多种消息类型。
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 回复私聊消息,需要机器人对用户有[可用性](https://open.feishu.cn/document/home/introduction-to-scope-and-authorization/availability);- 回复群组消息,需要机器人在群中 ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/reply ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/ReplyMessageSample.java ;
*/
public ReplyMessageResp reply(ReplyMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "POST"
, "/open-apis/im/v1/messages/:message_id/reply"
, Sets.newHashSet(AccessTokenType.Tenant, AccessTokenType.User)
, req);
// 反序列化
ReplyMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, ReplyMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/reply"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,编辑已发送的消息内容,当前仅支持编辑文本和富文本消息。
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=update&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UpdateMessageSample.java ;
*/
public UpdateMessageResp update(UpdateMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PUT"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UpdateMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UpdateMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* ,编辑已发送的消息内容,当前仅支持编辑文本和富文本消息。
*
官网API文档链接:https://open.feishu.cn/api-explorer?from=op_doc_tab&apiName=update&project=im&resource=message&version=v1 ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UpdateMessageSample.java ;
*/
public UpdateMessageResp update(UpdateMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PUT"
, "/open-apis/im/v1/messages/:message_id"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UpdateMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UpdateMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送应用内加急,对指定消息进行应用内加急。
*
特别说明:;- 默认接口限流为50 QPS,请谨慎调用 ;
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 不支持加急批量消息;- 只能加急机器人自己发送的消息;- 加急时机器人需要在加急消息所在的群中;- 调用本接口需要用户已阅读加急的消息才可以继续加急(用户未读的加急上限为200条) ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/urgent_app ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UrgentAppMessageSample.java ;
*/
public UrgentAppMessageResp urgentApp(UrgentAppMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id/urgent_app"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UrgentAppMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UrgentAppMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/urgent_app"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送应用内加急,对指定消息进行应用内加急。
*
特别说明:;- 默认接口限流为50 QPS,请谨慎调用 ;
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 不支持加急批量消息;- 只能加急机器人自己发送的消息;- 加急时机器人需要在加急消息所在的群中;- 调用本接口需要用户已阅读加急的消息才可以继续加急(用户未读的加急上限为200条) ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/urgent_app ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UrgentAppMessageSample.java ;
*/
public UrgentAppMessageResp urgentApp(UrgentAppMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id/urgent_app"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UrgentAppMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UrgentAppMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/urgent_app"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送电话加急,对指定消息进行应用内加急与电话加急。
*
特别说明:;- 通过接口产生的电话加急将消耗企业的加急额度,请慎重调用;- 通过[租户管理后台](https://admin.feishu.cn/)-费用中心-短信/电话加急 可以查看当前额度;- 默认接口限流为50 QPS,请谨慎调用 ;
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 只能加急机器人自己发送的消息;- 加急时机器人需要在加急消息所在的群组中;- 需要用户阅读已加急的消息才可以继续加急(用户未读的加急上限为200条) ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/urgent_phone ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UrgentPhoneMessageSample.java ;
*/
public UrgentPhoneMessageResp urgentPhone(UrgentPhoneMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id/urgent_phone"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UrgentPhoneMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UrgentPhoneMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/urgent_phone"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送电话加急,对指定消息进行应用内加急与电话加急。
*
特别说明:;- 通过接口产生的电话加急将消耗企业的加急额度,请慎重调用;- 通过[租户管理后台](https://admin.feishu.cn/)-费用中心-短信/电话加急 可以查看当前额度;- 默认接口限流为50 QPS,请谨慎调用 ;
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 只能加急机器人自己发送的消息;- 加急时机器人需要在加急消息所在的群组中;- 需要用户阅读已加急的消息才可以继续加急(用户未读的加急上限为200条) ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/urgent_phone ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UrgentPhoneMessageSample.java ;
*/
public UrgentPhoneMessageResp urgentPhone(UrgentPhoneMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id/urgent_phone"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UrgentPhoneMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UrgentPhoneMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/urgent_phone"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送短信加急,对指定消息进行应用内加急与短信加急。
*
特别说明:;- 通过接口产生的短信加急将消耗企业的加急额度,请慎重调用;- 通过[租户管理后台](https://admin.feishu.cn/)-费用中心-短信/电话加急 可以查看当前额度;- 默认接口限流为50 QPS,请谨慎调用 ;
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 只能加急机器人自己发送的消息;- 加急时机器人仍需要在加急消息所在的群组中;- 调用本接口需要用户已阅读加急的消息才可以继续加急(用户未读的加急上限为200条) ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/urgent_sms ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UrgentSmsMessageSample.java ;
*/
public UrgentSmsMessageResp urgentSms(UrgentSmsMessageReq req, RequestOptions reqOptions) throws Exception {
// 请求参数选项
if (reqOptions == null) {
reqOptions = new RequestOptions();
}
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id/urgent_sms"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UrgentSmsMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UrgentSmsMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/urgent_sms"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
/**
* 发送短信加急,对指定消息进行应用内加急与短信加急。
*
特别说明:;- 通过接口产生的短信加急将消耗企业的加急额度,请慎重调用;- 通过[租户管理后台](https://admin.feishu.cn/)-费用中心-短信/电话加急 可以查看当前额度;- 默认接口限流为50 QPS,请谨慎调用 ;
*
注意事项:;- 需要开启[机器人能力](https://open.feishu.cn/document/uAjLw4CM/ugTN1YjL4UTN24CO1UjN/trouble-shooting/how-to-enable-bot-ability) ;- 只能加急机器人自己发送的消息;- 加急时机器人仍需要在加急消息所在的群组中;- 调用本接口需要用户已阅读加急的消息才可以继续加急(用户未读的加急上限为200条) ;
*
官网API文档链接:https://open.feishu.cn/document/uAjLw4CM/ukTMukTMukTM/reference/im-v1/message/urgent_sms ;
*
使用Demo链接: https://github.com/larksuite/oapi-sdk-java/tree/v2_main/sample/src/main/java/com/lark/oapi/sample/apiall/imv1/UrgentSmsMessageSample.java ;
*/
public UrgentSmsMessageResp urgentSms(UrgentSmsMessageReq req) throws Exception {
// 请求参数选项
RequestOptions reqOptions = new RequestOptions();
// 发起请求
RawResponse httpResponse = Transport.send(config, reqOptions, "PATCH"
, "/open-apis/im/v1/messages/:message_id/urgent_sms"
, Sets.newHashSet(AccessTokenType.Tenant)
, req);
// 反序列化
UrgentSmsMessageResp resp = UnmarshalRespUtil.unmarshalResp(httpResponse, UrgentSmsMessageResp.class);
if (resp == null) {
log.error(String.format(
"%s,callError,req=%s,respHeader=%s,respStatusCode=%s,respBody=%s,", "/open-apis/im/v1/messages/:message_id/urgent_sms"
, Jsons.DEFAULT.toJson(req), Jsons.DEFAULT.toJson(httpResponse.getHeaders()),
httpResponse.getStatusCode(), new String(httpResponse.getBody(),
StandardCharsets.UTF_8)));
throw new IllegalArgumentException("The result returned by the server is illegal");
}
resp.setRawResponse(httpResponse);
resp.setRequest(req);
return resp;
}
}