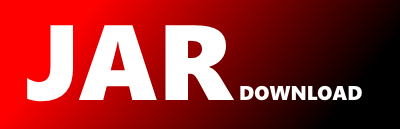
com.launchableinc.openai.completion.chat.ChatMessage Maven / Gradle / Ivy
Show all versions of api Show documentation
package com.launchableinc.openai.completion.chat;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.*;
/**
* Each object has a role (either "system", "user", or "assistant") and content (the content of
* the message). Conversations can be as short as 1 message or fill many pages.
* Typically, a conversation is formatted with a system message first, followed by alternating
* user and assistant messages.
* The system message helps set the behavior of the assistant. In the example above, the
* assistant was instructed with "You are a helpful assistant."
The user messages help instruct
* the assistant. They can be generated by the end users of an application, or set by a developer as
* an instruction.
The assistant messages help store prior responses. They can also be written
* by a developer to help give examples of desired behavior.
*
*
* see OpenAi documentation
*/
@Data
@NoArgsConstructor(force = true)
@RequiredArgsConstructor
@AllArgsConstructor
public class ChatMessage {
/**
* Must be either 'system', 'user', 'assistant' or 'function'.
You may use
* {@link ChatMessageRole} enum.
*/
@NonNull
String role;
@JsonInclude() // content should always exist in the call, even if it is null
String content;
//name is optional, The name of the author of this message. May contain a-z, A-Z, 0-9, and underscores, with a maximum length of 64 characters.
String name;
@JsonProperty("function_call")
ChatFunctionCall functionCall;
public ChatMessage(String role, String content) {
this.role = role;
this.content = content;
}
public ChatMessage(String role, String content, String name) {
this.role = role;
this.content = content;
this.name = name;
}
}