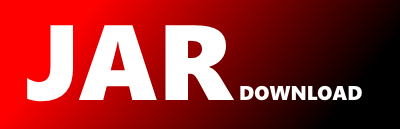
com.lazerycode.selenium.repository.DriverMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of driver-binary-downloader-maven-plugin Show documentation
Show all versions of driver-binary-downloader-maven-plugin Show documentation
A plugin to automatically download individual selenium standalone binaries (e.g. chromedriver.exe) for your mavenised selenium project.
package com.lazerycode.selenium.repository;
import java.util.*;
import static com.lazerycode.selenium.repository.OperatingSystem.getCurrentOperatingSystem;
import static com.lazerycode.selenium.repository.SystemArchitecture.getCurrentSystemArcitecture;
public class DriverMap {
protected HashMap> repository = new HashMap>();
public TreeMap getMapForDriverContext(DriverContext driverContext) {
if (!repository.containsKey(driverContext)) {
repository.put(driverContext, new TreeMap());
}
return repository.get(driverContext);
}
public DriverDetails getDetailsForVersionOfDriverContext(DriverContext driverContext, String version) throws IllegalArgumentException {
if (!repository.containsKey(driverContext)) {
throw new IllegalArgumentException("Driver context not found in driver repository");
}
TreeMap driverVersions = repository.get(driverContext);
DriverDetails detailsToReturn = driverVersions.get(driverVersions.lastKey());
if (detailsToReturn.hashCode() == 0) {
throw new NoSuchElementException("No driver version " + version + " exists for the context " + driverContext.toString());
}
return detailsToReturn;
}
public DriverDetails getDetailsForLatestVersionOfDriverContext(DriverContext driverContext) {
if (!repository.containsKey(driverContext)) {
throw new IllegalArgumentException("Driver context not found in driver repository");
}
TreeMap driverVersions = repository.get(driverContext);
return driverVersions.get(driverVersions.lastKey());
}
public Set getKeys() {
return repository.keySet();
}
public Set getAvailableVersionsForDriverContext(DriverContext driverContext) {
return repository.get(driverContext).keySet();
}
public ArrayList getDriverContextsForOperatingSystem(OperatingSystem operatingSystem, SystemArchitecture systemArchitecture) {
ArrayList matchingContexts = new ArrayList();
for (DriverContext driverContext : repository.keySet()) {
if (driverContext.getOperatingSystem().equals(operatingSystem) && driverContext.getSystemArchitecture().equals(systemArchitecture)) {
matchingContexts.add(driverContext);
}
}
return matchingContexts;
}
public ArrayList getDriverContextsForCurrentOperatingSystem() {
OperatingSystem operatingSystem = getCurrentOperatingSystem();
SystemArchitecture systemArchitecture = getCurrentSystemArcitecture();
return getDriverContextsForOperatingSystem(operatingSystem, systemArchitecture);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy