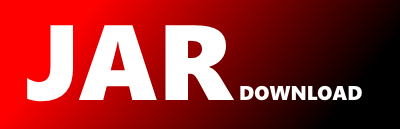
com.paypal.api.payments.AgreementTransaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:04 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class AgreementTransaction extends PayPalModel {
/**
* Id corresponding to this transaction.
*/
private String transactionId;
/**
* State of the subscription at this time.
*/
private String status;
/**
* Type of transaction, usually Recurring Payment.
*/
private String transactionType;
/**
* Amount for this transaction.
*/
private Currency amount;
/**
* Fee amount for this transaction.
*/
private Currency feeAmount;
/**
* Net amount for this transaction.
*/
private Currency netAmount;
/**
* Email id of payer.
*/
private String payerEmail;
/**
* Business name of payer.
*/
private String payerName;
/**
* Time zone of time_updated field.
*/
private String timeZone;
/**
* Time at which this transaction happened.
*/
private String timeStamp;
/**
* Default Constructor
*/
public AgreementTransaction() {
}
/**
* Parameterized Constructor
*/
public AgreementTransaction(Currency amount, Currency feeAmount, Currency netAmount) {
this.amount = amount;
this.feeAmount = feeAmount;
this.netAmount = netAmount;
}
/**
* @deprecated use setTimeStamp instead.
* Setter for timeUpdated
*/
public AgreementTransaction setTimeUpdated(String timeUpdated) {
this.timeStamp = timeUpdated;
return this;
}
/**
* @deprecated use getTimeStamp instead.
* Getter for timeUpdated
*/
public String getTimeUpdated() {
return this.timeStamp;
}
/**
* Id corresponding to this transaction.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionId() {
return this.transactionId;
}
/**
* State of the subscription at this time.
*/
@java.lang.SuppressWarnings("all")
public String getStatus() {
return this.status;
}
/**
* Type of transaction, usually Recurring Payment.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionType() {
return this.transactionType;
}
/**
* Amount for this transaction.
*/
@java.lang.SuppressWarnings("all")
public Currency getAmount() {
return this.amount;
}
/**
* Fee amount for this transaction.
*/
@java.lang.SuppressWarnings("all")
public Currency getFeeAmount() {
return this.feeAmount;
}
/**
* Net amount for this transaction.
*/
@java.lang.SuppressWarnings("all")
public Currency getNetAmount() {
return this.netAmount;
}
/**
* Email id of payer.
*/
@java.lang.SuppressWarnings("all")
public String getPayerEmail() {
return this.payerEmail;
}
/**
* Business name of payer.
*/
@java.lang.SuppressWarnings("all")
public String getPayerName() {
return this.payerName;
}
/**
* Time zone of time_updated field.
*/
@java.lang.SuppressWarnings("all")
public String getTimeZone() {
return this.timeZone;
}
/**
* Time at which this transaction happened.
*/
@java.lang.SuppressWarnings("all")
public String getTimeStamp() {
return this.timeStamp;
}
/**
* Id corresponding to this transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setTransactionId(final String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* State of the subscription at this time.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setStatus(final String status) {
this.status = status;
return this;
}
/**
* Type of transaction, usually Recurring Payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setTransactionType(final String transactionType) {
this.transactionType = transactionType;
return this;
}
/**
* Amount for this transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setAmount(final Currency amount) {
this.amount = amount;
return this;
}
/**
* Fee amount for this transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setFeeAmount(final Currency feeAmount) {
this.feeAmount = feeAmount;
return this;
}
/**
* Net amount for this transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setNetAmount(final Currency netAmount) {
this.netAmount = netAmount;
return this;
}
/**
* Email id of payer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setPayerEmail(final String payerEmail) {
this.payerEmail = payerEmail;
return this;
}
/**
* Business name of payer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setPayerName(final String payerName) {
this.payerName = payerName;
return this;
}
/**
* Time zone of time_updated field.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setTimeZone(final String timeZone) {
this.timeZone = timeZone;
return this;
}
/**
* Time at which this transaction happened.
* @return this
*/
@java.lang.SuppressWarnings("all")
public AgreementTransaction setTimeStamp(final String timeStamp) {
this.timeStamp = timeStamp;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof AgreementTransaction)) return false;
final AgreementTransaction other = (AgreementTransaction) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$transactionId = this.getTransactionId();
final java.lang.Object other$transactionId = other.getTransactionId();
if (this$transactionId == null ? other$transactionId != null : !this$transactionId.equals(other$transactionId)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$transactionType = this.getTransactionType();
final java.lang.Object other$transactionType = other.getTransactionType();
if (this$transactionType == null ? other$transactionType != null : !this$transactionType.equals(other$transactionType)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$feeAmount = this.getFeeAmount();
final java.lang.Object other$feeAmount = other.getFeeAmount();
if (this$feeAmount == null ? other$feeAmount != null : !this$feeAmount.equals(other$feeAmount)) return false;
final java.lang.Object this$netAmount = this.getNetAmount();
final java.lang.Object other$netAmount = other.getNetAmount();
if (this$netAmount == null ? other$netAmount != null : !this$netAmount.equals(other$netAmount)) return false;
final java.lang.Object this$payerEmail = this.getPayerEmail();
final java.lang.Object other$payerEmail = other.getPayerEmail();
if (this$payerEmail == null ? other$payerEmail != null : !this$payerEmail.equals(other$payerEmail)) return false;
final java.lang.Object this$payerName = this.getPayerName();
final java.lang.Object other$payerName = other.getPayerName();
if (this$payerName == null ? other$payerName != null : !this$payerName.equals(other$payerName)) return false;
final java.lang.Object this$timeZone = this.getTimeZone();
final java.lang.Object other$timeZone = other.getTimeZone();
if (this$timeZone == null ? other$timeZone != null : !this$timeZone.equals(other$timeZone)) return false;
final java.lang.Object this$timeStamp = this.getTimeStamp();
final java.lang.Object other$timeStamp = other.getTimeStamp();
if (this$timeStamp == null ? other$timeStamp != null : !this$timeStamp.equals(other$timeStamp)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof AgreementTransaction;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $transactionId = this.getTransactionId();
result = result * PRIME + ($transactionId == null ? 43 : $transactionId.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $transactionType = this.getTransactionType();
result = result * PRIME + ($transactionType == null ? 43 : $transactionType.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $feeAmount = this.getFeeAmount();
result = result * PRIME + ($feeAmount == null ? 43 : $feeAmount.hashCode());
final java.lang.Object $netAmount = this.getNetAmount();
result = result * PRIME + ($netAmount == null ? 43 : $netAmount.hashCode());
final java.lang.Object $payerEmail = this.getPayerEmail();
result = result * PRIME + ($payerEmail == null ? 43 : $payerEmail.hashCode());
final java.lang.Object $payerName = this.getPayerName();
result = result * PRIME + ($payerName == null ? 43 : $payerName.hashCode());
final java.lang.Object $timeZone = this.getTimeZone();
result = result * PRIME + ($timeZone == null ? 43 : $timeZone.hashCode());
final java.lang.Object $timeStamp = this.getTimeStamp();
result = result * PRIME + ($timeStamp == null ? 43 : $timeStamp.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy