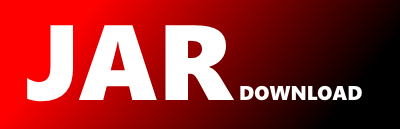
com.launchdarkly.client.FeatureStore Maven / Gradle / Ivy
Show all versions of launchdarkly-client Show documentation
package com.launchdarkly.client;
import java.io.Closeable;
import java.util.Map;
/**
* A thread-safe, versioned store for feature flags and related objects received from the
* streaming API. Implementations should permit concurrent access and updates.
*
* Delete and upsert requests are versioned-- if the version number in the request is less than
* the currently stored version of the object, the request should be ignored.
*
* These semantics support the primary use case for the store, which synchronizes a collection
* of objects based on update messages that may be received out-of-order.
* @since 3.0.0
*/
public interface FeatureStore extends Closeable {
/**
* Returns the object to which the specified key is mapped, or
* null if the key is not associated or the associated object has
* been deleted.
*
* @param class of the object that will be returned
* @param kind the kind of object to get
* @param key the key whose associated object is to be returned
* @return the object to which the specified key is mapped, or
* null if the key is not associated or the associated object has
* been deleted.
*/
T get(VersionedDataKind kind, String key);
/**
* Returns a {@link java.util.Map} of all associated objects of a given kind.
*
* @param class of the objects that will be returned in the map
* @param kind the kind of objects to get
* @return a map of all associated object.
*/
Map all(VersionedDataKind kind);
/**
* Initializes (or re-initializes) the store with the specified set of objects. Any existing entries
* will be removed. Implementations can assume that this set of objects is up to date-- there is no
* need to perform individual version comparisons between the existing objects and the supplied
* features.
*
* @param allData all objects to be stored
*/
void init(Map, Map> allData);
/**
* Deletes the object associated with the specified key, if it exists and its version
* is less than or equal to the specified version.
*
* @param class of the object to be deleted
* @param kind the kind of object to delete
* @param key the key of the object to be deleted
* @param version the version for the delete operation
*/
void delete(VersionedDataKind kind, String key, int version);
/**
* Update or insert the object associated with the specified key, if its version
* is less than or equal to the version specified in the argument object.
*
* @param class of the object to be updated
* @param kind the kind of object to update
* @param item the object to update or insert
*/
void upsert(VersionedDataKind kind, T item);
/**
* Returns true if this store has been initialized.
*
* @return true if this store has been initialized
*/
boolean initialized();
}