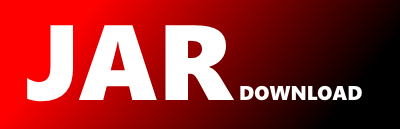
com.lark.oapi.service.mdm.v1.model.LegalEntityBank Maven / Gradle / Ivy
Show all versions of oapi-sdk Show documentation
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.mdm.v1.model;
import com.lark.oapi.core.response.EmptyData;
import com.lark.oapi.service.mdm.v1.enums.*;
import com.google.gson.annotations.SerializedName;
import com.google.gson.annotations.SerializedName;
import com.lark.oapi.core.annotation.Body;
import com.lark.oapi.core.annotation.Path;
import com.lark.oapi.core.annotation.Query;
import java.io.ByteArrayOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import com.lark.oapi.core.utils.Strings;
import com.lark.oapi.core.response.BaseResponse;
public class LegalEntityBank {
/**
* 法人实体银行账户id
* 示例值:1433492736852541442
*/
@SerializedName("id")
private String id;
/**
* 公司编码
*
示例值:1002
*/
@SerializedName("company_code")
private String companyCode;
/**
* 银行Id
*
示例值:MDBK00131739
*/
@SerializedName("bank_id")
private String bankId;
/**
* 银联号
*
示例值:001755053005
*/
@SerializedName("bank_code")
private String bankCode;
/**
* 银行名称
*
示例值:中国人民银行丽江市中心支行
*/
@SerializedName("bank_name")
private String bankName;
/**
* 总行英文缩写
*
示例值:PBC
*/
@SerializedName("bank_acronym")
private String bankAcronym;
/**
* 国家
*
示例值:CN
*/
@SerializedName("country")
private String country;
/**
* 账户名称
*
示例值:账户名称
*/
@SerializedName("account_name")
private String accountName;
/**
* 银行账号
*
示例值:122907287xxxxx9
*/
@SerializedName("bank_account")
private String bankAccount;
/**
* 银行SWIFT编码
*
示例值:CMBCCNBS
*/
@SerializedName("swift_code")
private String swiftCode;
/**
* 银行控制码
*
示例值:40001xxxxxxx00313261
*/
@SerializedName("bank_control_code")
private String bankControlCode;
/**
* 扩展字段相关信息列表
*
示例值:
*/
@SerializedName("extend_info")
private ExtendField[] extendInfo;
/**
* IBAN账号
*
示例值:6446777
*/
@SerializedName("iban_account")
private String ibanAccount;
/**
* 币种
*
示例值:RMB
*/
@SerializedName("currency")
private String currency;
/**
* 总账科目编码
*
示例值:123ASD
*/
@SerializedName("gl_account")
private String glAccount;
/**
* 清算科目编码
*
示例值:ASD123
*/
@SerializedName("clearing_account")
private String clearingAccount;
/**
* 账户属性描述
*
示例值:QWE123
*/
@SerializedName("account_attribute_desc")
private String accountAttributeDesc;
// builder 开始
public LegalEntityBank() {
}
public LegalEntityBank(Builder builder) {
/**
* 法人实体银行账户id
*
示例值:1433492736852541442
*/
this.id = builder.id;
/**
* 公司编码
*
示例值:1002
*/
this.companyCode = builder.companyCode;
/**
* 银行Id
*
示例值:MDBK00131739
*/
this.bankId = builder.bankId;
/**
* 银联号
*
示例值:001755053005
*/
this.bankCode = builder.bankCode;
/**
* 银行名称
*
示例值:中国人民银行丽江市中心支行
*/
this.bankName = builder.bankName;
/**
* 总行英文缩写
*
示例值:PBC
*/
this.bankAcronym = builder.bankAcronym;
/**
* 国家
*
示例值:CN
*/
this.country = builder.country;
/**
* 账户名称
*
示例值:账户名称
*/
this.accountName = builder.accountName;
/**
* 银行账号
*
示例值:122907287xxxxx9
*/
this.bankAccount = builder.bankAccount;
/**
* 银行SWIFT编码
*
示例值:CMBCCNBS
*/
this.swiftCode = builder.swiftCode;
/**
* 银行控制码
*
示例值:40001xxxxxxx00313261
*/
this.bankControlCode = builder.bankControlCode;
/**
* 扩展字段相关信息列表
*
示例值:
*/
this.extendInfo = builder.extendInfo;
/**
* IBAN账号
*
示例值:6446777
*/
this.ibanAccount = builder.ibanAccount;
/**
* 币种
*
示例值:RMB
*/
this.currency = builder.currency;
/**
* 总账科目编码
*
示例值:123ASD
*/
this.glAccount = builder.glAccount;
/**
* 清算科目编码
*
示例值:ASD123
*/
this.clearingAccount = builder.clearingAccount;
/**
* 账户属性描述
*
示例值:QWE123
*/
this.accountAttributeDesc = builder.accountAttributeDesc;
}
public static Builder newBuilder() {
return new Builder();
}
public String getId() {
return this.id;
}
public void setId(String id) {
this.id = id;
}
public String getCompanyCode() {
return this.companyCode;
}
public void setCompanyCode(String companyCode) {
this.companyCode = companyCode;
}
public String getBankId() {
return this.bankId;
}
public void setBankId(String bankId) {
this.bankId = bankId;
}
public String getBankCode() {
return this.bankCode;
}
public void setBankCode(String bankCode) {
this.bankCode = bankCode;
}
public String getBankName() {
return this.bankName;
}
public void setBankName(String bankName) {
this.bankName = bankName;
}
public String getBankAcronym() {
return this.bankAcronym;
}
public void setBankAcronym(String bankAcronym) {
this.bankAcronym = bankAcronym;
}
public String getCountry() {
return this.country;
}
public void setCountry(String country) {
this.country = country;
}
public String getAccountName() {
return this.accountName;
}
public void setAccountName(String accountName) {
this.accountName = accountName;
}
public String getBankAccount() {
return this.bankAccount;
}
public void setBankAccount(String bankAccount) {
this.bankAccount = bankAccount;
}
public String getSwiftCode() {
return this.swiftCode;
}
public void setSwiftCode(String swiftCode) {
this.swiftCode = swiftCode;
}
public String getBankControlCode() {
return this.bankControlCode;
}
public void setBankControlCode(String bankControlCode) {
this.bankControlCode = bankControlCode;
}
public ExtendField[] getExtendInfo() {
return this.extendInfo;
}
public void setExtendInfo(ExtendField[] extendInfo) {
this.extendInfo = extendInfo;
}
public String getIbanAccount() {
return this.ibanAccount;
}
public void setIbanAccount(String ibanAccount) {
this.ibanAccount = ibanAccount;
}
public String getCurrency() {
return this.currency;
}
public void setCurrency(String currency) {
this.currency = currency;
}
public String getGlAccount() {
return this.glAccount;
}
public void setGlAccount(String glAccount) {
this.glAccount = glAccount;
}
public String getClearingAccount() {
return this.clearingAccount;
}
public void setClearingAccount(String clearingAccount) {
this.clearingAccount = clearingAccount;
}
public String getAccountAttributeDesc() {
return this.accountAttributeDesc;
}
public void setAccountAttributeDesc(String accountAttributeDesc) {
this.accountAttributeDesc = accountAttributeDesc;
}
public static class Builder {
/**
* 法人实体银行账户id
*
示例值:1433492736852541442
*/
private String id;
/**
* 公司编码
*
示例值:1002
*/
private String companyCode;
/**
* 银行Id
*
示例值:MDBK00131739
*/
private String bankId;
/**
* 银联号
*
示例值:001755053005
*/
private String bankCode;
/**
* 银行名称
*
示例值:中国人民银行丽江市中心支行
*/
private String bankName;
/**
* 总行英文缩写
*
示例值:PBC
*/
private String bankAcronym;
/**
* 国家
*
示例值:CN
*/
private String country;
/**
* 账户名称
*
示例值:账户名称
*/
private String accountName;
/**
* 银行账号
*
示例值:122907287xxxxx9
*/
private String bankAccount;
/**
* 银行SWIFT编码
*
示例值:CMBCCNBS
*/
private String swiftCode;
/**
* 银行控制码
*
示例值:40001xxxxxxx00313261
*/
private String bankControlCode;
/**
* 扩展字段相关信息列表
*
示例值:
*/
private ExtendField[] extendInfo;
/**
* IBAN账号
*
示例值:6446777
*/
private String ibanAccount;
/**
* 币种
*
示例值:RMB
*/
private String currency;
/**
* 总账科目编码
*
示例值:123ASD
*/
private String glAccount;
/**
* 清算科目编码
*
示例值:ASD123
*/
private String clearingAccount;
/**
* 账户属性描述
*
示例值:QWE123
*/
private String accountAttributeDesc;
/**
* 法人实体银行账户id
*
示例值:1433492736852541442
*
* @param id
* @return
*/
public Builder id(String id) {
this.id = id;
return this;
}
/**
* 公司编码
*
示例值:1002
*
* @param companyCode
* @return
*/
public Builder companyCode(String companyCode) {
this.companyCode = companyCode;
return this;
}
/**
* 银行Id
*
示例值:MDBK00131739
*
* @param bankId
* @return
*/
public Builder bankId(String bankId) {
this.bankId = bankId;
return this;
}
/**
* 银联号
*
示例值:001755053005
*
* @param bankCode
* @return
*/
public Builder bankCode(String bankCode) {
this.bankCode = bankCode;
return this;
}
/**
* 银行名称
*
示例值:中国人民银行丽江市中心支行
*
* @param bankName
* @return
*/
public Builder bankName(String bankName) {
this.bankName = bankName;
return this;
}
/**
* 总行英文缩写
*
示例值:PBC
*
* @param bankAcronym
* @return
*/
public Builder bankAcronym(String bankAcronym) {
this.bankAcronym = bankAcronym;
return this;
}
/**
* 国家
*
示例值:CN
*
* @param country
* @return
*/
public Builder country(String country) {
this.country = country;
return this;
}
/**
* 账户名称
*
示例值:账户名称
*
* @param accountName
* @return
*/
public Builder accountName(String accountName) {
this.accountName = accountName;
return this;
}
/**
* 银行账号
*
示例值:122907287xxxxx9
*
* @param bankAccount
* @return
*/
public Builder bankAccount(String bankAccount) {
this.bankAccount = bankAccount;
return this;
}
/**
* 银行SWIFT编码
*
示例值:CMBCCNBS
*
* @param swiftCode
* @return
*/
public Builder swiftCode(String swiftCode) {
this.swiftCode = swiftCode;
return this;
}
/**
* 银行控制码
*
示例值:40001xxxxxxx00313261
*
* @param bankControlCode
* @return
*/
public Builder bankControlCode(String bankControlCode) {
this.bankControlCode = bankControlCode;
return this;
}
/**
* 扩展字段相关信息列表
*
示例值:
*
* @param extendInfo
* @return
*/
public Builder extendInfo(ExtendField[] extendInfo) {
this.extendInfo = extendInfo;
return this;
}
/**
* IBAN账号
*
示例值:6446777
*
* @param ibanAccount
* @return
*/
public Builder ibanAccount(String ibanAccount) {
this.ibanAccount = ibanAccount;
return this;
}
/**
* 币种
*
示例值:RMB
*
* @param currency
* @return
*/
public Builder currency(String currency) {
this.currency = currency;
return this;
}
/**
* 总账科目编码
*
示例值:123ASD
*
* @param glAccount
* @return
*/
public Builder glAccount(String glAccount) {
this.glAccount = glAccount;
return this;
}
/**
* 清算科目编码
*
示例值:ASD123
*
* @param clearingAccount
* @return
*/
public Builder clearingAccount(String clearingAccount) {
this.clearingAccount = clearingAccount;
return this;
}
/**
* 账户属性描述
*
示例值:QWE123
*
* @param accountAttributeDesc
* @return
*/
public Builder accountAttributeDesc(String accountAttributeDesc) {
this.accountAttributeDesc = accountAttributeDesc;
return this;
}
public LegalEntityBank build() {
return new LegalEntityBank(this);
}
}
}