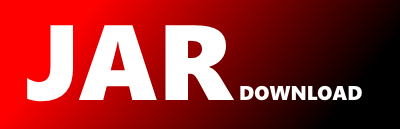
com.launchdarkly.sdk.LDValueObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of launchdarkly-java-sdk-common Show documentation
Show all versions of launchdarkly-java-sdk-common Show documentation
LaunchDarkly SDK Java Common Classes
package com.launchdarkly.sdk;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.util.Collections;
import java.util.Map;
@JsonAdapter(LDValueTypeAdapter.class)
final class LDValueObject extends LDValue {
private static final LDValueObject EMPTY = new LDValueObject(Collections.emptyMap());
private final Map map;
static LDValueObject fromMap(Map map) {
return map.isEmpty() ? EMPTY : new LDValueObject(map);
}
private LDValueObject(Map map) {
this.map = map;
}
public LDValueType getType() {
return LDValueType.OBJECT;
}
@Override
public int size() {
return map.size();
}
@Override
public Iterable keys() {
return map.keySet();
}
@Override
public Iterable values() {
return map.values();
}
@Override
public LDValue get(String name) {
LDValue v = map.get(name);
return v == null ? ofNull() : v;
}
@Override
void write(JsonWriter writer) throws IOException {
writer.beginObject();
for (Map.Entry e: map.entrySet()) {
writer.name(e.getKey());
e.getValue().write(writer);
}
writer.endObject();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy