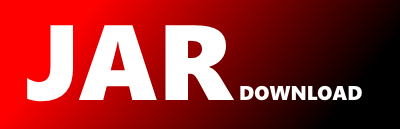
com.lewisd.maven.lint.rules.basic.DuplicateDependenciesRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lint-maven-plugin Show documentation
Show all versions of lint-maven-plugin Show documentation
Generates a report of suspicious/inconsistent POM elements, and optionally fails the build if violations are found.
The newest version!
package com.lewisd.maven.lint.rules.basic;
import com.lewisd.maven.lint.ResultCollector;
import com.lewisd.maven.lint.rules.AbstractRule;
import com.lewisd.maven.lint.util.ExpressionEvaluator;
import com.lewisd.maven.lint.util.ModelUtil;
import org.apache.commons.lang.ObjectUtils;
import org.apache.maven.model.Dependency;
import org.apache.maven.model.InputLocation;
import org.apache.maven.model.Model;
import org.apache.maven.project.MavenProject;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Map;
public class DuplicateDependenciesRule extends AbstractRule {
@Autowired
public DuplicateDependenciesRule(final ExpressionEvaluator expressionEvaluator, final ModelUtil modelUtil) {
super(expressionEvaluator, modelUtil);
}
@Override
public String getIdentifier() {
return "DuplicateDep";
}
@Override
public String getDescription() {
return "Multiple dependencies, in or , with the same co-ordinates are reduntant, " +
"and can be confusing. If they have different versions, they can lead to unexpected behaviour.";
}
@Override
public void invoke(final MavenProject mavenProject, final Map models, final ResultCollector resultCollector) {
final Model originalModel = mavenProject.getOriginalModel();
final Collection dependencies = expressionEvaluator.getPath(originalModel, "dependencies");
final Collection managedDependencies = expressionEvaluator.getPath(originalModel, "dependencyManagement/dependencies");
checkForDuplicateDependencies(mavenProject, resultCollector, dependencies, "Dependency");
checkForDuplicateDependencies(mavenProject, resultCollector, managedDependencies, "Managed dependency");
}
private void checkForDuplicateDependencies(final MavenProject mavenProject, final ResultCollector resultCollector,
final Collection dependencies,
final String dependencyDescription) {
final Collection otherDependencies = new LinkedList(dependencies);
for (final Dependency dependency : dependencies) {
checkForDuplicateArtifacts(mavenProject, resultCollector, dependency, otherDependencies, dependencyDescription);
}
}
private void checkForDuplicateArtifacts(final MavenProject mavenProject, final ResultCollector resultCollector,
final Dependency dependency, final Collection otherDependencies, final String dependencyDescription) {
for (final Iterator i = otherDependencies.iterator(); i.hasNext();) {
final Dependency otherManagedDependency = i.next();
if (otherManagedDependency.getManagementKey().equals(dependency.getManagementKey())) {
i.remove();
if (otherManagedDependency != dependency) {
final String version = modelUtil.getVersion(dependency);
final String otherVersion = modelUtil.getVersion(otherManagedDependency);
final InputLocation location = modelUtil.getLocation(dependency);
final InputLocation otherLocation = modelUtil.getLocation(otherManagedDependency);
if (ObjectUtils.equals(version, otherVersion)) {
resultCollector.addViolation(mavenProject, this, dependencyDescription + " '" + modelUtil.getKey(dependency) +
"' is declared multiple times with the same version: " +
otherLocation.getLineNumber() + ":" + otherLocation.getColumnNumber(), location);
} else {
resultCollector.addViolation(mavenProject, this, dependencyDescription + " '" + modelUtil.getKey(dependency) +
"' is declared multiple times with different versions (" + version + ", "
+ otherVersion + ")", location);
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy