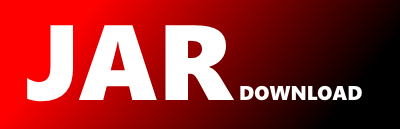
com.launchdarkly.client.Segment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of launchdarkly-client Show documentation
Show all versions of launchdarkly-client Show documentation
Official LaunchDarkly SDK for Java
package com.launchdarkly.client;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import com.google.gson.reflect.TypeToken;
class Segment implements VersionedData {
private static final Type mapType = new TypeToken
© 2015 - 2024 Weber Informatics LLC | Privacy Policy