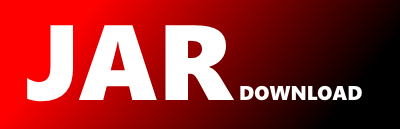
com.lark.oapi.service.mdm.v1.model.LegalEntity Maven / Gradle / Ivy
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.mdm.v1.model;
import com.lark.oapi.core.response.EmptyData;
import com.lark.oapi.service.mdm.v1.enums.*;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.SerializedName;
import com.lark.oapi.core.annotation.Body;
import com.lark.oapi.core.annotation.Path;
import com.lark.oapi.core.annotation.Query;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lark.oapi.core.utils.Strings;
import com.lark.oapi.core.response.BaseResponse;
public class LegalEntity {
/**
* 法人实体id
* 示例值:7003410079584092448
*/
@SerializedName("id")
private String id;
/**
* 法人实体编码(根据配置会有不同的生成规则)
*
示例值:L00002002
*/
@SerializedName("legal_entity")
private String legalEntity;
/**
* 法人实体名称
*
示例值:法人22
*/
@SerializedName("legal_entity_text")
private String legalEntityText;
/**
* 法人实体英文名称
*
示例值:legal_person
*/
@SerializedName("short_text")
private String shortText;
/**
* 证件类型
*
示例值:0
*/
@SerializedName("certification_type")
private String certificationType;
/**
* 证件id
*
示例值:91310120MA1H23N81AX
*/
@SerializedName("certification_id")
private String certificationId;
/**
* 法人
*
示例值:张三
*/
@SerializedName("legal_person")
private String legalPerson;
/**
* 国家
*
示例值:CN
*/
@SerializedName("country")
private String country;
/**
* 省份
*
示例值:MDPS00004000
*/
@SerializedName("province")
private String province;
/**
* 城市
*
示例值:MDCY00006000
*/
@SerializedName("city")
private String city;
/**
* 地址
*
示例值:地址
*/
@SerializedName("address")
private String address;
/**
* 纳税人类型
*
示例值:1
*/
@SerializedName("taxpayer_type")
private String taxpayerType;
/**
* 联系电话
*
示例值:010-58341796
*/
@SerializedName("telephone")
private String telephone;
/**
* 银行内部Id
*
示例值:MDBK00072319
*/
@SerializedName("bank_id")
private String bankId;
/**
* 开户银行名称
*
示例值:中原银行股份有限公司南阳华瑞支行
*/
@SerializedName("bank_name")
private String bankName;
/**
* 开户行账号
*
示例值:644666446
*/
@SerializedName("bank_account")
private String bankAccount;
/**
* 状态
*
示例值:1
*/
@SerializedName("status")
private Integer status;
/**
* 银行账户列表
*
示例值:
*/
@SerializedName("legal_entity_banks")
private LegalEntityBank[] legalEntityBanks;
/**
* 扩展字段相关信息列表
*
示例值:
*/
@SerializedName("extend_info")
private ExtendField[] extendInfo;
/**
* 附件列表
*
示例值:
*/
@SerializedName("appendix")
private Appendix[] appendix;
// builder 开始
public LegalEntity() {
}
public LegalEntity(Builder builder) {
/**
* 法人实体id
*
示例值:7003410079584092448
*/
this.id = builder.id;
/**
* 法人实体编码(根据配置会有不同的生成规则)
*
示例值:L00002002
*/
this.legalEntity = builder.legalEntity;
/**
* 法人实体名称
*
示例值:法人22
*/
this.legalEntityText = builder.legalEntityText;
/**
* 法人实体英文名称
*
示例值:legal_person
*/
this.shortText = builder.shortText;
/**
* 证件类型
*
示例值:0
*/
this.certificationType = builder.certificationType;
/**
* 证件id
*
示例值:91310120MA1H23N81AX
*/
this.certificationId = builder.certificationId;
/**
* 法人
*
示例值:张三
*/
this.legalPerson = builder.legalPerson;
/**
* 国家
*
示例值:CN
*/
this.country = builder.country;
/**
* 省份
*
示例值:MDPS00004000
*/
this.province = builder.province;
/**
* 城市
*
示例值:MDCY00006000
*/
this.city = builder.city;
/**
* 地址
*
示例值:地址
*/
this.address = builder.address;
/**
* 纳税人类型
*
示例值:1
*/
this.taxpayerType = builder.taxpayerType;
/**
* 联系电话
*
示例值:010-58341796
*/
this.telephone = builder.telephone;
/**
* 银行内部Id
*
示例值:MDBK00072319
*/
this.bankId = builder.bankId;
/**
* 开户银行名称
*
示例值:中原银行股份有限公司南阳华瑞支行
*/
this.bankName = builder.bankName;
/**
* 开户行账号
*
示例值:644666446
*/
this.bankAccount = builder.bankAccount;
/**
* 状态
*
示例值:1
*/
this.status = builder.status;
/**
* 银行账户列表
*
示例值:
*/
this.legalEntityBanks = builder.legalEntityBanks;
/**
* 扩展字段相关信息列表
*
示例值:
*/
this.extendInfo = builder.extendInfo;
/**
* 附件列表
*
示例值:
*/
this.appendix = builder.appendix;
}
public static Builder newBuilder() {
return new Builder();
}
public String getId() {
return this.id;
}
public void setId(String id) {
this.id = id;
}
public String getLegalEntity() {
return this.legalEntity;
}
public void setLegalEntity(String legalEntity) {
this.legalEntity = legalEntity;
}
public String getLegalEntityText() {
return this.legalEntityText;
}
public void setLegalEntityText(String legalEntityText) {
this.legalEntityText = legalEntityText;
}
public String getShortText() {
return this.shortText;
}
public void setShortText(String shortText) {
this.shortText = shortText;
}
public String getCertificationType() {
return this.certificationType;
}
public void setCertificationType(String certificationType) {
this.certificationType = certificationType;
}
public String getCertificationId() {
return this.certificationId;
}
public void setCertificationId(String certificationId) {
this.certificationId = certificationId;
}
public String getLegalPerson() {
return this.legalPerson;
}
public void setLegalPerson(String legalPerson) {
this.legalPerson = legalPerson;
}
public String getCountry() {
return this.country;
}
public void setCountry(String country) {
this.country = country;
}
public String getProvince() {
return this.province;
}
public void setProvince(String province) {
this.province = province;
}
public String getCity() {
return this.city;
}
public void setCity(String city) {
this.city = city;
}
public String getAddress() {
return this.address;
}
public void setAddress(String address) {
this.address = address;
}
public String getTaxpayerType() {
return this.taxpayerType;
}
public void setTaxpayerType(String taxpayerType) {
this.taxpayerType = taxpayerType;
}
public String getTelephone() {
return this.telephone;
}
public void setTelephone(String telephone) {
this.telephone = telephone;
}
public String getBankId() {
return this.bankId;
}
public void setBankId(String bankId) {
this.bankId = bankId;
}
public String getBankName() {
return this.bankName;
}
public void setBankName(String bankName) {
this.bankName = bankName;
}
public String getBankAccount() {
return this.bankAccount;
}
public void setBankAccount(String bankAccount) {
this.bankAccount = bankAccount;
}
public Integer getStatus() {
return this.status;
}
public void setStatus(Integer status) {
this.status = status;
}
public LegalEntityBank[] getLegalEntityBanks() {
return this.legalEntityBanks;
}
public void setLegalEntityBanks(LegalEntityBank[] legalEntityBanks) {
this.legalEntityBanks = legalEntityBanks;
}
public ExtendField[] getExtendInfo() {
return this.extendInfo;
}
public void setExtendInfo(ExtendField[] extendInfo) {
this.extendInfo = extendInfo;
}
public Appendix[] getAppendix() {
return this.appendix;
}
public void setAppendix(Appendix[] appendix) {
this.appendix = appendix;
}
public static class Builder {
/**
* 法人实体id
*
示例值:7003410079584092448
*/
private String id;
/**
* 法人实体编码(根据配置会有不同的生成规则)
*
示例值:L00002002
*/
private String legalEntity;
/**
* 法人实体名称
*
示例值:法人22
*/
private String legalEntityText;
/**
* 法人实体英文名称
*
示例值:legal_person
*/
private String shortText;
/**
* 证件类型
*
示例值:0
*/
private String certificationType;
/**
* 证件id
*
示例值:91310120MA1H23N81AX
*/
private String certificationId;
/**
* 法人
*
示例值:张三
*/
private String legalPerson;
/**
* 国家
*
示例值:CN
*/
private String country;
/**
* 省份
*
示例值:MDPS00004000
*/
private String province;
/**
* 城市
*
示例值:MDCY00006000
*/
private String city;
/**
* 地址
*
示例值:地址
*/
private String address;
/**
* 纳税人类型
*
示例值:1
*/
private String taxpayerType;
/**
* 联系电话
*
示例值:010-58341796
*/
private String telephone;
/**
* 银行内部Id
*
示例值:MDBK00072319
*/
private String bankId;
/**
* 开户银行名称
*
示例值:中原银行股份有限公司南阳华瑞支行
*/
private String bankName;
/**
* 开户行账号
*
示例值:644666446
*/
private String bankAccount;
/**
* 状态
*
示例值:1
*/
private Integer status;
/**
* 银行账户列表
*
示例值:
*/
private LegalEntityBank[] legalEntityBanks;
/**
* 扩展字段相关信息列表
*
示例值:
*/
private ExtendField[] extendInfo;
/**
* 附件列表
*
示例值:
*/
private Appendix[] appendix;
/**
* 法人实体id
*
示例值:7003410079584092448
*
* @param id
* @return
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* 法人实体编码(根据配置会有不同的生成规则)
*
示例值:L00002002
*
* @param legalEntity
* @return
*/
public Builder legalEntity(String legalEntity) {
this.legalEntity = legalEntity;
return this;
}
/**
* 法人实体名称
*
示例值:法人22
*
* @param legalEntityText
* @return
*/
public Builder legalEntityText(String legalEntityText) {
this.legalEntityText = legalEntityText;
return this;
}
/**
* 法人实体英文名称
*
示例值:legal_person
*
* @param shortText
* @return
*/
public Builder shortText(String shortText) {
this.shortText = shortText;
return this;
}
/**
* 证件类型
*
示例值:0
*
* @param certificationType
* @return
*/
public Builder certificationType(String certificationType) {
this.certificationType = certificationType;
return this;
}
/**
* 证件类型
*
示例值:0
*
* @param certificationType {@link com.lark.oapi.service.mdm.v1.enums.LegalEntityCertificationTypeEnum}
* @return
*/
public Builder certificationType(com.lark.oapi.service.mdm.v1.enums.LegalEntityCertificationTypeEnum certificationType) {
this.certificationType = certificationType.getValue();
return this;
}
/**
* 证件id
*
示例值:91310120MA1H23N81AX
*
* @param certificationId
* @return
*/
public Builder certificationId(String certificationId) {
this.certificationId = certificationId;
return this;
}
/**
* 法人
*
示例值:张三
*
* @param legalPerson
* @return
*/
public Builder legalPerson(String legalPerson) {
this.legalPerson = legalPerson;
return this;
}
/**
* 国家
*
示例值:CN
*
* @param country
* @return
*/
public Builder country(String country) {
this.country = country;
return this;
}
/**
* 省份
*
示例值:MDPS00004000
*
* @param province
* @return
*/
public Builder province(String province) {
this.province = province;
return this;
}
/**
* 城市
*
示例值:MDCY00006000
*
* @param city
* @return
*/
public Builder city(String city) {
this.city = city;
return this;
}
/**
* 地址
*
示例值:地址
*
* @param address
* @return
*/
public Builder address(String address) {
this.address = address;
return this;
}
/**
* 纳税人类型
*
示例值:1
*
* @param taxpayerType
* @return
*/
public Builder taxpayerType(String taxpayerType) {
this.taxpayerType = taxpayerType;
return this;
}
/**
* 纳税人类型
*
示例值:1
*
* @param taxpayerType {@link com.lark.oapi.service.mdm.v1.enums.LegalEntityTaxpayerTypeEnum}
* @return
*/
public Builder taxpayerType(com.lark.oapi.service.mdm.v1.enums.LegalEntityTaxpayerTypeEnum taxpayerType) {
this.taxpayerType = taxpayerType.getValue();
return this;
}
/**
* 联系电话
*
示例值:010-58341796
*
* @param telephone
* @return
*/
public Builder telephone(String telephone) {
this.telephone = telephone;
return this;
}
/**
* 银行内部Id
*
示例值:MDBK00072319
*
* @param bankId
* @return
*/
public Builder bankId(String bankId) {
this.bankId = bankId;
return this;
}
/**
* 开户银行名称
*
示例值:中原银行股份有限公司南阳华瑞支行
*
* @param bankName
* @return
*/
public Builder bankName(String bankName) {
this.bankName = bankName;
return this;
}
/**
* 开户行账号
*
示例值:644666446
*
* @param bankAccount
* @return
*/
public Builder bankAccount(String bankAccount) {
this.bankAccount = bankAccount;
return this;
}
/**
* 状态
*
示例值:1
*
* @param status
* @return
*/
public Builder status(Integer status) {
this.status = status;
return this;
}
/**
* 状态
*
示例值:1
*
* @param status {@link com.lark.oapi.service.mdm.v1.enums.LegalEntityStatusEnum}
* @return
*/
public Builder status(com.lark.oapi.service.mdm.v1.enums.LegalEntityStatusEnum status) {
this.status = status.getValue();
return this;
}
/**
* 银行账户列表
*
示例值:
*
* @param legalEntityBanks
* @return
*/
public Builder legalEntityBanks(LegalEntityBank[] legalEntityBanks) {
this.legalEntityBanks = legalEntityBanks;
return this;
}
/**
* 扩展字段相关信息列表
*
示例值:
*
* @param extendInfo
* @return
*/
public Builder extendInfo(ExtendField[] extendInfo) {
this.extendInfo = extendInfo;
return this;
}
/**
* 附件列表
*
示例值:
*
* @param appendix
* @return
*/
public Builder appendix(Appendix[] appendix) {
this.appendix = appendix;
return this;
}
public LegalEntity build() {
return new LegalEntity(this);
}
}
}