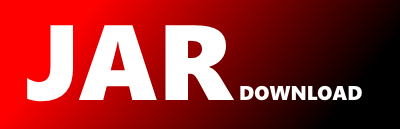
com.paypal.api.payments.CreditFinancingOffered Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:04 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class CreditFinancingOffered extends PayPalModel {
/**
* This is the estimated total payment amount including interest and fees the user will pay during the lifetime of the loan.
*/
private Currency totalCost;
/**
* Length of financing terms in month
*/
private float term;
/**
* This is the estimated amount per month that the customer will need to pay including fees and interest.
*/
private Currency monthlyPayment;
/**
* Estimated interest or fees amount the payer will have to pay during the lifetime of the loan.
*/
private Currency totalInterest;
/**
* Status on whether the customer ultimately was approved for and chose to make the payment using the approved installment credit.
*/
private Boolean payerAcceptance;
/**
* Indicates whether the cart amount is editable after payer's acceptance on PayPal side
*/
private Boolean cartAmountImmutable;
/**
* Default Constructor
*/
public CreditFinancingOffered() {
}
/**
* Parameterized Constructor
*/
public CreditFinancingOffered(Currency totalCost, float term, Currency monthlyPayment, Currency totalInterest, Boolean payerAcceptance) {
this.totalCost = totalCost;
this.term = term;
this.monthlyPayment = monthlyPayment;
this.totalInterest = totalInterest;
this.payerAcceptance = payerAcceptance;
}
/**
* This is the estimated total payment amount including interest and fees the user will pay during the lifetime of the loan.
*/
@java.lang.SuppressWarnings("all")
public Currency getTotalCost() {
return this.totalCost;
}
/**
* Length of financing terms in month
*/
@java.lang.SuppressWarnings("all")
public float getTerm() {
return this.term;
}
/**
* This is the estimated amount per month that the customer will need to pay including fees and interest.
*/
@java.lang.SuppressWarnings("all")
public Currency getMonthlyPayment() {
return this.monthlyPayment;
}
/**
* Estimated interest or fees amount the payer will have to pay during the lifetime of the loan.
*/
@java.lang.SuppressWarnings("all")
public Currency getTotalInterest() {
return this.totalInterest;
}
/**
* Status on whether the customer ultimately was approved for and chose to make the payment using the approved installment credit.
*/
@java.lang.SuppressWarnings("all")
public Boolean getPayerAcceptance() {
return this.payerAcceptance;
}
/**
* Indicates whether the cart amount is editable after payer's acceptance on PayPal side
*/
@java.lang.SuppressWarnings("all")
public Boolean getCartAmountImmutable() {
return this.cartAmountImmutable;
}
/**
* This is the estimated total payment amount including interest and fees the user will pay during the lifetime of the loan.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditFinancingOffered setTotalCost(final Currency totalCost) {
this.totalCost = totalCost;
return this;
}
/**
* Length of financing terms in month
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditFinancingOffered setTerm(final float term) {
this.term = term;
return this;
}
/**
* This is the estimated amount per month that the customer will need to pay including fees and interest.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditFinancingOffered setMonthlyPayment(final Currency monthlyPayment) {
this.monthlyPayment = monthlyPayment;
return this;
}
/**
* Estimated interest or fees amount the payer will have to pay during the lifetime of the loan.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditFinancingOffered setTotalInterest(final Currency totalInterest) {
this.totalInterest = totalInterest;
return this;
}
/**
* Status on whether the customer ultimately was approved for and chose to make the payment using the approved installment credit.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditFinancingOffered setPayerAcceptance(final Boolean payerAcceptance) {
this.payerAcceptance = payerAcceptance;
return this;
}
/**
* Indicates whether the cart amount is editable after payer's acceptance on PayPal side
* @return this
*/
@java.lang.SuppressWarnings("all")
public CreditFinancingOffered setCartAmountImmutable(final Boolean cartAmountImmutable) {
this.cartAmountImmutable = cartAmountImmutable;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CreditFinancingOffered)) return false;
final CreditFinancingOffered other = (CreditFinancingOffered) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$totalCost = this.getTotalCost();
final java.lang.Object other$totalCost = other.getTotalCost();
if (this$totalCost == null ? other$totalCost != null : !this$totalCost.equals(other$totalCost)) return false;
if (java.lang.Float.compare(this.getTerm(), other.getTerm()) != 0) return false;
final java.lang.Object this$monthlyPayment = this.getMonthlyPayment();
final java.lang.Object other$monthlyPayment = other.getMonthlyPayment();
if (this$monthlyPayment == null ? other$monthlyPayment != null : !this$monthlyPayment.equals(other$monthlyPayment)) return false;
final java.lang.Object this$totalInterest = this.getTotalInterest();
final java.lang.Object other$totalInterest = other.getTotalInterest();
if (this$totalInterest == null ? other$totalInterest != null : !this$totalInterest.equals(other$totalInterest)) return false;
final java.lang.Object this$payerAcceptance = this.getPayerAcceptance();
final java.lang.Object other$payerAcceptance = other.getPayerAcceptance();
if (this$payerAcceptance == null ? other$payerAcceptance != null : !this$payerAcceptance.equals(other$payerAcceptance)) return false;
final java.lang.Object this$cartAmountImmutable = this.getCartAmountImmutable();
final java.lang.Object other$cartAmountImmutable = other.getCartAmountImmutable();
if (this$cartAmountImmutable == null ? other$cartAmountImmutable != null : !this$cartAmountImmutable.equals(other$cartAmountImmutable)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CreditFinancingOffered;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $totalCost = this.getTotalCost();
result = result * PRIME + ($totalCost == null ? 43 : $totalCost.hashCode());
result = result * PRIME + java.lang.Float.floatToIntBits(this.getTerm());
final java.lang.Object $monthlyPayment = this.getMonthlyPayment();
result = result * PRIME + ($monthlyPayment == null ? 43 : $monthlyPayment.hashCode());
final java.lang.Object $totalInterest = this.getTotalInterest();
result = result * PRIME + ($totalInterest == null ? 43 : $totalInterest.hashCode());
final java.lang.Object $payerAcceptance = this.getPayerAcceptance();
result = result * PRIME + ($payerAcceptance == null ? 43 : $payerAcceptance.hashCode());
final java.lang.Object $cartAmountImmutable = this.getCartAmountImmutable();
result = result * PRIME + ($cartAmountImmutable == null ? 43 : $cartAmountImmutable.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy