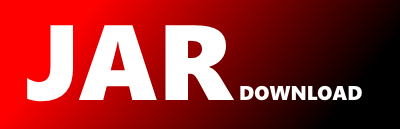
com.leondesilva.jcor.Handler Maven / Gradle / Ivy
package com.leondesilva.jcor;
/**
* Interface to represent a handler in JCor.
* Handler is equal to a single node or processor in chain of responsibility design.
*
* @param the input object
* @param the status object
*/
public interface Handler {
/**
* Method to evaluate whether the current handler is capable for processing the given input object.
*
* @param input the input object
* @param status the status object
* @return true if processing should happen through the current handler, else returns false
*/
boolean evaluate(I input, S status);
/**
* Method to do the processing.
*
* @param input the input object
* @param status the status object keep track of the status of execution
* @throws Exception if an error occurs
*/
void process(I input, S status) throws Exception;
/**
* Method to let the executor know whether the processing should stop from the current handler or not.
*
* @param status the status object
* @return true if processing should end from the current handler, false if processing should also go for next handler
*/
boolean shouldExitChainAfterProcessing(S status);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy