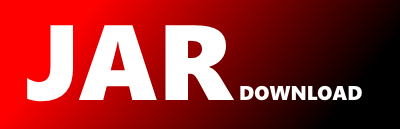
com.legstar.mq.mqcih.bind.MqcihBinding Maven / Gradle / Ivy
/*******************************************************************************
* Copyright (c) 2010 LegSem.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the GNU Lesser Public License v2.1
* which accompanies this distribution, and is available at
* http://www.gnu.org/licenses/old-licenses/gpl-2.0.html
*
* Contributors:
* LegSem - initial API and implementation
******************************************************************************/
package com.legstar.mq.mqcih.bind;
import com.legstar.coxb.ICobolBinding;
import com.legstar.coxb.common.CComplexBinding;
import com.legstar.coxb.ICobolStringBinding;
import com.legstar.coxb.CobolBindingFactory;
import com.legstar.coxb.ICobolBindingFactory;
import com.legstar.coxb.ICobolBinaryBinding;
import com.legstar.coxb.ICobolComplexBinding;
import com.legstar.coxb.host.HostException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import com.legstar.mq.mqcih.Mqcih;
import com.legstar.mq.mqcih.ObjectFactory;
/**
* LegStar Binding for Complex element :
* Mqcih.
*
* This class was generated by LegStar Binding generator.
*/
public class MqcihBinding
extends CComplexBinding {
/** Value object to which this cobol complex element is bound. */
private Mqcih mValueObject;
/** Indicates that the associated Value object just came from the constructor
* and doesn't need to be recreated. */
private boolean mUnusedValueObject = false;
/** Maximum host bytes size for this complex object. */
private static final int BYTE_LENGTH = 180;
/** Child bound to value object property MqcihStrucid(String). */
public ICobolStringBinding _mqcihStrucid;
/** Child bound to value object property MqcihVersion(Integer). */
public ICobolBinaryBinding _mqcihVersion;
/** Child bound to value object property MqcihStruclength(Integer). */
public ICobolBinaryBinding _mqcihStruclength;
/** Child bound to value object property MqcihEncoding(Integer). */
public ICobolBinaryBinding _mqcihEncoding;
/** Child bound to value object property MqcihCodedcharsetid(Integer). */
public ICobolBinaryBinding _mqcihCodedcharsetid;
/** Child bound to value object property MqcihFormat(String). */
public ICobolStringBinding _mqcihFormat;
/** Child bound to value object property MqcihFlags(Integer). */
public ICobolBinaryBinding _mqcihFlags;
/** Child bound to value object property MqcihReturncode(Integer). */
public ICobolBinaryBinding _mqcihReturncode;
/** Child bound to value object property MqcihCompcode(Integer). */
public ICobolBinaryBinding _mqcihCompcode;
/** Child bound to value object property MqcihReason(Integer). */
public ICobolBinaryBinding _mqcihReason;
/** Child bound to value object property MqcihUowcontrol(Integer). */
public ICobolBinaryBinding _mqcihUowcontrol;
/** Child bound to value object property MqcihGetwaitinterval(Integer). */
public ICobolBinaryBinding _mqcihGetwaitinterval;
/** Child bound to value object property MqcihLinktype(Integer). */
public ICobolBinaryBinding _mqcihLinktype;
/** Child bound to value object property MqcihOutputdatalength(Integer). */
public ICobolBinaryBinding _mqcihOutputdatalength;
/** Child bound to value object property MqcihFacilitykeeptime(Integer). */
public ICobolBinaryBinding _mqcihFacilitykeeptime;
/** Child bound to value object property MqcihAdsdescriptor(Integer). */
public ICobolBinaryBinding _mqcihAdsdescriptor;
/** Child bound to value object property MqcihConversationaltask(Integer). */
public ICobolBinaryBinding _mqcihConversationaltask;
/** Child bound to value object property MqcihTaskendstatus(Integer). */
public ICobolBinaryBinding _mqcihTaskendstatus;
/** Child bound to value object property MqcihFacility(String). */
public ICobolStringBinding _mqcihFacility;
/** Child bound to value object property MqcihFunction(String). */
public ICobolStringBinding _mqcihFunction;
/** Child bound to value object property MqcihAbendcode(String). */
public ICobolStringBinding _mqcihAbendcode;
/** Child bound to value object property MqcihAuthenticator(String). */
public ICobolStringBinding _mqcihAuthenticator;
/** Child bound to value object property MqcihReserved1(String). */
public ICobolStringBinding _mqcihReserved1;
/** Child bound to value object property MqcihReplytoformat(String). */
public ICobolStringBinding _mqcihReplytoformat;
/** Child bound to value object property MqcihRemotesysid(String). */
public ICobolStringBinding _mqcihRemotesysid;
/** Child bound to value object property MqcihRemotetransid(String). */
public ICobolStringBinding _mqcihRemotetransid;
/** Child bound to value object property MqcihTransactionid(String). */
public ICobolStringBinding _mqcihTransactionid;
/** Child bound to value object property MqcihFacilitylike(String). */
public ICobolStringBinding _mqcihFacilitylike;
/** Child bound to value object property MqcihAttentionid(String). */
public ICobolStringBinding _mqcihAttentionid;
/** Child bound to value object property MqcihStartcode(String). */
public ICobolStringBinding _mqcihStartcode;
/** Child bound to value object property MqcihCancelcode(String). */
public ICobolStringBinding _mqcihCancelcode;
/** Child bound to value object property MqcihNexttransactionid(String). */
public ICobolStringBinding _mqcihNexttransactionid;
/** Child bound to value object property MqcihReserved2(String). */
public ICobolStringBinding _mqcihReserved2;
/** Child bound to value object property MqcihReserved3(String). */
public ICobolStringBinding _mqcihReserved3;
/** Child bound to value object property MqcihCursorposition(Integer). */
public ICobolBinaryBinding _mqcihCursorposition;
/** Child bound to value object property MqcihErroroffset(Integer). */
public ICobolBinaryBinding _mqcihErroroffset;
/** Child bound to value object property MqcihInputitem(Integer). */
public ICobolBinaryBinding _mqcihInputitem;
/** Child bound to value object property MqcihReserved4(Integer). */
public ICobolBinaryBinding _mqcihReserved4;
/** Logger. */
private final Log _log = LogFactory.getLog(getClass());
/** Binding factory. */
private static final ICobolBindingFactory BF
= CobolBindingFactory.getBindingFactory();
/** Static reference to Value object factory to be used as default. */
private static final ObjectFactory JF = new ObjectFactory();
/** Current Value object factory (Defaults to the static one but can be
* changed). */
private ObjectFactory mValueObjectFactory = JF;
/**
* Constructor for a root Complex element without a bound Value object.
*/
public MqcihBinding() {
this(null);
}
/**
* Constructor for a root Complex element with a bound Value object.
*
* @param valueObject the concrete Value object instance bound to this
* complex element
*/
public MqcihBinding(
final Mqcih valueObject) {
this("", "", null, valueObject);
}
/**
* Constructor for a Complex element as a child of another element and
* an associated Value object.
*
* @param bindingName the identifier for this binding
* @param fieldName field name in parent Value object
* @param valueObject the concrete Value object instance bound to this
* complex element
* @param parentBinding a reference to the parent binding
*/
public MqcihBinding(
final String bindingName,
final String fieldName,
final ICobolComplexBinding parentBinding,
final Mqcih valueObject) {
super(bindingName, fieldName, Mqcih.class, null, parentBinding);
mValueObject = valueObject;
if (mValueObject != null) {
mUnusedValueObject = true;
}
initChildren();
setByteLength(BYTE_LENGTH);
}
/** Creates a binding property for each child. */
private void initChildren() {
if (_log.isDebugEnabled()) {
_log.debug("Initializing started");
}
/* Create binding children instances */
_mqcihStrucid = BF.createStringBinding("MqcihStrucid",
"MqcihStrucid", String.class, this);
_mqcihStrucid.setCobolName("MQCIH-STRUCID");
_mqcihStrucid.setByteLength(4);
_mqcihVersion = BF.createBinaryBinding("MqcihVersion",
"MqcihVersion", Integer.class, this);
_mqcihVersion.setCobolName("MQCIH-VERSION");
_mqcihVersion.setByteLength(4);
_mqcihVersion.setTotalDigits(9);
_mqcihVersion.setIsSigned(true);
_mqcihStruclength = BF.createBinaryBinding("MqcihStruclength",
"MqcihStruclength", Integer.class, this);
_mqcihStruclength.setCobolName("MQCIH-STRUCLENGTH");
_mqcihStruclength.setByteLength(4);
_mqcihStruclength.setTotalDigits(9);
_mqcihStruclength.setIsSigned(true);
_mqcihEncoding = BF.createBinaryBinding("MqcihEncoding",
"MqcihEncoding", Integer.class, this);
_mqcihEncoding.setCobolName("MQCIH-ENCODING");
_mqcihEncoding.setByteLength(4);
_mqcihEncoding.setTotalDigits(9);
_mqcihEncoding.setIsSigned(true);
_mqcihCodedcharsetid = BF.createBinaryBinding("MqcihCodedcharsetid",
"MqcihCodedcharsetid", Integer.class, this);
_mqcihCodedcharsetid.setCobolName("MQCIH-CODEDCHARSETID");
_mqcihCodedcharsetid.setByteLength(4);
_mqcihCodedcharsetid.setTotalDigits(9);
_mqcihCodedcharsetid.setIsSigned(true);
_mqcihFormat = BF.createStringBinding("MqcihFormat",
"MqcihFormat", String.class, this);
_mqcihFormat.setCobolName("MQCIH-FORMAT");
_mqcihFormat.setByteLength(8);
_mqcihFlags = BF.createBinaryBinding("MqcihFlags",
"MqcihFlags", Integer.class, this);
_mqcihFlags.setCobolName("MQCIH-FLAGS");
_mqcihFlags.setByteLength(4);
_mqcihFlags.setTotalDigits(9);
_mqcihFlags.setIsSigned(true);
_mqcihReturncode = BF.createBinaryBinding("MqcihReturncode",
"MqcihReturncode", Integer.class, this);
_mqcihReturncode.setCobolName("MQCIH-RETURNCODE");
_mqcihReturncode.setByteLength(4);
_mqcihReturncode.setTotalDigits(9);
_mqcihReturncode.setIsSigned(true);
_mqcihCompcode = BF.createBinaryBinding("MqcihCompcode",
"MqcihCompcode", Integer.class, this);
_mqcihCompcode.setCobolName("MQCIH-COMPCODE");
_mqcihCompcode.setByteLength(4);
_mqcihCompcode.setTotalDigits(9);
_mqcihCompcode.setIsSigned(true);
_mqcihReason = BF.createBinaryBinding("MqcihReason",
"MqcihReason", Integer.class, this);
_mqcihReason.setCobolName("MQCIH-REASON");
_mqcihReason.setByteLength(4);
_mqcihReason.setTotalDigits(9);
_mqcihReason.setIsSigned(true);
_mqcihUowcontrol = BF.createBinaryBinding("MqcihUowcontrol",
"MqcihUowcontrol", Integer.class, this);
_mqcihUowcontrol.setCobolName("MQCIH-UOWCONTROL");
_mqcihUowcontrol.setByteLength(4);
_mqcihUowcontrol.setTotalDigits(9);
_mqcihUowcontrol.setIsSigned(true);
_mqcihGetwaitinterval = BF.createBinaryBinding("MqcihGetwaitinterval",
"MqcihGetwaitinterval", Integer.class, this);
_mqcihGetwaitinterval.setCobolName("MQCIH-GETWAITINTERVAL");
_mqcihGetwaitinterval.setByteLength(4);
_mqcihGetwaitinterval.setTotalDigits(9);
_mqcihGetwaitinterval.setIsSigned(true);
_mqcihLinktype = BF.createBinaryBinding("MqcihLinktype",
"MqcihLinktype", Integer.class, this);
_mqcihLinktype.setCobolName("MQCIH-LINKTYPE");
_mqcihLinktype.setByteLength(4);
_mqcihLinktype.setTotalDigits(9);
_mqcihLinktype.setIsSigned(true);
_mqcihOutputdatalength = BF.createBinaryBinding("MqcihOutputdatalength",
"MqcihOutputdatalength", Integer.class, this);
_mqcihOutputdatalength.setCobolName("MQCIH-OUTPUTDATALENGTH");
_mqcihOutputdatalength.setByteLength(4);
_mqcihOutputdatalength.setTotalDigits(9);
_mqcihOutputdatalength.setIsSigned(true);
_mqcihFacilitykeeptime = BF.createBinaryBinding("MqcihFacilitykeeptime",
"MqcihFacilitykeeptime", Integer.class, this);
_mqcihFacilitykeeptime.setCobolName("MQCIH-FACILITYKEEPTIME");
_mqcihFacilitykeeptime.setByteLength(4);
_mqcihFacilitykeeptime.setTotalDigits(9);
_mqcihFacilitykeeptime.setIsSigned(true);
_mqcihAdsdescriptor = BF.createBinaryBinding("MqcihAdsdescriptor",
"MqcihAdsdescriptor", Integer.class, this);
_mqcihAdsdescriptor.setCobolName("MQCIH-ADSDESCRIPTOR");
_mqcihAdsdescriptor.setByteLength(4);
_mqcihAdsdescriptor.setTotalDigits(9);
_mqcihAdsdescriptor.setIsSigned(true);
_mqcihConversationaltask = BF.createBinaryBinding("MqcihConversationaltask",
"MqcihConversationaltask", Integer.class, this);
_mqcihConversationaltask.setCobolName("MQCIH-CONVERSATIONALTASK");
_mqcihConversationaltask.setByteLength(4);
_mqcihConversationaltask.setTotalDigits(9);
_mqcihConversationaltask.setIsSigned(true);
_mqcihTaskendstatus = BF.createBinaryBinding("MqcihTaskendstatus",
"MqcihTaskendstatus", Integer.class, this);
_mqcihTaskendstatus.setCobolName("MQCIH-TASKENDSTATUS");
_mqcihTaskendstatus.setByteLength(4);
_mqcihTaskendstatus.setTotalDigits(9);
_mqcihTaskendstatus.setIsSigned(true);
_mqcihFacility = BF.createStringBinding("MqcihFacility",
"MqcihFacility", String.class, this);
_mqcihFacility.setCobolName("MQCIH-FACILITY");
_mqcihFacility.setByteLength(8);
_mqcihFunction = BF.createStringBinding("MqcihFunction",
"MqcihFunction", String.class, this);
_mqcihFunction.setCobolName("MQCIH-FUNCTION");
_mqcihFunction.setByteLength(4);
_mqcihAbendcode = BF.createStringBinding("MqcihAbendcode",
"MqcihAbendcode", String.class, this);
_mqcihAbendcode.setCobolName("MQCIH-ABENDCODE");
_mqcihAbendcode.setByteLength(4);
_mqcihAuthenticator = BF.createStringBinding("MqcihAuthenticator",
"MqcihAuthenticator", String.class, this);
_mqcihAuthenticator.setCobolName("MQCIH-AUTHENTICATOR");
_mqcihAuthenticator.setByteLength(8);
_mqcihReserved1 = BF.createStringBinding("MqcihReserved1",
"MqcihReserved1", String.class, this);
_mqcihReserved1.setCobolName("MQCIH-RESERVED1");
_mqcihReserved1.setByteLength(8);
_mqcihReplytoformat = BF.createStringBinding("MqcihReplytoformat",
"MqcihReplytoformat", String.class, this);
_mqcihReplytoformat.setCobolName("MQCIH-REPLYTOFORMAT");
_mqcihReplytoformat.setByteLength(8);
_mqcihRemotesysid = BF.createStringBinding("MqcihRemotesysid",
"MqcihRemotesysid", String.class, this);
_mqcihRemotesysid.setCobolName("MQCIH-REMOTESYSID");
_mqcihRemotesysid.setByteLength(4);
_mqcihRemotetransid = BF.createStringBinding("MqcihRemotetransid",
"MqcihRemotetransid", String.class, this);
_mqcihRemotetransid.setCobolName("MQCIH-REMOTETRANSID");
_mqcihRemotetransid.setByteLength(4);
_mqcihTransactionid = BF.createStringBinding("MqcihTransactionid",
"MqcihTransactionid", String.class, this);
_mqcihTransactionid.setCobolName("MQCIH-TRANSACTIONID");
_mqcihTransactionid.setByteLength(4);
_mqcihFacilitylike = BF.createStringBinding("MqcihFacilitylike",
"MqcihFacilitylike", String.class, this);
_mqcihFacilitylike.setCobolName("MQCIH-FACILITYLIKE");
_mqcihFacilitylike.setByteLength(4);
_mqcihAttentionid = BF.createStringBinding("MqcihAttentionid",
"MqcihAttentionid", String.class, this);
_mqcihAttentionid.setCobolName("MQCIH-ATTENTIONID");
_mqcihAttentionid.setByteLength(4);
_mqcihStartcode = BF.createStringBinding("MqcihStartcode",
"MqcihStartcode", String.class, this);
_mqcihStartcode.setCobolName("MQCIH-STARTCODE");
_mqcihStartcode.setByteLength(4);
_mqcihCancelcode = BF.createStringBinding("MqcihCancelcode",
"MqcihCancelcode", String.class, this);
_mqcihCancelcode.setCobolName("MQCIH-CANCELCODE");
_mqcihCancelcode.setByteLength(4);
_mqcihNexttransactionid = BF.createStringBinding("MqcihNexttransactionid",
"MqcihNexttransactionid", String.class, this);
_mqcihNexttransactionid.setCobolName("MQCIH-NEXTTRANSACTIONID");
_mqcihNexttransactionid.setByteLength(4);
_mqcihReserved2 = BF.createStringBinding("MqcihReserved2",
"MqcihReserved2", String.class, this);
_mqcihReserved2.setCobolName("MQCIH-RESERVED2");
_mqcihReserved2.setByteLength(8);
_mqcihReserved3 = BF.createStringBinding("MqcihReserved3",
"MqcihReserved3", String.class, this);
_mqcihReserved3.setCobolName("MQCIH-RESERVED3");
_mqcihReserved3.setByteLength(8);
_mqcihCursorposition = BF.createBinaryBinding("MqcihCursorposition",
"MqcihCursorposition", Integer.class, this);
_mqcihCursorposition.setCobolName("MQCIH-CURSORPOSITION");
_mqcihCursorposition.setByteLength(4);
_mqcihCursorposition.setTotalDigits(9);
_mqcihCursorposition.setIsSigned(true);
_mqcihErroroffset = BF.createBinaryBinding("MqcihErroroffset",
"MqcihErroroffset", Integer.class, this);
_mqcihErroroffset.setCobolName("MQCIH-ERROROFFSET");
_mqcihErroroffset.setByteLength(4);
_mqcihErroroffset.setTotalDigits(9);
_mqcihErroroffset.setIsSigned(true);
_mqcihInputitem = BF.createBinaryBinding("MqcihInputitem",
"MqcihInputitem", Integer.class, this);
_mqcihInputitem.setCobolName("MQCIH-INPUTITEM");
_mqcihInputitem.setByteLength(4);
_mqcihInputitem.setTotalDigits(9);
_mqcihInputitem.setIsSigned(true);
_mqcihReserved4 = BF.createBinaryBinding("MqcihReserved4",
"MqcihReserved4", Integer.class, this);
_mqcihReserved4.setCobolName("MQCIH-RESERVED4");
_mqcihReserved4.setByteLength(4);
_mqcihReserved4.setTotalDigits(9);
_mqcihReserved4.setIsSigned(true);
/* Add children to children list */
getChildrenList().add(_mqcihStrucid);
getChildrenList().add(_mqcihVersion);
getChildrenList().add(_mqcihStruclength);
getChildrenList().add(_mqcihEncoding);
getChildrenList().add(_mqcihCodedcharsetid);
getChildrenList().add(_mqcihFormat);
getChildrenList().add(_mqcihFlags);
getChildrenList().add(_mqcihReturncode);
getChildrenList().add(_mqcihCompcode);
getChildrenList().add(_mqcihReason);
getChildrenList().add(_mqcihUowcontrol);
getChildrenList().add(_mqcihGetwaitinterval);
getChildrenList().add(_mqcihLinktype);
getChildrenList().add(_mqcihOutputdatalength);
getChildrenList().add(_mqcihFacilitykeeptime);
getChildrenList().add(_mqcihAdsdescriptor);
getChildrenList().add(_mqcihConversationaltask);
getChildrenList().add(_mqcihTaskendstatus);
getChildrenList().add(_mqcihFacility);
getChildrenList().add(_mqcihFunction);
getChildrenList().add(_mqcihAbendcode);
getChildrenList().add(_mqcihAuthenticator);
getChildrenList().add(_mqcihReserved1);
getChildrenList().add(_mqcihReplytoformat);
getChildrenList().add(_mqcihRemotesysid);
getChildrenList().add(_mqcihRemotetransid);
getChildrenList().add(_mqcihTransactionid);
getChildrenList().add(_mqcihFacilitylike);
getChildrenList().add(_mqcihAttentionid);
getChildrenList().add(_mqcihStartcode);
getChildrenList().add(_mqcihCancelcode);
getChildrenList().add(_mqcihNexttransactionid);
getChildrenList().add(_mqcihReserved2);
getChildrenList().add(_mqcihReserved3);
getChildrenList().add(_mqcihCursorposition);
getChildrenList().add(_mqcihErroroffset);
getChildrenList().add(_mqcihInputitem);
getChildrenList().add(_mqcihReserved4);
if (_log.isDebugEnabled()) {
_log.debug("Initializing successful");
}
}
/** {@inheritDoc} */
public void createValueObject() throws HostException {
/* Since this complex binding has a constructor that takes a
* Value object, we might already have a Value object that
* was not used yet. */
if (mUnusedValueObject && mValueObject != null) {
mUnusedValueObject = false;
return;
}
mValueObject = mValueObjectFactory.createMqcih();
}
/** {@inheritDoc} */
public void setChildrenValues() throws HostException {
/* Make sure there is an associated Value object*/
if (mValueObject == null) {
createValueObject();
}
/* Get Value object property _mqcihStrucid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihStrucid"
+ " value=" + mValueObject.getMqcihStrucid());
}
_mqcihStrucid.setObjectValue(mValueObject.getMqcihStrucid());
/* Get Value object property _mqcihVersion */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihVersion"
+ " value=" + mValueObject.getMqcihVersion());
}
_mqcihVersion.setObjectValue(mValueObject.getMqcihVersion());
/* Get Value object property _mqcihStruclength */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihStruclength"
+ " value=" + mValueObject.getMqcihStruclength());
}
_mqcihStruclength.setObjectValue(mValueObject.getMqcihStruclength());
/* Get Value object property _mqcihEncoding */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihEncoding"
+ " value=" + mValueObject.getMqcihEncoding());
}
_mqcihEncoding.setObjectValue(mValueObject.getMqcihEncoding());
/* Get Value object property _mqcihCodedcharsetid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihCodedcharsetid"
+ " value=" + mValueObject.getMqcihCodedcharsetid());
}
_mqcihCodedcharsetid.setObjectValue(mValueObject.getMqcihCodedcharsetid());
/* Get Value object property _mqcihFormat */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihFormat"
+ " value=" + mValueObject.getMqcihFormat());
}
_mqcihFormat.setObjectValue(mValueObject.getMqcihFormat());
/* Get Value object property _mqcihFlags */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihFlags"
+ " value=" + mValueObject.getMqcihFlags());
}
_mqcihFlags.setObjectValue(mValueObject.getMqcihFlags());
/* Get Value object property _mqcihReturncode */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReturncode"
+ " value=" + mValueObject.getMqcihReturncode());
}
_mqcihReturncode.setObjectValue(mValueObject.getMqcihReturncode());
/* Get Value object property _mqcihCompcode */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihCompcode"
+ " value=" + mValueObject.getMqcihCompcode());
}
_mqcihCompcode.setObjectValue(mValueObject.getMqcihCompcode());
/* Get Value object property _mqcihReason */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReason"
+ " value=" + mValueObject.getMqcihReason());
}
_mqcihReason.setObjectValue(mValueObject.getMqcihReason());
/* Get Value object property _mqcihUowcontrol */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihUowcontrol"
+ " value=" + mValueObject.getMqcihUowcontrol());
}
_mqcihUowcontrol.setObjectValue(mValueObject.getMqcihUowcontrol());
/* Get Value object property _mqcihGetwaitinterval */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihGetwaitinterval"
+ " value=" + mValueObject.getMqcihGetwaitinterval());
}
_mqcihGetwaitinterval.setObjectValue(mValueObject.getMqcihGetwaitinterval());
/* Get Value object property _mqcihLinktype */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihLinktype"
+ " value=" + mValueObject.getMqcihLinktype());
}
_mqcihLinktype.setObjectValue(mValueObject.getMqcihLinktype());
/* Get Value object property _mqcihOutputdatalength */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihOutputdatalength"
+ " value=" + mValueObject.getMqcihOutputdatalength());
}
_mqcihOutputdatalength.setObjectValue(mValueObject.getMqcihOutputdatalength());
/* Get Value object property _mqcihFacilitykeeptime */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihFacilitykeeptime"
+ " value=" + mValueObject.getMqcihFacilitykeeptime());
}
_mqcihFacilitykeeptime.setObjectValue(mValueObject.getMqcihFacilitykeeptime());
/* Get Value object property _mqcihAdsdescriptor */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihAdsdescriptor"
+ " value=" + mValueObject.getMqcihAdsdescriptor());
}
_mqcihAdsdescriptor.setObjectValue(mValueObject.getMqcihAdsdescriptor());
/* Get Value object property _mqcihConversationaltask */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihConversationaltask"
+ " value=" + mValueObject.getMqcihConversationaltask());
}
_mqcihConversationaltask.setObjectValue(mValueObject.getMqcihConversationaltask());
/* Get Value object property _mqcihTaskendstatus */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihTaskendstatus"
+ " value=" + mValueObject.getMqcihTaskendstatus());
}
_mqcihTaskendstatus.setObjectValue(mValueObject.getMqcihTaskendstatus());
/* Get Value object property _mqcihFacility */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihFacility"
+ " value=" + mValueObject.getMqcihFacility());
}
_mqcihFacility.setObjectValue(mValueObject.getMqcihFacility());
/* Get Value object property _mqcihFunction */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihFunction"
+ " value=" + mValueObject.getMqcihFunction());
}
_mqcihFunction.setObjectValue(mValueObject.getMqcihFunction());
/* Get Value object property _mqcihAbendcode */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihAbendcode"
+ " value=" + mValueObject.getMqcihAbendcode());
}
_mqcihAbendcode.setObjectValue(mValueObject.getMqcihAbendcode());
/* Get Value object property _mqcihAuthenticator */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihAuthenticator"
+ " value=" + mValueObject.getMqcihAuthenticator());
}
_mqcihAuthenticator.setObjectValue(mValueObject.getMqcihAuthenticator());
/* Get Value object property _mqcihReserved1 */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReserved1"
+ " value=" + mValueObject.getMqcihReserved1());
}
_mqcihReserved1.setObjectValue(mValueObject.getMqcihReserved1());
/* Get Value object property _mqcihReplytoformat */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReplytoformat"
+ " value=" + mValueObject.getMqcihReplytoformat());
}
_mqcihReplytoformat.setObjectValue(mValueObject.getMqcihReplytoformat());
/* Get Value object property _mqcihRemotesysid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihRemotesysid"
+ " value=" + mValueObject.getMqcihRemotesysid());
}
_mqcihRemotesysid.setObjectValue(mValueObject.getMqcihRemotesysid());
/* Get Value object property _mqcihRemotetransid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihRemotetransid"
+ " value=" + mValueObject.getMqcihRemotetransid());
}
_mqcihRemotetransid.setObjectValue(mValueObject.getMqcihRemotetransid());
/* Get Value object property _mqcihTransactionid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihTransactionid"
+ " value=" + mValueObject.getMqcihTransactionid());
}
_mqcihTransactionid.setObjectValue(mValueObject.getMqcihTransactionid());
/* Get Value object property _mqcihFacilitylike */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihFacilitylike"
+ " value=" + mValueObject.getMqcihFacilitylike());
}
_mqcihFacilitylike.setObjectValue(mValueObject.getMqcihFacilitylike());
/* Get Value object property _mqcihAttentionid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihAttentionid"
+ " value=" + mValueObject.getMqcihAttentionid());
}
_mqcihAttentionid.setObjectValue(mValueObject.getMqcihAttentionid());
/* Get Value object property _mqcihStartcode */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihStartcode"
+ " value=" + mValueObject.getMqcihStartcode());
}
_mqcihStartcode.setObjectValue(mValueObject.getMqcihStartcode());
/* Get Value object property _mqcihCancelcode */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihCancelcode"
+ " value=" + mValueObject.getMqcihCancelcode());
}
_mqcihCancelcode.setObjectValue(mValueObject.getMqcihCancelcode());
/* Get Value object property _mqcihNexttransactionid */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihNexttransactionid"
+ " value=" + mValueObject.getMqcihNexttransactionid());
}
_mqcihNexttransactionid.setObjectValue(mValueObject.getMqcihNexttransactionid());
/* Get Value object property _mqcihReserved2 */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReserved2"
+ " value=" + mValueObject.getMqcihReserved2());
}
_mqcihReserved2.setObjectValue(mValueObject.getMqcihReserved2());
/* Get Value object property _mqcihReserved3 */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReserved3"
+ " value=" + mValueObject.getMqcihReserved3());
}
_mqcihReserved3.setObjectValue(mValueObject.getMqcihReserved3());
/* Get Value object property _mqcihCursorposition */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihCursorposition"
+ " value=" + mValueObject.getMqcihCursorposition());
}
_mqcihCursorposition.setObjectValue(mValueObject.getMqcihCursorposition());
/* Get Value object property _mqcihErroroffset */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihErroroffset"
+ " value=" + mValueObject.getMqcihErroroffset());
}
_mqcihErroroffset.setObjectValue(mValueObject.getMqcihErroroffset());
/* Get Value object property _mqcihInputitem */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihInputitem"
+ " value=" + mValueObject.getMqcihInputitem());
}
_mqcihInputitem.setObjectValue(mValueObject.getMqcihInputitem());
/* Get Value object property _mqcihReserved4 */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "_mqcihReserved4"
+ " value=" + mValueObject.getMqcihReserved4());
}
_mqcihReserved4.setObjectValue(mValueObject.getMqcihReserved4());
}
/** {@inheritDoc} */
public void setPropertyValue(final int index) throws HostException {
ICobolBinding child = getChildrenList().get(index);
/* Children that are not bound to a value object are ignored.
* This includes Choices and dynamically generated counters
* for instance. */
if (!child.isBound()) {
return;
}
/* Set the Value object property value from binding object */
Object bindingValue = null;
switch (index) {
case 0:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihStrucid((String) bindingValue);
break;
case 1:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihVersion((Integer) bindingValue);
break;
case 2:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihStruclength((Integer) bindingValue);
break;
case 3:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihEncoding((Integer) bindingValue);
break;
case 4:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihCodedcharsetid((Integer) bindingValue);
break;
case 5:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihFormat((String) bindingValue);
break;
case 6:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihFlags((Integer) bindingValue);
break;
case 7:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihReturncode((Integer) bindingValue);
break;
case 8:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihCompcode((Integer) bindingValue);
break;
case 9:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihReason((Integer) bindingValue);
break;
case 10:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihUowcontrol((Integer) bindingValue);
break;
case 11:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihGetwaitinterval((Integer) bindingValue);
break;
case 12:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihLinktype((Integer) bindingValue);
break;
case 13:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihOutputdatalength((Integer) bindingValue);
break;
case 14:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihFacilitykeeptime((Integer) bindingValue);
break;
case 15:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihAdsdescriptor((Integer) bindingValue);
break;
case 16:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihConversationaltask((Integer) bindingValue);
break;
case 17:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihTaskendstatus((Integer) bindingValue);
break;
case 18:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihFacility((String) bindingValue);
break;
case 19:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihFunction((String) bindingValue);
break;
case 20:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihAbendcode((String) bindingValue);
break;
case 21:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihAuthenticator((String) bindingValue);
break;
case 22:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihReserved1((String) bindingValue);
break;
case 23:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihReplytoformat((String) bindingValue);
break;
case 24:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihRemotesysid((String) bindingValue);
break;
case 25:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihRemotetransid((String) bindingValue);
break;
case 26:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihTransactionid((String) bindingValue);
break;
case 27:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihFacilitylike((String) bindingValue);
break;
case 28:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihAttentionid((String) bindingValue);
break;
case 29:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihStartcode((String) bindingValue);
break;
case 30:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihCancelcode((String) bindingValue);
break;
case 31:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihNexttransactionid((String) bindingValue);
break;
case 32:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihReserved2((String) bindingValue);
break;
case 33:
bindingValue = child.getObjectValue(String.class);
mValueObject.setMqcihReserved3((String) bindingValue);
break;
case 34:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihCursorposition((Integer) bindingValue);
break;
case 35:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihErroroffset((Integer) bindingValue);
break;
case 36:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihInputitem((Integer) bindingValue);
break;
case 37:
bindingValue = child.getObjectValue(Integer.class);
mValueObject.setMqcihReserved4((Integer) bindingValue);
break;
default:
break;
}
if (_log.isDebugEnabled()) {
_log.debug("Setting value of Value object property "
+ child.getJaxbName()
+ " value=" + bindingValue);
}
}
/** {@inheritDoc} */
public Object getObjectValue(
final Class < ? > type) throws HostException {
if (type.equals(Mqcih.class)) {
return mValueObject;
} else {
throw new HostException("Attempt to get binding " + getBindingName()
+ " as an incompatible type " + type);
}
}
/** {@inheritDoc} */
public void setObjectValue(
final Object bindingValue) throws HostException {
if (bindingValue == null) {
mValueObject = null;
return;
}
if (bindingValue.getClass().equals(Mqcih.class)) {
mValueObject = (Mqcih) bindingValue;
} else {
throw new HostException("Attempt to set binding " + getBindingName()
+ " from an incompatible value " + bindingValue);
}
}
/**
* @return the java object factory for objects creation
*/
public ObjectFactory getObjectFactory() {
return mValueObjectFactory;
}
/**
* @param valueObjectFactory the java object factory for objects creation
*/
public void setObjectFactory(final Object valueObjectFactory) {
mValueObjectFactory = (ObjectFactory) valueObjectFactory;
}
/** {@inheritDoc} */
public boolean isSet() {
return (mValueObject != null);
}
/**
* @return the bound Value object
*/
public Mqcih getMqcih() {
return mValueObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy