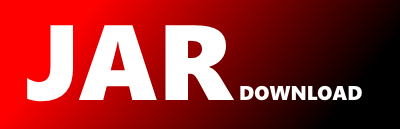
com.leftins.tools.ListCom.CollectionHelper Maven / Gradle / Ivy
package com.leftins.tools.ListCom;
import com.leftins.tools.tools.ComUtil;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
/**
* @author zhaojiwei YGCollectionHelper 2015年4月16日
*/
public class CollectionHelper {
public static T first(Collection list) {
if (ComUtil.isEmpty(list)) return null;
int i = 0;
for (T t : list) {
if (i++ == 0) {
return t;
}
}
return null;
}
public static boolean exists(Collection list, IMatch collectionInterface) {
if (ComUtil.isEmpty(list)) {
return false;
}
for (T t : list) {
if (collectionInterface.match(t)) {
return true;
}
}
return false;
}
public static boolean all(Collection list, IMatch collectionInterface) {
if (ComUtil.isEmpty(list)) {
return false;
}
for (T t : list) {
if (!collectionInterface.match(t)) {
return false;
}
}
return true;
}
public static boolean any(Collection list, IMatch collectionInterface) {
return exists(list, collectionInterface);
}
public static T find(Collection list, IMatch collectionInterface) {
if (ComUtil.isEmpty(list)) {
return null;
}
for (T t : list) {
if (collectionInterface.match(t)) {
return t;
}
}
return null;
}
public static List findAll(Collection list, IMatch collectionInterface) {
if (ComUtil.isEmpty(list)) {
return null;
}
List ts = new ArrayList<>();
for (T t : list) {
if (t != null && collectionInterface.match(t)) {
ts.add(t);
}
}
return ts;
}
public static List select(Collection list, ISingleMapping singleMappingInterface) {
if (ComUtil.isEmpty(list)) {
return null;
}
List result = new ArrayList<>();
for (T t : list) {
E e = singleMappingInterface.func(t);
if (e != null) {
result.add(e);
}
}
return result;
}
public static List selectMany(Collection list, IManyMapping manyMapping) {
if (ComUtil.isEmpty(list)) {
return null;
}
List result = new ArrayList<>();
Collection e;
for (T t : list) {
e = manyMapping.selectMany(t);
if (e != null) {
result.addAll(e);
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy