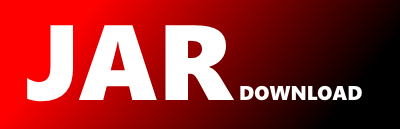
com.paypal.api.payments.Order Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.liferay.commerce.payment.method.paypal
Show all versions of com.liferay.commerce.payment.method.paypal
Liferay Commerce Payment Method PayPal
The newest version!
// Generated by delombok at Thu Nov 16 13:48:05 CST 2017
package com.paypal.api.payments;
import com.paypal.base.rest.*;
import java.util.List;
public class Order extends PayPalResource {
/**
* Identifier of the order transaction.
*/
private String id;
/**
* Identifier to the purchase unit associated with this object. Obsolete. Use one in cart_base.
*/
private String purchaseUnitReferenceId;
/**
* @deprecated Use {@link #purchaseUnitReferenceId} instead
* Identifier to the purchase unit associated with this object. Obsolete. Use one in cart_base.
*/
@Deprecated
private String referenceId;
/**
* Amount being collected.
*/
private Amount amount;
/**
* specifies payment mode of the transaction
*/
private String paymentMode;
/**
* State of the order transaction.
*/
private String state;
/**
* Reason code for the transaction state being Pending or Reversed. This field will replace pending_reason field eventually. Only supported when the `payment_method` is set to `paypal`.
*/
private String reasonCode;
/**
* @deprecated Reason code for the transaction state being Pending. Obsolete. Retained for backward compatability. Use reason_code field above instead.
*/
@Deprecated
private String pendingReason;
/**
* The level of seller protection in force for the transaction.
*/
private String protectionEligibility;
/**
* The kind of seller protection in force for the transaction. This property is returned only when the `protection_eligibility` property is set to `ELIGIBLE`or `PARTIALLY_ELIGIBLE`. Only supported when the `payment_method` is set to `paypal`. Allowed values:
`ITEM_NOT_RECEIVED_ELIGIBLE`- Sellers are protected against claims for items not received.
`UNAUTHORIZED_PAYMENT_ELIGIBLE`- Sellers are protected against claims for unauthorized payments.
One or both of the allowed values can be returned.
*/
private String protectionEligibilityType;
/**
* ID of the Payment resource that this transaction is based on.
*/
private String parentPayment;
/**
* Fraud Management Filter (FMF) details applied for the payment that could result in accept/deny/pending action.
*/
private FmfDetails fmfDetails;
/**
* Time the resource was created in UTC ISO8601 format.
*/
private String createTime;
/**
* Time the resource was last updated in UTC ISO8601 format.
*/
private String updateTime;
/**
*/
private List links;
/**
* Default Constructor
*/
public Order() {
}
/**
* Parameterized Constructor
*/
public Order(Amount amount) {
this.amount = amount;
}
/**
* Shows details for an order, by ID.
* @deprecated Please use {@link #get(APIContext, String)} instead.
* @param accessToken
* Access Token used for the API call.
* @param orderId
* String
* @return Order
* @throws PayPalRESTException
*/
public static Order get(String accessToken, String orderId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, orderId);
}
/**
* Shows details for an order, by ID.
* @param apiContext
* {@link APIContext} used for the API call.
* @param orderId
* String
* @return Order
* @throws PayPalRESTException
*/
public static Order get(APIContext apiContext, String orderId) throws PayPalRESTException {
if (orderId == null) {
throw new IllegalArgumentException("orderId cannot be null");
}
Object[] parameters = new Object[] {orderId};
String pattern = "v1/payments/orders/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Order.class);
}
/**
* Captures a payment for an order, by ID. To use this call, the original payment call must specify an intent of `order`. In the JSON request body, include the payment amount and indicate whether this capture is the final capture for the authorization.
* @deprecated Please use {@link #capture(APIContext, Capture)} instead.
* @param accessToken
* Access Token used for the API call.
* @param capture
* Capture
* @return Capture
* @throws PayPalRESTException
*/
public Capture capture(String accessToken, Capture capture) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return capture(apiContext, capture);
}
/**
* Captures a payment for an order, by ID. To use this call, the original payment call must specify an intent of `order`. In the JSON request body, include the payment amount and indicate whether this capture is the final capture for the authorization.
* @param apiContext
* {@link APIContext} used for the API call.
* @param capture
* Capture
* @return Capture
* @throws PayPalRESTException
*/
public Capture capture(APIContext apiContext, Capture capture) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (capture == null) {
throw new IllegalArgumentException("capture cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/orders/{0}/capture";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = capture.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Capture.class);
}
/**
* Voids, or cancels, an order, by ID. You cannot void an order if a payment has already been partially or fully captured.
* @deprecated Please use {@link #doVoid(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return Order
* @throws PayPalRESTException
*/
public Order doVoid(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return doVoid(apiContext);
}
/**
* Voids, or cancels, an order, by ID. You cannot void an order if a payment has already been partially or fully captured.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Order
* @throws PayPalRESTException
*/
public Order doVoid(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/orders/{0}/do-void";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Order.class);
}
/**
* Authorizes an order, by ID. Include an `amount` object in the JSON request body.
* @deprecated Please use {@link #authorize(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return Authorization
* @throws PayPalRESTException
*/
public Authorization authorize(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return authorize(apiContext);
}
/**
* Authorizes an order, by ID. Include an `amount` object in the JSON request body.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Authorization
* @throws PayPalRESTException
*/
public Authorization authorize(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/payments/orders/{0}/authorize";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Authorization.class);
}
/**
* Identifier of the order transaction.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* Identifier to the purchase unit associated with this object. Obsolete. Use one in cart_base.
*/
@java.lang.SuppressWarnings("all")
public String getPurchaseUnitReferenceId() {
return this.purchaseUnitReferenceId;
}
/**
* @deprecated Use {@link #purchaseUnitReferenceId} instead
* Identifier to the purchase unit associated with this object. Obsolete. Use one in cart_base.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public String getReferenceId() {
return this.referenceId;
}
/**
* Amount being collected.
*/
@java.lang.SuppressWarnings("all")
public Amount getAmount() {
return this.amount;
}
/**
* specifies payment mode of the transaction
*/
@java.lang.SuppressWarnings("all")
public String getPaymentMode() {
return this.paymentMode;
}
/**
* State of the order transaction.
*/
@java.lang.SuppressWarnings("all")
public String getState() {
return this.state;
}
/**
* Reason code for the transaction state being Pending or Reversed. This field will replace pending_reason field eventually. Only supported when the `payment_method` is set to `paypal`.
*/
@java.lang.SuppressWarnings("all")
public String getReasonCode() {
return this.reasonCode;
}
/**
* @deprecated Reason code for the transaction state being Pending. Obsolete. Retained for backward compatability. Use reason_code field above instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public String getPendingReason() {
return this.pendingReason;
}
/**
* The level of seller protection in force for the transaction.
*/
@java.lang.SuppressWarnings("all")
public String getProtectionEligibility() {
return this.protectionEligibility;
}
/**
* The kind of seller protection in force for the transaction. This property is returned only when the `protection_eligibility` property is set to `ELIGIBLE`or `PARTIALLY_ELIGIBLE`. Only supported when the `payment_method` is set to `paypal`. Allowed values:
`ITEM_NOT_RECEIVED_ELIGIBLE`- Sellers are protected against claims for items not received.
`UNAUTHORIZED_PAYMENT_ELIGIBLE`- Sellers are protected against claims for unauthorized payments.
One or both of the allowed values can be returned.
*/
@java.lang.SuppressWarnings("all")
public String getProtectionEligibilityType() {
return this.protectionEligibilityType;
}
/**
* ID of the Payment resource that this transaction is based on.
*/
@java.lang.SuppressWarnings("all")
public String getParentPayment() {
return this.parentPayment;
}
/**
* Fraud Management Filter (FMF) details applied for the payment that could result in accept/deny/pending action.
*/
@java.lang.SuppressWarnings("all")
public FmfDetails getFmfDetails() {
return this.fmfDetails;
}
/**
* Time the resource was created in UTC ISO8601 format.
*/
@java.lang.SuppressWarnings("all")
public String getCreateTime() {
return this.createTime;
}
/**
* Time the resource was last updated in UTC ISO8601 format.
*/
@java.lang.SuppressWarnings("all")
public String getUpdateTime() {
return this.updateTime;
}
/**
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* Identifier of the order transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setId(final String id) {
this.id = id;
return this;
}
/**
* Identifier to the purchase unit associated with this object. Obsolete. Use one in cart_base.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setPurchaseUnitReferenceId(final String purchaseUnitReferenceId) {
this.purchaseUnitReferenceId = purchaseUnitReferenceId;
return this;
}
/**
* @deprecated Use {@link #purchaseUnitReferenceId} instead
* Identifier to the purchase unit associated with this object. Obsolete. Use one in cart_base.
* @return this
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Order setReferenceId(final String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Amount being collected.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setAmount(final Amount amount) {
this.amount = amount;
return this;
}
/**
* specifies payment mode of the transaction
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setPaymentMode(final String paymentMode) {
this.paymentMode = paymentMode;
return this;
}
/**
* State of the order transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setState(final String state) {
this.state = state;
return this;
}
/**
* Reason code for the transaction state being Pending or Reversed. This field will replace pending_reason field eventually. Only supported when the `payment_method` is set to `paypal`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setReasonCode(final String reasonCode) {
this.reasonCode = reasonCode;
return this;
}
/**
* @deprecated Reason code for the transaction state being Pending. Obsolete. Retained for backward compatability. Use reason_code field above instead.
* @return this
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Order setPendingReason(final String pendingReason) {
this.pendingReason = pendingReason;
return this;
}
/**
* The level of seller protection in force for the transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setProtectionEligibility(final String protectionEligibility) {
this.protectionEligibility = protectionEligibility;
return this;
}
/**
* The kind of seller protection in force for the transaction. This property is returned only when the `protection_eligibility` property is set to `ELIGIBLE`or `PARTIALLY_ELIGIBLE`. Only supported when the `payment_method` is set to `paypal`. Allowed values:
`ITEM_NOT_RECEIVED_ELIGIBLE`- Sellers are protected against claims for items not received.
`UNAUTHORIZED_PAYMENT_ELIGIBLE`- Sellers are protected against claims for unauthorized payments.
One or both of the allowed values can be returned.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setProtectionEligibilityType(final String protectionEligibilityType) {
this.protectionEligibilityType = protectionEligibilityType;
return this;
}
/**
* ID of the Payment resource that this transaction is based on.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setParentPayment(final String parentPayment) {
this.parentPayment = parentPayment;
return this;
}
/**
* Fraud Management Filter (FMF) details applied for the payment that could result in accept/deny/pending action.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setFmfDetails(final FmfDetails fmfDetails) {
this.fmfDetails = fmfDetails;
return this;
}
/**
* Time the resource was created in UTC ISO8601 format.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setCreateTime(final String createTime) {
this.createTime = createTime;
return this;
}
/**
* Time the resource was last updated in UTC ISO8601 format.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setUpdateTime(final String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public Order setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Order)) return false;
final Order other = (Order) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$purchaseUnitReferenceId = this.getPurchaseUnitReferenceId();
final java.lang.Object other$purchaseUnitReferenceId = other.getPurchaseUnitReferenceId();
if (this$purchaseUnitReferenceId == null ? other$purchaseUnitReferenceId != null : !this$purchaseUnitReferenceId.equals(other$purchaseUnitReferenceId)) return false;
final java.lang.Object this$referenceId = this.getReferenceId();
final java.lang.Object other$referenceId = other.getReferenceId();
if (this$referenceId == null ? other$referenceId != null : !this$referenceId.equals(other$referenceId)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$paymentMode = this.getPaymentMode();
final java.lang.Object other$paymentMode = other.getPaymentMode();
if (this$paymentMode == null ? other$paymentMode != null : !this$paymentMode.equals(other$paymentMode)) return false;
final java.lang.Object this$state = this.getState();
final java.lang.Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
final java.lang.Object this$reasonCode = this.getReasonCode();
final java.lang.Object other$reasonCode = other.getReasonCode();
if (this$reasonCode == null ? other$reasonCode != null : !this$reasonCode.equals(other$reasonCode)) return false;
final java.lang.Object this$pendingReason = this.getPendingReason();
final java.lang.Object other$pendingReason = other.getPendingReason();
if (this$pendingReason == null ? other$pendingReason != null : !this$pendingReason.equals(other$pendingReason)) return false;
final java.lang.Object this$protectionEligibility = this.getProtectionEligibility();
final java.lang.Object other$protectionEligibility = other.getProtectionEligibility();
if (this$protectionEligibility == null ? other$protectionEligibility != null : !this$protectionEligibility.equals(other$protectionEligibility)) return false;
final java.lang.Object this$protectionEligibilityType = this.getProtectionEligibilityType();
final java.lang.Object other$protectionEligibilityType = other.getProtectionEligibilityType();
if (this$protectionEligibilityType == null ? other$protectionEligibilityType != null : !this$protectionEligibilityType.equals(other$protectionEligibilityType)) return false;
final java.lang.Object this$parentPayment = this.getParentPayment();
final java.lang.Object other$parentPayment = other.getParentPayment();
if (this$parentPayment == null ? other$parentPayment != null : !this$parentPayment.equals(other$parentPayment)) return false;
final java.lang.Object this$fmfDetails = this.getFmfDetails();
final java.lang.Object other$fmfDetails = other.getFmfDetails();
if (this$fmfDetails == null ? other$fmfDetails != null : !this$fmfDetails.equals(other$fmfDetails)) return false;
final java.lang.Object this$createTime = this.getCreateTime();
final java.lang.Object other$createTime = other.getCreateTime();
if (this$createTime == null ? other$createTime != null : !this$createTime.equals(other$createTime)) return false;
final java.lang.Object this$updateTime = this.getUpdateTime();
final java.lang.Object other$updateTime = other.getUpdateTime();
if (this$updateTime == null ? other$updateTime != null : !this$updateTime.equals(other$updateTime)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Order;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $purchaseUnitReferenceId = this.getPurchaseUnitReferenceId();
result = result * PRIME + ($purchaseUnitReferenceId == null ? 43 : $purchaseUnitReferenceId.hashCode());
final java.lang.Object $referenceId = this.getReferenceId();
result = result * PRIME + ($referenceId == null ? 43 : $referenceId.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $paymentMode = this.getPaymentMode();
result = result * PRIME + ($paymentMode == null ? 43 : $paymentMode.hashCode());
final java.lang.Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
final java.lang.Object $reasonCode = this.getReasonCode();
result = result * PRIME + ($reasonCode == null ? 43 : $reasonCode.hashCode());
final java.lang.Object $pendingReason = this.getPendingReason();
result = result * PRIME + ($pendingReason == null ? 43 : $pendingReason.hashCode());
final java.lang.Object $protectionEligibility = this.getProtectionEligibility();
result = result * PRIME + ($protectionEligibility == null ? 43 : $protectionEligibility.hashCode());
final java.lang.Object $protectionEligibilityType = this.getProtectionEligibilityType();
result = result * PRIME + ($protectionEligibilityType == null ? 43 : $protectionEligibilityType.hashCode());
final java.lang.Object $parentPayment = this.getParentPayment();
result = result * PRIME + ($parentPayment == null ? 43 : $parentPayment.hashCode());
final java.lang.Object $fmfDetails = this.getFmfDetails();
result = result * PRIME + ($fmfDetails == null ? 43 : $fmfDetails.hashCode());
final java.lang.Object $createTime = this.getCreateTime();
result = result * PRIME + ($createTime == null ? 43 : $createTime.hashCode());
final java.lang.Object $updateTime = this.getUpdateTime();
result = result * PRIME + ($updateTime == null ? 43 : $updateTime.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy