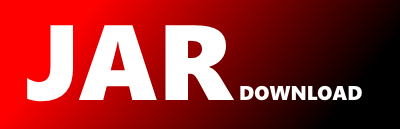
com.paypal.api.payments.InvoicingSearch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class InvoicingSearch extends PayPalModel {
/**
* Initial letters of the email address.
*/
private String email;
/**
* Initial letters of the recipient's first name.
*/
private String recipientFirstName;
/**
* Initial letters of the recipient's last name.
*/
private String recipientLastName;
/**
* Initial letters of the recipient's business name.
*/
private String recipientBusinessName;
/**
* The invoice number that appears on the invoice.
*/
private String number;
/**
* Status of the invoice.
*/
private String status;
/**
* Lower limit of total amount.
*/
private Currency lowerTotalAmount;
/**
* Upper limit of total amount.
*/
private Currency upperTotalAmount;
/**
* Start invoice date.
*/
private String startInvoiceDate;
/**
* End invoice date.
*/
private String endInvoiceDate;
/**
* Start invoice due date.
*/
private String startDueDate;
/**
* End invoice due date.
*/
private String endDueDate;
/**
* Start invoice payment date.
*/
private String startPaymentDate;
/**
* End invoice payment date.
*/
private String endPaymentDate;
/**
* Start invoice creation date.
*/
private String startCreationDate;
/**
* End invoice creation date.
*/
private String endCreationDate;
/**
* Offset of the search results.
*/
private float page;
/**
* Page size of the search results.
*/
private float pageSize;
/**
* A flag indicating whether total count is required in the response.
*/
private Boolean totalCountRequired;
/**
* Default Constructor
*/
public InvoicingSearch() {
}
/**
* Initial letters of the email address.
*/
@java.lang.SuppressWarnings("all")
public String getEmail() {
return this.email;
}
/**
* Initial letters of the recipient's first name.
*/
@java.lang.SuppressWarnings("all")
public String getRecipientFirstName() {
return this.recipientFirstName;
}
/**
* Initial letters of the recipient's last name.
*/
@java.lang.SuppressWarnings("all")
public String getRecipientLastName() {
return this.recipientLastName;
}
/**
* Initial letters of the recipient's business name.
*/
@java.lang.SuppressWarnings("all")
public String getRecipientBusinessName() {
return this.recipientBusinessName;
}
/**
* The invoice number that appears on the invoice.
*/
@java.lang.SuppressWarnings("all")
public String getNumber() {
return this.number;
}
/**
* Status of the invoice.
*/
@java.lang.SuppressWarnings("all")
public String getStatus() {
return this.status;
}
/**
* Lower limit of total amount.
*/
@java.lang.SuppressWarnings("all")
public Currency getLowerTotalAmount() {
return this.lowerTotalAmount;
}
/**
* Upper limit of total amount.
*/
@java.lang.SuppressWarnings("all")
public Currency getUpperTotalAmount() {
return this.upperTotalAmount;
}
/**
* Start invoice date.
*/
@java.lang.SuppressWarnings("all")
public String getStartInvoiceDate() {
return this.startInvoiceDate;
}
/**
* End invoice date.
*/
@java.lang.SuppressWarnings("all")
public String getEndInvoiceDate() {
return this.endInvoiceDate;
}
/**
* Start invoice due date.
*/
@java.lang.SuppressWarnings("all")
public String getStartDueDate() {
return this.startDueDate;
}
/**
* End invoice due date.
*/
@java.lang.SuppressWarnings("all")
public String getEndDueDate() {
return this.endDueDate;
}
/**
* Start invoice payment date.
*/
@java.lang.SuppressWarnings("all")
public String getStartPaymentDate() {
return this.startPaymentDate;
}
/**
* End invoice payment date.
*/
@java.lang.SuppressWarnings("all")
public String getEndPaymentDate() {
return this.endPaymentDate;
}
/**
* Start invoice creation date.
*/
@java.lang.SuppressWarnings("all")
public String getStartCreationDate() {
return this.startCreationDate;
}
/**
* End invoice creation date.
*/
@java.lang.SuppressWarnings("all")
public String getEndCreationDate() {
return this.endCreationDate;
}
/**
* Offset of the search results.
*/
@java.lang.SuppressWarnings("all")
public float getPage() {
return this.page;
}
/**
* Page size of the search results.
*/
@java.lang.SuppressWarnings("all")
public float getPageSize() {
return this.pageSize;
}
/**
* A flag indicating whether total count is required in the response.
*/
@java.lang.SuppressWarnings("all")
public Boolean getTotalCountRequired() {
return this.totalCountRequired;
}
/**
* Initial letters of the email address.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setEmail(final String email) {
this.email = email;
return this;
}
/**
* Initial letters of the recipient's first name.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setRecipientFirstName(final String recipientFirstName) {
this.recipientFirstName = recipientFirstName;
return this;
}
/**
* Initial letters of the recipient's last name.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setRecipientLastName(final String recipientLastName) {
this.recipientLastName = recipientLastName;
return this;
}
/**
* Initial letters of the recipient's business name.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setRecipientBusinessName(final String recipientBusinessName) {
this.recipientBusinessName = recipientBusinessName;
return this;
}
/**
* The invoice number that appears on the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setNumber(final String number) {
this.number = number;
return this;
}
/**
* Status of the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setStatus(final String status) {
this.status = status;
return this;
}
/**
* Lower limit of total amount.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setLowerTotalAmount(final Currency lowerTotalAmount) {
this.lowerTotalAmount = lowerTotalAmount;
return this;
}
/**
* Upper limit of total amount.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setUpperTotalAmount(final Currency upperTotalAmount) {
this.upperTotalAmount = upperTotalAmount;
return this;
}
/**
* Start invoice date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setStartInvoiceDate(final String startInvoiceDate) {
this.startInvoiceDate = startInvoiceDate;
return this;
}
/**
* End invoice date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setEndInvoiceDate(final String endInvoiceDate) {
this.endInvoiceDate = endInvoiceDate;
return this;
}
/**
* Start invoice due date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setStartDueDate(final String startDueDate) {
this.startDueDate = startDueDate;
return this;
}
/**
* End invoice due date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setEndDueDate(final String endDueDate) {
this.endDueDate = endDueDate;
return this;
}
/**
* Start invoice payment date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setStartPaymentDate(final String startPaymentDate) {
this.startPaymentDate = startPaymentDate;
return this;
}
/**
* End invoice payment date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setEndPaymentDate(final String endPaymentDate) {
this.endPaymentDate = endPaymentDate;
return this;
}
/**
* Start invoice creation date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setStartCreationDate(final String startCreationDate) {
this.startCreationDate = startCreationDate;
return this;
}
/**
* End invoice creation date.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setEndCreationDate(final String endCreationDate) {
this.endCreationDate = endCreationDate;
return this;
}
/**
* Offset of the search results.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setPage(final float page) {
this.page = page;
return this;
}
/**
* Page size of the search results.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setPageSize(final float pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A flag indicating whether total count is required in the response.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingSearch setTotalCountRequired(final Boolean totalCountRequired) {
this.totalCountRequired = totalCountRequired;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof InvoicingSearch)) return false;
final InvoicingSearch other = (InvoicingSearch) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$recipientFirstName = this.getRecipientFirstName();
final java.lang.Object other$recipientFirstName = other.getRecipientFirstName();
if (this$recipientFirstName == null ? other$recipientFirstName != null : !this$recipientFirstName.equals(other$recipientFirstName)) return false;
final java.lang.Object this$recipientLastName = this.getRecipientLastName();
final java.lang.Object other$recipientLastName = other.getRecipientLastName();
if (this$recipientLastName == null ? other$recipientLastName != null : !this$recipientLastName.equals(other$recipientLastName)) return false;
final java.lang.Object this$recipientBusinessName = this.getRecipientBusinessName();
final java.lang.Object other$recipientBusinessName = other.getRecipientBusinessName();
if (this$recipientBusinessName == null ? other$recipientBusinessName != null : !this$recipientBusinessName.equals(other$recipientBusinessName)) return false;
final java.lang.Object this$number = this.getNumber();
final java.lang.Object other$number = other.getNumber();
if (this$number == null ? other$number != null : !this$number.equals(other$number)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$lowerTotalAmount = this.getLowerTotalAmount();
final java.lang.Object other$lowerTotalAmount = other.getLowerTotalAmount();
if (this$lowerTotalAmount == null ? other$lowerTotalAmount != null : !this$lowerTotalAmount.equals(other$lowerTotalAmount)) return false;
final java.lang.Object this$upperTotalAmount = this.getUpperTotalAmount();
final java.lang.Object other$upperTotalAmount = other.getUpperTotalAmount();
if (this$upperTotalAmount == null ? other$upperTotalAmount != null : !this$upperTotalAmount.equals(other$upperTotalAmount)) return false;
final java.lang.Object this$startInvoiceDate = this.getStartInvoiceDate();
final java.lang.Object other$startInvoiceDate = other.getStartInvoiceDate();
if (this$startInvoiceDate == null ? other$startInvoiceDate != null : !this$startInvoiceDate.equals(other$startInvoiceDate)) return false;
final java.lang.Object this$endInvoiceDate = this.getEndInvoiceDate();
final java.lang.Object other$endInvoiceDate = other.getEndInvoiceDate();
if (this$endInvoiceDate == null ? other$endInvoiceDate != null : !this$endInvoiceDate.equals(other$endInvoiceDate)) return false;
final java.lang.Object this$startDueDate = this.getStartDueDate();
final java.lang.Object other$startDueDate = other.getStartDueDate();
if (this$startDueDate == null ? other$startDueDate != null : !this$startDueDate.equals(other$startDueDate)) return false;
final java.lang.Object this$endDueDate = this.getEndDueDate();
final java.lang.Object other$endDueDate = other.getEndDueDate();
if (this$endDueDate == null ? other$endDueDate != null : !this$endDueDate.equals(other$endDueDate)) return false;
final java.lang.Object this$startPaymentDate = this.getStartPaymentDate();
final java.lang.Object other$startPaymentDate = other.getStartPaymentDate();
if (this$startPaymentDate == null ? other$startPaymentDate != null : !this$startPaymentDate.equals(other$startPaymentDate)) return false;
final java.lang.Object this$endPaymentDate = this.getEndPaymentDate();
final java.lang.Object other$endPaymentDate = other.getEndPaymentDate();
if (this$endPaymentDate == null ? other$endPaymentDate != null : !this$endPaymentDate.equals(other$endPaymentDate)) return false;
final java.lang.Object this$startCreationDate = this.getStartCreationDate();
final java.lang.Object other$startCreationDate = other.getStartCreationDate();
if (this$startCreationDate == null ? other$startCreationDate != null : !this$startCreationDate.equals(other$startCreationDate)) return false;
final java.lang.Object this$endCreationDate = this.getEndCreationDate();
final java.lang.Object other$endCreationDate = other.getEndCreationDate();
if (this$endCreationDate == null ? other$endCreationDate != null : !this$endCreationDate.equals(other$endCreationDate)) return false;
if (java.lang.Float.compare(this.getPage(), other.getPage()) != 0) return false;
if (java.lang.Float.compare(this.getPageSize(), other.getPageSize()) != 0) return false;
final java.lang.Object this$totalCountRequired = this.getTotalCountRequired();
final java.lang.Object other$totalCountRequired = other.getTotalCountRequired();
if (this$totalCountRequired == null ? other$totalCountRequired != null : !this$totalCountRequired.equals(other$totalCountRequired)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof InvoicingSearch;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $recipientFirstName = this.getRecipientFirstName();
result = result * PRIME + ($recipientFirstName == null ? 43 : $recipientFirstName.hashCode());
final java.lang.Object $recipientLastName = this.getRecipientLastName();
result = result * PRIME + ($recipientLastName == null ? 43 : $recipientLastName.hashCode());
final java.lang.Object $recipientBusinessName = this.getRecipientBusinessName();
result = result * PRIME + ($recipientBusinessName == null ? 43 : $recipientBusinessName.hashCode());
final java.lang.Object $number = this.getNumber();
result = result * PRIME + ($number == null ? 43 : $number.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $lowerTotalAmount = this.getLowerTotalAmount();
result = result * PRIME + ($lowerTotalAmount == null ? 43 : $lowerTotalAmount.hashCode());
final java.lang.Object $upperTotalAmount = this.getUpperTotalAmount();
result = result * PRIME + ($upperTotalAmount == null ? 43 : $upperTotalAmount.hashCode());
final java.lang.Object $startInvoiceDate = this.getStartInvoiceDate();
result = result * PRIME + ($startInvoiceDate == null ? 43 : $startInvoiceDate.hashCode());
final java.lang.Object $endInvoiceDate = this.getEndInvoiceDate();
result = result * PRIME + ($endInvoiceDate == null ? 43 : $endInvoiceDate.hashCode());
final java.lang.Object $startDueDate = this.getStartDueDate();
result = result * PRIME + ($startDueDate == null ? 43 : $startDueDate.hashCode());
final java.lang.Object $endDueDate = this.getEndDueDate();
result = result * PRIME + ($endDueDate == null ? 43 : $endDueDate.hashCode());
final java.lang.Object $startPaymentDate = this.getStartPaymentDate();
result = result * PRIME + ($startPaymentDate == null ? 43 : $startPaymentDate.hashCode());
final java.lang.Object $endPaymentDate = this.getEndPaymentDate();
result = result * PRIME + ($endPaymentDate == null ? 43 : $endPaymentDate.hashCode());
final java.lang.Object $startCreationDate = this.getStartCreationDate();
result = result * PRIME + ($startCreationDate == null ? 43 : $startCreationDate.hashCode());
final java.lang.Object $endCreationDate = this.getEndCreationDate();
result = result * PRIME + ($endCreationDate == null ? 43 : $endCreationDate.hashCode());
result = result * PRIME + java.lang.Float.floatToIntBits(this.getPage());
result = result * PRIME + java.lang.Float.floatToIntBits(this.getPageSize());
final java.lang.Object $totalCountRequired = this.getTotalCountRequired();
result = result * PRIME + ($totalCountRequired == null ? 43 : $totalCountRequired.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy