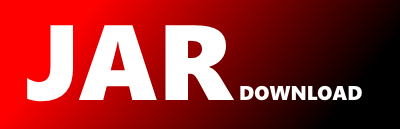
com.paypal.api.payments.InvoicingMetaData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class InvoicingMetaData extends PayPalModel {
/**
* Date when the resource was created.
*/
private String createdDate;
/**
* Email address of the account that created the resource.
*/
private String createdBy;
/**
* Date when the resource was cancelled.
*/
private String cancelledDate;
/**
* Actor who cancelled the resource.
*/
private String cancelledBy;
/**
* Date when the resource was last edited.
*/
private String lastUpdatedDate;
/**
* Email address of the account that last edited the resource.
*/
private String lastUpdatedBy;
/**
* Date when the resource was first sent.
*/
private String firstSentDate;
/**
* Date when the resource was last sent.
*/
private String lastSentDate;
/**
* Email address of the account that last sent the resource.
*/
private String lastSentBy;
/**
* Default Constructor
*/
public InvoicingMetaData() {
}
/**
* Date when the resource was created.
*/
@java.lang.SuppressWarnings("all")
public String getCreatedDate() {
return this.createdDate;
}
/**
* Email address of the account that created the resource.
*/
@java.lang.SuppressWarnings("all")
public String getCreatedBy() {
return this.createdBy;
}
/**
* Date when the resource was cancelled.
*/
@java.lang.SuppressWarnings("all")
public String getCancelledDate() {
return this.cancelledDate;
}
/**
* Actor who cancelled the resource.
*/
@java.lang.SuppressWarnings("all")
public String getCancelledBy() {
return this.cancelledBy;
}
/**
* Date when the resource was last edited.
*/
@java.lang.SuppressWarnings("all")
public String getLastUpdatedDate() {
return this.lastUpdatedDate;
}
/**
* Email address of the account that last edited the resource.
*/
@java.lang.SuppressWarnings("all")
public String getLastUpdatedBy() {
return this.lastUpdatedBy;
}
/**
* Date when the resource was first sent.
*/
@java.lang.SuppressWarnings("all")
public String getFirstSentDate() {
return this.firstSentDate;
}
/**
* Date when the resource was last sent.
*/
@java.lang.SuppressWarnings("all")
public String getLastSentDate() {
return this.lastSentDate;
}
/**
* Email address of the account that last sent the resource.
*/
@java.lang.SuppressWarnings("all")
public String getLastSentBy() {
return this.lastSentBy;
}
/**
* Date when the resource was created.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setCreatedDate(final String createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Email address of the account that created the resource.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setCreatedBy(final String createdBy) {
this.createdBy = createdBy;
return this;
}
/**
* Date when the resource was cancelled.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setCancelledDate(final String cancelledDate) {
this.cancelledDate = cancelledDate;
return this;
}
/**
* Actor who cancelled the resource.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setCancelledBy(final String cancelledBy) {
this.cancelledBy = cancelledBy;
return this;
}
/**
* Date when the resource was last edited.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setLastUpdatedDate(final String lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
return this;
}
/**
* Email address of the account that last edited the resource.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setLastUpdatedBy(final String lastUpdatedBy) {
this.lastUpdatedBy = lastUpdatedBy;
return this;
}
/**
* Date when the resource was first sent.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setFirstSentDate(final String firstSentDate) {
this.firstSentDate = firstSentDate;
return this;
}
/**
* Date when the resource was last sent.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setLastSentDate(final String lastSentDate) {
this.lastSentDate = lastSentDate;
return this;
}
/**
* Email address of the account that last sent the resource.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingMetaData setLastSentBy(final String lastSentBy) {
this.lastSentBy = lastSentBy;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof InvoicingMetaData)) return false;
final InvoicingMetaData other = (InvoicingMetaData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$createdDate = this.getCreatedDate();
final java.lang.Object other$createdDate = other.getCreatedDate();
if (this$createdDate == null ? other$createdDate != null : !this$createdDate.equals(other$createdDate)) return false;
final java.lang.Object this$createdBy = this.getCreatedBy();
final java.lang.Object other$createdBy = other.getCreatedBy();
if (this$createdBy == null ? other$createdBy != null : !this$createdBy.equals(other$createdBy)) return false;
final java.lang.Object this$cancelledDate = this.getCancelledDate();
final java.lang.Object other$cancelledDate = other.getCancelledDate();
if (this$cancelledDate == null ? other$cancelledDate != null : !this$cancelledDate.equals(other$cancelledDate)) return false;
final java.lang.Object this$cancelledBy = this.getCancelledBy();
final java.lang.Object other$cancelledBy = other.getCancelledBy();
if (this$cancelledBy == null ? other$cancelledBy != null : !this$cancelledBy.equals(other$cancelledBy)) return false;
final java.lang.Object this$lastUpdatedDate = this.getLastUpdatedDate();
final java.lang.Object other$lastUpdatedDate = other.getLastUpdatedDate();
if (this$lastUpdatedDate == null ? other$lastUpdatedDate != null : !this$lastUpdatedDate.equals(other$lastUpdatedDate)) return false;
final java.lang.Object this$lastUpdatedBy = this.getLastUpdatedBy();
final java.lang.Object other$lastUpdatedBy = other.getLastUpdatedBy();
if (this$lastUpdatedBy == null ? other$lastUpdatedBy != null : !this$lastUpdatedBy.equals(other$lastUpdatedBy)) return false;
final java.lang.Object this$firstSentDate = this.getFirstSentDate();
final java.lang.Object other$firstSentDate = other.getFirstSentDate();
if (this$firstSentDate == null ? other$firstSentDate != null : !this$firstSentDate.equals(other$firstSentDate)) return false;
final java.lang.Object this$lastSentDate = this.getLastSentDate();
final java.lang.Object other$lastSentDate = other.getLastSentDate();
if (this$lastSentDate == null ? other$lastSentDate != null : !this$lastSentDate.equals(other$lastSentDate)) return false;
final java.lang.Object this$lastSentBy = this.getLastSentBy();
final java.lang.Object other$lastSentBy = other.getLastSentBy();
if (this$lastSentBy == null ? other$lastSentBy != null : !this$lastSentBy.equals(other$lastSentBy)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof InvoicingMetaData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $createdDate = this.getCreatedDate();
result = result * PRIME + ($createdDate == null ? 43 : $createdDate.hashCode());
final java.lang.Object $createdBy = this.getCreatedBy();
result = result * PRIME + ($createdBy == null ? 43 : $createdBy.hashCode());
final java.lang.Object $cancelledDate = this.getCancelledDate();
result = result * PRIME + ($cancelledDate == null ? 43 : $cancelledDate.hashCode());
final java.lang.Object $cancelledBy = this.getCancelledBy();
result = result * PRIME + ($cancelledBy == null ? 43 : $cancelledBy.hashCode());
final java.lang.Object $lastUpdatedDate = this.getLastUpdatedDate();
result = result * PRIME + ($lastUpdatedDate == null ? 43 : $lastUpdatedDate.hashCode());
final java.lang.Object $lastUpdatedBy = this.getLastUpdatedBy();
result = result * PRIME + ($lastUpdatedBy == null ? 43 : $lastUpdatedBy.hashCode());
final java.lang.Object $firstSentDate = this.getFirstSentDate();
result = result * PRIME + ($firstSentDate == null ? 43 : $firstSentDate.hashCode());
final java.lang.Object $lastSentDate = this.getLastSentDate();
result = result * PRIME + ($lastSentDate == null ? 43 : $lastSentDate.hashCode());
final java.lang.Object $lastSentBy = this.getLastSentBy();
result = result * PRIME + ($lastSentBy == null ? 43 : $lastSentBy.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy