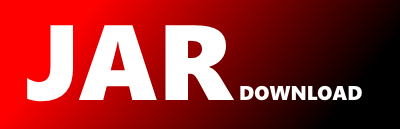
com.legyver.fenxlib.widgets.filetree.tree.FileTreeItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fenxlib.widgets.filetree Show documentation
Show all versions of fenxlib.widgets.filetree Show documentation
FileTree widget for Fenxlib projects
package com.legyver.fenxlib.widgets.filetree.tree;
import com.legyver.fenxlib.core.api.locator.IPropertyAware;
import com.legyver.fenxlib.widgets.filetree.nodes.INodeReference;
import com.legyver.fenxlib.widgets.filetree.search.BinarySearch;
import com.legyver.fenxlib.widgets.filetree.search.INamedItem;
import com.legyver.fenxlib.widgets.filetree.tree.internal.RefreshingTreeItem;
import javafx.application.Platform;
import javafx.beans.property.BooleanProperty;
import javafx.beans.property.SimpleBooleanProperty;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.collections.ObservableMap;
import javafx.scene.Node;
import javafx.scene.control.TreeItem;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
/**
* Base FileTreeItem class adding functionality to the base {@link TreeItem} implementation.
* - association with the INodeReference for the TreeItem
* - placeholder text for while folder is refreshing
* - listens on the {@link TreeItem#expandedProperty()} and refreshes the folder children every time it is expanded
*
* @param the type of the {@link INodeReference} associated with the particular node.
*/
public abstract class FileTreeItem extends TreeItem implements INamedItem, IPropertyAware {
private static final Logger logger = LogManager.getLogger(FileTreeItem.class);
/**
* Properties to associate with the node. Primarily used to set GUIDs on the TreeItem similarly to how it is done on Node. See {@link Node#getProperties()}
*/
private final ObservableMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy