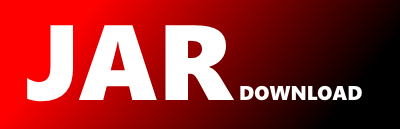
com.leacox.motif.cases.OptionalCases Maven / Gradle / Ivy
// /*
// * Copyright (C) 2015 John Leacox
// *
// * Licensed under the Apache License, Version 2.0 (the "License");
// * you may not use this file except in compliance with the License.
// * You may obtain a copy of the License at
// *
// * http://www.apache.org/licenses/LICENSE-2.0
// *
// * Unless required by applicable law or agreed to in writing, software
// * distributed under the License is distributed on an "AS IS" BASIS,
// * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// * See the License for the specific language governing permissions and
// * limitations under the License.
// */
//
// Generated by Motif. Do Not Edit!
//
package com.leacox.motif.cases;
import com.leacox.motif.MatchesAny;
import com.leacox.motif.MatchesExact;
import com.leacox.motif.extract.DecomposableMatchBuilder0;
import com.leacox.motif.extract.DecomposableMatchBuilder1;
import com.leacox.motif.extract.DecomposableMatchBuilder2;
import com.leacox.motif.extract.DecomposableMatchBuilder3;
import com.leacox.motif.extract.matchers.ArgumentMatchers;
import com.leacox.motif.extract.matchers.Matcher;
import com.leacox.motif.extract.util.Lists;
import java.util.List;
import java.util.Optional;
/**
* Motif cases for matching an {@link Optional}.
*/
public final class OptionalCases {
private OptionalCases() {
}
/**
* Matches an empty {@link Optional}.
*/
public static DecomposableMatchBuilder0> none() {
List> matchers = Lists.of();
return new DecomposableMatchBuilder0>(matchers, new OptionalNoneFieldExtractor<>());
}
/**
* Matches a non-empty {@link Optional}.
*/
public static DecomposableMatchBuilder0> some(MatchesExact t) {
List> matchers = Lists.of(ArgumentMatchers.eq(t.t));
return new DecomposableMatchBuilder0>(matchers, new OptionalFieldExtractor<>());
}
/**
* Matches a non-empty {@link Optional}.
*
* If matched, the {@code t} value is extracted.
*/
public static DecomposableMatchBuilder1, T> some(MatchesAny t) {
List> matchers = Lists.of(ArgumentMatchers.any());
return new DecomposableMatchBuilder1, T>(
matchers, 0, new OptionalFieldExtractor<>());
}
/**
* Matches a non-empty {@link Optional}.
*
* If matched, the {@code t} value is decomposed to 0.
*/
public static DecomposableMatchBuilder0> some(
DecomposableMatchBuilder0 t) {
List> matchers = Lists.of(ArgumentMatchers.any());
return new DecomposableMatchBuilder1, ET>(
matchers, 0, new OptionalFieldExtractor<>()).decomposeFirst(t);
}
/**
* Matches a non-empty {@link Optional}.
*
* If matched, the {@code t} value is decomposed to 1.
*/
public static DecomposableMatchBuilder1, A1> some(
DecomposableMatchBuilder1 t) {
List> matchers = Lists.of(ArgumentMatchers.any());
return new DecomposableMatchBuilder1, ET>(
matchers, 0, new OptionalFieldExtractor<>()).decomposeFirst(t);
}
/**
* Matches a non-empty {@link Optional}.
*
* If matched, the {@code t} value is decomposed to 2.
*/
public static DecomposableMatchBuilder2, A1, A2> some(
DecomposableMatchBuilder2 t) {
List> matchers = Lists.of(ArgumentMatchers.any());
return new DecomposableMatchBuilder1, ET>(
matchers, 0, new OptionalFieldExtractor<>()).decomposeFirst(t);
}
/**
* Matches a non-empty {@link Optional}.
*
* If matched, the {@code t} value is decomposed to 3.
*/
public static DecomposableMatchBuilder3, A1, A2, A3> some(
DecomposableMatchBuilder3 t) {
List> matchers = Lists.of(ArgumentMatchers.any());
return new DecomposableMatchBuilder1, ET>(
matchers, 0, new OptionalFieldExtractor<>()).decomposeFirst(t);
}
}