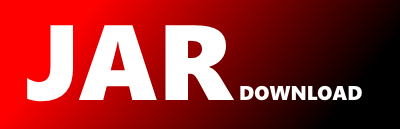
com.paypal.api.payments.CarrierAccount Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:04 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class CarrierAccount extends PayPalModel {
/**
* The ID of the carrier account of the payer. Use in subsequent REST API calls. For example, to make payments.
*/
private String id;
/**
* The phone number of the payer, in E.164 format.
*/
private String phoneNumber;
/**
* The ID of the customer, as created by the merchant.
*/
private String externalCustomerId;
/**
* The method used to obtain the phone number. Value is `READ_FROM_DEVICE` or `USER_PROVIDED`.
*/
private String phoneSource;
/**
* The ISO 3166-1 alpha-2 country code where the phone number is registered.
*/
private CountryCode countryCode;
/**
* Default Constructor
*/
public CarrierAccount() {
}
/**
* Parameterized Constructor
*/
public CarrierAccount(String externalCustomerId, CountryCode countryCode) {
this.externalCustomerId = externalCustomerId;
this.countryCode = countryCode;
}
/**
* The ID of the carrier account of the payer. Use in subsequent REST API calls. For example, to make payments.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* The phone number of the payer, in E.164 format.
*/
@java.lang.SuppressWarnings("all")
public String getPhoneNumber() {
return this.phoneNumber;
}
/**
* The ID of the customer, as created by the merchant.
*/
@java.lang.SuppressWarnings("all")
public String getExternalCustomerId() {
return this.externalCustomerId;
}
/**
* The method used to obtain the phone number. Value is `READ_FROM_DEVICE` or `USER_PROVIDED`.
*/
@java.lang.SuppressWarnings("all")
public String getPhoneSource() {
return this.phoneSource;
}
/**
* The ISO 3166-1 alpha-2 country code where the phone number is registered.
*/
@java.lang.SuppressWarnings("all")
public CountryCode getCountryCode() {
return this.countryCode;
}
/**
* The ID of the carrier account of the payer. Use in subsequent REST API calls. For example, to make payments.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CarrierAccount setId(final String id) {
this.id = id;
return this;
}
/**
* The phone number of the payer, in E.164 format.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CarrierAccount setPhoneNumber(final String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* The ID of the customer, as created by the merchant.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CarrierAccount setExternalCustomerId(final String externalCustomerId) {
this.externalCustomerId = externalCustomerId;
return this;
}
/**
* The method used to obtain the phone number. Value is `READ_FROM_DEVICE` or `USER_PROVIDED`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CarrierAccount setPhoneSource(final String phoneSource) {
this.phoneSource = phoneSource;
return this;
}
/**
* The ISO 3166-1 alpha-2 country code where the phone number is registered.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CarrierAccount setCountryCode(final CountryCode countryCode) {
this.countryCode = countryCode;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CarrierAccount)) return false;
final CarrierAccount other = (CarrierAccount) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$phoneNumber = this.getPhoneNumber();
final java.lang.Object other$phoneNumber = other.getPhoneNumber();
if (this$phoneNumber == null ? other$phoneNumber != null : !this$phoneNumber.equals(other$phoneNumber)) return false;
final java.lang.Object this$externalCustomerId = this.getExternalCustomerId();
final java.lang.Object other$externalCustomerId = other.getExternalCustomerId();
if (this$externalCustomerId == null ? other$externalCustomerId != null : !this$externalCustomerId.equals(other$externalCustomerId)) return false;
final java.lang.Object this$phoneSource = this.getPhoneSource();
final java.lang.Object other$phoneSource = other.getPhoneSource();
if (this$phoneSource == null ? other$phoneSource != null : !this$phoneSource.equals(other$phoneSource)) return false;
final java.lang.Object this$countryCode = this.getCountryCode();
final java.lang.Object other$countryCode = other.getCountryCode();
if (this$countryCode == null ? other$countryCode != null : !this$countryCode.equals(other$countryCode)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CarrierAccount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $phoneNumber = this.getPhoneNumber();
result = result * PRIME + ($phoneNumber == null ? 43 : $phoneNumber.hashCode());
final java.lang.Object $externalCustomerId = this.getExternalCustomerId();
result = result * PRIME + ($externalCustomerId == null ? 43 : $externalCustomerId.hashCode());
final java.lang.Object $phoneSource = this.getPhoneSource();
result = result * PRIME + ($phoneSource == null ? 43 : $phoneSource.hashCode());
final java.lang.Object $countryCode = this.getCountryCode();
result = result * PRIME + ($countryCode == null ? 43 : $countryCode.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy