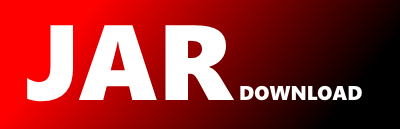
com.legstar.cobol.CobolStructureLexer Maven / Gradle / Ivy
// $ANTLR 3.5.2 com\\legstar\\cobol\\CobolStructureLexer.g 2015-02-26 16:23:21
package com.legstar.cobol;
import java.util.HashMap;
import java.util.Map;
import org.antlr.runtime.*;
import java.util.Stack;
import java.util.List;
import java.util.ArrayList;
/*******************************************************************************
* Copyright (c) 2009 LegSem.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the GNU Lesser Public License v2.1
* which accompanies this distribution, and is available at
* http://www.gnu.org/licenses/old-licenses/gpl-2.0.html
*
* Contributors:
* LegSem - initial API and implementation
******************************************************************************/
@SuppressWarnings("all")
public class CobolStructureLexer extends Lexer {
public static final int EOF=-1;
public static final int ALL_CONSTANT=4;
public static final int ALPHANUM_LITERAL_FRAGMENT=5;
public static final int ALPHANUM_LITERAL_STRING=6;
public static final int APOST=7;
public static final int ARE_KEYWORD=8;
public static final int ASCENDING_KEYWORD=9;
public static final int BINARY_KEYWORD=10;
public static final int BLANK_KEYWORD=11;
public static final int BY_KEYWORD=12;
public static final int CHARACTER_KEYWORD=13;
public static final int CONDITION_LEVEL=14;
public static final int CONTINUATION_CHAR=15;
public static final int CONTINUED_ALPHANUM_LITERAL_FRAGMENT=16;
public static final int DATA_ITEM_LEVEL=17;
public static final int DATA_NAME=18;
public static final int DATE_FORMAT_KEYWORD=19;
public static final int DATE_KEYWORD=20;
public static final int DATE_PATTERN=21;
public static final int DBCS_LITERAL_STRING=22;
public static final int DECIMAL_POINT=23;
public static final int DEPENDING_KEYWORD=24;
public static final int DESCENDING_KEYWORD=25;
public static final int DISPLAY_1_KEYWORD=26;
public static final int DISPLAY_KEYWORD=27;
public static final int DOUBLE_FLOAT_KEYWORD=28;
public static final int EXTERNAL_KEYWORD=29;
public static final int FLOAT_PART2=30;
public static final int FUNCTION_POINTER_KEYWORD=31;
public static final int GLOBAL_KEYWORD=32;
public static final int GROUP_USAGE_KEYWORD=33;
public static final int HEX_LITERAL_STRING=34;
public static final int HIGH_VALUE_CONSTANT=35;
public static final int INDEXED_KEYWORD=36;
public static final int INDEX_KEYWORD=37;
public static final int INT=38;
public static final int IS_KEYWORD=39;
public static final int JUSTIFIED_KEYWORD=40;
public static final int KEY_KEYWORD=41;
public static final int LEFT_KEYWORD=42;
public static final int LETTER=43;
public static final int LOW_VALUE_CONSTANT=44;
public static final int NATIONAL_HEX_LITERAL_STRING=45;
public static final int NATIONAL_KEYWORD=46;
public static final int NATIONAL_LITERAL_STRING=47;
public static final int NATIVE_BINARY_KEYWORD=48;
public static final int NEWLINE=49;
public static final int NULL_CONSTANT=50;
public static final int OCCURS_KEYWORD=51;
public static final int ON_KEYWORD=52;
public static final int PACKED_DECIMAL_KEYWORD=53;
public static final int PERIOD=54;
public static final int PICTURE_CHAR=55;
public static final int PICTURE_KEYWORD=56;
public static final int PICTURE_PART=57;
public static final int POINTER_KEYWORD=58;
public static final int PROCEDURE_POINTER_KEYWORD=59;
public static final int QUOTE=60;
public static final int QUOTE_CONSTANT=61;
public static final int REDEFINES_KEYWORD=62;
public static final int RENAMES_KEYWORD=63;
public static final int RENAMES_LEVEL=64;
public static final int RIGHT_KEYWORD=65;
public static final int SEPARATE_KEYWORD=66;
public static final int SIGNED_INT=67;
public static final int SIGN_KEYWORD=68;
public static final int SIGN_LEADING_KEYWORD=69;
public static final int SIGN_TRAILING_KEYWORD=70;
public static final int SINGLE_FLOAT_KEYWORD=71;
public static final int SPACE=72;
public static final int SPACE_CONSTANT=73;
public static final int SYNCHRONIZED_KEYWORD=74;
public static final int THROUGH_KEYWORD=75;
public static final int TIMES_KEYWORD=76;
public static final int TO_KEYWORD=77;
public static final int USAGE_KEYWORD=78;
public static final int VALUE_KEYWORD=79;
public static final int WHEN_KEYWORD=80;
public static final int WHITESPACE=81;
public static final int ZERO_CONSTANT=82;
public static final int ZERO_LITERAL_STRING=83;
/** Keeps track of the last COBOL keyword recognized. This helps
disambiguate lexing rules. */
private int lastKeyword = PERIOD;
/** True when a picture string is being built (potentially from multiple parts). */
private boolean pictureStarted;
/** Map to help with COBOL keyword recognition.*/
private static Map KEYWORDS_MAP = new HashMap ()
{{
put("RENAMES", RENAMES_KEYWORD);
put("THROUGH", THROUGH_KEYWORD);
put("THRU", THROUGH_KEYWORD);
put("REDEFINES", REDEFINES_KEYWORD);
put("BLANK", BLANK_KEYWORD);
put("WHEN", Token.SKIP_TOKEN.getType());
put("EXTERNAL", EXTERNAL_KEYWORD);
put("GLOBAL", GLOBAL_KEYWORD);
put("GROUP-USAGE", GROUP_USAGE_KEYWORD);
put("IS", Token.SKIP_TOKEN.getType());
put("ARE", Token.SKIP_TOKEN.getType());
put("NATIONAL", NATIONAL_KEYWORD);
put("JUSTIFIED", JUSTIFIED_KEYWORD);
put("JUST", JUSTIFIED_KEYWORD);
put("RIGHT", RIGHT_KEYWORD);
put("OCCURS", OCCURS_KEYWORD);
put("TIMES", Token.SKIP_TOKEN.getType());
put("TO", TO_KEYWORD);
put("ASCENDING", ASCENDING_KEYWORD);
put("DESCENDING", DESCENDING_KEYWORD);
put("KEY", KEY_KEYWORD);
put("INDEXED", INDEXED_KEYWORD);
put("BY", Token.SKIP_TOKEN.getType());
put("PICTURE", PICTURE_KEYWORD);
put("PIC", PICTURE_KEYWORD);
put("DEPENDING", DEPENDING_KEYWORD);
put("ON", Token.SKIP_TOKEN.getType());
put("SIGN", Token.SKIP_TOKEN.getType());
put("LEADING", SIGN_LEADING_KEYWORD);
put("TRAILING", SIGN_TRAILING_KEYWORD);
put("SEPARATE", SEPARATE_KEYWORD);
put("CHARACTER", Token.SKIP_TOKEN.getType());
put("SYNCHRONIZED", SYNCHRONIZED_KEYWORD);
put("SYNC", SYNCHRONIZED_KEYWORD);
put("LEFT", LEFT_KEYWORD);
put("USAGE", USAGE_KEYWORD);
put("COMPUTATIONAL-1", SINGLE_FLOAT_KEYWORD);
put("COMP-1", SINGLE_FLOAT_KEYWORD);
put("COMPUTATIONAL-2", DOUBLE_FLOAT_KEYWORD);
put("COMP-2", DOUBLE_FLOAT_KEYWORD);
put("COMPUTATIONAL-5", NATIVE_BINARY_KEYWORD);
put("COMP-5", NATIVE_BINARY_KEYWORD);
put("COMPUTATIONAL-3", PACKED_DECIMAL_KEYWORD);
put("COMP-3", PACKED_DECIMAL_KEYWORD);
put("PACKED-DECIMAL", PACKED_DECIMAL_KEYWORD);
put("COMPUTATIONAL", BINARY_KEYWORD);
put("COMP", BINARY_KEYWORD);
put("COMPUTATIONAL-4", BINARY_KEYWORD);
put("COMP-4", BINARY_KEYWORD);
put("BINARY", BINARY_KEYWORD);
put("DISPLAY-1", DISPLAY_1_KEYWORD);
put("DISPLAY", DISPLAY_KEYWORD);
put("INDEX", INDEX_KEYWORD);
put("POINTER", POINTER_KEYWORD);
put("PROCEDURE-POINTER", PROCEDURE_POINTER_KEYWORD);
put("FUNCTION-POINTER", FUNCTION_POINTER_KEYWORD);
put("VALUES", VALUE_KEYWORD);
put("VALUE", VALUE_KEYWORD);
put("DATE", DATE_KEYWORD);
put("FORMAT", DATE_FORMAT_KEYWORD);
put("ZEROES", ZERO_CONSTANT);
put("ZEROS", ZERO_CONSTANT);
put("ZERO", ZERO_CONSTANT);
put("SPACES", SPACE_CONSTANT);
put("SPACE", SPACE_CONSTANT);
put("HIGH-VALUES", HIGH_VALUE_CONSTANT);
put("HIGH-VALUE", HIGH_VALUE_CONSTANT);
put("LOW-VALUES", LOW_VALUE_CONSTANT);
put("LOW-VALUE", LOW_VALUE_CONSTANT);
put("QUOTES", QUOTE_CONSTANT);
put("QUOTE", QUOTE_CONSTANT);
put("ALL", ALL_CONSTANT);
put("NULLS", NULL_CONSTANT);
put("NULL", NULL_CONSTANT);
}};
/**
* Adding all the COBOL keywords directly generates too much code. It
* is more efficient, with the current release of ANTLR, to recognize
* keywords manually.
* @param text the text to match with keywords
* @param originalType the initial token type
* @return the keyword type if a match is found otherwise the original type
*/
public int matchKeywords(
final String text,
final int originalType) {
Integer type = KEYWORDS_MAP.get(text.toUpperCase());
if (type == null) {
return originalType;
}
if (type == Token.SKIP_TOKEN.getType()) {
skip();
return type;
} else if(type == PICTURE_KEYWORD) {
/* Just found a PICTURE keyword, start collecting picture string parts */
pictureStarted = true;
} else if(type == DATE_KEYWORD) {
skip();
} else if(type == DATE_FORMAT_KEYWORD) {
/* Format is a date format only if preceded by date */
if (lastKeyword != DATE_KEYWORD) {
type = DATA_NAME;
}
}
lastKeyword = type;
return type;
}
/**
* Check that a string is a valid data name.
* @param string a proposed data name
* @throws FailedPredicateException if this is not a valid data name
*/
public void checkDataName(final String string) throws FailedPredicateException {
if (!string.matches("[a-zA-Z0-9][a-zA-Z0-9_\\-]*")) {
throw new FailedPredicateException(
input, "DATA_NAME", "Syntax error in last clause");
}
}
/**
* Check that a string is a valid part of a picture string.
*
* Check that we are in the context of collecting picture string parts.
*
* When string is a valid picture part, close picture string sequence
* if the next character is space or new line.
*
* @param string a picture string or part of a picture string
* @throws FailedPredicateException if this is not a valid picture string part
*/
public void checkPicture(final String string) throws FailedPredicateException {
if (!pictureStarted) {
throw new FailedPredicateException(
input, "PICTURE_PART", "Syntax error in last picture clause");
}
if (input.LA(1) == ' ' || input.LA(1) == '\r' || input.LA(1) == '\n' || input.LA(1) == -1) {
pictureStarted = false;
}
}
// delegates
// delegators
public Lexer[] getDelegates() {
return new Lexer[] {};
}
public CobolStructureLexer() {}
public CobolStructureLexer(CharStream input) {
this(input, new RecognizerSharedState());
}
public CobolStructureLexer(CharStream input, RecognizerSharedState state) {
super(input,state);
}
@Override public String getGrammarFileName() { return "com\\legstar\\cobol\\CobolStructureLexer.g"; }
// $ANTLR start "PERIOD"
public final void mPERIOD() throws RecognitionException {
try {
int _type = PERIOD;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:262:5: ( '.' )
// com\\legstar\\cobol\\CobolStructureLexer.g:263:5: '.'
{
/* If this period is not followed by a space or a newline, then we consider
* it is a decimal point and not to be used as a sentence delimiter.*/
if (input.LA(2) != ' ' && input.LA(2) != '\r' && input.LA(2) != '\n' && input.LA(2) != -1) {
_type = DECIMAL_POINT;
} else {
/* This will set the context as the end of a data entry */
lastKeyword = PERIOD;
}
match('.');
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PERIOD"
// $ANTLR start "INT"
public final void mINT() throws RecognitionException {
try {
int _type = INT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:280:5: ( ( '0' .. '9' )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:280:9: ( '0' .. '9' )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:280:9: ( '0' .. '9' )+
int cnt1=0;
loop1:
while (true) {
int alt1=2;
int LA1_0 = input.LA(1);
if ( ((LA1_0 >= '0' && LA1_0 <= '9')) ) {
alt1=1;
}
switch (alt1) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt1 >= 1 ) break loop1;
EarlyExitException eee = new EarlyExitException(1, input);
throw eee;
}
cnt1++;
}
if (lastKeyword == PICTURE_KEYWORD) {
checkPicture(getText());
_type = PICTURE_PART;
}
if (lastKeyword == PERIOD) {
int level = Integer.parseInt(getText());
if (level == 66) {
_type = RENAMES_LEVEL;
} else if (level == 88) {
_type = CONDITION_LEVEL;
} else {
_type = DATA_ITEM_LEVEL;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "INT"
// $ANTLR start "SIGNED_INT"
public final void mSIGNED_INT() throws RecognitionException {
try {
int _type = SIGNED_INT;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:300:5: ( ( '+' | '-' ) ( '0' .. '9' )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:300:7: ( '+' | '-' ) ( '0' .. '9' )+
{
if ( input.LA(1)=='+'||input.LA(1)=='-' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
// com\\legstar\\cobol\\CobolStructureLexer.g:300:19: ( '0' .. '9' )+
int cnt2=0;
loop2:
while (true) {
int alt2=2;
int LA2_0 = input.LA(1);
if ( ((LA2_0 >= '0' && LA2_0 <= '9')) ) {
alt2=1;
}
switch (alt2) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt2 >= 1 ) break loop2;
EarlyExitException eee = new EarlyExitException(2, input);
throw eee;
}
cnt2++;
}
if (lastKeyword == PICTURE_KEYWORD) {
checkPicture(getText());
_type = PICTURE_PART;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SIGNED_INT"
// $ANTLR start "FLOAT_PART2"
public final void mFLOAT_PART2() throws RecognitionException {
try {
int _type = FLOAT_PART2;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:317:5: ( ( '0' .. '9' )+ 'E' ( '+' | '-' )? ( '0' .. '9' )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:317:7: ( '0' .. '9' )+ 'E' ( '+' | '-' )? ( '0' .. '9' )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:317:7: ( '0' .. '9' )+
int cnt3=0;
loop3:
while (true) {
int alt3=2;
int LA3_0 = input.LA(1);
if ( ((LA3_0 >= '0' && LA3_0 <= '9')) ) {
alt3=1;
}
switch (alt3) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt3 >= 1 ) break loop3;
EarlyExitException eee = new EarlyExitException(3, input);
throw eee;
}
cnt3++;
}
match('E');
// com\\legstar\\cobol\\CobolStructureLexer.g:317:21: ( '+' | '-' )?
int alt4=2;
int LA4_0 = input.LA(1);
if ( (LA4_0=='+'||LA4_0=='-') ) {
alt4=1;
}
switch (alt4) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( input.LA(1)=='+'||input.LA(1)=='-' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
}
// com\\legstar\\cobol\\CobolStructureLexer.g:317:34: ( '0' .. '9' )+
int cnt5=0;
loop5:
while (true) {
int alt5=2;
int LA5_0 = input.LA(1);
if ( ((LA5_0 >= '0' && LA5_0 <= '9')) ) {
alt5=1;
}
switch (alt5) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt5 >= 1 ) break loop5;
EarlyExitException eee = new EarlyExitException(5, input);
throw eee;
}
cnt5++;
}
if (lastKeyword == PICTURE_KEYWORD) {
checkPicture(getText());
_type = PICTURE_PART;
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "FLOAT_PART2"
// $ANTLR start "DATE_PATTERN"
public final void mDATE_PATTERN() throws RecognitionException {
try {
int _type = DATE_PATTERN;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:335:5: ( ( 'X' | 'Y' )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:335:7: ( 'X' | 'Y' )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:335:7: ( 'X' | 'Y' )+
int cnt6=0;
loop6:
while (true) {
int alt6=2;
int LA6_0 = input.LA(1);
if ( ((LA6_0 >= 'X' && LA6_0 <= 'Y')) ) {
alt6=1;
}
switch (alt6) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= 'X' && input.LA(1) <= 'Y') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt6 >= 1 ) break loop6;
EarlyExitException eee = new EarlyExitException(6, input);
throw eee;
}
cnt6++;
}
if (lastKeyword != DATE_FORMAT_KEYWORD) {
if (lastKeyword == PICTURE_KEYWORD) {
checkPicture(getText());
_type = PICTURE_PART;
} else {
_type = DATA_NAME;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DATE_PATTERN"
// $ANTLR start "DATA_NAME"
public final void mDATA_NAME() throws RecognitionException {
try {
int _type = DATA_NAME;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:357:5: ( ( LETTER | '0' .. '9' ) ( LETTER | '0' .. '9' | '-' | '_' )* )
// com\\legstar\\cobol\\CobolStructureLexer.g:357:7: ( LETTER | '0' .. '9' ) ( LETTER | '0' .. '9' | '-' | '_' )*
{
if ( (input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
// com\\legstar\\cobol\\CobolStructureLexer.g:357:25: ( LETTER | '0' .. '9' | '-' | '_' )*
loop7:
while (true) {
int alt7=2;
int LA7_0 = input.LA(1);
if ( (LA7_0=='-'||(LA7_0 >= '0' && LA7_0 <= '9')||(LA7_0 >= 'A' && LA7_0 <= 'Z')||LA7_0=='_'||(LA7_0 >= 'a' && LA7_0 <= 'z')) ) {
alt7=1;
}
switch (alt7) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( input.LA(1)=='-'||(input.LA(1) >= '0' && input.LA(1) <= '9')||(input.LA(1) >= 'A' && input.LA(1) <= 'Z')||input.LA(1)=='_'||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop7;
}
}
_type = matchKeywords(getText(), _type);
if (_type == DATA_NAME) {
if (lastKeyword == PICTURE_KEYWORD) {
checkPicture(getText());
_type = PICTURE_PART;
}
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DATA_NAME"
// $ANTLR start "PICTURE_PART"
public final void mPICTURE_PART() throws RecognitionException {
try {
int _type = PICTURE_PART;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:378:5: ( ( PICTURE_CHAR )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:378:7: ( PICTURE_CHAR )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:378:7: ( PICTURE_CHAR )+
int cnt8=0;
loop8:
while (true) {
int alt8=2;
int LA8_0 = input.LA(1);
if ( ((LA8_0 >= '\u0000' && LA8_0 <= '\b')||(LA8_0 >= '\u000B' && LA8_0 <= '\f')||(LA8_0 >= '\u000E' && LA8_0 <= '\u001F')||LA8_0=='!'||(LA8_0 >= '#' && LA8_0 <= '&')||(LA8_0 >= '(' && LA8_0 <= '-')||(LA8_0 >= '/' && LA8_0 <= '\uFFFF')) ) {
alt8=1;
}
switch (alt8) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '\b')||(input.LA(1) >= '\u000B' && input.LA(1) <= '\f')||(input.LA(1) >= '\u000E' && input.LA(1) <= '\u001F')||input.LA(1)=='!'||(input.LA(1) >= '#' && input.LA(1) <= '&')||(input.LA(1) >= '(' && input.LA(1) <= '-')||(input.LA(1) >= '/' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt8 >= 1 ) break loop8;
EarlyExitException eee = new EarlyExitException(8, input);
throw eee;
}
cnt8++;
}
if (lastKeyword != PICTURE_KEYWORD) {
checkDataName(getText());
_type = DATA_NAME;
} else {
checkPicture(getText());
}
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PICTURE_PART"
// $ANTLR start "PICTURE_CHAR"
public final void mPICTURE_CHAR() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:399:5: (~ ( QUOTE | APOST | SPACE | '\\r' | '\\n' | '.' ) )
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= '\u0000' && input.LA(1) <= '\b')||(input.LA(1) >= '\u000B' && input.LA(1) <= '\f')||(input.LA(1) >= '\u000E' && input.LA(1) <= '\u001F')||input.LA(1)=='!'||(input.LA(1) >= '#' && input.LA(1) <= '&')||(input.LA(1) >= '(' && input.LA(1) <= '-')||(input.LA(1) >= '/' && input.LA(1) <= '\uFFFF') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "PICTURE_CHAR"
// $ANTLR start "ALPHANUM_LITERAL_STRING"
public final void mALPHANUM_LITERAL_STRING() throws RecognitionException {
try {
int _type = ALPHANUM_LITERAL_STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:414:5: ( ( ALPHANUM_LITERAL_FRAGMENT )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:414:9: ( ALPHANUM_LITERAL_FRAGMENT )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:414:9: ( ALPHANUM_LITERAL_FRAGMENT )+
int cnt9=0;
loop9:
while (true) {
int alt9=2;
int LA9_0 = input.LA(1);
if ( (LA9_0=='\"'||LA9_0=='\'') ) {
alt9=1;
}
switch (alt9) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:414:9: ALPHANUM_LITERAL_FRAGMENT
{
mALPHANUM_LITERAL_FRAGMENT();
}
break;
default :
if ( cnt9 >= 1 ) break loop9;
EarlyExitException eee = new EarlyExitException(9, input);
throw eee;
}
cnt9++;
}
setText(getText().replaceAll("(\\r)?\\n(\\s)*\\-(\\s)*(\"|\')",""));
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ALPHANUM_LITERAL_STRING"
// $ANTLR start "ALPHANUM_LITERAL_FRAGMENT"
public final void mALPHANUM_LITERAL_FRAGMENT() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:420:5: ( QUOTE ( options {greedy=false; } : . )* ( QUOTE | CONTINUED_ALPHANUM_LITERAL_FRAGMENT ) | APOST ( options {greedy=false; } : . )* ( APOST | CONTINUED_ALPHANUM_LITERAL_FRAGMENT ) )
int alt14=2;
int LA14_0 = input.LA(1);
if ( (LA14_0=='\"') ) {
alt14=1;
}
else if ( (LA14_0=='\'') ) {
alt14=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 14, 0, input);
throw nvae;
}
switch (alt14) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:420:9: QUOTE ( options {greedy=false; } : . )* ( QUOTE | CONTINUED_ALPHANUM_LITERAL_FRAGMENT )
{
mQUOTE();
// com\\legstar\\cobol\\CobolStructureLexer.g:420:15: ( options {greedy=false; } : . )*
loop10:
while (true) {
int alt10=2;
alt10 = dfa10.predict(input);
switch (alt10) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:420:42: .
{
matchAny();
}
break;
default :
break loop10;
}
}
// com\\legstar\\cobol\\CobolStructureLexer.g:420:46: ( QUOTE | CONTINUED_ALPHANUM_LITERAL_FRAGMENT )
int alt11=2;
int LA11_0 = input.LA(1);
if ( (LA11_0=='\"') ) {
alt11=1;
}
else if ( (LA11_0=='\n'||LA11_0=='\r') ) {
alt11=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 11, 0, input);
throw nvae;
}
switch (alt11) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:420:48: QUOTE
{
mQUOTE();
}
break;
case 2 :
// com\\legstar\\cobol\\CobolStructureLexer.g:420:56: CONTINUED_ALPHANUM_LITERAL_FRAGMENT
{
mCONTINUED_ALPHANUM_LITERAL_FRAGMENT();
}
break;
}
}
break;
case 2 :
// com\\legstar\\cobol\\CobolStructureLexer.g:421:9: APOST ( options {greedy=false; } : . )* ( APOST | CONTINUED_ALPHANUM_LITERAL_FRAGMENT )
{
mAPOST();
// com\\legstar\\cobol\\CobolStructureLexer.g:421:15: ( options {greedy=false; } : . )*
loop12:
while (true) {
int alt12=2;
alt12 = dfa12.predict(input);
switch (alt12) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:421:42: .
{
matchAny();
}
break;
default :
break loop12;
}
}
// com\\legstar\\cobol\\CobolStructureLexer.g:421:46: ( APOST | CONTINUED_ALPHANUM_LITERAL_FRAGMENT )
int alt13=2;
int LA13_0 = input.LA(1);
if ( (LA13_0=='\'') ) {
alt13=1;
}
else if ( (LA13_0=='\n'||LA13_0=='\r') ) {
alt13=2;
}
else {
NoViableAltException nvae =
new NoViableAltException("", 13, 0, input);
throw nvae;
}
switch (alt13) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:421:48: APOST
{
mAPOST();
}
break;
case 2 :
// com\\legstar\\cobol\\CobolStructureLexer.g:421:56: CONTINUED_ALPHANUM_LITERAL_FRAGMENT
{
mCONTINUED_ALPHANUM_LITERAL_FRAGMENT();
}
break;
}
}
break;
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ALPHANUM_LITERAL_FRAGMENT"
// $ANTLR start "CONTINUED_ALPHANUM_LITERAL_FRAGMENT"
public final void mCONTINUED_ALPHANUM_LITERAL_FRAGMENT() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:426:3: ( ( ( '\\r' )? '\\n' ) ( SPACE )+ CONTINUATION_CHAR ( SPACE )* ( ALPHANUM_LITERAL_FRAGMENT )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:426:6: ( ( '\\r' )? '\\n' ) ( SPACE )+ CONTINUATION_CHAR ( SPACE )* ( ALPHANUM_LITERAL_FRAGMENT )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:426:6: ( ( '\\r' )? '\\n' )
// com\\legstar\\cobol\\CobolStructureLexer.g:426:7: ( '\\r' )? '\\n'
{
// com\\legstar\\cobol\\CobolStructureLexer.g:426:7: ( '\\r' )?
int alt15=2;
int LA15_0 = input.LA(1);
if ( (LA15_0=='\r') ) {
alt15=1;
}
switch (alt15) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:426:7: '\\r'
{
match('\r');
}
break;
}
match('\n');
}
// com\\legstar\\cobol\\CobolStructureLexer.g:426:19: ( SPACE )+
int cnt16=0;
loop16:
while (true) {
int alt16=2;
int LA16_0 = input.LA(1);
if ( (LA16_0=='\t'||LA16_0==' ') ) {
alt16=1;
}
switch (alt16) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( input.LA(1)=='\t'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt16 >= 1 ) break loop16;
EarlyExitException eee = new EarlyExitException(16, input);
throw eee;
}
cnt16++;
}
mCONTINUATION_CHAR();
// com\\legstar\\cobol\\CobolStructureLexer.g:426:44: ( SPACE )*
loop17:
while (true) {
int alt17=2;
int LA17_0 = input.LA(1);
if ( (LA17_0=='\t'||LA17_0==' ') ) {
alt17=1;
}
switch (alt17) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( input.LA(1)=='\t'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
break loop17;
}
}
// com\\legstar\\cobol\\CobolStructureLexer.g:426:51: ( ALPHANUM_LITERAL_FRAGMENT )+
int cnt18=0;
loop18:
while (true) {
int alt18=2;
int LA18_0 = input.LA(1);
if ( (LA18_0=='\"'||LA18_0=='\'') ) {
alt18=1;
}
switch (alt18) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:426:51: ALPHANUM_LITERAL_FRAGMENT
{
mALPHANUM_LITERAL_FRAGMENT();
}
break;
default :
if ( cnt18 >= 1 ) break loop18;
EarlyExitException eee = new EarlyExitException(18, input);
throw eee;
}
cnt18++;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CONTINUED_ALPHANUM_LITERAL_FRAGMENT"
// $ANTLR start "CONTINUATION_CHAR"
public final void mCONTINUATION_CHAR() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:431:5: ({...}? => '-' )
// com\\legstar\\cobol\\CobolStructureLexer.g:431:9: {...}? => '-'
{
if ( !((getCharPositionInLine() == 6)) ) {
throw new FailedPredicateException(input, "CONTINUATION_CHAR", "getCharPositionInLine() == 6");
}
match('-');
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "CONTINUATION_CHAR"
// $ANTLR start "HEX_LITERAL_STRING"
public final void mHEX_LITERAL_STRING() throws RecognitionException {
try {
int _type = HEX_LITERAL_STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:438:5: ( 'X' ALPHANUM_LITERAL_STRING )
// com\\legstar\\cobol\\CobolStructureLexer.g:438:9: 'X' ALPHANUM_LITERAL_STRING
{
match('X');
mALPHANUM_LITERAL_STRING();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "HEX_LITERAL_STRING"
// $ANTLR start "ZERO_LITERAL_STRING"
public final void mZERO_LITERAL_STRING() throws RecognitionException {
try {
int _type = ZERO_LITERAL_STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:445:5: ( 'Z' ALPHANUM_LITERAL_STRING )
// com\\legstar\\cobol\\CobolStructureLexer.g:445:9: 'Z' ALPHANUM_LITERAL_STRING
{
match('Z');
mALPHANUM_LITERAL_STRING();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "ZERO_LITERAL_STRING"
// $ANTLR start "DBCS_LITERAL_STRING"
public final void mDBCS_LITERAL_STRING() throws RecognitionException {
try {
int _type = DBCS_LITERAL_STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:452:5: ( 'G' ALPHANUM_LITERAL_STRING )
// com\\legstar\\cobol\\CobolStructureLexer.g:452:9: 'G' ALPHANUM_LITERAL_STRING
{
match('G');
mALPHANUM_LITERAL_STRING();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "DBCS_LITERAL_STRING"
// $ANTLR start "NATIONAL_LITERAL_STRING"
public final void mNATIONAL_LITERAL_STRING() throws RecognitionException {
try {
int _type = NATIONAL_LITERAL_STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:459:5: ( 'N' ALPHANUM_LITERAL_STRING )
// com\\legstar\\cobol\\CobolStructureLexer.g:459:9: 'N' ALPHANUM_LITERAL_STRING
{
match('N');
mALPHANUM_LITERAL_STRING();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NATIONAL_LITERAL_STRING"
// $ANTLR start "NATIONAL_HEX_LITERAL_STRING"
public final void mNATIONAL_HEX_LITERAL_STRING() throws RecognitionException {
try {
int _type = NATIONAL_HEX_LITERAL_STRING;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:466:5: ( 'NX' ALPHANUM_LITERAL_STRING )
// com\\legstar\\cobol\\CobolStructureLexer.g:466:9: 'NX' ALPHANUM_LITERAL_STRING
{
match("NX");
mALPHANUM_LITERAL_STRING();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NATIONAL_HEX_LITERAL_STRING"
// $ANTLR start "WHITESPACE"
public final void mWHITESPACE() throws RecognitionException {
try {
int _type = WHITESPACE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:474:5: ( ( SPACE )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:474:9: ( SPACE )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:474:9: ( SPACE )+
int cnt19=0;
loop19:
while (true) {
int alt19=2;
int LA19_0 = input.LA(1);
if ( (LA19_0=='\t'||LA19_0==' ') ) {
alt19=1;
}
switch (alt19) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( input.LA(1)=='\t'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
break;
default :
if ( cnt19 >= 1 ) break loop19;
EarlyExitException eee = new EarlyExitException(19, input);
throw eee;
}
cnt19++;
}
skip();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "WHITESPACE"
// $ANTLR start "NEWLINE"
public final void mNEWLINE() throws RecognitionException {
try {
int _type = NEWLINE;
int _channel = DEFAULT_TOKEN_CHANNEL;
// com\\legstar\\cobol\\CobolStructureLexer.g:481:5: ( ( ( '\\r' )? '\\n' )+ )
// com\\legstar\\cobol\\CobolStructureLexer.g:481:9: ( ( '\\r' )? '\\n' )+
{
// com\\legstar\\cobol\\CobolStructureLexer.g:481:9: ( ( '\\r' )? '\\n' )+
int cnt21=0;
loop21:
while (true) {
int alt21=2;
int LA21_0 = input.LA(1);
if ( (LA21_0=='\n'||LA21_0=='\r') ) {
alt21=1;
}
switch (alt21) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:481:10: ( '\\r' )? '\\n'
{
// com\\legstar\\cobol\\CobolStructureLexer.g:481:10: ( '\\r' )?
int alt20=2;
int LA20_0 = input.LA(1);
if ( (LA20_0=='\r') ) {
alt20=1;
}
switch (alt20) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:481:10: '\\r'
{
match('\r');
}
break;
}
match('\n');
}
break;
default :
if ( cnt21 >= 1 ) break loop21;
EarlyExitException eee = new EarlyExitException(21, input);
throw eee;
}
cnt21++;
}
skip();
}
state.type = _type;
state.channel = _channel;
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "NEWLINE"
// $ANTLR start "LETTER"
public final void mLETTER() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:488:21: ( 'A' .. 'Z' | 'a' .. 'z' )
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( (input.LA(1) >= 'A' && input.LA(1) <= 'Z')||(input.LA(1) >= 'a' && input.LA(1) <= 'z') ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "LETTER"
// $ANTLR start "SPACE"
public final void mSPACE() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:489:21: ( ' ' | '\\t' )
// com\\legstar\\cobol\\CobolStructureLexer.g:
{
if ( input.LA(1)=='\t'||input.LA(1)==' ' ) {
input.consume();
}
else {
MismatchedSetException mse = new MismatchedSetException(null,input);
recover(mse);
throw mse;
}
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "SPACE"
// $ANTLR start "QUOTE"
public final void mQUOTE() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:490:21: ( '\"' )
// com\\legstar\\cobol\\CobolStructureLexer.g:490:23: '\"'
{
match('\"');
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "QUOTE"
// $ANTLR start "APOST"
public final void mAPOST() throws RecognitionException {
try {
// com\\legstar\\cobol\\CobolStructureLexer.g:491:21: ( '\\'' )
// com\\legstar\\cobol\\CobolStructureLexer.g:491:23: '\\''
{
match('\'');
}
}
finally {
// do for sure before leaving
}
}
// $ANTLR end "APOST"
@Override
public void mTokens() throws RecognitionException {
// com\\legstar\\cobol\\CobolStructureLexer.g:1:8: ( PERIOD | INT | SIGNED_INT | FLOAT_PART2 | DATE_PATTERN | DATA_NAME | PICTURE_PART | ALPHANUM_LITERAL_STRING | HEX_LITERAL_STRING | ZERO_LITERAL_STRING | DBCS_LITERAL_STRING | NATIONAL_LITERAL_STRING | NATIONAL_HEX_LITERAL_STRING | WHITESPACE | NEWLINE )
int alt22=15;
alt22 = dfa22.predict(input);
switch (alt22) {
case 1 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:10: PERIOD
{
mPERIOD();
}
break;
case 2 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:17: INT
{
mINT();
}
break;
case 3 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:21: SIGNED_INT
{
mSIGNED_INT();
}
break;
case 4 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:32: FLOAT_PART2
{
mFLOAT_PART2();
}
break;
case 5 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:44: DATE_PATTERN
{
mDATE_PATTERN();
}
break;
case 6 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:57: DATA_NAME
{
mDATA_NAME();
}
break;
case 7 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:67: PICTURE_PART
{
mPICTURE_PART();
}
break;
case 8 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:80: ALPHANUM_LITERAL_STRING
{
mALPHANUM_LITERAL_STRING();
}
break;
case 9 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:104: HEX_LITERAL_STRING
{
mHEX_LITERAL_STRING();
}
break;
case 10 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:123: ZERO_LITERAL_STRING
{
mZERO_LITERAL_STRING();
}
break;
case 11 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:143: DBCS_LITERAL_STRING
{
mDBCS_LITERAL_STRING();
}
break;
case 12 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:163: NATIONAL_LITERAL_STRING
{
mNATIONAL_LITERAL_STRING();
}
break;
case 13 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:187: NATIONAL_HEX_LITERAL_STRING
{
mNATIONAL_HEX_LITERAL_STRING();
}
break;
case 14 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:215: WHITESPACE
{
mWHITESPACE();
}
break;
case 15 :
// com\\legstar\\cobol\\CobolStructureLexer.g:1:226: NEWLINE
{
mNEWLINE();
}
break;
}
}
protected DFA10 dfa10 = new DFA10(this);
protected DFA12 dfa12 = new DFA12(this);
protected DFA22 dfa22 = new DFA22(this);
static final String DFA10_eotS =
"\7\uffff";
static final String DFA10_eofS =
"\7\uffff";
static final String DFA10_minS =
"\1\0\2\uffff\1\0\1\uffff\1\0\1\uffff";
static final String DFA10_maxS =
"\1\uffff\2\uffff\1\uffff\1\uffff\1\uffff\1\uffff";
static final String DFA10_acceptS =
"\1\uffff\2\2\1\uffff\1\1\1\uffff\1\2";
static final String DFA10_specialS =
"\1\2\2\uffff\1\1\1\uffff\1\0\1\uffff}>";
static final String[] DFA10_transitionS = {
"\12\4\1\3\2\4\1\2\24\4\1\1\uffdd\4",
"",
"",
"\11\4\1\5\26\4\1\5\uffdf\4",
"",
"\11\4\1\5\26\4\1\5\14\4\1\6\uffd2\4",
""
};
static final short[] DFA10_eot = DFA.unpackEncodedString(DFA10_eotS);
static final short[] DFA10_eof = DFA.unpackEncodedString(DFA10_eofS);
static final char[] DFA10_min = DFA.unpackEncodedStringToUnsignedChars(DFA10_minS);
static final char[] DFA10_max = DFA.unpackEncodedStringToUnsignedChars(DFA10_maxS);
static final short[] DFA10_accept = DFA.unpackEncodedString(DFA10_acceptS);
static final short[] DFA10_special = DFA.unpackEncodedString(DFA10_specialS);
static final short[][] DFA10_transition;
static {
int numStates = DFA10_transitionS.length;
DFA10_transition = new short[numStates][];
for (int i=0; i= '\u0000' && LA10_5 <= '\b')||(LA10_5 >= '\n' && LA10_5 <= '\u001F')||(LA10_5 >= '!' && LA10_5 <= ',')||(LA10_5 >= '.' && LA10_5 <= '\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
case 1 :
int LA10_3 = input.LA(1);
s = -1;
if ( (LA10_3=='\t'||LA10_3==' ') ) {s = 5;}
else if ( ((LA10_3 >= '\u0000' && LA10_3 <= '\b')||(LA10_3 >= '\n' && LA10_3 <= '\u001F')||(LA10_3 >= '!' && LA10_3 <= '\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
case 2 :
int LA10_0 = input.LA(1);
s = -1;
if ( (LA10_0=='\"') ) {s = 1;}
else if ( (LA10_0=='\r') ) {s = 2;}
else if ( (LA10_0=='\n') ) {s = 3;}
else if ( ((LA10_0 >= '\u0000' && LA10_0 <= '\t')||(LA10_0 >= '\u000B' && LA10_0 <= '\f')||(LA10_0 >= '\u000E' && LA10_0 <= '!')||(LA10_0 >= '#' && LA10_0 <= '\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 10, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA12_eotS =
"\7\uffff";
static final String DFA12_eofS =
"\7\uffff";
static final String DFA12_minS =
"\1\0\2\uffff\1\0\1\uffff\1\0\1\uffff";
static final String DFA12_maxS =
"\1\uffff\2\uffff\1\uffff\1\uffff\1\uffff\1\uffff";
static final String DFA12_acceptS =
"\1\uffff\2\2\1\uffff\1\1\1\uffff\1\2";
static final String DFA12_specialS =
"\1\1\2\uffff\1\0\1\uffff\1\2\1\uffff}>";
static final String[] DFA12_transitionS = {
"\12\4\1\3\2\4\1\2\31\4\1\1\uffd8\4",
"",
"",
"\11\4\1\5\26\4\1\5\uffdf\4",
"",
"\11\4\1\5\26\4\1\5\14\4\1\6\uffd2\4",
""
};
static final short[] DFA12_eot = DFA.unpackEncodedString(DFA12_eotS);
static final short[] DFA12_eof = DFA.unpackEncodedString(DFA12_eofS);
static final char[] DFA12_min = DFA.unpackEncodedStringToUnsignedChars(DFA12_minS);
static final char[] DFA12_max = DFA.unpackEncodedStringToUnsignedChars(DFA12_maxS);
static final short[] DFA12_accept = DFA.unpackEncodedString(DFA12_acceptS);
static final short[] DFA12_special = DFA.unpackEncodedString(DFA12_specialS);
static final short[][] DFA12_transition;
static {
int numStates = DFA12_transitionS.length;
DFA12_transition = new short[numStates][];
for (int i=0; i= '\u0000' && LA12_3 <= '\b')||(LA12_3 >= '\n' && LA12_3 <= '\u001F')||(LA12_3 >= '!' && LA12_3 <= '\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
case 1 :
int LA12_0 = input.LA(1);
s = -1;
if ( (LA12_0=='\'') ) {s = 1;}
else if ( (LA12_0=='\r') ) {s = 2;}
else if ( (LA12_0=='\n') ) {s = 3;}
else if ( ((LA12_0 >= '\u0000' && LA12_0 <= '\t')||(LA12_0 >= '\u000B' && LA12_0 <= '\f')||(LA12_0 >= '\u000E' && LA12_0 <= '&')||(LA12_0 >= '(' && LA12_0 <= '\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
case 2 :
int LA12_5 = input.LA(1);
s = -1;
if ( (LA12_5=='-') ) {s = 6;}
else if ( (LA12_5=='\t'||LA12_5==' ') ) {s = 5;}
else if ( ((LA12_5 >= '\u0000' && LA12_5 <= '\b')||(LA12_5 >= '\n' && LA12_5 <= '\u001F')||(LA12_5 >= '!' && LA12_5 <= ',')||(LA12_5 >= '.' && LA12_5 <= '\uFFFF')) ) {s = 4;}
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 12, _s, input);
error(nvae);
throw nvae;
}
}
static final String DFA22_eotS =
"\2\uffff\1\16\1\6\1\23\1\26\2\uffff\1\23\3\26\3\uffff\1\16\2\26\1\36\1"+
"\uffff\1\23\4\uffff\1\26\1\uffff\1\26\1\40\1\6\3\uffff\1\40";
static final String DFA22_eofS =
"\42\uffff";
static final String DFA22_minS =
"\1\0\1\uffff\1\0\1\60\2\0\2\uffff\4\0\3\uffff\4\0\1\uffff\1\0\4\uffff"+
"\1\0\1\uffff\2\0\1\60\3\uffff\1\0";
static final String DFA22_maxS =
"\1\uffff\1\uffff\1\uffff\1\71\2\uffff\2\uffff\4\uffff\3\uffff\4\uffff"+
"\1\uffff\1\uffff\4\uffff\1\uffff\1\uffff\2\uffff\1\71\3\uffff\1\uffff";
static final String DFA22_acceptS =
"\1\uffff\1\1\4\uffff\1\7\1\10\4\uffff\1\16\1\17\1\2\4\uffff\1\5\1\uffff"+
"\1\11\1\6\1\12\1\13\1\uffff\1\14\3\uffff\1\3\1\15\1\4\1\uffff";
static final String DFA22_specialS =
"\1\2\1\uffff\1\20\1\uffff\1\3\1\4\2\uffff\1\14\1\11\1\5\1\7\3\uffff\1"+
"\1\1\13\1\0\1\17\1\uffff\1\12\4\uffff\1\16\1\uffff\1\10\1\15\4\uffff\1"+
"\6}>";
static final String[] DFA22_transitionS = {
"\11\6\1\14\1\15\2\6\1\15\22\6\1\14\1\6\1\7\4\6\1\7\3\6\1\3\1\6\1\3\1"+
"\1\1\6\12\2\7\6\6\13\1\11\6\13\1\12\11\13\1\4\1\10\1\5\6\6\32\13\uff85"+
"\6",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\17\7\6\4\21\1\20\25\21\4\6\1\21\1\6\32\21\uff85\6",
"\12\22",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\25\4\6\1\25\5\6\1\21\1"+
"\uffff\1\6\12\21\7\6\27\21\2\24\1\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\27\4\6\1\27\5\6\1\21\1"+
"\uffff\1\6\12\21\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\21\7\6\27\21\2\24\1\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\30\4\6\1\30\5\6\1\21\1"+
"\uffff\1\6\12\21\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\32\4\6\1\32\5\6\1\21\1"+
"\uffff\1\6\12\21\7\6\27\21\1\31\2\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\21\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"",
"",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\17\7\6\4\21\1\20\25\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\3\6\1"+
"\35\1\6\1\33\1\uffff\1\6\12\34\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\21\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\6\6\1"+
"\uffff\1\6\12\22\uffc6\6",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\21\7\6\27\21\2\24\1\21\4\6\1\21\1\6\32\21\uff85\6",
"",
"",
"",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\37\4\6\1\37\5\6\1\21\1"+
"\uffff\1\6\12\21\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\34\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\5\6\1"+
"\21\1\uffff\1\6\12\34\7\6\32\21\4\6\1\21\1\6\32\21\uff85\6",
"\12\41",
"",
"",
"",
"\11\6\2\uffff\2\6\1\uffff\22\6\1\uffff\1\6\1\uffff\4\6\1\uffff\6\6\1"+
"\uffff\1\6\12\41\uffc6\6"
};
static final short[] DFA22_eot = DFA.unpackEncodedString(DFA22_eotS);
static final short[] DFA22_eof = DFA.unpackEncodedString(DFA22_eofS);
static final char[] DFA22_min = DFA.unpackEncodedStringToUnsignedChars(DFA22_minS);
static final char[] DFA22_max = DFA.unpackEncodedStringToUnsignedChars(DFA22_maxS);
static final short[] DFA22_accept = DFA.unpackEncodedString(DFA22_acceptS);
static final short[] DFA22_special = DFA.unpackEncodedString(DFA22_specialS);
static final short[][] DFA22_transition;
static {
int numStates = DFA22_transitionS.length;
DFA22_transition = new short[numStates][];
for (int i=0; i= '0' && LA22_17 <= '9')||(LA22_17 >= 'A' && LA22_17 <= 'Z')||LA22_17=='_'||(LA22_17 >= 'a' && LA22_17 <= 'z')) ) {s = 17;}
else if ( ((LA22_17 >= '\u0000' && LA22_17 <= '\b')||(LA22_17 >= '\u000B' && LA22_17 <= '\f')||(LA22_17 >= '\u000E' && LA22_17 <= '\u001F')||LA22_17=='!'||(LA22_17 >= '#' && LA22_17 <= '&')||(LA22_17 >= '(' && LA22_17 <= ',')||LA22_17=='/'||(LA22_17 >= ':' && LA22_17 <= '@')||(LA22_17 >= '[' && LA22_17 <= '^')||LA22_17=='`'||(LA22_17 >= '{' && LA22_17 <= '\uFFFF')) ) {s = 6;}
else s = 22;
if ( s>=0 ) return s;
break;
case 1 :
int LA22_15 = input.LA(1);
s = -1;
if ( ((LA22_15 >= '0' && LA22_15 <= '9')) ) {s = 15;}
else if ( (LA22_15=='E') ) {s = 16;}
else if ( (LA22_15=='-'||(LA22_15 >= 'A' && LA22_15 <= 'D')||(LA22_15 >= 'F' && LA22_15 <= 'Z')||LA22_15=='_'||(LA22_15 >= 'a' && LA22_15 <= 'z')) ) {s = 17;}
else if ( ((LA22_15 >= '\u0000' && LA22_15 <= '\b')||(LA22_15 >= '\u000B' && LA22_15 <= '\f')||(LA22_15 >= '\u000E' && LA22_15 <= '\u001F')||LA22_15=='!'||(LA22_15 >= '#' && LA22_15 <= '&')||(LA22_15 >= '(' && LA22_15 <= ',')||LA22_15=='/'||(LA22_15 >= ':' && LA22_15 <= '@')||(LA22_15 >= '[' && LA22_15 <= '^')||LA22_15=='`'||(LA22_15 >= '{' && LA22_15 <= '\uFFFF')) ) {s = 6;}
else s = 14;
if ( s>=0 ) return s;
break;
case 2 :
int LA22_0 = input.LA(1);
s = -1;
if ( (LA22_0=='.') ) {s = 1;}
else if ( ((LA22_0 >= '0' && LA22_0 <= '9')) ) {s = 2;}
else if ( (LA22_0=='+'||LA22_0=='-') ) {s = 3;}
else if ( (LA22_0=='X') ) {s = 4;}
else if ( (LA22_0=='Z') ) {s = 5;}
else if ( ((LA22_0 >= '\u0000' && LA22_0 <= '\b')||(LA22_0 >= '\u000B' && LA22_0 <= '\f')||(LA22_0 >= '\u000E' && LA22_0 <= '\u001F')||LA22_0=='!'||(LA22_0 >= '#' && LA22_0 <= '&')||(LA22_0 >= '(' && LA22_0 <= '*')||LA22_0==','||LA22_0=='/'||(LA22_0 >= ':' && LA22_0 <= '@')||(LA22_0 >= '[' && LA22_0 <= '`')||(LA22_0 >= '{' && LA22_0 <= '\uFFFF')) ) {s = 6;}
else if ( (LA22_0=='\"'||LA22_0=='\'') ) {s = 7;}
else if ( (LA22_0=='Y') ) {s = 8;}
else if ( (LA22_0=='G') ) {s = 9;}
else if ( (LA22_0=='N') ) {s = 10;}
else if ( ((LA22_0 >= 'A' && LA22_0 <= 'F')||(LA22_0 >= 'H' && LA22_0 <= 'M')||(LA22_0 >= 'O' && LA22_0 <= 'W')||(LA22_0 >= 'a' && LA22_0 <= 'z')) ) {s = 11;}
else if ( (LA22_0=='\t'||LA22_0==' ') ) {s = 12;}
else if ( (LA22_0=='\n'||LA22_0=='\r') ) {s = 13;}
if ( s>=0 ) return s;
break;
case 3 :
int LA22_4 = input.LA(1);
s = -1;
if ( ((LA22_4 >= 'X' && LA22_4 <= 'Y')) ) {s = 20;}
else if ( (LA22_4=='-'||(LA22_4 >= '0' && LA22_4 <= '9')||(LA22_4 >= 'A' && LA22_4 <= 'W')||LA22_4=='Z'||LA22_4=='_'||(LA22_4 >= 'a' && LA22_4 <= 'z')) ) {s = 17;}
else if ( ((LA22_4 >= '\u0000' && LA22_4 <= '\b')||(LA22_4 >= '\u000B' && LA22_4 <= '\f')||(LA22_4 >= '\u000E' && LA22_4 <= '\u001F')||LA22_4=='!'||(LA22_4 >= '#' && LA22_4 <= '&')||(LA22_4 >= '(' && LA22_4 <= ',')||LA22_4=='/'||(LA22_4 >= ':' && LA22_4 <= '@')||(LA22_4 >= '[' && LA22_4 <= '^')||LA22_4=='`'||(LA22_4 >= '{' && LA22_4 <= '\uFFFF')) ) {s = 6;}
else if ( (LA22_4=='\"'||LA22_4=='\'') ) {s = 21;}
else s = 19;
if ( s>=0 ) return s;
break;
case 4 :
int LA22_5 = input.LA(1);
s = -1;
if ( (LA22_5=='-'||(LA22_5 >= '0' && LA22_5 <= '9')||(LA22_5 >= 'A' && LA22_5 <= 'Z')||LA22_5=='_'||(LA22_5 >= 'a' && LA22_5 <= 'z')) ) {s = 17;}
else if ( ((LA22_5 >= '\u0000' && LA22_5 <= '\b')||(LA22_5 >= '\u000B' && LA22_5 <= '\f')||(LA22_5 >= '\u000E' && LA22_5 <= '\u001F')||LA22_5=='!'||(LA22_5 >= '#' && LA22_5 <= '&')||(LA22_5 >= '(' && LA22_5 <= ',')||LA22_5=='/'||(LA22_5 >= ':' && LA22_5 <= '@')||(LA22_5 >= '[' && LA22_5 <= '^')||LA22_5=='`'||(LA22_5 >= '{' && LA22_5 <= '\uFFFF')) ) {s = 6;}
else if ( (LA22_5=='\"'||LA22_5=='\'') ) {s = 23;}
else s = 22;
if ( s>=0 ) return s;
break;
case 5 :
int LA22_10 = input.LA(1);
s = -1;
if ( (LA22_10=='X') ) {s = 25;}
else if ( (LA22_10=='-'||(LA22_10 >= '0' && LA22_10 <= '9')||(LA22_10 >= 'A' && LA22_10 <= 'W')||(LA22_10 >= 'Y' && LA22_10 <= 'Z')||LA22_10=='_'||(LA22_10 >= 'a' && LA22_10 <= 'z')) ) {s = 17;}
else if ( ((LA22_10 >= '\u0000' && LA22_10 <= '\b')||(LA22_10 >= '\u000B' && LA22_10 <= '\f')||(LA22_10 >= '\u000E' && LA22_10 <= '\u001F')||LA22_10=='!'||(LA22_10 >= '#' && LA22_10 <= '&')||(LA22_10 >= '(' && LA22_10 <= ',')||LA22_10=='/'||(LA22_10 >= ':' && LA22_10 <= '@')||(LA22_10 >= '[' && LA22_10 <= '^')||LA22_10=='`'||(LA22_10 >= '{' && LA22_10 <= '\uFFFF')) ) {s = 6;}
else if ( (LA22_10=='\"'||LA22_10=='\'') ) {s = 26;}
else s = 22;
if ( s>=0 ) return s;
break;
case 6 :
int LA22_33 = input.LA(1);
s = -1;
if ( ((LA22_33 >= '0' && LA22_33 <= '9')) ) {s = 33;}
else if ( ((LA22_33 >= '\u0000' && LA22_33 <= '\b')||(LA22_33 >= '\u000B' && LA22_33 <= '\f')||(LA22_33 >= '\u000E' && LA22_33 <= '\u001F')||LA22_33=='!'||(LA22_33 >= '#' && LA22_33 <= '&')||(LA22_33 >= '(' && LA22_33 <= '-')||LA22_33=='/'||(LA22_33 >= ':' && LA22_33 <= '\uFFFF')) ) {s = 6;}
else s = 32;
if ( s>=0 ) return s;
break;
case 7 :
int LA22_11 = input.LA(1);
s = -1;
if ( (LA22_11=='-'||(LA22_11 >= '0' && LA22_11 <= '9')||(LA22_11 >= 'A' && LA22_11 <= 'Z')||LA22_11=='_'||(LA22_11 >= 'a' && LA22_11 <= 'z')) ) {s = 17;}
else if ( ((LA22_11 >= '\u0000' && LA22_11 <= '\b')||(LA22_11 >= '\u000B' && LA22_11 <= '\f')||(LA22_11 >= '\u000E' && LA22_11 <= '\u001F')||LA22_11=='!'||(LA22_11 >= '#' && LA22_11 <= '&')||(LA22_11 >= '(' && LA22_11 <= ',')||LA22_11=='/'||(LA22_11 >= ':' && LA22_11 <= '@')||(LA22_11 >= '[' && LA22_11 <= '^')||LA22_11=='`'||(LA22_11 >= '{' && LA22_11 <= '\uFFFF')) ) {s = 6;}
else s = 22;
if ( s>=0 ) return s;
break;
case 8 :
int LA22_27 = input.LA(1);
s = -1;
if ( ((LA22_27 >= '0' && LA22_27 <= '9')) ) {s = 28;}
else if ( (LA22_27=='-'||(LA22_27 >= 'A' && LA22_27 <= 'Z')||LA22_27=='_'||(LA22_27 >= 'a' && LA22_27 <= 'z')) ) {s = 17;}
else if ( ((LA22_27 >= '\u0000' && LA22_27 <= '\b')||(LA22_27 >= '\u000B' && LA22_27 <= '\f')||(LA22_27 >= '\u000E' && LA22_27 <= '\u001F')||LA22_27=='!'||(LA22_27 >= '#' && LA22_27 <= '&')||(LA22_27 >= '(' && LA22_27 <= ',')||LA22_27=='/'||(LA22_27 >= ':' && LA22_27 <= '@')||(LA22_27 >= '[' && LA22_27 <= '^')||LA22_27=='`'||(LA22_27 >= '{' && LA22_27 <= '\uFFFF')) ) {s = 6;}
else s = 22;
if ( s>=0 ) return s;
break;
case 9 :
int LA22_9 = input.LA(1);
s = -1;
if ( (LA22_9=='-'||(LA22_9 >= '0' && LA22_9 <= '9')||(LA22_9 >= 'A' && LA22_9 <= 'Z')||LA22_9=='_'||(LA22_9 >= 'a' && LA22_9 <= 'z')) ) {s = 17;}
else if ( ((LA22_9 >= '\u0000' && LA22_9 <= '\b')||(LA22_9 >= '\u000B' && LA22_9 <= '\f')||(LA22_9 >= '\u000E' && LA22_9 <= '\u001F')||LA22_9=='!'||(LA22_9 >= '#' && LA22_9 <= '&')||(LA22_9 >= '(' && LA22_9 <= ',')||LA22_9=='/'||(LA22_9 >= ':' && LA22_9 <= '@')||(LA22_9 >= '[' && LA22_9 <= '^')||LA22_9=='`'||(LA22_9 >= '{' && LA22_9 <= '\uFFFF')) ) {s = 6;}
else if ( (LA22_9=='\"'||LA22_9=='\'') ) {s = 24;}
else s = 22;
if ( s>=0 ) return s;
break;
case 10 :
int LA22_20 = input.LA(1);
s = -1;
if ( ((LA22_20 >= 'X' && LA22_20 <= 'Y')) ) {s = 20;}
else if ( (LA22_20=='-'||(LA22_20 >= '0' && LA22_20 <= '9')||(LA22_20 >= 'A' && LA22_20 <= 'W')||LA22_20=='Z'||LA22_20=='_'||(LA22_20 >= 'a' && LA22_20 <= 'z')) ) {s = 17;}
else if ( ((LA22_20 >= '\u0000' && LA22_20 <= '\b')||(LA22_20 >= '\u000B' && LA22_20 <= '\f')||(LA22_20 >= '\u000E' && LA22_20 <= '\u001F')||LA22_20=='!'||(LA22_20 >= '#' && LA22_20 <= '&')||(LA22_20 >= '(' && LA22_20 <= ',')||LA22_20=='/'||(LA22_20 >= ':' && LA22_20 <= '@')||(LA22_20 >= '[' && LA22_20 <= '^')||LA22_20=='`'||(LA22_20 >= '{' && LA22_20 <= '\uFFFF')) ) {s = 6;}
else s = 19;
if ( s>=0 ) return s;
break;
case 11 :
int LA22_16 = input.LA(1);
s = -1;
if ( (LA22_16=='-') ) {s = 27;}
else if ( ((LA22_16 >= '0' && LA22_16 <= '9')) ) {s = 28;}
else if ( (LA22_16=='+') ) {s = 29;}
else if ( ((LA22_16 >= 'A' && LA22_16 <= 'Z')||LA22_16=='_'||(LA22_16 >= 'a' && LA22_16 <= 'z')) ) {s = 17;}
else if ( ((LA22_16 >= '\u0000' && LA22_16 <= '\b')||(LA22_16 >= '\u000B' && LA22_16 <= '\f')||(LA22_16 >= '\u000E' && LA22_16 <= '\u001F')||LA22_16=='!'||(LA22_16 >= '#' && LA22_16 <= '&')||(LA22_16 >= '(' && LA22_16 <= '*')||LA22_16==','||LA22_16=='/'||(LA22_16 >= ':' && LA22_16 <= '@')||(LA22_16 >= '[' && LA22_16 <= '^')||LA22_16=='`'||(LA22_16 >= '{' && LA22_16 <= '\uFFFF')) ) {s = 6;}
else s = 22;
if ( s>=0 ) return s;
break;
case 12 :
int LA22_8 = input.LA(1);
s = -1;
if ( ((LA22_8 >= 'X' && LA22_8 <= 'Y')) ) {s = 20;}
else if ( (LA22_8=='-'||(LA22_8 >= '0' && LA22_8 <= '9')||(LA22_8 >= 'A' && LA22_8 <= 'W')||LA22_8=='Z'||LA22_8=='_'||(LA22_8 >= 'a' && LA22_8 <= 'z')) ) {s = 17;}
else if ( ((LA22_8 >= '\u0000' && LA22_8 <= '\b')||(LA22_8 >= '\u000B' && LA22_8 <= '\f')||(LA22_8 >= '\u000E' && LA22_8 <= '\u001F')||LA22_8=='!'||(LA22_8 >= '#' && LA22_8 <= '&')||(LA22_8 >= '(' && LA22_8 <= ',')||LA22_8=='/'||(LA22_8 >= ':' && LA22_8 <= '@')||(LA22_8 >= '[' && LA22_8 <= '^')||LA22_8=='`'||(LA22_8 >= '{' && LA22_8 <= '\uFFFF')) ) {s = 6;}
else s = 19;
if ( s>=0 ) return s;
break;
case 13 :
int LA22_28 = input.LA(1);
s = -1;
if ( ((LA22_28 >= '0' && LA22_28 <= '9')) ) {s = 28;}
else if ( (LA22_28=='-'||(LA22_28 >= 'A' && LA22_28 <= 'Z')||LA22_28=='_'||(LA22_28 >= 'a' && LA22_28 <= 'z')) ) {s = 17;}
else if ( ((LA22_28 >= '\u0000' && LA22_28 <= '\b')||(LA22_28 >= '\u000B' && LA22_28 <= '\f')||(LA22_28 >= '\u000E' && LA22_28 <= '\u001F')||LA22_28=='!'||(LA22_28 >= '#' && LA22_28 <= '&')||(LA22_28 >= '(' && LA22_28 <= ',')||LA22_28=='/'||(LA22_28 >= ':' && LA22_28 <= '@')||(LA22_28 >= '[' && LA22_28 <= '^')||LA22_28=='`'||(LA22_28 >= '{' && LA22_28 <= '\uFFFF')) ) {s = 6;}
else s = 32;
if ( s>=0 ) return s;
break;
case 14 :
int LA22_25 = input.LA(1);
s = -1;
if ( (LA22_25=='\"'||LA22_25=='\'') ) {s = 31;}
else if ( (LA22_25=='-'||(LA22_25 >= '0' && LA22_25 <= '9')||(LA22_25 >= 'A' && LA22_25 <= 'Z')||LA22_25=='_'||(LA22_25 >= 'a' && LA22_25 <= 'z')) ) {s = 17;}
else if ( ((LA22_25 >= '\u0000' && LA22_25 <= '\b')||(LA22_25 >= '\u000B' && LA22_25 <= '\f')||(LA22_25 >= '\u000E' && LA22_25 <= '\u001F')||LA22_25=='!'||(LA22_25 >= '#' && LA22_25 <= '&')||(LA22_25 >= '(' && LA22_25 <= ',')||LA22_25=='/'||(LA22_25 >= ':' && LA22_25 <= '@')||(LA22_25 >= '[' && LA22_25 <= '^')||LA22_25=='`'||(LA22_25 >= '{' && LA22_25 <= '\uFFFF')) ) {s = 6;}
else s = 22;
if ( s>=0 ) return s;
break;
case 15 :
int LA22_18 = input.LA(1);
s = -1;
if ( ((LA22_18 >= '0' && LA22_18 <= '9')) ) {s = 18;}
else if ( ((LA22_18 >= '\u0000' && LA22_18 <= '\b')||(LA22_18 >= '\u000B' && LA22_18 <= '\f')||(LA22_18 >= '\u000E' && LA22_18 <= '\u001F')||LA22_18=='!'||(LA22_18 >= '#' && LA22_18 <= '&')||(LA22_18 >= '(' && LA22_18 <= '-')||LA22_18=='/'||(LA22_18 >= ':' && LA22_18 <= '\uFFFF')) ) {s = 6;}
else s = 30;
if ( s>=0 ) return s;
break;
case 16 :
int LA22_2 = input.LA(1);
s = -1;
if ( ((LA22_2 >= '0' && LA22_2 <= '9')) ) {s = 15;}
else if ( (LA22_2=='E') ) {s = 16;}
else if ( (LA22_2=='-'||(LA22_2 >= 'A' && LA22_2 <= 'D')||(LA22_2 >= 'F' && LA22_2 <= 'Z')||LA22_2=='_'||(LA22_2 >= 'a' && LA22_2 <= 'z')) ) {s = 17;}
else if ( ((LA22_2 >= '\u0000' && LA22_2 <= '\b')||(LA22_2 >= '\u000B' && LA22_2 <= '\f')||(LA22_2 >= '\u000E' && LA22_2 <= '\u001F')||LA22_2=='!'||(LA22_2 >= '#' && LA22_2 <= '&')||(LA22_2 >= '(' && LA22_2 <= ',')||LA22_2=='/'||(LA22_2 >= ':' && LA22_2 <= '@')||(LA22_2 >= '[' && LA22_2 <= '^')||LA22_2=='`'||(LA22_2 >= '{' && LA22_2 <= '\uFFFF')) ) {s = 6;}
else s = 14;
if ( s>=0 ) return s;
break;
}
NoViableAltException nvae =
new NoViableAltException(getDescription(), 22, _s, input);
error(nvae);
throw nvae;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy