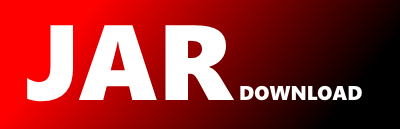
com.lark.oapi.service.vc.v1.V1 Maven / Gradle / Ivy
// Code generated by lark suite oapi sdk gen
/*
* MIT License
*
* Copyright (c) 2022 Lark Technologies Pte. Ltd.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice, shall be included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.lark.oapi.service.vc.v1;
import com.lark.oapi.core.Config;
import com.lark.oapi.service.vc.v1.resource.*;
public class V1 {
private final Alert alert; // 告警中心
private final Export export; // 导出
private final Meeting meeting; // 会议
private final MeetingRecording meetingRecording; // 录制
private final MeetingList meetingList; // meeting_list
private final ParticipantList participantList; // participant_list
private final ParticipantQualityList participantQualityList; // participant_quality_list
private final Report report; // 会议报告
private final Reserve reserve; // 预约
private final ReserveConfig reserveConfig; // reserve_config
private final ReserveConfigAdmin reserveConfigAdmin; // reserve_config.admin
private final ReserveConfigDisableInform reserveConfigDisableInform; // reserve_config.disable_inform
private final ReserveConfigForm reserveConfigForm; // reserve_config.form
private final ResourceReservationList resourceReservationList; // resource_reservation_list
private final Room room; // 会议室
private final RoomConfig roomConfig; // room_config
private final RoomLevel roomLevel; // 会议室层级
private final ScopeConfig scopeConfig; // 会议室配置
public V1(Config config) {
this.alert = new Alert(config);
this.export = new Export(config);
this.meeting = new Meeting(config);
this.meetingRecording = new MeetingRecording(config);
this.meetingList = new MeetingList(config);
this.participantList = new ParticipantList(config);
this.participantQualityList = new ParticipantQualityList(config);
this.report = new Report(config);
this.reserve = new Reserve(config);
this.reserveConfig = new ReserveConfig(config);
this.reserveConfigAdmin = new ReserveConfigAdmin(config);
this.reserveConfigDisableInform = new ReserveConfigDisableInform(config);
this.reserveConfigForm = new ReserveConfigForm(config);
this.resourceReservationList = new ResourceReservationList(config);
this.room = new Room(config);
this.roomConfig = new RoomConfig(config);
this.roomLevel = new RoomLevel(config);
this.scopeConfig = new ScopeConfig(config);
}
public Alert alert() {
return alert;
}
public Export export() {
return export;
}
public Meeting meeting() {
return meeting;
}
public MeetingRecording meetingRecording() {
return meetingRecording;
}
public MeetingList meetingList() {
return meetingList;
}
public ParticipantList participantList() {
return participantList;
}
public ParticipantQualityList participantQualityList() {
return participantQualityList;
}
public Report report() {
return report;
}
public Reserve reserve() {
return reserve;
}
public ReserveConfig reserveConfig() {
return reserveConfig;
}
public ReserveConfigAdmin reserveConfigAdmin() {
return reserveConfigAdmin;
}
public ReserveConfigDisableInform reserveConfigDisableInform() {
return reserveConfigDisableInform;
}
public ReserveConfigForm reserveConfigForm() {
return reserveConfigForm;
}
public ResourceReservationList resourceReservationList() {
return resourceReservationList;
}
public Room room() {
return room;
}
public RoomConfig roomConfig() {
return roomConfig;
}
public RoomLevel roomLevel() {
return roomLevel;
}
public ScopeConfig scopeConfig() {
return scopeConfig;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy