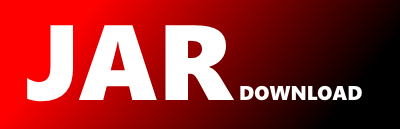
vlc.coxb-bind-complex.vm Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of legstar-distribution
Show all versions of legstar-distribution
Used to create a single distribution for the entire LegStar project.
The newest version!
#**
Complex binding class Velocity Template.
@author Fady
@version 1.2
*#
## ==================================================================
## Complex element binding code
##
#parse("vlc/coxb-bind-common-imports.vm")
package ${coxbContext.getCoxbPackageName()};
import com.legstar.coxb.ICobolBinding;
import com.legstar.coxb.common.CComplexBinding;
#foreach($importType in $importTypes)
import ${importType};
#end
#if (!$importTypes.contains("com.legstar.coxb.ICobolComplexBinding"))
import com.legstar.coxb.ICobolComplexBinding;
#end
import com.legstar.coxb.host.HostException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
## Determine the value Object type
#set ($valueObjectType = $coxbHelper.getBoundTypeName($binding))
import ${binding.getValueObjectClassName()};
#if (${binding.getValueObjectsFactoryClassName()})
import ${binding.getValueObjectsFactoryClassName()};
#end
/**
* LegStar Binding for Complex element :
* $valueObjectType.
*
* This class was generated by ${generatorName}.
*/
public class $binding-class-name
extends CComplexBinding {
/** Value object to which this cobol complex element is bound. */
private $valueObjectType mValueObject;
/** Indicates that the associated Value object just came from the constructor
* and doesn't need to be recreated. */
private boolean mUnusedValueObject = false;
/** Maximum host bytes size for this complex object. */
private static final int BYTE_LENGTH = ${binding.getByteLength()};
#foreach ($child in $binding.childrenList)
#set ($childObjectType = $coxbHelper.getBoundTypeName($child))
#if($child.isBound())
/** Child bound to value object property ${child.jaxbName}(${childObjectType}). */
#else
/** Unbound child. */
#end
public $coxbHelper.getBindingInterfaceName($child) $coxbHelper.getFieldName($child);
#if ($coxbHelper.getGenericType($child).equals("complexArray"))
/** Binding item for complex array binding ${childObjectType}. */
public ICobolComplexBinding $coxbHelper.getFieldName($child)Item;
#end
#end
/** Logger. */
private final Log _log = LogFactory.getLog(getClass());
#if(${hasSimpleChilds} == true)
/** Binding factory. */
private static final ICobolBindingFactory BF
= CobolBindingFactory.getBindingFactory();
#end
#if (${binding.getValueObjectsFactoryClassName()})
/** Static reference to Value object factory to be used as default. */
private static final ObjectFactory JF = new ObjectFactory();
/** Current Value object factory (Defaults to the static one but can be
* changed). */
private ObjectFactory mValueObjectFactory = JF;
#end
/**
* Constructor for a root Complex element without a bound Value object.
*/
public ${binding-class-name}() {
this(null);
}
/**
* Constructor for a root Complex element with a bound Value object.
*
* @param valueObject the concrete Value object instance bound to this
* complex element
*/
public ${binding-class-name}(
final $valueObjectType valueObject) {
#if($helper.isEmpty(${complexBinding.bindingName}) == true)
#set($bindingName = "")
#else
#set($bindingName = ${complexBinding.bindingName})
#end
#if($helper.isEmpty(${complexBinding.jaxbName}) == true)
#set($fieldName = "")
#else
#set($fieldName = ${complexBinding.jaxbName})
#end
this("${bindingName}", "${fieldName}", null, valueObject);
}
/**
* Constructor for a Complex element as a child of another element and
* an associated Value object.
*
* @param bindingName the identifier for this binding
* @param fieldName field name in parent Value object
* @param valueObject the concrete Value object instance bound to this
* complex element
* @param parentBinding a reference to the parent binding
*/
public ${binding-class-name}(
final String bindingName,
final String fieldName,
final ICobolComplexBinding parentBinding,
final $valueObjectType valueObject) {
super(bindingName, fieldName, ${valueObjectType}.class, null, parentBinding);
mValueObject = valueObject;
if (mValueObject != null) {
mUnusedValueObject = true;
}
initChildren();
setByteLength(BYTE_LENGTH);
}
/** Creates a binding property for each child. */
private void initChildren() {
if (_log.isDebugEnabled()) {
_log.debug("Initializing started");
}
/* Create binding children instances */
#set($parent-binding = "this")
#set($childrenList = $binding.childrenList)
#parse("vlc/coxb-bind-init-children.vm")
/* Add children to children list */
#foreach ($child in $binding.childrenList)
#if($child.isBound())
getChildrenList().add(${coxbHelper.getFieldName($child)});
#else
#if($child.isODOObject())
##
## An unbound ODOObject is a dynamic counter
storeCounter(${coxbHelper.getFieldName($child)});
#else
getChildrenList().add(${coxbHelper.getFieldName($child)});
#end
#end
#end ##foreach
if (_log.isDebugEnabled()) {
_log.debug("Initializing successful");
}
}
/** {@inheritDoc} */
public void createValueObject() throws HostException {
/* Since this complex binding has a constructor that takes a
* Value object, we might already have a Value object that
* was not used yet. */
if (mUnusedValueObject && mValueObject != null) {
mUnusedValueObject = false;
return;
}
#if (${binding.getValueObjectsFactoryClassName()})
mValueObject = mValueObjectFactory.create${valueObjectType}();
#else
mValueObject = new ${valueObjectType}();
#end
}
/** {@inheritDoc} */
public void setChildrenValues() throws HostException {
/* Make sure there is an associated Value object*/
if (mValueObject == null) {
createValueObject();
}
#foreach ($child in $binding.childrenList)
#if($child.isBound())
/* Get Value object property ${coxbHelper.getFieldName($child)} */
if (_log.isDebugEnabled()) {
_log.debug("Getting value from Value object property "
+ "${coxbHelper.getFieldName($child)}"
+ " value=" + mValueObject.${coxbHelper.getterMethodName($child)}());
}
#if(${coxbHelper.isArray($child)} && ${coxbHelper.isJaxbArray($binding, $child)})
${coxbHelper.getFieldName($child)}.setObjectValue(java.util.Arrays
.asList(mValueObject.${coxbHelper.getterMethodName($child)}()));
#else
${coxbHelper.getFieldName($child)}.setObjectValue(mValueObject.${coxbHelper.getterMethodName($child)}());
#end
#if(${coxbHelper.isVariableSizeArray($child)})
/* For variable size array or list, we make sure any
* associated counter is updated */
setCounterValue(${coxbHelper.getFieldName($child)}.getDependingOn(),
((List < ? >) mValueObject.${coxbHelper.getterMethodName($child)}()).size());
#else
#if(${coxbHelper.isOptional($child)})
/* If object exist make sure associated counter is 1 */
if (null != mValueObject.${coxbHelper.getterMethodName($child)}()) {
setCounterValue(${coxbHelper.getFieldName($child)}.getDependingOn(), 1);
}
#end
#end
#end
#end ##foreach
}
/** {@inheritDoc} */
public void setPropertyValue(final int index) throws HostException {
ICobolBinding child = getChildrenList().get(index);
/* Children that are not bound to a value object are ignored.
* This includes Choices and dynamically generated counters
* for instance. */
if (!child.isBound()) {
return;
}
/* Set the Value object property value from binding object */
Object bindingValue = null;
switch (index) {
#set($counter = 0)
#foreach ($child in $binding.childrenList)
case ${counter}:
#if($child.isBound())
#set ($childObjectType = $coxbHelper.getBoundTypeName($child))
bindingValue = child.getObjectValue(${childObjectType}.class);
#if(${coxbHelper.isArray($child)})
List < $childObjectType > list${child.bindingName} = cast(bindingValue);
#if(${coxbHelper.isJaxbArray($binding, $child)})
mValueObject.${coxbHelper.setterMethodName($child)}(list${child.bindingName}.toArray(
new ${childObjectType}[] {}));
#else
mValueObject.${coxbHelper.getterMethodName($child)}().clear();
mValueObject.${coxbHelper.getterMethodName($child)}().addAll(list${child.bindingName});
#end
#else
mValueObject.${coxbHelper.setterMethodName($child)}(($childObjectType) bindingValue);
#end
#end
break;
#set($counter = $counter + 1)
#end ##foreach
default:
break;
}
if (_log.isDebugEnabled()) {
_log.debug("Setting value of Value object property "
+ child.getJaxbName()
+ " value=" + bindingValue);
}
}
/** {@inheritDoc} */
public Object getObjectValue(
final Class < ? > type) throws HostException {
if (type.equals(${valueObjectType}.class)) {
return mValueObject;
} else {
throw new HostException("Attempt to get binding " + getBindingName()
+ " as an incompatible type " + type);
}
}
/** {@inheritDoc} */
public void setObjectValue(
final Object bindingValue) throws HostException {
if (bindingValue == null) {
mValueObject = null;
return;
}
if (bindingValue.getClass().equals(${valueObjectType}.class)) {
mValueObject = ($valueObjectType) bindingValue;
} else {
throw new HostException("Attempt to set binding " + getBindingName()
+ " from an incompatible value " + bindingValue);
}
}
/**
* @return the java object factory for objects creation
*/
#if (${binding.getValueObjectsFactoryClassName()})
public ObjectFactory getObjectFactory() {
return mValueObjectFactory;
#else
public Object getObjectFactory() {
return null;
#end
}
/**
* @param valueObjectFactory the java object factory for objects creation
*/
public void setObjectFactory(final Object valueObjectFactory) {
#if (${binding.getValueObjectsFactoryClassName()})
mValueObjectFactory = (ObjectFactory) valueObjectFactory;
#end
}
/** {@inheritDoc} */
public boolean isSet() {
return (mValueObject != null);
}
/**
* @return the bound Value object
*/
public $valueObjectType get${valueObjectType}() {
return mValueObject;
}
/**
* The COBOL complex element maximum length in bytes.
*
* @return COBOL complex element maximum length in bytes
*/
public int getByteLength() {
return BYTE_LENGTH;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy