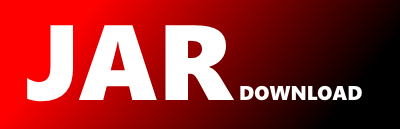
com.launchdarkly.sdk.server.migrations.MigrationBuilder Maven / Gradle / Ivy
Show all versions of launchdarkly-java-server-sdk Show documentation
package com.launchdarkly.sdk.server.migrations;
import com.launchdarkly.sdk.server.interfaces.LDClientInterface;
import org.jetbrains.annotations.NotNull;
import java.util.Optional;
/**
* This builder is used to construct {@link Migration} instances.
*
* This class is not thread-safe. The builder should be used on one thread and then the
* built {@link Migration} is thread safe.
*
* @param The result type for reads.
* @param The result type for writes.
* @param The input parameter type for reads.
* @param The input type for writes.
*/
public class MigrationBuilder {
private Migration.Reader readOld;
private Migration.Reader readNew;
private Migration.Writer writeOld;
private Migration.Writer writeNew;
private Migration.ReadConsistencyChecker checker;
private MigrationExecution execution = MigrationExecution.Parallel();
private boolean latencyTracking = true;
private boolean errorTracking = true;
private final LDClientInterface client;
/**
* Construct a new builder.
*
* @param client this client will be used for {@link Migration}s built from
* this builder
*/
public MigrationBuilder(LDClientInterface client) {
this.client = client;
}
/**
* Enable or disable latency tracking. Tracking is enabled by default.
*
* @param track true to enable tracking, false to disable it
* @return a reference to this builder
*/
@NotNull
public MigrationBuilder trackLatency(boolean track) {
this.latencyTracking = track;
return this;
}
/**
* Enable or disable error tracking. Tracking is enabled by default.
*
* @param track true to enable error tracking, false to disable it
* @return a reference to this builder
*/
@NotNull
public MigrationBuilder trackErrors(boolean track) {
this.errorTracking = track;
return this;
}
/**
* Influences the level of concurrency when the migration stage calls for multiple execution reads.
*
* The default read execution is {@link MigrationExecution#Parallel()}.
*
* Setting the execution to randomized serial order.
*
* builder.readExecution(MigrationExecution.Serial(MigrationSerialOrder.RANDOM));
*
*
* @param execution the execution configuration
* @return a reference to this builder
*/
@NotNull
public MigrationBuilder readExecution(MigrationExecution execution) {
this.execution = execution;
return this;
}
/**
* Configure the read methods of the migration.
*
* Users are required to provide two different read methods -- one to read from the old migration source, and one to
* read from the new source. This method allows specifying a check method for consistency tracking.
*
* If you do not want consistency tracking, then use
* {@link MigrationBuilder#read(Migration.Reader, Migration.Reader)}.
*
* @param oldImpl method for reading from the "old" migration source
* @param newImpl method for reading from the "new" migration source
* @param checker method which checks the consistency of the "old" and "new" source
* @return a reference to this builder
*/
@NotNull
public MigrationBuilder read(
@NotNull Migration.Reader oldImpl,
@NotNull Migration.Reader newImpl,
@NotNull Migration.ReadConsistencyChecker checker
) {
this.readOld = oldImpl;
this.readNew = newImpl;
this.checker = checker;
return this;
}
/**
* Configure the read methods of the migration.
*
* Users are required to provide two different read methods -- one to read from the old migration source, and one to
* read from the new source. This method does not enable consistency tracking.
*
* If you do want consistency tracking, then use
* {@link MigrationBuilder#read(Migration.Reader, Migration.Reader, Migration.ReadConsistencyChecker)}.
*
* @param oldImpl method for reading from the "old" migration source
* @param newImpl method for reading from the "new" migration source
* @return a reference to this builder
*/
@NotNull
public MigrationBuilder read(
@NotNull Migration.Reader oldImpl,
@NotNull Migration.Reader newImpl
) {
this.readOld = oldImpl;
this.readNew = newImpl;
return this;
}
/**
* Configure the write methods of the migration.
*
* Users are required to provide two different write methods -- one to write to the old migration source, and one to
* write to the new source. Not every stage requires
*
* @param oldImpl method which writes to the "old" source
* @param newImpl method which writes to the "new" source
* @return a reference to this builder
*/
@NotNull
public MigrationBuilder write(
@NotNull Migration.Writer oldImpl,
@NotNull Migration.Writer newImpl
) {
this.writeOld = oldImpl;
this.writeNew = newImpl;
return this;
}
/**
* Build a {@link Migration}.
*
* A migration requires that both the read and write methods are defined. If they have not been defined, then
* a migration cannot be constructed. In this case an empty optional will be returned.
*
* @return Either an empty optional or an optional containing a {@link Migration}.
*/
@NotNull
public Optional> build() {
// All the methods must be set to make a valid migration.
if (
readNew == null ||
readOld == null ||
writeNew == null ||
writeOld == null
) {
// TODO: Log something.
return Optional.empty();
}
return Optional.of(new Migration<>(
client, readOld, readNew, writeOld, writeNew,
checker, execution, latencyTracking, errorTracking
));
}
}