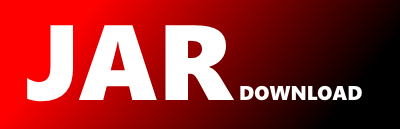
com.paypal.api.payments.CurrencyConversion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:04 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class CurrencyConversion extends PayPalModel {
/**
* Date of validity for the conversion rate.
*/
private String conversionDate;
/**
* 3 letter currency code
*/
private String fromCurrency;
/**
* Amount participating in currency conversion, set to 1 as default
*/
private String fromAmount;
/**
* 3 letter currency code
*/
private String toCurrency;
/**
* Amount resulting from currency conversion.
*/
private String toAmount;
/**
* Field indicating conversion type applied.
*/
private String conversionType;
/**
* Allow Payer to change conversion type.
*/
private Boolean conversionTypeChangeable;
/**
* Base URL to web applications endpoint
*/
private String webUrl;
/**
*/
private List links;
/**
* Default Constructor
*/
public CurrencyConversion() {
}
/**
* Parameterized Constructor
*/
public CurrencyConversion(String fromCurrency, String fromAmount, String toCurrency, String toAmount) {
this.fromCurrency = fromCurrency;
this.fromAmount = fromAmount;
this.toCurrency = toCurrency;
this.toAmount = toAmount;
}
/**
* Date of validity for the conversion rate.
*/
@java.lang.SuppressWarnings("all")
public String getConversionDate() {
return this.conversionDate;
}
/**
* 3 letter currency code
*/
@java.lang.SuppressWarnings("all")
public String getFromCurrency() {
return this.fromCurrency;
}
/**
* Amount participating in currency conversion, set to 1 as default
*/
@java.lang.SuppressWarnings("all")
public String getFromAmount() {
return this.fromAmount;
}
/**
* 3 letter currency code
*/
@java.lang.SuppressWarnings("all")
public String getToCurrency() {
return this.toCurrency;
}
/**
* Amount resulting from currency conversion.
*/
@java.lang.SuppressWarnings("all")
public String getToAmount() {
return this.toAmount;
}
/**
* Field indicating conversion type applied.
*/
@java.lang.SuppressWarnings("all")
public String getConversionType() {
return this.conversionType;
}
/**
* Allow Payer to change conversion type.
*/
@java.lang.SuppressWarnings("all")
public Boolean getConversionTypeChangeable() {
return this.conversionTypeChangeable;
}
/**
* Base URL to web applications endpoint
*/
@java.lang.SuppressWarnings("all")
public String getWebUrl() {
return this.webUrl;
}
/**
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* Date of validity for the conversion rate.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setConversionDate(final String conversionDate) {
this.conversionDate = conversionDate;
return this;
}
/**
* 3 letter currency code
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setFromCurrency(final String fromCurrency) {
this.fromCurrency = fromCurrency;
return this;
}
/**
* Amount participating in currency conversion, set to 1 as default
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setFromAmount(final String fromAmount) {
this.fromAmount = fromAmount;
return this;
}
/**
* 3 letter currency code
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setToCurrency(final String toCurrency) {
this.toCurrency = toCurrency;
return this;
}
/**
* Amount resulting from currency conversion.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setToAmount(final String toAmount) {
this.toAmount = toAmount;
return this;
}
/**
* Field indicating conversion type applied.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setConversionType(final String conversionType) {
this.conversionType = conversionType;
return this;
}
/**
* Allow Payer to change conversion type.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setConversionTypeChangeable(final Boolean conversionTypeChangeable) {
this.conversionTypeChangeable = conversionTypeChangeable;
return this;
}
/**
* Base URL to web applications endpoint
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setWebUrl(final String webUrl) {
this.webUrl = webUrl;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public CurrencyConversion setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CurrencyConversion)) return false;
final CurrencyConversion other = (CurrencyConversion) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$conversionDate = this.getConversionDate();
final java.lang.Object other$conversionDate = other.getConversionDate();
if (this$conversionDate == null ? other$conversionDate != null : !this$conversionDate.equals(other$conversionDate)) return false;
final java.lang.Object this$fromCurrency = this.getFromCurrency();
final java.lang.Object other$fromCurrency = other.getFromCurrency();
if (this$fromCurrency == null ? other$fromCurrency != null : !this$fromCurrency.equals(other$fromCurrency)) return false;
final java.lang.Object this$fromAmount = this.getFromAmount();
final java.lang.Object other$fromAmount = other.getFromAmount();
if (this$fromAmount == null ? other$fromAmount != null : !this$fromAmount.equals(other$fromAmount)) return false;
final java.lang.Object this$toCurrency = this.getToCurrency();
final java.lang.Object other$toCurrency = other.getToCurrency();
if (this$toCurrency == null ? other$toCurrency != null : !this$toCurrency.equals(other$toCurrency)) return false;
final java.lang.Object this$toAmount = this.getToAmount();
final java.lang.Object other$toAmount = other.getToAmount();
if (this$toAmount == null ? other$toAmount != null : !this$toAmount.equals(other$toAmount)) return false;
final java.lang.Object this$conversionType = this.getConversionType();
final java.lang.Object other$conversionType = other.getConversionType();
if (this$conversionType == null ? other$conversionType != null : !this$conversionType.equals(other$conversionType)) return false;
final java.lang.Object this$conversionTypeChangeable = this.getConversionTypeChangeable();
final java.lang.Object other$conversionTypeChangeable = other.getConversionTypeChangeable();
if (this$conversionTypeChangeable == null ? other$conversionTypeChangeable != null : !this$conversionTypeChangeable.equals(other$conversionTypeChangeable)) return false;
final java.lang.Object this$webUrl = this.getWebUrl();
final java.lang.Object other$webUrl = other.getWebUrl();
if (this$webUrl == null ? other$webUrl != null : !this$webUrl.equals(other$webUrl)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CurrencyConversion;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $conversionDate = this.getConversionDate();
result = result * PRIME + ($conversionDate == null ? 43 : $conversionDate.hashCode());
final java.lang.Object $fromCurrency = this.getFromCurrency();
result = result * PRIME + ($fromCurrency == null ? 43 : $fromCurrency.hashCode());
final java.lang.Object $fromAmount = this.getFromAmount();
result = result * PRIME + ($fromAmount == null ? 43 : $fromAmount.hashCode());
final java.lang.Object $toCurrency = this.getToCurrency();
result = result * PRIME + ($toCurrency == null ? 43 : $toCurrency.hashCode());
final java.lang.Object $toAmount = this.getToAmount();
result = result * PRIME + ($toAmount == null ? 43 : $toAmount.hashCode());
final java.lang.Object $conversionType = this.getConversionType();
result = result * PRIME + ($conversionType == null ? 43 : $conversionType.hashCode());
final java.lang.Object $conversionTypeChangeable = this.getConversionTypeChangeable();
result = result * PRIME + ($conversionTypeChangeable == null ? 43 : $conversionTypeChangeable.hashCode());
final java.lang.Object $webUrl = this.getWebUrl();
result = result * PRIME + ($webUrl == null ? 43 : $webUrl.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy