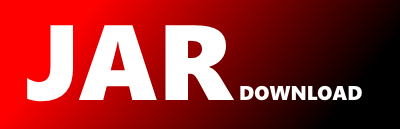
com.paypal.api.payments.Error Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class Error extends PayPalModel {
/**
* Human readable, unique name of the error.
*/
private String name;
/**
* Message describing the error.
*/
private String message;
/**
* Additional details of the error
*/
private List details;
/**
* URI for detailed information related to this error for the developer.
*/
private String informationLink;
/**
* PayPal internal identifier used for correlation purposes.
*/
private String debugId;
/**
* Links
*/
private List links;
/**
* @deprecated This property is not available publicly
* PayPal internal error code.
*/
@Deprecated
private String code;
/**
* @deprecated This property is not available publicly
* Fraud filter details. Only supported when the `payment_method` is set to `credit_card`
*/
@Deprecated
private FmfDetails fmfDetails;
/**
* @deprecated This property is not available publicly
* response codes returned from a payment processor such as avs, cvv, etc. Only supported when the `payment_method` is set to `credit_card`.
*/
@Deprecated
private ProcessorResponse processorResponse;
/**
* @deprecated This property is not available publicly
* Reference ID of the purchase_unit associated with this error
*/
@Deprecated
private String purchaseUnitReferenceId;
/**
* Default Constructor
*/
public Error() {
}
/**
* Parameterized Constructor
*/
public Error(String name, String message, String informationLink, String debugId) {
this.name = name;
this.message = message;
this.informationLink = informationLink;
this.debugId = debugId;
}
public String toString() {
return "name: " + this.name + "\tmessage: " + this.message + "\tdetails: " + this.details + "\tdebug-id: " + this.debugId + "\tinformation-link: " + this.informationLink;
}
/**
* Human readable, unique name of the error.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Message describing the error.
*/
@java.lang.SuppressWarnings("all")
public String getMessage() {
return this.message;
}
/**
* Additional details of the error
*/
@java.lang.SuppressWarnings("all")
public List getDetails() {
return this.details;
}
/**
* URI for detailed information related to this error for the developer.
*/
@java.lang.SuppressWarnings("all")
public String getInformationLink() {
return this.informationLink;
}
/**
* PayPal internal identifier used for correlation purposes.
*/
@java.lang.SuppressWarnings("all")
public String getDebugId() {
return this.debugId;
}
/**
* Links
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* @deprecated This property is not available publicly
* PayPal internal error code.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* @deprecated This property is not available publicly
* Fraud filter details. Only supported when the `payment_method` is set to `credit_card`
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public FmfDetails getFmfDetails() {
return this.fmfDetails;
}
/**
* @deprecated This property is not available publicly
* response codes returned from a payment processor such as avs, cvv, etc. Only supported when the `payment_method` is set to `credit_card`.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public ProcessorResponse getProcessorResponse() {
return this.processorResponse;
}
/**
* @deprecated This property is not available publicly
* Reference ID of the purchase_unit associated with this error
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public String getPurchaseUnitReferenceId() {
return this.purchaseUnitReferenceId;
}
/**
* Human readable, unique name of the error.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Error setName(final String name) {
this.name = name;
return this;
}
/**
* Message describing the error.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Error setMessage(final String message) {
this.message = message;
return this;
}
/**
* Additional details of the error
* @return this
*/
@java.lang.SuppressWarnings("all")
public Error setDetails(final List details) {
this.details = details;
return this;
}
/**
* URI for detailed information related to this error for the developer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Error setInformationLink(final String informationLink) {
this.informationLink = informationLink;
return this;
}
/**
* PayPal internal identifier used for correlation purposes.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Error setDebugId(final String debugId) {
this.debugId = debugId;
return this;
}
/**
* Links
* @return this
*/
@java.lang.SuppressWarnings("all")
public Error setLinks(final List links) {
this.links = links;
return this;
}
/**
* @deprecated This property is not available publicly
* PayPal internal error code.
* @return this
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Error setCode(final String code) {
this.code = code;
return this;
}
/**
* @deprecated This property is not available publicly
* Fraud filter details. Only supported when the `payment_method` is set to `credit_card`
* @return this
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Error setFmfDetails(final FmfDetails fmfDetails) {
this.fmfDetails = fmfDetails;
return this;
}
/**
* @deprecated This property is not available publicly
* response codes returned from a payment processor such as avs, cvv, etc. Only supported when the `payment_method` is set to `credit_card`.
* @return this
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Error setProcessorResponse(final ProcessorResponse processorResponse) {
this.processorResponse = processorResponse;
return this;
}
/**
* @deprecated This property is not available publicly
* Reference ID of the purchase_unit associated with this error
* @return this
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Error setPurchaseUnitReferenceId(final String purchaseUnitReferenceId) {
this.purchaseUnitReferenceId = purchaseUnitReferenceId;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Error)) return false;
final Error other = (Error) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$message = this.getMessage();
final java.lang.Object other$message = other.getMessage();
if (this$message == null ? other$message != null : !this$message.equals(other$message)) return false;
final java.lang.Object this$details = this.getDetails();
final java.lang.Object other$details = other.getDetails();
if (this$details == null ? other$details != null : !this$details.equals(other$details)) return false;
final java.lang.Object this$informationLink = this.getInformationLink();
final java.lang.Object other$informationLink = other.getInformationLink();
if (this$informationLink == null ? other$informationLink != null : !this$informationLink.equals(other$informationLink)) return false;
final java.lang.Object this$debugId = this.getDebugId();
final java.lang.Object other$debugId = other.getDebugId();
if (this$debugId == null ? other$debugId != null : !this$debugId.equals(other$debugId)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
final java.lang.Object this$fmfDetails = this.getFmfDetails();
final java.lang.Object other$fmfDetails = other.getFmfDetails();
if (this$fmfDetails == null ? other$fmfDetails != null : !this$fmfDetails.equals(other$fmfDetails)) return false;
final java.lang.Object this$processorResponse = this.getProcessorResponse();
final java.lang.Object other$processorResponse = other.getProcessorResponse();
if (this$processorResponse == null ? other$processorResponse != null : !this$processorResponse.equals(other$processorResponse)) return false;
final java.lang.Object this$purchaseUnitReferenceId = this.getPurchaseUnitReferenceId();
final java.lang.Object other$purchaseUnitReferenceId = other.getPurchaseUnitReferenceId();
if (this$purchaseUnitReferenceId == null ? other$purchaseUnitReferenceId != null : !this$purchaseUnitReferenceId.equals(other$purchaseUnitReferenceId)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Error;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $message = this.getMessage();
result = result * PRIME + ($message == null ? 43 : $message.hashCode());
final java.lang.Object $details = this.getDetails();
result = result * PRIME + ($details == null ? 43 : $details.hashCode());
final java.lang.Object $informationLink = this.getInformationLink();
result = result * PRIME + ($informationLink == null ? 43 : $informationLink.hashCode());
final java.lang.Object $debugId = this.getDebugId();
result = result * PRIME + ($debugId == null ? 43 : $debugId.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
final java.lang.Object $fmfDetails = this.getFmfDetails();
result = result * PRIME + ($fmfDetails == null ? 43 : $fmfDetails.hashCode());
final java.lang.Object $processorResponse = this.getProcessorResponse();
result = result * PRIME + ($processorResponse == null ? 43 : $processorResponse.hashCode());
final java.lang.Object $purchaseUnitReferenceId = this.getPurchaseUnitReferenceId();
result = result * PRIME + ($purchaseUnitReferenceId == null ? 43 : $purchaseUnitReferenceId.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy